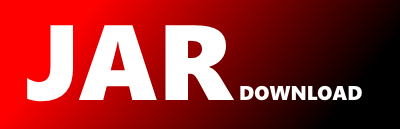
com.vaadin.flow.component.tabs.GeneratedVaadinTab Maven / Gradle / Ivy
/*
* Copyright 2000-2017 Vaadin Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.vaadin.flow.component.tabs;
import javax.annotation.Generated;
import com.vaadin.flow.component.Tag;
import com.vaadin.flow.component.dependency.HtmlImport;
import com.vaadin.flow.component.HasStyle;
import com.vaadin.flow.component.Component;
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* {@code } is a Polymer 2 element providing an accessible and
* customizable tab.
*
*
* {@code
Tab 1
}
*
*
* The following state attributes are available for styling:
*
*
*
*
* Attribute
* Description
* Part name
*
*
*
* {@code disabled}
* Set to a disabled tab
* :host
*
*
* {@code focused}
* Set when the element is focused
* :host
*
*
* {@code focus-ring}
* Set when the element is keyboard focused
* :host
*
*
* {@code selected}
* Set when the tab is selected
* :host
*
*
* {@code active}
* Set when mousedown or enter/spacebar pressed
* :host
*
*
* {@code orientation}
* Set to {@code horizontal} or {@code vertical} depending on the direction
* of items
* :host
*
*
*
*
* See ThemableMixin –
* how to apply styles for shadow parts
*
*/
@Generated({ "Generator: com.vaadin.generator.ComponentGenerator#1.0-SNAPSHOT",
"WebComponent: Vaadin.TabElement#2.0.0", "Flow#1.0-SNAPSHOT" })
@Tag("vaadin-tab")
@HtmlImport("frontend://bower_components/vaadin-tabs/src/vaadin-tab.html")
public abstract class GeneratedVaadinTab>
extends Component implements HasStyle {
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Submittable string value. The default value is the trimmed text content
* of the element.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code value} property from the webcomponent
*/
protected String getValueString() {
return getElement().getProperty("value");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Submittable string value. The default value is the trimmed text content
* of the element.
*
*
* @param value
* the String value to set
*/
protected void setValue(String value) {
getElement().setProperty("value", value == null ? "" : value);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* If true, the user cannot interact with this element.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code disabled} property from the webcomponent
*/
protected boolean isDisabledBoolean() {
return getElement().getProperty("disabled", false);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* If true, the user cannot interact with this element.
*
*
* @param disabled
* the boolean value to set
*/
protected void setDisabled(boolean disabled) {
getElement().setProperty("disabled", disabled);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* If true, the item is in selected state.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code selected} property from the webcomponent
*/
protected boolean isSelectedBoolean() {
return getElement().getProperty("selected", false);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* If true, the item is in selected state.
*
*
* @param selected
* the boolean value to set
*/
protected void setSelected(boolean selected) {
getElement().setProperty("selected", selected);
}
}