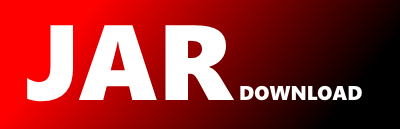
com.vaadin.data.util.converter.StringToEnumConverter Maven / Gradle / Ivy
/*
* Vaadin Framework 7
*
* Copyright (C) 2000-2024 Vaadin Ltd
*
* This program is available under Vaadin Commercial License and Service Terms.
*
* See for the full
* license.
*/
package com.vaadin.data.util.converter;
import java.util.EnumSet;
import java.util.Locale;
/**
* A converter that converts from {@link String} to an {@link Enum} and back.
*
* Designed to provide nice human readable strings for {@link Enum} classes
* conforming to one of these patterns:
*
* - The constants are named SOME_UPPERCASE_WORDS and there's no toString
* implementation.
* - toString() always returns the same human readable string that is not the
* same as its name() value. Each constant in the enum type returns a distinct
* toString() value.
*
* Will not necessarily work correctly for other cases.
*
*
* @author Vaadin Ltd
* @since 7.4
*/
public class StringToEnumConverter implements Converter {
@Override
public Enum convertToModel(String value, Class extends Enum> targetType,
Locale locale) throws ConversionException {
if (value == null || value.trim().equals("")) {
return null;
}
return stringToEnum(value, targetType, locale);
}
/**
* Converts the given string to the given enum type using the given locale
*
* Compatible with {@link #enumToString(Enum, Locale)}
*
* @param value
* The string value to convert
* @param enumType
* The type of enum to create
* @param locale
* The locale to use for conversion. If null, the JVM default
* locale will be used
* @return The enum which matches the given string
* @throws ConversionException
* if the conversion fails
*/
public static > T stringToEnum(String value,
Class enumType, Locale locale) throws ConversionException {
if (locale == null) {
locale = Locale.getDefault();
}
if (!enumType.isEnum()) {
throw new ConversionException(
enumType.getName() + " is not an enum type");
}
// First test for the human-readable value since that's the more likely
// input
String upperCaseValue = value.toUpperCase(locale);
T match = null;
for (T e : EnumSet.allOf(enumType)) {
String upperCase = enumToString(e, locale).toUpperCase(locale);
if (upperCase.equals(upperCaseValue)) {
if (match != null) {
throw new ConversionException("Both " + match.name()
+ " and " + e.name()
+ " are matching the input string " + value);
}
match = e;
}
}
if (match != null) {
return match;
}
// Then fall back to using a strict match based on name()
try {
return Enum.valueOf(enumType, upperCaseValue);
} catch (Exception ee) {
throw new ConversionException(ee);
}
}
/**
* Converts the given enum to a human readable string using the given locale
*
* Compatible with {@link #stringToEnum(String, Class, Locale)}
*
* @param value
* The enum value to convert
* @param locale
* The locale to use for conversion. If null, the JVM default
* locale will be used
* @return A human readable string based on the enum
* @throws ConversionException
* if the conversion fails
*/
public static String enumToString(Enum> value, Locale locale) {
if (locale == null) {
locale = Locale.getDefault();
}
String enumString = value.toString();
if (enumString.equals(value.name())) {
// FOO -> Foo
// FOO_BAR -> Foo bar
// _FOO -> _foo
String result = enumString.substring(0, 1).toUpperCase(locale);
result += enumString.substring(1).toLowerCase(locale).replace('_',
' ');
return result;
} else {
return enumString;
}
}
@Override
public String convertToPresentation(Enum value,
Class extends String> targetType, Locale locale)
throws ConversionException {
if (value == null) {
return null;
}
return enumToString(value, locale);
}
@Override
public Class getModelType() {
return Enum.class;
}
@Override
public Class getPresentationType() {
return String.class;
}
}