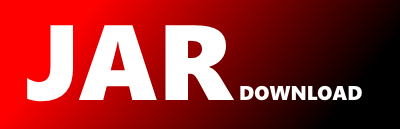
com.vaadin.flow.component.upload.GeneratedVaadinUpload Maven / Gradle / Ivy
/**
* Copyright (C) 2000-2023 Vaadin Ltd
*
* This program is available under Vaadin Commercial License and Service Terms.
*
* See for the full
* license.
*/
package com.vaadin.flow.component.upload;
import com.vaadin.flow.component.Component;
import com.vaadin.flow.component.ComponentEvent;
import com.vaadin.flow.component.ComponentEventListener;
import com.vaadin.flow.component.DomEvent;
import com.vaadin.flow.component.EventData;
import com.vaadin.flow.component.HasStyle;
import com.vaadin.flow.component.Synchronize;
import com.vaadin.flow.component.Tag;
import com.vaadin.flow.component.dependency.JsModule;
import com.vaadin.flow.component.dependency.NpmPackage;
import com.vaadin.flow.dom.Element;
import com.vaadin.flow.shared.Registration;
import elemental.json.JsonArray;
import elemental.json.JsonObject;
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* {@code } is a Web Component for uploading multiple files with
* drag and drop support.
*
*
* Example:
*
*
* {@code }
*
* Styling
*
* The following shadow DOM parts are available for styling:
*
*
*
*
* Part name
* Description
*
*
*
* {@code primary-buttons}
* Upload container
*
*
* {@code upload-button}
* Upload button
*
*
* {@code drop-label}
* Label for drop indicator
*
*
* {@code drop-label-icon}
* Icon for drop indicator
*
*
* {@code file-list}
* File list container
*
*
*
*
* The following state attributes are available for styling:
*
*
*
*
* Attribute
* Description
* Part name
*
*
*
* {@code nodrop}
* Set when drag and drop is disabled (e. g., on touch devices)
* {@code :host}
*
*
* {@code dragover}
* A file is being dragged over the element
* {@code :host}
*
*
* {@code dragover-valid}
* A dragged file is valid with {@code maxFiles} and {@code accept} criteria
*
* {@code :host}
*
*
*
*
* See
* ThemableMixin
* – how to apply styles for shadow parts
*
*/
@Tag("vaadin-upload")
@NpmPackage(value = "@vaadin/polymer-legacy-adapter", version = "22.1.0")
@JsModule("@vaadin/polymer-legacy-adapter/style-modules.js")
@NpmPackage(value = "@vaadin/upload", version = "22.1.0")
@NpmPackage(value = "@vaadin/vaadin-upload", version = "22.1.0")
@JsModule("@vaadin/upload/src/vaadin-upload.js")
public abstract class GeneratedVaadinUpload>
extends Component implements HasStyle {
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Define whether the element supports dropping files on it for uploading.
* By default it's enabled in desktop and disabled in touch devices because
* mobile devices do not support drag events in general. Setting it false
* means that drop is enabled even in touch-devices, and true disables drop
* in all devices.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code nodrop} property from the webcomponent
*/
protected boolean isNodropBoolean() {
return getElement().getProperty("nodrop", false);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Define whether the element supports dropping files on it for uploading.
* By default it's enabled in desktop and disabled in touch devices because
* mobile devices do not support drag events in general. Setting it false
* means that drop is enabled even in touch-devices, and true disables drop
* in all devices.
*
*
* @param nodrop
* the boolean value to set
*/
protected void setNodrop(boolean nodrop) {
getElement().setProperty("nodrop", nodrop);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* The server URL. The default value is an empty string, which means that
* window.location will be used.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code target} property from the webcomponent
*/
protected String getTargetString() {
return getElement().getProperty("target");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* The server URL. The default value is an empty string, which means that
* window.location will be used.
*
*
* @param target
* the String value to set
*/
protected void setTarget(String target) {
getElement().setProperty("target", target == null ? "" : target);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* HTTP Method used to send the files. Only POST and PUT are allowed.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code method} property from the webcomponent
*/
protected String getMethodString() {
return getElement().getProperty("method");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* HTTP Method used to send the files. Only POST and PUT are allowed.
*
*
* @param method
* the String value to set
*/
protected void setMethod(String method) {
getElement().setProperty("method", method == null ? "" : method);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Key-Value map to send to the server. If you set this property as an
* attribute, use a valid JSON string, for example: {@code }
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code headers} property from the webcomponent
*/
protected JsonObject getHeadersJsonObject() {
return (JsonObject) getElement().getPropertyRaw("headers");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Key-Value map to send to the server. If you set this property as an
* attribute, use a valid JSON string, for example: {@code }
*
*
* @param headers
* the JsonObject value to set
*/
protected void setHeaders(JsonObject headers) {
getElement().setPropertyJson("headers", headers);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Max time in milliseconds for the entire upload process, if exceeded the
* request will be aborted. Zero means that there is no timeout.
*
*
*
* <p>This property is not synchronized automatically from the client side, so the returned value may not be the same as in client side.
*
*
*
* @return the {@code timeout} property from the webcomponent
*/
protected double getTimeoutDouble() {
return getElement().getProperty("timeout", 0.0);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Max time in milliseconds for the entire upload process, if exceeded the
* request will be aborted. Zero means that there is no timeout.
*
*
* @param timeout
* the double value to set
*/
protected void setTimeout(double timeout) {
getElement().setProperty("timeout", timeout);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* The array of files being processed, or already uploaded.
*
*
* Each element is a {@code File}
* object with a number of extra properties to track the upload process:
*
*
* - {@code uploadTarget}: The target URL used to upload this file.
* - {@code elapsed}: Elapsed time since the upload started.
* - {@code elapsedStr}: Human-readable elapsed time.
* - {@code remaining}: Number of seconds remaining for the upload to
* finish.
* - {@code remainingStr}: Human-readable remaining time for the upload to
* finish.
* - {@code progress}: Percentage of the file already uploaded.
* - {@code speed}: Upload speed in kB/s.
* - {@code size}: File size in bytes.
* - {@code totalStr}: Human-readable total size of the file.
* - {@code loaded}: Bytes transferred so far.
* - {@code loadedStr}: Human-readable uploaded size at the moment.
* - {@code status}: Status of the upload process.
* - {@code error}: Error message in case the upload failed.
* - {@code abort}: True if the file was canceled by the user.
* - {@code complete}: True when the file was transferred to the
* server.
* - {@code uploading}: True while transferring data to the server.
*
* This property is synchronized automatically from client side when a
* 'files-changed' event happens.
*
*
* @return the {@code files} property from the webcomponent
*/
@Synchronize(property = "files", value = "files-changed")
protected JsonArray getFilesJsonArray() {
return (JsonArray) getElement().getPropertyRaw("files");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* The array of files being processed, or already uploaded.
*
*
* Each element is a {@code File}
* object with a number of extra properties to track the upload process:
*
*
* - {@code uploadTarget}: The target URL used to upload this file.
* - {@code elapsed}: Elapsed time since the upload started.
* - {@code elapsedStr}: Human-readable elapsed time.
* - {@code remaining}: Number of seconds remaining for the upload to
* finish.
* - {@code remainingStr}: Human-readable remaining time for the upload to
* finish.
* - {@code progress}: Percentage of the file already uploaded.
* - {@code speed}: Upload speed in kB/s.
* - {@code size}: File size in bytes.
* - {@code totalStr}: Human-readable total size of the file.
* - {@code loaded}: Bytes transferred so far.
* - {@code loadedStr}: Human-readable uploaded size at the moment.
* - {@code status}: Status of the upload process.
* - {@code error}: Error message in case the upload failed.
* - {@code abort}: True if the file was canceled by the user.
* - {@code complete}: True when the file was transferred to the
* server.
* - {@code uploading}: True while transferring data to the server.
*
*
* @param files
* the JsonArray value to set
*/
protected void setFiles(JsonArray files) {
getElement().setPropertyJson("files", files);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Limit of files to upload, by default it is unlimited. If the value is set
* to one, native file browser will prevent selecting multiple files.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code maxFiles} property from the webcomponent
*/
protected double getMaxFilesDouble() {
return getElement().getProperty("maxFiles", 0.0);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Limit of files to upload, by default it is unlimited. If the value is set
* to one, native file browser will prevent selecting multiple files.
*
*
* @param maxFiles
* the double value to set
*/
protected void setMaxFiles(double maxFiles) {
getElement().setProperty("maxFiles", maxFiles);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Specifies if the maximum number of files have been uploaded
*
* This property is synchronized automatically from client side when a
* 'max-files-reached-changed' event happens.
*
*
* @return the {@code maxFilesReached} property from the webcomponent
*/
@Synchronize(property = "maxFilesReached", value = "max-files-reached-changed")
protected boolean isMaxFilesReachedBoolean() {
return getElement().getProperty("maxFilesReached", false);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Specifies the types of files that the server accepts. Syntax: a
* comma-separated list of MIME type patterns (wildcards are allowed) or
* file extensions. Notice that MIME types are widely supported, while file
* extensions are only implemented in certain browsers, so avoid using it.
* Example: accept="video/*,image/tiff" or
* accept=".pdf,audio/mp3"
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code accept} property from the webcomponent
*/
protected String getAcceptString() {
return getElement().getProperty("accept");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Specifies the types of files that the server accepts. Syntax: a
* comma-separated list of MIME type patterns (wildcards are allowed) or
* file extensions. Notice that MIME types are widely supported, while file
* extensions are only implemented in certain browsers, so avoid using it.
* Example: accept="video/*,image/tiff" or
* accept=".pdf,audio/mp3"
*
*
* @param accept
* the String value to set
*/
protected void setAccept(String accept) {
getElement().setProperty("accept", accept == null ? "" : accept);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Specifies the maximum file size in bytes allowed to upload. Notice that
* it is a client-side constraint, which will be checked before sending the
* request. Obviously you need to do the same validation in the server-side
* and be sure that they are aligned.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code maxFileSize} property from the webcomponent
*/
protected double getMaxFileSizeDouble() {
return getElement().getProperty("maxFileSize", 0.0);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Specifies the maximum file size in bytes allowed to upload. Notice that
* it is a client-side constraint, which will be checked before sending the
* request. Obviously you need to do the same validation in the server-side
* and be sure that they are aligned.
*
*
* @param maxFileSize
* the double value to set
*/
protected void setMaxFileSize(double maxFileSize) {
getElement().setProperty("maxFileSize", maxFileSize);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Specifies the 'name' property at Content-Disposition
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code formDataName} property from the webcomponent
*/
protected String getFormDataNameString() {
return getElement().getProperty("formDataName");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Specifies the 'name' property at Content-Disposition
*
*
* @param formDataName
* the String value to set
*/
protected void setFormDataName(String formDataName) {
getElement().setProperty("formDataName",
formDataName == null ? "" : formDataName);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Prevents upload(s) from immediately uploading upon adding file(s). When
* set, you must manually trigger uploads using the {@code uploadFiles}
* method
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code noAuto} property from the webcomponent
*/
protected boolean isNoAutoBoolean() {
return getElement().getProperty("noAuto", false);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Prevents upload(s) from immediately uploading upon adding file(s). When
* set, you must manually trigger uploads using the {@code uploadFiles}
* method
*
*
* @param noAuto
* the boolean value to set
*/
protected void setNoAuto(boolean noAuto) {
getElement().setProperty("noAuto", noAuto);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Set the withCredentials flag on the request.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code withCredentials} property from the webcomponent
*/
protected boolean isWithCredentialsBoolean() {
return getElement().getProperty("withCredentials", false);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Set the withCredentials flag on the request.
*
*
* @param withCredentials
* the boolean value to set
*/
protected void setWithCredentials(boolean withCredentials) {
getElement().setProperty("withCredentials", withCredentials);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Pass-through to input's capture attribute. Allows user to trigger device
* inputs such as camera or microphone immediately.
*
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
*
* @return the {@code capture} property from the webcomponent
*/
protected String getCaptureString() {
return getElement().getProperty("capture");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Pass-through to input's capture attribute. Allows user to trigger device
* inputs such as camera or microphone immediately.
*
*
* @param capture
* the String value to set
*/
protected void setCapture(String capture) {
getElement().setProperty("capture", capture == null ? "" : capture);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* The object used to localize this component. For changing the default
* localization, change the entire i18n object or just the property
* you want to modify.
*
*
* The object has the following JSON structure and default values:
*
*
*
* {
* dropFiles: {
* one: 'Drop file here
* many: 'Drop files here
* },
* addFiles: {
* one: 'Select File...',
* many: 'Upload Files...'
* },
* cancel: 'Cancel',
* error: {
* tooManyFiles: 'Too Many Files.',
* fileIsTooBig: 'File is Too Big.',
* incorrectFileType: 'Incorrect File Type.'
* },
* uploading: {
* status: {
* connecting: 'Connecting...',
* stalled: 'Stalled.',
* processing: 'Processing File...',
* held: 'Queued'
* },
* remainingTime: {
* prefix: 'remaining time: ',
* unknown: 'unknown remaining time'
* },
* error: {
* serverUnavailable: 'Server Unavailable',
* unexpectedServerError: 'Unexpected Server Error',
* forbidden: 'Forbidden'
* }
* },
* units: {
* size: ['B', 'kB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB']
* },
* formatSize: function(bytes) {
* // returns the size followed by the best suitable unit
* },
* formatTime: function(seconds, [secs, mins, hours]) {
* // returns a 'HH:MM:SS' string
* }
* }<p>This property is not synchronized automatically from the client side, so the returned value may not be the same as in client side.
*
*
*
* @return the {@code i18n} property from the webcomponent
*/
protected JsonObject getI18nJsonObject() {
return (JsonObject) getElement().getPropertyRaw("i18n");
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* The object used to localize this component. For changing the default
* localization, change the entire i18n object or just the property
* you want to modify.
*
*
* The object has the following JSON structure and default values:
*
*
*
* {
* dropFiles: {
* one: 'Drop file here
* many: 'Drop files here
* },
* addFiles: {
* one: 'Select File...',
* many: 'Upload Files...'
* },
* cancel: 'Cancel',
* error: {
* tooManyFiles: 'Too Many Files.',
* fileIsTooBig: 'File is Too Big.',
* incorrectFileType: 'Incorrect File Type.'
* },
* uploading: {
* status: {
* connecting: 'Connecting...',
* stalled: 'Stalled.',
* processing: 'Processing File...',
* held: 'Queued'
* },
* remainingTime: {
* prefix: 'remaining time: ',
* unknown: 'unknown remaining time'
* },
* error: {
* serverUnavailable: 'Server Unavailable',
* unexpectedServerError: 'Unexpected Server Error',
* forbidden: 'Forbidden'
* }
* },
* units: {
* size: ['B', 'kB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB']
* },
* formatSize: function(bytes) {
* // returns the size followed by the best suitable unit
* },
* formatTime: function(seconds, [secs, mins, hours]) {
* // returns a 'HH:MM:SS' string
* }
* }
*
*
*
* @param i18n
* the JsonObject value to set
*/
protected void setI18n(JsonObject i18n) {
getElement().setPropertyJson("i18n", i18n);
}
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* Triggers the upload of any files that are not completed
*
*
* @param files
* Missing documentation!
*/
protected void uploadFiles(JsonObject files) {
getElement().callJsFunction("uploadFiles", files);
}
@DomEvent("file-reject")
public static class FileRejectEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailFile;
private final String detailError;
public FileRejectEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.file") JsonObject detailFile,
@EventData("event.detail.error") String detailError) {
super(source, fromClient);
this.detail = detail;
this.detailFile = detailFile;
this.detailError = detailError;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailFile() {
return detailFile;
}
public String getDetailError() {
return detailError;
}
}
/**
* Adds a listener for {@code file-reject} events fired by the webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addFileRejectListener(
ComponentEventListener> listener) {
return addListener(FileRejectEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-abort")
public static class UploadAbortEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
public UploadAbortEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code upload-abort} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadAbortListener(
ComponentEventListener> listener) {
return addListener(UploadAbortEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-before")
public static class UploadBeforeEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
private final JsonObject detailFileUploadTarget;
public UploadBeforeEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile,
@EventData("event.detail.file.uploadTarget") JsonObject detailFileUploadTarget) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
this.detailFileUploadTarget = detailFileUploadTarget;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
public JsonObject getDetailFileUploadTarget() {
return detailFileUploadTarget;
}
}
/**
* Adds a listener for {@code upload-before} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadBeforeListener(
ComponentEventListener> listener) {
return addListener(UploadBeforeEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-error")
public static class UploadErrorEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
public UploadErrorEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code upload-error} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadErrorListener(
ComponentEventListener> listener) {
return addListener(UploadErrorEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-progress")
public static class UploadProgressEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
public UploadProgressEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code upload-progress} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadProgressListener(
ComponentEventListener> listener) {
return addListener(UploadProgressEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-request")
public static class UploadRequestEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
private final JsonObject detailFormData;
public UploadRequestEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile,
@EventData("event.detail.formData") JsonObject detailFormData) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
this.detailFormData = detailFormData;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
public JsonObject getDetailFormData() {
return detailFormData;
}
}
/**
* Adds a listener for {@code upload-request} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadRequestListener(
ComponentEventListener> listener) {
return addListener(UploadRequestEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-response")
public static class UploadResponseEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
public UploadResponseEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code upload-response} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadResponseListener(
ComponentEventListener> listener) {
return addListener(UploadResponseEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-retry")
public static class UploadRetryEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
public UploadRetryEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code upload-retry} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadRetryListener(
ComponentEventListener> listener) {
return addListener(UploadRetryEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-start")
public static class UploadStartEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
public UploadStartEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code upload-start} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadStartListener(
ComponentEventListener> listener) {
return addListener(UploadStartEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("upload-success")
public static class UploadSuccessEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailXhr;
private final JsonObject detailFile;
public UploadSuccessEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.xhr") JsonObject detailXhr,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailXhr = detailXhr;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailXhr() {
return detailXhr;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code upload-success} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addUploadSuccessListener(
ComponentEventListener> listener) {
return addListener(UploadSuccessEvent.class,
(ComponentEventListener) listener);
}
public static class FilesChangeEvent>
extends ComponentEvent {
private final JsonArray files;
public FilesChangeEvent(R source, boolean fromClient) {
super(source, fromClient);
this.files = source.getFilesJsonArray();
}
public JsonArray getFiles() {
return files;
}
}
/**
* Adds a listener for {@code files-changed} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
protected Registration addFilesChangeListener(
ComponentEventListener> listener) {
return getElement()
.addPropertyChangeListener("files",
event -> listener.onComponentEvent(
new FilesChangeEvent((R) this,
event.isUserOriginated())));
}
public static class MaxFilesReachedChangeEvent>
extends ComponentEvent {
private final boolean maxFilesReached;
public MaxFilesReachedChangeEvent(R source, boolean fromClient) {
super(source, fromClient);
this.maxFilesReached = source.isMaxFilesReachedBoolean();
}
public boolean isMaxFilesReached() {
return maxFilesReached;
}
}
/**
* Adds a listener for {@code max-files-reached-changed} events fired by the
* webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
protected Registration addMaxFilesReachedChangeListener(
ComponentEventListener> listener) {
return getElement().addPropertyChangeListener("maxFilesReached",
event -> listener.onComponentEvent(
new MaxFilesReachedChangeEvent((R) this,
event.isUserOriginated())));
}
/**
* Adds the given components as children of this component at the slot
* 'add-button'.
*
* @param components
* The components to add.
* @see MDN
* page about slots
* @see Spec
* website about slots
*/
protected void addToAddButton(Component... components) {
for (Component component : components) {
component.getElement().setAttribute("slot", "add-button");
getElement().appendChild(component.getElement());
}
}
/**
* Adds the given components as children of this component at the slot
* 'drop-label-icon'.
*
* @param components
* The components to add.
* @see MDN
* page about slots
* @see Spec
* website about slots
*/
protected void addToDropLabelIcon(Component... components) {
for (Component component : components) {
component.getElement().setAttribute("slot", "drop-label-icon");
getElement().appendChild(component.getElement());
}
}
/**
* Adds the given components as children of this component at the slot
* 'drop-label'.
*
* @param components
* The components to add.
* @see MDN
* page about slots
* @see Spec
* website about slots
*/
protected void addToDropLabel(Component... components) {
for (Component component : components) {
component.getElement().setAttribute("slot", "drop-label");
getElement().appendChild(component.getElement());
}
}
/**
* Adds the given components as children of this component at the slot
* 'file-list'.
*
* @param components
* The components to add.
* @see MDN
* page about slots
* @see Spec
* website about slots
*/
protected void addToFileList(Component... components) {
for (Component component : components) {
component.getElement().setAttribute("slot", "file-list");
getElement().appendChild(component.getElement());
}
}
/**
* Removes the given child components from this component.
*
* @param components
* The components to remove.
* @throws IllegalArgumentException
* if any of the components is not a child of this component.
*/
protected void remove(Component... components) {
for (Component component : components) {
if (getElement().equals(component.getElement().getParent())) {
component.getElement().removeAttribute("slot");
getElement().removeChild(component.getElement());
} else {
throw new IllegalArgumentException("The given component ("
+ component + ") is not a child of this component");
}
}
}
/**
* Removes all contents from this component, this includes child components,
* text content as well as child elements that have been added directly to
* this component using the {@link Element} API.
*/
protected void removeAll() {
getElement().getChildren()
.forEach(child -> child.removeAttribute("slot"));
getElement().removeAllChildren();
}
}