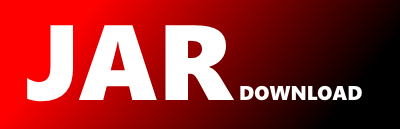
com.vaadin.event.SelectionEvent Maven / Gradle / Ivy
/*
* Vaadin Framework 7
*
* Copyright (C) 2000-2024 Vaadin Ltd
*
* This program is available under Vaadin Commercial License and Service Terms.
*
* See for the full
* license.
*/
package com.vaadin.event;
import java.io.Serializable;
import java.util.Collection;
import java.util.Collections;
import java.util.EventObject;
import java.util.LinkedHashSet;
import java.util.Set;
/**
* An event that specifies what in a selection has changed, and where the
* selection took place.
*
* @since 7.4
* @author Vaadin Ltd
*/
public class SelectionEvent extends EventObject {
private LinkedHashSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy