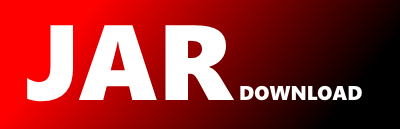
com.vaadin.flow.component.spreadsheet.shared.SpreadsheetState Maven / Gradle / Ivy
/**
* Copyright 2000-2024 Vaadin Ltd.
*
* This program is available under Vaadin Commercial License and Service Terms.
*
* See {@literal } for the full
* license.
*/
package com.vaadin.flow.component.spreadsheet.shared;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.vaadin.flow.component.spreadsheet.client.MergedRegion;
import com.vaadin.flow.component.spreadsheet.client.OverlayInfo;
@SuppressWarnings("serial")
public class SpreadsheetState {
// from TabIndexState
public int tabIndex = 0;
// from AbstractComponentState
public String height = "";
public String width = "";
public String description = "";
public ContentMode descriptionContentMode = ContentMode.PREFORMATTED;
// Note: for the caption, there is a difference between null and an empty
// string!
public String caption = null;
public List styles = null;
public String id = null;
public String primaryStyleName = null;
/** HTML formatted error message for the component. */
public String errorMessage = null;
/**
* Level of error.
*
* @since 8.2
*/
public ErrorLevel errorLevel = null;
public boolean captionAsHtml = false;
// from SaredState
public Map resources = new HashMap<>();
public boolean enabled = true;
/**
* A set of event identifiers with registered listeners.
*/
public Set registeredEventListeners;
// spreadsheetState
// @DelegateToWidget
public int rowBufferSize = 200;
// @DelegateToWidget
public int columnBufferSize = 200;
// @DelegateToWidget
public int rows;
// @DelegateToWidget
public int cols;
// @DelegateToWidget
public List colGroupingData;
// @DelegateToWidget
public List rowGroupingData;
// @DelegateToWidget
public int colGroupingMax;
// @DelegateToWidget
public int rowGroupingMax;
// @DelegateToWidget
public boolean colGroupingInversed;
// @DelegateToWidget
public boolean rowGroupingInversed;
// @DelegateToWidget
public float defRowH;
// @DelegateToWidget
public int defColW;
// @DelegateToWidget
public float[] rowH;
// @DelegateToWidget
public int[] colW;
/** should the sheet be reloaded on client side */
public boolean reload;
/** 1-based */
public int sheetIndex = 1;
public String[] sheetNames = null;
// @DelegateToWidget
public HashMap cellStyleToCSSStyle = null;
// @DelegateToWidget
public HashMap rowIndexToStyleIndex = null;
// @DelegateToWidget
public HashMap columnIndexToStyleIndex = null;
// @DelegateToWidget
public Set lockedColumnIndexes = null;
// @DelegateToWidget
public Set lockedRowIndexes = null;
// @DelegateToWidget
public ArrayList shiftedCellBorderStyles = null;
/**
* All conditional formatting styles for this sheet.
*/
// @DelegateToWidget
public HashMap conditionalFormattingStyles = null;
/** 1-based */
// @DelegateToWidget
public ArrayList hiddenColumnIndexes = null;
/** 1-based */
// @DelegateToWidget
public ArrayList hiddenRowIndexes = null;
// @DelegateToWidget
public int[] verticalScrollPositions;
// @DelegateToWidget
public int[] horizontalScrollPositions;
public boolean sheetProtected;
// @DelegateToWidget
public boolean workbookProtected;
public HashMap cellKeysToEditorIdMap;
public HashMap componentIDtoCellKeysMap;
// Cell CSS key to link tooltip (usually same as address)
// @DelegateToWidget
public HashMap hyperlinksTooltips;
public HashMap cellComments;
public HashMap cellCommentAuthors;
public ArrayList visibleCellComments;
public Set invalidFormulaCells;
public boolean hasActions;
public HashMap overlays;
public ArrayList mergedRegions;
// @DelegateToWidget
public boolean displayGridlines = true;
// @DelegateToWidget
public boolean displayRowColHeadings = true;
// @DelegateToWidget
public int verticalSplitPosition = 0;
// @DelegateToWidget
public int horizontalSplitPosition = 0;
// @DelegateToWidget
public String infoLabelValue;
public boolean workbookChangeToggle;
// @DelegateToWidget
public String invalidFormulaErrorMessage = "Invalid formula";
// @DelegateToWidget
public boolean lockFormatColumns = true;
// @DelegateToWidget
public boolean lockFormatRows = true;
// @DelegateToWidget
public List namedRanges;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy