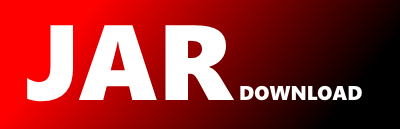
com.vaadin.flow.component.upload.GeneratedVaadinUploadFile Maven / Gradle / Ivy
/**
* Copyright (C) 2000-2023 Vaadin Ltd
*
* This program is available under Vaadin Commercial License and Service Terms.
*
* See for the full
* license.
*/
package com.vaadin.flow.component.upload;
import com.vaadin.flow.component.Component;
import com.vaadin.flow.component.ComponentEvent;
import com.vaadin.flow.component.ComponentEventListener;
import com.vaadin.flow.component.DomEvent;
import com.vaadin.flow.component.EventData;
import com.vaadin.flow.component.HasStyle;
import com.vaadin.flow.component.Tag;
import com.vaadin.flow.component.dependency.JsModule;
import com.vaadin.flow.component.dependency.NpmPackage;
import com.vaadin.flow.shared.Registration;
import elemental.json.JsonObject;
/**
*
* Description copied from corresponding location in WebComponent:
*
*
* {@code } element represents a file in the file list of
* {@code }.
*
* Styling
*
* The following shadow DOM parts are available for styling:
*
*
*
*
* Part name
* Description
*
*
*
* {@code row}
* File container
*
*
* {@code info}
* Container for file status icon, file name, status and error messages
*
*
* {@code done-icon}
* File done status icon
*
*
* {@code warning-icon}
* File warning status icon
*
*
* {@code meta}
* Container for file name, status and error messages
*
*
* {@code name}
* File name
*
*
* {@code error}
* Error message, shown when error happens
*
*
* {@code status}
* Status message
*
*
* {@code commands}
* Container for file command icons
*
*
* {@code start-button}
* Start file upload button
*
*
* {@code retry-button}
* Retry file upload button
*
*
* {@code clear-button}
* Clear file button
*
*
* {@code progress}
* Progress bar
*
*
*
*
* The following state attributes are available for styling:
*
*
*
*
* Attribute
* Description
* Part name
*
*
*
* {@code error}
* An error has happened during uploading
* {@code :host}
*
*
* {@code indeterminate}
* Uploading is in progress, but the progress value is unknown
* {@code :host}
*
*
* {@code uploading}
* Uploading is in progress
* {@code :host}
*
*
* {@code complete}
* Uploading has finished successfully
* {@code :host}
*
*
*
*
* See
* ThemableMixin
* – how to apply styles for shadow parts
*
*/
@Tag("vaadin-upload-file")
@NpmPackage(value = "@vaadin/polymer-legacy-adapter", version = "22.1.0")
@JsModule("@vaadin/polymer-legacy-adapter/style-modules.js")
@JsModule("@vaadin/upload/src/vaadin-upload-file.js")
public abstract class GeneratedVaadinUploadFile>
extends Component implements HasStyle {
/**
* This property is not synchronized automatically from the client side, so
* the returned value may not be the same as in client side.
*
* @return the {@code file} property from the webcomponent
*/
protected JsonObject getFileJsonObject() {
return (JsonObject) getElement().getPropertyRaw("file");
}
/**
* @param file
* the JsonObject value to set
*/
protected void setFile(JsonObject file) {
getElement().setPropertyJson("file", file);
}
@DomEvent("file-abort")
public static class FileAbortEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailFile;
public FileAbortEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code file-abort} events fired by the webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addFileAbortListener(
ComponentEventListener> listener) {
return addListener(FileAbortEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("file-remove")
public static class FileRemoveEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailFile;
public FileRemoveEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code file-remove} events fired by the webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addFileRemoveListener(
ComponentEventListener> listener) {
return addListener(FileRemoveEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("file-retry")
public static class FileRetryEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailFile;
public FileRetryEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code file-retry} events fired by the webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addFileRetryListener(
ComponentEventListener> listener) {
return addListener(FileRetryEvent.class,
(ComponentEventListener) listener);
}
@DomEvent("file-start")
public static class FileStartEvent>
extends ComponentEvent {
private final JsonObject detail;
private final JsonObject detailFile;
public FileStartEvent(R source, boolean fromClient,
@EventData("event.detail") JsonObject detail,
@EventData("event.detail.file") JsonObject detailFile) {
super(source, fromClient);
this.detail = detail;
this.detailFile = detailFile;
}
public JsonObject getDetail() {
return detail;
}
public JsonObject getDetailFile() {
return detailFile;
}
}
/**
* Adds a listener for {@code file-start} events fired by the webcomponent.
*
* @param listener
* the listener
* @return a {@link Registration} for removing the event listener
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected Registration addFileStartListener(
ComponentEventListener> listener) {
return addListener(FileStartEvent.class,
(ComponentEventListener) listener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy