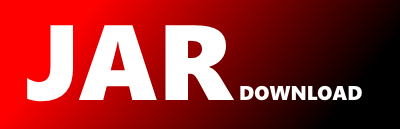
com.varmateo.yawg.util.SimpleMap Maven / Gradle / Ivy
/**************************************************************************
*
* Copyright (c) 2016-2020 Yawg project contributors.
*
**************************************************************************/
package com.varmateo.yawg.util;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import com.varmateo.yawg.api.YawgException;
/**
* Wrapper for an unmodifiable map with utility methods for retrieving
* values.
*/
public final class SimpleMap {
private final Map _map;
/**
* Initializes this simple map with the given map.
*
* As a matter of optimization we assume the given map will
* never be modified. It is up to the caller to ensure no changes
* are performed in the givan map
.
*
* @param map The map to be wrapped by this simple map.
*/
public SimpleMap(final Map map) {
_map = Objects.requireNonNull(map);
}
/**
* Fetches a view on the contents of this simple map as an
* unmodifiable map.
*
* @return A unmodifiable view of the contents of this simple map.
*/
public Map asMap() {
return Collections.unmodifiableMap(_map);
}
/**
*
*/
public Optional get(
final String key,
final Class klass) {
final T value = getWithType(key, klass);
return Optional.ofNullable(value);
}
/**
*
*/
public Optional getString(final String key) {
return get(key, String.class);
}
/**
*
*/
public Optional getMap(final String key) {
@SuppressWarnings("unchecked")
final Map value = (Map) getWithType(key, Map.class);
return Optional.ofNullable(value)
.map(SimpleMap::new);
}
/**
*
*/
public Optional> getList(
final String key,
final Class itemsClass) {
@SuppressWarnings("unchecked")
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy