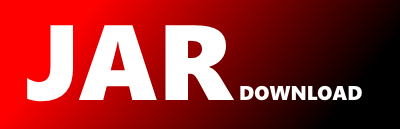
com.vaadin.data.provider.CallbackDataProvider Maven / Gradle / Ivy
/*
* Copyright (C) 2000-2024 Vaadin Ltd
*
* This program is available under Vaadin Commercial License and Service Terms.
*
* See for the full
* license.
*/
package com.vaadin.data.provider;
import java.io.Serializable;
import java.util.Objects;
import java.util.stream.Stream;
import com.vaadin.data.ValueProvider;
/**
* Data provider that uses one callback for fetching items from a back end and
* another callback for counting the number of available items.
*
* @author Vaadin Ltd
* @since 8.0
*
* @param
* data provider data type
* @param
* data provider filter type
*/
public class CallbackDataProvider
extends AbstractBackEndDataProvider {
/**
* Callback interface for fetching a stream of items from a backend based on
* a query.
*
* @param
* the type of the items to fetch
* @param
* the type of the optional filter in the query,
* Void
if filtering is not supported
*/
@FunctionalInterface
public interface FetchCallback extends Serializable {
/**
* Fetches a stream of items based on a query. The query defines the
* paging of the items to fetch through {@link Query#getOffset()} and
* {@link Query#getLimit()}, the sorting through
* {@link Query#getSortOrders()} and optionally also any filtering to
* use through {@link Query#getFilter()}.
*
* @param query
* the query that defines which items to fetch
* @return a stream of items
*/
public Stream fetch(Query query);
}
/**
* Callback interface for counting the number of items in a backend based on
* a query.
*
* @param
* the type of the items to count
* @param
* the type of the optional filter in the query,
* Void
if filtering is not supported
*/
@FunctionalInterface
public interface CountCallback extends Serializable {
/**
* Counts the number of available items based on a query. The query
* optionally defines any filtering to use through
* {@link Query#getFilter()}. The query also contains information about
* paging and sorting although that information is generally not
* applicable for determining the number of items.
*
* @param query
* the query that defines which items to count
* @return the number of available items
*/
public int count(Query query);
}
private final FetchCallback fetchCallback;
private final CountCallback countCallback;
private final ValueProvider idGetter;
/**
* Constructs a new DataProvider to request data using callbacks for
* fetching and counting items in the back end.
*
* @param fetchCallback
* function that returns a stream of items from the back end for
* a query
* @param countCallback
* function that return the number of items in the back end for a
* query
*
* @see #CallbackDataProvider(FetchCallback, CountCallback, ValueProvider)
*/
public CallbackDataProvider(FetchCallback fetchCallback,
CountCallback countCallback) {
this(fetchCallback, countCallback, t -> t);
}
/**
* Constructs a new DataProvider to request data using callbacks for
* fetching and counting items in the back end.
*
* @param fetchCallBack
* function that requests data from back end based on query
* @param countCallback
* function that returns the amount of data in back end for query
* @param identifierGetter
* function that returns the identifier for a given item
*/
public CallbackDataProvider(FetchCallback fetchCallBack,
CountCallback countCallback,
ValueProvider identifierGetter) {
Objects.requireNonNull(fetchCallBack, "Request function can't be null");
Objects.requireNonNull(countCallback, "Count callback can't be null");
Objects.requireNonNull(identifierGetter,
"Identifier getter function can't be null");
this.fetchCallback = fetchCallBack;
this.countCallback = countCallback;
this.idGetter = identifierGetter;
}
@Override
public Stream fetchFromBackEnd(Query query) {
return fetchCallback.fetch(query);
}
@Override
protected int sizeInBackEnd(Query query) {
return countCallback.count(query);
}
@Override
public Object getId(T item) {
Object itemId = idGetter.apply(item);
assert itemId != null : "CallbackDataProvider got null as an id for item: "
+ item;
return itemId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy