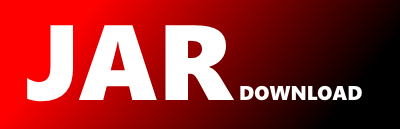
com.vaadin.data.BindingValidationStatus Maven / Gradle / Ivy
/*
* Copyright (C) 2000-2024 Vaadin Ltd
*
* This program is available under Vaadin Commercial License and Service Terms.
*
* See for the full
* license.
*/
package com.vaadin.data;
import java.io.Serializable;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import com.vaadin.data.Binder.Binding;
import com.vaadin.data.Binder.BindingBuilder;
/**
* Represents the status of field validation. Status can be {@code Status.OK},
* {@code Status.ERROR} or {@code Status.UNRESOLVED}. Status OK and ERROR are
* always associated with a ValidationResult {@link #getResult}.
*
* Use
* {@link BindingBuilder#withValidationStatusHandler(BindingValidationStatusHandler)}
* to register a handler for field level validation status changes.
*
* @author Vaadin Ltd
*
* @param
* the target data type of the binding for which the validation
* status changed, matches the field type unless a converter has been
* set
*
* @see BindingBuilder#withValidationStatusHandler(BindingValidationStatusHandler)
* @see Binding#validate()
* @see BindingValidationStatusHandler
* @see BinderValidationStatus
*
* @since 8.0
*/
public class BindingValidationStatus implements Serializable {
/**
* Status of the validation.
*
* The status is the part of {@link BindingValidationStatus} which indicates
* whether the validation failed or not, or whether it is in unresolved
* state (e.g. after clear or reset).
*/
public enum Status {
/** Validation passed. */
OK,
/** Validation failed. */
ERROR,
/**
* Unresolved status, e.g field has not yet been validated because value
* was cleared.
*
* In practice this status means that the value might be invalid, but
* validation errors should be hidden.
*/
UNRESOLVED;
}
private final Status status;
private final List results;
private final Binding, TARGET> binding;
private Result result;
/**
* Convenience method for creating a {@link Status#UNRESOLVED} validation
* status for the given binding.
*
* @param source
* the source binding
* @return unresolved validation status
* @param
* the target data type of the binding for which the validation
* status was reset
*/
public static BindingValidationStatus createUnresolvedStatus(
Binding, TARGET> source) {
return new BindingValidationStatus(null, source);
}
/**
* Creates a new validation status for the given binding and validation
* result.
*
* @param source
* the source binding
* @param result
* the result of the validation
*/
@Deprecated
public BindingValidationStatus(Binding, TARGET> source,
ValidationResult result) {
this(source, result.isError() ? Status.ERROR : Status.OK, result);
}
/**
* Creates a new status change event.
*
* The {@code message} must be {@code null} if the {@code status} is
* {@link Status#OK}.
*
* @param source
* field whose status has changed, not {@code null}
* @param status
* updated status value, not {@code null}
* @param result
* the related result, may be {@code null}
*/
@Deprecated
public BindingValidationStatus(Binding, TARGET> source, Status status,
ValidationResult result) {
this(result.isError() ? Result.error(result.getErrorMessage())
: Result.ok(null), source);
}
/**
* Creates a new status change event.
*
* If {@code result} is {@code null}, the {@code status} is
* {@link Status#UNRESOLVED}.
*
* @param result
* the related result object, may be {@code null}
* @param source
* field whose status has changed, not {@code null}
*
* @since 8.2
*/
public BindingValidationStatus(Result result,
Binding, TARGET> source) {
Objects.requireNonNull(source, "Event source may not be null");
binding = source;
if (result != null) {
this.status = result.isError() ? Status.ERROR : Status.OK;
if (result instanceof ValidationResultWrap) {
results = ((ValidationResultWrap) result)
.getValidationResults();
} else {
results = Collections.emptyList();
}
} else {
this.status = Status.UNRESOLVED;
results = Collections.emptyList();
}
this.result = result;
}
/**
* Gets status of the validation.
*
* @return status
*/
public Status getStatus() {
return status;
}
/**
* Gets whether the validation failed or not.
*
* @return {@code true} if validation failed, {@code false} if validation
* passed
*/
public boolean isError() {
return status == Status.ERROR;
}
/**
* Gets error validation message if status is {@link Status#ERROR}.
*
* @return an optional validation error status or an empty optional if
* status is not an error
*/
public Optional getMessage() {
if (getStatus() == Status.OK || result == null) {
return Optional.empty();
}
return result.getMessage();
}
/**
* Gets the validation result if status is either {@link Status#OK} or
* {@link Status#ERROR} or an empty optional if status is
* {@link Status#UNRESOLVED}.
*
* @return the validation result
*/
public Optional getResult() {
if (result == null) {
return Optional.empty();
}
return Optional.of(result.isError()
? ValidationResult.error(result.getMessage().orElse(""))
: ValidationResult.ok());
}
/**
* Gets all the validation results related to this binding validation
* status.
*
* @return list of validation results
*
* @since 8.2
*/
public List getValidationResults() {
return Collections.unmodifiableList(results);
}
/**
* Gets the source binding of the validation status.
*
* @return the source binding
*/
public Binding, TARGET> getBinding() {
return binding;
}
/**
* Gets the bound field for this status.
*
* @return the field
*/
public HasValue> getField() {
return getBinding().getField();
}
}