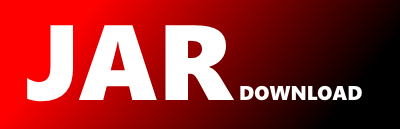
com.vaadin.gradle.PrepareFrontendInputProperties.kt Maven / Gradle / Ivy
/**
* Copyright 2000-2023 Vaadin Ltd
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.vaadin.gradle
import com.vaadin.flow.server.frontend.FrontendTools
import com.vaadin.flow.server.frontend.FrontendToolsSettings
import com.vaadin.flow.server.frontend.FrontendUtils
import org.gradle.api.tasks.Input
import org.gradle.api.tasks.InputDirectory
import org.gradle.api.tasks.InputFile
import org.gradle.api.tasks.Optional
import org.gradle.api.tasks.PathSensitive
import org.gradle.api.tasks.PathSensitivity
import java.io.File
import java.net.URI
import com.vaadin.experimental.FeatureFlags
import org.gradle.api.provider.ListProperty
import org.gradle.api.provider.Provider
/**
* Declaratively defines the inputs of the [VaadinPrepareFrontendTask]:
* configurable parameters, Node.js and npm/pnpm versions. Being used for
* caching the results of vaadinPrepareFrontend task to not run it again if
* inputs are the same.
*/
internal class PrepareFrontendInputProperties(private val config: PluginEffectiveConfiguration) {
private val tools: Provider = initialiseFrontendToolsSettings()
@Input
public fun getProductionMode(): Provider = config.productionMode
@Input
@Optional
public fun getWebpackOutputDirectory(): Provider = config.webpackOutputDirectory
.filterExists()
.absolutePath
@Input
public fun getNpmFolder(): Provider = config.npmFolder.absolutePath
@Input
public fun getFrontendDirectory(): Provider = config.frontendDirectory.absolutePath
@Input
public fun getGenerateBundle(): Provider = config.generateBundle
@Input
public fun getRunNpmInstall(): Provider = config.runNpmInstall
@Input
public fun getGenerateEmbeddableWebComponent(): Provider = config.generateEmbeddableWebComponents
@InputDirectory
@Optional
@PathSensitive(PathSensitivity.ABSOLUTE)
public fun getFrontendResourcesDirectory(): Provider = config.frontendResourcesDirectory.filterExists()
@Input
public fun getOptimizeBundle(): Provider = config.optimizeBundle
@Input
public fun getPnpmEnable(): Provider = config.pnpmEnable
@Input
public fun getUseGlobalPnpm(): Provider = config.useGlobalPnpm
@Input
public fun getRequireHomeNodeExec(): Provider = config.requireHomeNodeExec
@Input
public fun getEagerServerLoad(): Provider = config.eagerServerLoad
@InputFile
@Optional
@PathSensitive(PathSensitivity.NONE)
public fun getApplicationProperties(): Provider = config.applicationProperties.filterExists()
@InputFile
@Optional
@PathSensitive(PathSensitivity.ABSOLUTE)
public fun getOpenApiJsonFile(): Provider = config.openApiJsonFile.filterExists()
@InputFile
@Optional
@PathSensitive(PathSensitivity.ABSOLUTE)
public fun getFeatureFlagsFile(): Provider = config.javaResourceFolder
.map { it.resolve(FeatureFlags.PROPERTIES_FILENAME) }
.filterExists()
@Input
public fun getJavaSourceFolder(): Provider = config.javaSourceFolder.absolutePath
@Input
public fun getJavaResourceFolder(): Provider = config.javaResourceFolder.absolutePath
@Input
public fun getGeneratedTsFolder(): Provider = config.generatedTsFolder.absolutePath
@Input
public fun getNodeVersion(): Provider = config.nodeVersion
@Input
public fun getNodeDownloadRoot(): Provider = config.nodeDownloadRoot
@Input
public fun getNodeAutoUpdate(): Provider = config.nodeAutoUpdate
@Input
public fun getProjectBuildDir(): Provider = config.projectBuildDir
@Input
public fun getPostInstallPackages(): ListProperty = config.postinstallPackages
@Input
public fun getFrontendHotdeploy(): Provider = config.frontendHotdeploy
@Input
public fun getCiBuild(): Provider = config.ciBuild
@Input
public fun getSkipDevBundleBuild(): Provider = config.skipDevBundleBuild
@Input
public fun getForceProductionBuild(): Provider = config.forceProductionBuild
@Input
@Optional
public fun getNodeExecutablePath(): Provider = tools
.mapOrNull { it.nodeBinary }
.filterExists()
@Input
@Optional
public fun getNpmExecutablePath(): Provider = tools.map { tools ->
val npmExecutable = tools.npmExecutable ?: listOf()
npmExecutable.joinToString(" ")
}
@Input
@Optional
public fun getPnpmExecutablePath(): Provider = config.pnpmEnable.map { pnpmEnable ->
if (!pnpmEnable) {
return@map ""
}
val pnpmExecutable = tools.get().pnpmExecutable ?: listOf()
pnpmExecutable.joinToString(" ")
}
private fun initialiseFrontendToolsSettings(): Provider = config.npmFolder.map { npmFolder ->
val settings = FrontendToolsSettings(npmFolder.absolutePath) {
FrontendUtils.getVaadinHomeDirectory()
.absolutePath
}
settings.nodeDownloadRoot = URI(config.nodeDownloadRoot.get())
settings.isForceAlternativeNode = config.requireHomeNodeExec.get()
settings.isUseGlobalPnpm = config.useGlobalPnpm.get()
settings.isAutoUpdate = config.nodeAutoUpdate.get()
FrontendTools(settings)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy