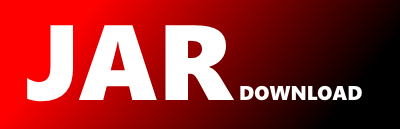
com.varmateo.yawg.asciidoctor.AdocUtils Maven / Gradle / Ivy
/**************************************************************************
*
* Copyright (c) 2017-2019 Yawg project contributors.
*
**************************************************************************/
package com.varmateo.yawg.asciidoctor;
import java.io.File;
import java.nio.file.Path;
import io.vavr.control.Option;
import org.asciidoctor.AttributesBuilder;
import org.asciidoctor.OptionsBuilder;
import org.asciidoctor.SafeMode;
import com.varmateo.yawg.spi.PageVars;
/**
* Utility functions simplifying Asciidoctor usage.
*/
final class AdocUtils {
/**
* No instances of this class are to be created.
*/
private AdocUtils() {
// Nothing to do.
}
/**
*
*/
public static OptionsBuilder buildOptionsForBakeWithoutTemplate(
final Path sourcePath,
final Path targetDir,
final Path targetPath,
final PageVars pageVars) {
final File targetFile = targetPath.toFile();
final AttributesBuilder attributes =
buildCommonAttributes(sourcePath, targetDir, pageVars)
.noFooter(false);
return buildCommonOptions(attributes)
.toFile(targetFile);
}
/**
*
*/
public static OptionsBuilder buildOptionsForBakeWithTemplate(
final Path sourcePath,
final Path targetDir,
final PageVars pageVars) {
final AttributesBuilder attributes =
buildCommonAttributes(sourcePath, targetDir, pageVars);
return buildCommonOptions(attributes)
.headerFooter(false);
}
/**
*
*/
private static OptionsBuilder buildCommonOptions(
final AttributesBuilder attributes) {
return OptionsBuilder.options()
.attributes(attributes)
.safe(SafeMode.UNSAFE);
}
/**
* Prepares a set of attributes that affect how Asciidoctor works.
*/
private static AttributesBuilder buildCommonAttributes(
final Path sourcePath,
final Path targetDir,
final PageVars pageVars) {
final String docDir = Option.of(sourcePath.getParent())
.map(Object::toString)
.getOrElse(".");
final AttributesBuilder attributes = AttributesBuilder.attributes();
// First add all the existing page vars as attributes visible
// to Asciidoc content.
attributes.attributes(pageVars.asMap());
// And now add , or override, some well known attributes which
// control behavior in the Asciidoctor renderer.
// Path of directory that contains the document. It is needed
// for the PlantUML AsciidoctorJ extension to find its input
// files relative to the AsciiDoc source document. At least in
// version 1.3.1 of asciidoctorj-diagrams
attributes.attribute("docdir", docDir);
// Path of directory where image files generated by PlantUML
// will be created.
final String imagesOutDir = targetDir.toAbsolutePath().normalize().toString();
attributes.attribute("imagesoutdir", imagesOutDir);
// Enable basic syntax highlithing by default.
attributes.attribute("source-highlighter", "coderay");
attributes.attribute("coderay-css", "style");
// HACK - THIS MAKES THE AsciiDoc BAKER NOT THREAD SAFE.
System.setProperty("plantuml.include.path", docDir);
return attributes;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy