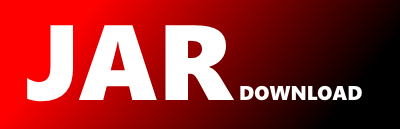
com.vaadin.sass.internal.visitor.IfElseNodeHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vaadin-sass-compiler Show documentation
Show all versions of vaadin-sass-compiler Show documentation
A pure Java implementation of the http://sass-lang.com compiler
/*
* Copyright 2000-2014 Vaadin Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.vaadin.sass.internal.visitor;
import java.util.ArrayList;
import java.util.List;
import org.w3c.flute.parser.ParseException;
import com.vaadin.sass.internal.ScssStylesheet;
import com.vaadin.sass.internal.expression.BinaryOperator;
import com.vaadin.sass.internal.parser.SassListItem;
import com.vaadin.sass.internal.tree.Node;
import com.vaadin.sass.internal.tree.controldirective.ElseNode;
import com.vaadin.sass.internal.tree.controldirective.IfElseDefNode;
import com.vaadin.sass.internal.tree.controldirective.IfNode;
public class IfElseNodeHandler {
public static void traverse(IfElseDefNode node) throws Exception {
for (final Node child : node.getChildren()) {
if (child instanceof IfNode) {
SassListItem expression = ((IfNode) child).getExpression();
expression = expression.replaceVariables(ScssStylesheet
.getVariables());
expression = expression.evaluateFunctionsAndExpressions(true);
if (BinaryOperator.isTrue(expression)) {
replaceDefNodeWithCorrectChild(node, child);
break;
}
} else {
if (!(child instanceof ElseNode)
&& node.getChildren().indexOf(child) == node
.getChildren().size() - 1) {
throw new ParseException(
"Invalid @if/@else in scss file for " + node);
} else {
replaceDefNodeWithCorrectChild(node, child);
break;
}
}
}
// in case the node hasn't been removed already
node.removeFromParent();
}
private static void replaceDefNodeWithCorrectChild(
IfElseDefNode ifElseNode, final Node child) {
List nodesToAdd = new ArrayList(child.getChildren());
ifElseNode.getParentNode().replaceNode(ifElseNode, nodesToAdd);
for (Node node : nodesToAdd) {
node.traverse();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy