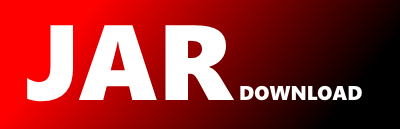
com.vaynberg.wicket.select2.HomePage Maven / Gradle / Ivy
/*
* Copyright 2012 Igor Vaynberg
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this work except in compliance with
* the License. You may obtain a copy of the License in the LICENSE file, or at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.vaynberg.wicket.select2;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import org.apache.wicket.markup.html.WebPage;
import org.apache.wicket.markup.html.basic.Label;
import org.apache.wicket.markup.html.form.Form;
import org.apache.wicket.model.PropertyModel;
import org.apache.wicket.request.mapper.parameter.PageParameters;
/**
* Example page.
*
* @author igor
*
*/
@SuppressWarnings("unused")
public class HomePage extends WebPage {
private static final int PAGE_SIZE = 20;
private Country country = Country.US;
private List countries = new ArrayList(Arrays.asList(new Country[] { Country.US, Country.CA }));
public HomePage() {
// single-select example
add(new Label("country", new PropertyModel
© 2015 - 2025 Weber Informatics LLC | Privacy Policy