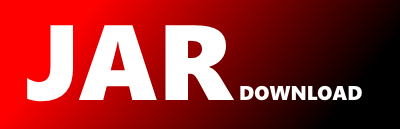
com.gargoylesoftware.htmlunit.javascript.host.Screen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vaadin-client-compiler-deps Show documentation
Show all versions of vaadin-client-compiler-deps Show documentation
Vaadin is a web application framework for Rich Internet Applications (RIA).
Vaadin enables easy development and maintenance of fast and
secure rich web
applications with a stunning look and feel and a wide browser support.
It features a server-side architecture with the majority of the logic
running
on the server. Ajax technology is used at the browser-side to ensure a
rich
and interactive user experience.
/*
* Copyright (c) 2002-2011 Gargoyle Software Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gargoylesoftware.htmlunit.javascript.host;
import com.gargoylesoftware.htmlunit.javascript.SimpleScriptable;
/**
* A JavaScript object for a Screen. Combines properties from both Mozilla's DOM
* and IE's DOM.
*
* @version $Revision: 6204 $
* @author Mike Bowler
* @author Daniel Gredler
* @author Chris Erskine
*
* @see
* MSDN documentation
* @see Mozilla documentation
*/
public class Screen extends SimpleScriptable {
private int left_;
private int top_;
private int width_;
private int height_;
private int colorDepth_;
private int bufferDepth_;
private int dpi_;
private boolean fontSmoothingEnabled_;
private int updateInterval_;
/**
* Creates an instance. JavaScript objects must have a default constructor.
*/
public Screen() {
left_ = 0;
top_ = 0;
width_ = 1024;
height_ = 768;
colorDepth_ = 24;
bufferDepth_ = 24;
dpi_ = 96;
fontSmoothingEnabled_ = true;
updateInterval_ = 0;
}
/**
* Returns the availHeight property.
* @return the availHeight property
*/
public int jsxGet_availHeight() {
return height_;
}
/**
* Returns the availLeft property.
* @return the availLeft property
*/
public int jsxGet_availLeft() {
return left_;
}
/**
* Returns the availTop property.
* @return the availTop property
*/
public int jsxGet_availTop() {
return top_;
}
/**
* Returns the availWidth property.
* @return the availWidth property
*/
public int jsxGet_availWidth() {
return width_;
}
/**
* Returns the bufferDepth property.
* @return the bufferDepth property
*/
public int jsxGet_bufferDepth() {
return bufferDepth_;
}
/**
* Sets the bufferDepth property.
* @param bufferDepth the bufferDepth property
*/
public void jsxSet_bufferDepth(final int bufferDepth) {
bufferDepth_ = bufferDepth;
}
/**
* Returns the colorDepth property.
* @return the colorDepth property
*/
public int jsxGet_colorDepth() {
return colorDepth_;
}
/**
* Returns the deviceXDPI property.
* @return the deviceXDPI property
*/
public int jsxGet_deviceXDPI() {
return dpi_;
}
/**
* Returns the deviceYDPI property.
* @return the deviceYDPI property
*/
public int jsxGet_deviceYDPI() {
return dpi_;
}
/**
* Returns the fontSmoothingEnabled property.
* @return the fontSmoothingEnabled property
*/
public boolean jsxGet_fontSmoothingEnabled() {
return fontSmoothingEnabled_;
}
/**
* Returns the height property.
* @return the height property
*/
public int jsxGet_height() {
return height_;
}
/**
* Returns the left property.
* @return the left property
*/
public int jsxGet_left() {
return left_;
}
/**
* Sets the left property.
* @param left the left property
*/
public void jsxSet_left(final int left) {
left_ = left;
}
/**
* Returns the logicalXDPI property.
* @return the logicalXDPI property
*/
public int jsxGet_logicalXDPI() {
return dpi_;
}
/**
* Returns the logicalYDPI property.
* @return the logicalYDPI property
*/
public int jsxGet_logicalYDPI() {
return dpi_;
}
/**
* Returns the pixelDepth property.
* @return the pixelDepth property
*/
public int jsxGet_pixelDepth() {
return colorDepth_;
}
/**
* Returns the top property.
* @return the top property
*/
public int jsxGet_top() {
return top_;
}
/**
* Sets the top property.
* @param top the top property
*/
public void jsxSet_top(final int top) {
top_ = top;
}
/**
* Returns the updateInterval property.
* @return the updateInterval property
*/
public int jsxGet_updateInterval() {
return updateInterval_;
}
/**
* Sets the updateInterval property.
* @param updateInterval the updateInterval property
*/
public void jsxSet_updateInterval(final int updateInterval) {
updateInterval_ = updateInterval;
}
/**
* Returns the width property.
* @return the width property
*/
public int jsxGet_width() {
return width_;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy