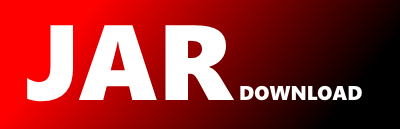
com.gargoylesoftware.htmlunit.CookieManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vaadin-client-compiler-deps Show documentation
Show all versions of vaadin-client-compiler-deps Show documentation
Vaadin is a web application framework for Rich Internet Applications (RIA).
Vaadin enables easy development and maintenance of fast and
secure rich web
applications with a stunning look and feel and a wide browser support.
It features a server-side architecture with the majority of the logic
running
on the server. Ajax technology is used at the browser-side to ensure a
rich
and interactive user experience.
/*
* Copyright (c) 2002-2011 Gargoyle Software Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gargoylesoftware.htmlunit;
import java.io.Serializable;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.apache.commons.collections.set.ListOrderedSet;
import org.apache.commons.lang.StringUtils;
import org.apache.http.client.CookieStore;
import org.apache.http.client.params.CookiePolicy;
import org.apache.http.cookie.CookieOrigin;
import org.apache.http.cookie.CookieSpec;
import org.apache.http.cookie.CookieSpecRegistry;
import org.apache.http.impl.client.DefaultHttpClient;
import com.gargoylesoftware.htmlunit.util.Cookie;
/**
* Manages cookies for a {@link WebClient}. This class is thread-safe.
*
* @version $Revision: 6373 $
* @author Daniel Gredler
* @author Ahmed Ashour
* @author Nicolas Belisle
*/
public class CookieManager implements Serializable {
/**
* HtmlUnit's cookie policy is to be browser-compatible. Code which requires access to
* HtmlUnit's cookie policy should use this constant, rather than making assumptions and using
* one of the HttpClient {@link CookiePolicy} constants directly.
*/
public static final String HTMLUNIT_COOKIE_POLICY = CookiePolicy.BROWSER_COMPATIBILITY;
/** Whether or not cookies are enabled. */
private boolean cookiesEnabled_;
/** The cookies added to this cookie manager. */
@SuppressWarnings("unchecked")
private final Set cookies_ = new ListOrderedSet();
/** The cookies spec registry */
private final transient CookieSpecRegistry registry_ = new DefaultHttpClient().getCookieSpecs();
/**
* Creates a new instance.
*/
public CookieManager() {
cookiesEnabled_ = true;
}
/**
* Enables/disables cookie support. Cookies are enabled by default.
* @param enabled true to enable cookie support, false otherwise
*/
public synchronized void setCookiesEnabled(final boolean enabled) {
cookiesEnabled_ = enabled;
}
/**
* Returns true if cookies are enabled. Cookies are enabled by default.
* @return true if cookies are enabled, false otherwise
*/
public synchronized boolean isCookiesEnabled() {
return cookiesEnabled_;
}
/**
* Returns the currently configured cookies, in an unmodifiable set.
* @return the currently configured cookies, in an unmodifiable set
*/
public synchronized Set getCookies() {
return Collections.unmodifiableSet(cookies_);
}
/**
* Returns the currently configured cookies applicable to the specified URL, in an unmodifiable set.
* @param url the URL on which to filter the returned cookies
* @return the currently configured cookies applicable to the specified URL, in an unmodifiable set
*/
public synchronized Set getCookies(final URL url) {
final String host = url.getHost();
final String path = url.getPath();
final String protocol = url.getProtocol();
final boolean secure = "https".equals(protocol);
// URLs like "about:blank" don't have cookies and we need to catch these
// cases here before HttpClient complains
if (host.length() == 0) {
return Collections.emptySet();
}
final int port = getPort(url);
// discard expired cookies
final Date now = new Date();
for (final Iterator iter = cookies_.iterator(); iter.hasNext();) {
final Cookie cookie = iter.next();
if (cookie.getExpires() != null && now.after(cookie.getExpires())) {
iter.remove();
}
}
final CookieSpec spec = registry_.getCookieSpec(HTMLUNIT_COOKIE_POLICY);
final org.apache.http.cookie.Cookie[] all = Cookie.toHttpClient(cookies_);
final CookieOrigin cookieOrigin = new CookieOrigin(host, port, path, secure);
final List matches = new ArrayList();
for (final org.apache.http.cookie.Cookie cookie : all) {
if (spec.match(cookie, cookieOrigin)) {
matches.add(cookie);
}
}
final Set cookies = new LinkedHashSet();
cookies.addAll(Cookie.fromHttpClient(matches));
return Collections.unmodifiableSet(cookies);
}
/**
* Gets the port of the URL.
* This functionality is implemented here as protected method to allow subclass to change it
* as workaround to © 2015 - 2025 Weber Informatics LLC | Privacy Policy