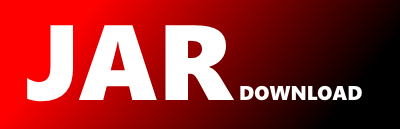
com.vaadin.flow.component.spreadsheet.IteratorChain Maven / Gradle / Ivy
/**
* Copyright 2000-2024 Vaadin Ltd.
*
* This program is available under Vaadin Commercial License and Service Terms.
*
* See {@literal } for the full
* license.
*/
package com.vaadin.flow.component.spreadsheet;
import java.io.Serializable;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.NoSuchElementException;
import java.util.Queue;
@SuppressWarnings("serial")
class IteratorChain implements Iterator, Serializable {
private static class EmptyIterator implements Iterator, Serializable {
@Override
public boolean hasNext() {
return false;
}
@Override
public E next() {
throw new NoSuchElementException();
}
@Override
public void remove() {
throw new UnsupportedOperationException("remove");
}
}
private final Queue> iteratorChain = new LinkedList>();
private Iterator extends E> currentIterator = null;
public IteratorChain(Collection> iterators) {
iteratorChain.addAll(iterators);
}
private void updateCurrentIterator() {
if (currentIterator == null) {
if (iteratorChain.isEmpty()) {
currentIterator = new EmptyIterator();
} else {
currentIterator = iteratorChain.remove();
}
}
while (currentIterator.hasNext() == false && !iteratorChain.isEmpty()) {
currentIterator = iteratorChain.remove();
}
}
@Override
public boolean hasNext() {
updateCurrentIterator();
return currentIterator.hasNext();
}
@Override
public E next() {
updateCurrentIterator();
return currentIterator.next();
}
@Override
public void remove() {
throw new UnsupportedOperationException("remove");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy