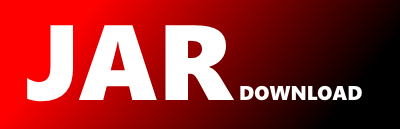
com.vektorsoft.demux.test.DMXTestServiceReference Maven / Gradle / Ivy
/******************************************************************************
*
* Copyright 2012 - 2013 Vektor Software.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*****************************************************************************/
package com.vektorsoft.demux.test;
import java.util.Dictionary;
import java.util.Properties;
import org.osgi.framework.Bundle;
import org.osgi.framework.ServiceReference;
/**
* Simple implementation of {@link org.osgi.framework.ServiceReference} for use in testing. This
* class implements only a minimal set of methods needed to pass compilation and be used for
* basic unit testing.
*
* @param service reference type
* @author Vladimir Djurovic
*/
public class DMXTestServiceReference implements ServiceReference {
/** Registered properties. */
private Properties props;
/** Bundle which registered service. */
private Bundle bundle;
/**
* Creates new instance.
*
* @param dict
*/
public DMXTestServiceReference(Dictionary dict) {
props = new Properties();
if (dict != null) {
while (dict.keys().hasMoreElements()) {
String key = dict.keys().nextElement();
props.put(key, dict.get(key));
}
}
}
@Override
public Object getProperty(String key) {
return props.get(key);
}
@Override
public String[] getPropertyKeys() {
return props.keySet().toArray(new String[1]);
}
@Override
public Bundle getBundle() {
return bundle;
}
@Override
public Bundle[] getUsingBundles() {
return new Bundle[0];
}
@Override
public boolean isAssignableTo(Bundle bundle, String string) {
return false;
}
@Override
public int compareTo(Object o) {
return -1;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy