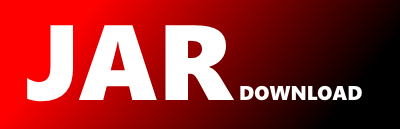
com.vendasta.sales.v1.generated.ApiProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: sales/v1/api.proto
package com.vendasta.sales.v1.generated;
public final class ApiProto {
private ApiProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code sales.v1.TagMatchType}
*/
public enum TagMatchType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Accounts containing all of the tags will match this rule.
*
*
* TAG_MATCH_TYPE_ALL = 0;
*/
TAG_MATCH_TYPE_ALL(0),
/**
*
* Accounts containing any of the tags will match this rule.
*
*
* TAG_MATCH_TYPE_ANY = 1;
*/
TAG_MATCH_TYPE_ANY(1),
UNRECOGNIZED(-1),
;
/**
*
* Accounts containing all of the tags will match this rule.
*
*
* TAG_MATCH_TYPE_ALL = 0;
*/
public static final int TAG_MATCH_TYPE_ALL_VALUE = 0;
/**
*
* Accounts containing any of the tags will match this rule.
*
*
* TAG_MATCH_TYPE_ANY = 1;
*/
public static final int TAG_MATCH_TYPE_ANY_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TagMatchType valueOf(int value) {
return forNumber(value);
}
public static TagMatchType forNumber(int value) {
switch (value) {
case 0: return TAG_MATCH_TYPE_ALL;
case 1: return TAG_MATCH_TYPE_ANY;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TagMatchType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TagMatchType findValueByNumber(int number) {
return TagMatchType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.getDescriptor().getEnumTypes().get(0);
}
private static final TagMatchType[] VALUES = values();
public static TagMatchType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private TagMatchType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sales.v1.TagMatchType)
}
/**
* Protobuf enum {@code sales.v1.CriteriaMatchType}
*/
public enum CriteriaMatchType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Accounts matching all of the criteria will match this rule.
*
*
* CRITERIA_MATCH_TYPE_ALL = 0;
*/
CRITERIA_MATCH_TYPE_ALL(0),
/**
*
* Accounts matching any of the criteria will match this rule.
*
*
* CRITERIA_MATCH_TYPE_ANY = 1;
*/
CRITERIA_MATCH_TYPE_ANY(1),
UNRECOGNIZED(-1),
;
/**
*
* Accounts matching all of the criteria will match this rule.
*
*
* CRITERIA_MATCH_TYPE_ALL = 0;
*/
public static final int CRITERIA_MATCH_TYPE_ALL_VALUE = 0;
/**
*
* Accounts matching any of the criteria will match this rule.
*
*
* CRITERIA_MATCH_TYPE_ANY = 1;
*/
public static final int CRITERIA_MATCH_TYPE_ANY_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CriteriaMatchType valueOf(int value) {
return forNumber(value);
}
public static CriteriaMatchType forNumber(int value) {
switch (value) {
case 0: return CRITERIA_MATCH_TYPE_ALL;
case 1: return CRITERIA_MATCH_TYPE_ANY;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CriteriaMatchType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CriteriaMatchType findValueByNumber(int number) {
return CriteriaMatchType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.getDescriptor().getEnumTypes().get(1);
}
private static final CriteriaMatchType[] VALUES = values();
public static CriteriaMatchType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private CriteriaMatchType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sales.v1.CriteriaMatchType)
}
public interface AutoAssignCriteriaOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.AutoAssignCriteria)
com.google.protobuf.MessageOrBuilder {
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria getLocationCriteria();
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteriaOrBuilder getLocationCriteriaOrBuilder();
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria getCategoryCriteria();
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteriaOrBuilder getCategoryCriteriaOrBuilder();
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria getTagCriteria();
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteriaOrBuilder getTagCriteriaOrBuilder();
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria getConversionPointCriteria();
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteriaOrBuilder getConversionPointCriteriaOrBuilder();
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CriteriaCase getCriteriaCase();
}
/**
* Protobuf type {@code sales.v1.AutoAssignCriteria}
*/
public static final class AutoAssignCriteria extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.AutoAssignCriteria)
AutoAssignCriteriaOrBuilder {
// Use AutoAssignCriteria.newBuilder() to construct.
private AutoAssignCriteria(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AutoAssignCriteria() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private AutoAssignCriteria(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.Builder subBuilder = null;
if (criteriaCase_ == 1) {
subBuilder = ((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_).toBuilder();
}
criteria_ =
input.readMessage(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_);
criteria_ = subBuilder.buildPartial();
}
criteriaCase_ = 1;
break;
}
case 18: {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.Builder subBuilder = null;
if (criteriaCase_ == 2) {
subBuilder = ((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_).toBuilder();
}
criteria_ =
input.readMessage(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_);
criteria_ = subBuilder.buildPartial();
}
criteriaCase_ = 2;
break;
}
case 26: {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.Builder subBuilder = null;
if (criteriaCase_ == 3) {
subBuilder = ((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_).toBuilder();
}
criteria_ =
input.readMessage(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_);
criteria_ = subBuilder.buildPartial();
}
criteriaCase_ = 3;
break;
}
case 34: {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder subBuilder = null;
if (criteriaCase_ == 4) {
subBuilder = ((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_).toBuilder();
}
criteria_ =
input.readMessage(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_);
criteria_ = subBuilder.buildPartial();
}
criteriaCase_ = 4;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder.class);
}
public interface LocationCriteriaOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.AutoAssignCriteria.LocationCriteria)
com.google.protobuf.MessageOrBuilder {
/**
* string country = 1;
*/
java.lang.String getCountry();
/**
* string country = 1;
*/
com.google.protobuf.ByteString
getCountryBytes();
/**
* string state = 2;
*/
java.lang.String getState();
/**
* string state = 2;
*/
com.google.protobuf.ByteString
getStateBytes();
/**
* string city = 3;
*/
java.lang.String getCity();
/**
* string city = 3;
*/
com.google.protobuf.ByteString
getCityBytes();
}
/**
*
* Matches accounts based on their geographical location.
*
*
* Protobuf type {@code sales.v1.AutoAssignCriteria.LocationCriteria}
*/
public static final class LocationCriteria extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.AutoAssignCriteria.LocationCriteria)
LocationCriteriaOrBuilder {
// Use LocationCriteria.newBuilder() to construct.
private LocationCriteria(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocationCriteria() {
country_ = "";
state_ = "";
city_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private LocationCriteria(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
country_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
state_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
city_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.Builder.class);
}
public static final int COUNTRY_FIELD_NUMBER = 1;
private volatile java.lang.Object country_;
/**
* string country = 1;
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
country_ = s;
return s;
}
}
/**
* string country = 1;
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATE_FIELD_NUMBER = 2;
private volatile java.lang.Object state_;
/**
* string state = 2;
*/
public java.lang.String getState() {
java.lang.Object ref = state_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
state_ = s;
return s;
}
}
/**
* string state = 2;
*/
public com.google.protobuf.ByteString
getStateBytes() {
java.lang.Object ref = state_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
state_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CITY_FIELD_NUMBER = 3;
private volatile java.lang.Object city_;
/**
* string city = 3;
*/
public java.lang.String getCity() {
java.lang.Object ref = city_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
city_ = s;
return s;
}
}
/**
* string city = 3;
*/
public com.google.protobuf.ByteString
getCityBytes() {
java.lang.Object ref = city_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
city_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getCountryBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, country_);
}
if (!getStateBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, state_);
}
if (!getCityBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, city_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getCountryBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, country_);
}
if (!getStateBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, state_);
}
if (!getCityBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, city_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria other = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) obj;
boolean result = true;
result = result && getCountry()
.equals(other.getCountry());
result = result && getState()
.equals(other.getState());
result = result && getCity()
.equals(other.getCity());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COUNTRY_FIELD_NUMBER;
hash = (53 * hash) + getCountry().hashCode();
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + getState().hashCode();
hash = (37 * hash) + CITY_FIELD_NUMBER;
hash = (53 * hash) + getCity().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Matches accounts based on their geographical location.
*
*
* Protobuf type {@code sales.v1.AutoAssignCriteria.LocationCriteria}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.AutoAssignCriteria.LocationCriteria)
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteriaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
country_ = "";
state_ = "";
city_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria build() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria result = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria(this);
result.country_ = country_;
result.state_ = state_;
result.city_ = city_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance()) return this;
if (!other.getCountry().isEmpty()) {
country_ = other.country_;
onChanged();
}
if (!other.getState().isEmpty()) {
state_ = other.state_;
onChanged();
}
if (!other.getCity().isEmpty()) {
city_ = other.city_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object country_ = "";
/**
* string country = 1;
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
country_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string country = 1;
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string country = 1;
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
country_ = value;
onChanged();
return this;
}
/**
* string country = 1;
*/
public Builder clearCountry() {
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* string country = 1;
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
country_ = value;
onChanged();
return this;
}
private java.lang.Object state_ = "";
/**
* string state = 2;
*/
public java.lang.String getState() {
java.lang.Object ref = state_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
state_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string state = 2;
*/
public com.google.protobuf.ByteString
getStateBytes() {
java.lang.Object ref = state_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
state_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string state = 2;
*/
public Builder setState(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
state_ = value;
onChanged();
return this;
}
/**
* string state = 2;
*/
public Builder clearState() {
state_ = getDefaultInstance().getState();
onChanged();
return this;
}
/**
* string state = 2;
*/
public Builder setStateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
state_ = value;
onChanged();
return this;
}
private java.lang.Object city_ = "";
/**
* string city = 3;
*/
public java.lang.String getCity() {
java.lang.Object ref = city_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
city_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string city = 3;
*/
public com.google.protobuf.ByteString
getCityBytes() {
java.lang.Object ref = city_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
city_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string city = 3;
*/
public Builder setCity(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
city_ = value;
onChanged();
return this;
}
/**
* string city = 3;
*/
public Builder clearCity() {
city_ = getDefaultInstance().getCity();
onChanged();
return this;
}
/**
* string city = 3;
*/
public Builder setCityBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
city_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.AutoAssignCriteria.LocationCriteria)
}
// @@protoc_insertion_point(class_scope:sales.v1.AutoAssignCriteria.LocationCriteria)
private static final com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria();
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public LocationCriteria parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LocationCriteria(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CategoryCriteriaOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.AutoAssignCriteria.CategoryCriteria)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string categories = 2;
*/
java.util.List
getCategoriesList();
/**
* repeated string categories = 2;
*/
int getCategoriesCount();
/**
* repeated string categories = 2;
*/
java.lang.String getCategories(int index);
/**
* repeated string categories = 2;
*/
com.google.protobuf.ByteString
getCategoriesBytes(int index);
}
/**
*
* Matches accounts based on the business categories (also known as the business taxonomy).
*
*
* Protobuf type {@code sales.v1.AutoAssignCriteria.CategoryCriteria}
*/
public static final class CategoryCriteria extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.AutoAssignCriteria.CategoryCriteria)
CategoryCriteriaOrBuilder {
// Use CategoryCriteria.newBuilder() to construct.
private CategoryCriteria(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CategoryCriteria() {
categories_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private CategoryCriteria(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
categories_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
categories_.add(s);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
categories_ = categories_.getUnmodifiableView();
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.Builder.class);
}
public static final int CATEGORIES_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList categories_;
/**
* repeated string categories = 2;
*/
public com.google.protobuf.ProtocolStringList
getCategoriesList() {
return categories_;
}
/**
* repeated string categories = 2;
*/
public int getCategoriesCount() {
return categories_.size();
}
/**
* repeated string categories = 2;
*/
public java.lang.String getCategories(int index) {
return categories_.get(index);
}
/**
* repeated string categories = 2;
*/
public com.google.protobuf.ByteString
getCategoriesBytes(int index) {
return categories_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < categories_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, categories_.getRaw(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < categories_.size(); i++) {
dataSize += computeStringSizeNoTag(categories_.getRaw(i));
}
size += dataSize;
size += 1 * getCategoriesList().size();
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria other = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) obj;
boolean result = true;
result = result && getCategoriesList()
.equals(other.getCategoriesList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCategoriesCount() > 0) {
hash = (37 * hash) + CATEGORIES_FIELD_NUMBER;
hash = (53 * hash) + getCategoriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Matches accounts based on the business categories (also known as the business taxonomy).
*
*
* Protobuf type {@code sales.v1.AutoAssignCriteria.CategoryCriteria}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.AutoAssignCriteria.CategoryCriteria)
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteriaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
categories_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria build() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria result = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
categories_ = categories_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.categories_ = categories_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance()) return this;
if (!other.categories_.isEmpty()) {
if (categories_.isEmpty()) {
categories_ = other.categories_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCategoriesIsMutable();
categories_.addAll(other.categories_);
}
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList categories_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureCategoriesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
categories_ = new com.google.protobuf.LazyStringArrayList(categories_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string categories = 2;
*/
public com.google.protobuf.ProtocolStringList
getCategoriesList() {
return categories_.getUnmodifiableView();
}
/**
* repeated string categories = 2;
*/
public int getCategoriesCount() {
return categories_.size();
}
/**
* repeated string categories = 2;
*/
public java.lang.String getCategories(int index) {
return categories_.get(index);
}
/**
* repeated string categories = 2;
*/
public com.google.protobuf.ByteString
getCategoriesBytes(int index) {
return categories_.getByteString(index);
}
/**
* repeated string categories = 2;
*/
public Builder setCategories(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCategoriesIsMutable();
categories_.set(index, value);
onChanged();
return this;
}
/**
* repeated string categories = 2;
*/
public Builder addCategories(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCategoriesIsMutable();
categories_.add(value);
onChanged();
return this;
}
/**
* repeated string categories = 2;
*/
public Builder addAllCategories(
java.lang.Iterable values) {
ensureCategoriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, categories_);
onChanged();
return this;
}
/**
* repeated string categories = 2;
*/
public Builder clearCategories() {
categories_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string categories = 2;
*/
public Builder addCategoriesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureCategoriesIsMutable();
categories_.add(value);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.AutoAssignCriteria.CategoryCriteria)
}
// @@protoc_insertion_point(class_scope:sales.v1.AutoAssignCriteria.CategoryCriteria)
private static final com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria();
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CategoryCriteria parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CategoryCriteria(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TagCriteriaOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.AutoAssignCriteria.TagCriteria)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string tags = 1;
*/
java.util.List
getTagsList();
/**
* repeated string tags = 1;
*/
int getTagsCount();
/**
* repeated string tags = 1;
*/
java.lang.String getTags(int index);
/**
* repeated string tags = 1;
*/
com.google.protobuf.ByteString
getTagsBytes(int index);
/**
* .sales.v1.TagMatchType match_type = 2;
*/
int getMatchTypeValue();
/**
* .sales.v1.TagMatchType match_type = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.TagMatchType getMatchType();
}
/**
*
* Matches accounts based on the generic tags applied.
*
*
* Protobuf type {@code sales.v1.AutoAssignCriteria.TagCriteria}
*/
public static final class TagCriteria extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.AutoAssignCriteria.TagCriteria)
TagCriteriaOrBuilder {
// Use TagCriteria.newBuilder() to construct.
private TagCriteria(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TagCriteria() {
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
matchType_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private TagCriteria(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
tags_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
tags_.add(s);
break;
}
case 16: {
int rawValue = input.readEnum();
matchType_ = rawValue;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
tags_ = tags_.getUnmodifiableView();
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_TagCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_TagCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.Builder.class);
}
private int bitField0_;
public static final int TAGS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList tags_;
/**
* repeated string tags = 1;
*/
public com.google.protobuf.ProtocolStringList
getTagsList() {
return tags_;
}
/**
* repeated string tags = 1;
*/
public int getTagsCount() {
return tags_.size();
}
/**
* repeated string tags = 1;
*/
public java.lang.String getTags(int index) {
return tags_.get(index);
}
/**
* repeated string tags = 1;
*/
public com.google.protobuf.ByteString
getTagsBytes(int index) {
return tags_.getByteString(index);
}
public static final int MATCH_TYPE_FIELD_NUMBER = 2;
private int matchType_;
/**
* .sales.v1.TagMatchType match_type = 2;
*/
public int getMatchTypeValue() {
return matchType_;
}
/**
* .sales.v1.TagMatchType match_type = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.TagMatchType getMatchType() {
com.vendasta.sales.v1.generated.ApiProto.TagMatchType result = com.vendasta.sales.v1.generated.ApiProto.TagMatchType.valueOf(matchType_);
return result == null ? com.vendasta.sales.v1.generated.ApiProto.TagMatchType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < tags_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, tags_.getRaw(i));
}
if (matchType_ != com.vendasta.sales.v1.generated.ApiProto.TagMatchType.TAG_MATCH_TYPE_ALL.getNumber()) {
output.writeEnum(2, matchType_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < tags_.size(); i++) {
dataSize += computeStringSizeNoTag(tags_.getRaw(i));
}
size += dataSize;
size += 1 * getTagsList().size();
}
if (matchType_ != com.vendasta.sales.v1.generated.ApiProto.TagMatchType.TAG_MATCH_TYPE_ALL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, matchType_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria other = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) obj;
boolean result = true;
result = result && getTagsList()
.equals(other.getTagsList());
result = result && matchType_ == other.matchType_;
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTagsCount() > 0) {
hash = (37 * hash) + TAGS_FIELD_NUMBER;
hash = (53 * hash) + getTagsList().hashCode();
}
hash = (37 * hash) + MATCH_TYPE_FIELD_NUMBER;
hash = (53 * hash) + matchType_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Matches accounts based on the generic tags applied.
*
*
* Protobuf type {@code sales.v1.AutoAssignCriteria.TagCriteria}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.AutoAssignCriteria.TagCriteria)
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteriaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_TagCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_TagCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
matchType_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_TagCriteria_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria build() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria result = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
tags_ = tags_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tags_ = tags_;
result.matchType_ = matchType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance()) return this;
if (!other.tags_.isEmpty()) {
if (tags_.isEmpty()) {
tags_ = other.tags_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTagsIsMutable();
tags_.addAll(other.tags_);
}
onChanged();
}
if (other.matchType_ != 0) {
setMatchTypeValue(other.getMatchTypeValue());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTagsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
tags_ = new com.google.protobuf.LazyStringArrayList(tags_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string tags = 1;
*/
public com.google.protobuf.ProtocolStringList
getTagsList() {
return tags_.getUnmodifiableView();
}
/**
* repeated string tags = 1;
*/
public int getTagsCount() {
return tags_.size();
}
/**
* repeated string tags = 1;
*/
public java.lang.String getTags(int index) {
return tags_.get(index);
}
/**
* repeated string tags = 1;
*/
public com.google.protobuf.ByteString
getTagsBytes(int index) {
return tags_.getByteString(index);
}
/**
* repeated string tags = 1;
*/
public Builder setTags(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.set(index, value);
onChanged();
return this;
}
/**
* repeated string tags = 1;
*/
public Builder addTags(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.add(value);
onChanged();
return this;
}
/**
* repeated string tags = 1;
*/
public Builder addAllTags(
java.lang.Iterable values) {
ensureTagsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tags_);
onChanged();
return this;
}
/**
* repeated string tags = 1;
*/
public Builder clearTags() {
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string tags = 1;
*/
public Builder addTagsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureTagsIsMutable();
tags_.add(value);
onChanged();
return this;
}
private int matchType_ = 0;
/**
* .sales.v1.TagMatchType match_type = 2;
*/
public int getMatchTypeValue() {
return matchType_;
}
/**
* .sales.v1.TagMatchType match_type = 2;
*/
public Builder setMatchTypeValue(int value) {
matchType_ = value;
onChanged();
return this;
}
/**
* .sales.v1.TagMatchType match_type = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.TagMatchType getMatchType() {
com.vendasta.sales.v1.generated.ApiProto.TagMatchType result = com.vendasta.sales.v1.generated.ApiProto.TagMatchType.valueOf(matchType_);
return result == null ? com.vendasta.sales.v1.generated.ApiProto.TagMatchType.UNRECOGNIZED : result;
}
/**
* .sales.v1.TagMatchType match_type = 2;
*/
public Builder setMatchType(com.vendasta.sales.v1.generated.ApiProto.TagMatchType value) {
if (value == null) {
throw new NullPointerException();
}
matchType_ = value.getNumber();
onChanged();
return this;
}
/**
* .sales.v1.TagMatchType match_type = 2;
*/
public Builder clearMatchType() {
matchType_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.AutoAssignCriteria.TagCriteria)
}
// @@protoc_insertion_point(class_scope:sales.v1.AutoAssignCriteria.TagCriteria)
private static final com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria();
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TagCriteria parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TagCriteria(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConversionPointCriteriaOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.AutoAssignCriteria.ConversionPointCriteria)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string conversion_point = 1;
*/
java.util.List
getConversionPointList();
/**
* repeated string conversion_point = 1;
*/
int getConversionPointCount();
/**
* repeated string conversion_point = 1;
*/
java.lang.String getConversionPoint(int index);
/**
* repeated string conversion_point = 1;
*/
com.google.protobuf.ByteString
getConversionPointBytes(int index);
}
/**
*
* Matches accounts based on the conversion_point custom field applied. (VMF Only as of Sep 2019)
*
*
* Protobuf type {@code sales.v1.AutoAssignCriteria.ConversionPointCriteria}
*/
public static final class ConversionPointCriteria extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.AutoAssignCriteria.ConversionPointCriteria)
ConversionPointCriteriaOrBuilder {
// Use ConversionPointCriteria.newBuilder() to construct.
private ConversionPointCriteria(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConversionPointCriteria() {
conversionPoint_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ConversionPointCriteria(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
conversionPoint_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
conversionPoint_.add(s);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
conversionPoint_ = conversionPoint_.getUnmodifiableView();
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder.class);
}
public static final int CONVERSION_POINT_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList conversionPoint_;
/**
* repeated string conversion_point = 1;
*/
public com.google.protobuf.ProtocolStringList
getConversionPointList() {
return conversionPoint_;
}
/**
* repeated string conversion_point = 1;
*/
public int getConversionPointCount() {
return conversionPoint_.size();
}
/**
* repeated string conversion_point = 1;
*/
public java.lang.String getConversionPoint(int index) {
return conversionPoint_.get(index);
}
/**
* repeated string conversion_point = 1;
*/
public com.google.protobuf.ByteString
getConversionPointBytes(int index) {
return conversionPoint_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < conversionPoint_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, conversionPoint_.getRaw(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < conversionPoint_.size(); i++) {
dataSize += computeStringSizeNoTag(conversionPoint_.getRaw(i));
}
size += dataSize;
size += 1 * getConversionPointList().size();
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria other = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) obj;
boolean result = true;
result = result && getConversionPointList()
.equals(other.getConversionPointList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getConversionPointCount() > 0) {
hash = (37 * hash) + CONVERSION_POINT_FIELD_NUMBER;
hash = (53 * hash) + getConversionPointList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Matches accounts based on the conversion_point custom field applied. (VMF Only as of Sep 2019)
*
*
* Protobuf type {@code sales.v1.AutoAssignCriteria.ConversionPointCriteria}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.AutoAssignCriteria.ConversionPointCriteria)
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteriaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
conversionPoint_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria build() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria result = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
conversionPoint_ = conversionPoint_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.conversionPoint_ = conversionPoint_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance()) return this;
if (!other.conversionPoint_.isEmpty()) {
if (conversionPoint_.isEmpty()) {
conversionPoint_ = other.conversionPoint_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureConversionPointIsMutable();
conversionPoint_.addAll(other.conversionPoint_);
}
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList conversionPoint_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureConversionPointIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
conversionPoint_ = new com.google.protobuf.LazyStringArrayList(conversionPoint_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string conversion_point = 1;
*/
public com.google.protobuf.ProtocolStringList
getConversionPointList() {
return conversionPoint_.getUnmodifiableView();
}
/**
* repeated string conversion_point = 1;
*/
public int getConversionPointCount() {
return conversionPoint_.size();
}
/**
* repeated string conversion_point = 1;
*/
public java.lang.String getConversionPoint(int index) {
return conversionPoint_.get(index);
}
/**
* repeated string conversion_point = 1;
*/
public com.google.protobuf.ByteString
getConversionPointBytes(int index) {
return conversionPoint_.getByteString(index);
}
/**
* repeated string conversion_point = 1;
*/
public Builder setConversionPoint(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureConversionPointIsMutable();
conversionPoint_.set(index, value);
onChanged();
return this;
}
/**
* repeated string conversion_point = 1;
*/
public Builder addConversionPoint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureConversionPointIsMutable();
conversionPoint_.add(value);
onChanged();
return this;
}
/**
* repeated string conversion_point = 1;
*/
public Builder addAllConversionPoint(
java.lang.Iterable values) {
ensureConversionPointIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, conversionPoint_);
onChanged();
return this;
}
/**
* repeated string conversion_point = 1;
*/
public Builder clearConversionPoint() {
conversionPoint_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string conversion_point = 1;
*/
public Builder addConversionPointBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureConversionPointIsMutable();
conversionPoint_.add(value);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.AutoAssignCriteria.ConversionPointCriteria)
}
// @@protoc_insertion_point(class_scope:sales.v1.AutoAssignCriteria.ConversionPointCriteria)
private static final com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria();
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ConversionPointCriteria parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConversionPointCriteria(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int criteriaCase_ = 0;
private java.lang.Object criteria_;
public enum CriteriaCase
implements com.google.protobuf.Internal.EnumLite {
LOCATION_CRITERIA(1),
CATEGORY_CRITERIA(2),
TAG_CRITERIA(3),
CONVERSION_POINT_CRITERIA(4),
CRITERIA_NOT_SET(0);
private final int value;
private CriteriaCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CriteriaCase valueOf(int value) {
return forNumber(value);
}
public static CriteriaCase forNumber(int value) {
switch (value) {
case 1: return LOCATION_CRITERIA;
case 2: return CATEGORY_CRITERIA;
case 3: return TAG_CRITERIA;
case 4: return CONVERSION_POINT_CRITERIA;
case 0: return CRITERIA_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public CriteriaCase
getCriteriaCase() {
return CriteriaCase.forNumber(
criteriaCase_);
}
public static final int LOCATION_CRITERIA_FIELD_NUMBER = 1;
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria getLocationCriteria() {
if (criteriaCase_ == 1) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance();
}
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteriaOrBuilder getLocationCriteriaOrBuilder() {
if (criteriaCase_ == 1) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance();
}
public static final int CATEGORY_CRITERIA_FIELD_NUMBER = 2;
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria getCategoryCriteria() {
if (criteriaCase_ == 2) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance();
}
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteriaOrBuilder getCategoryCriteriaOrBuilder() {
if (criteriaCase_ == 2) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance();
}
public static final int TAG_CRITERIA_FIELD_NUMBER = 3;
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria getTagCriteria() {
if (criteriaCase_ == 3) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance();
}
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteriaOrBuilder getTagCriteriaOrBuilder() {
if (criteriaCase_ == 3) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance();
}
public static final int CONVERSION_POINT_CRITERIA_FIELD_NUMBER = 4;
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria getConversionPointCriteria() {
if (criteriaCase_ == 4) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance();
}
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteriaOrBuilder getConversionPointCriteriaOrBuilder() {
if (criteriaCase_ == 4) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (criteriaCase_ == 1) {
output.writeMessage(1, (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_);
}
if (criteriaCase_ == 2) {
output.writeMessage(2, (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_);
}
if (criteriaCase_ == 3) {
output.writeMessage(3, (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_);
}
if (criteriaCase_ == 4) {
output.writeMessage(4, (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (criteriaCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_);
}
if (criteriaCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_);
}
if (criteriaCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_);
}
if (criteriaCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria other = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria) obj;
boolean result = true;
result = result && getCriteriaCase().equals(
other.getCriteriaCase());
if (!result) return false;
switch (criteriaCase_) {
case 1:
result = result && getLocationCriteria()
.equals(other.getLocationCriteria());
break;
case 2:
result = result && getCategoryCriteria()
.equals(other.getCategoryCriteria());
break;
case 3:
result = result && getTagCriteria()
.equals(other.getTagCriteria());
break;
case 4:
result = result && getConversionPointCriteria()
.equals(other.getConversionPointCriteria());
break;
case 0:
default:
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (criteriaCase_) {
case 1:
hash = (37 * hash) + LOCATION_CRITERIA_FIELD_NUMBER;
hash = (53 * hash) + getLocationCriteria().hashCode();
break;
case 2:
hash = (37 * hash) + CATEGORY_CRITERIA_FIELD_NUMBER;
hash = (53 * hash) + getCategoryCriteria().hashCode();
break;
case 3:
hash = (37 * hash) + TAG_CRITERIA_FIELD_NUMBER;
hash = (53 * hash) + getTagCriteria().hashCode();
break;
case 4:
hash = (37 * hash) + CONVERSION_POINT_CRITERIA_FIELD_NUMBER;
hash = (53 * hash) + getConversionPointCriteria().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.AutoAssignCriteria}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.AutoAssignCriteria)
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
criteriaCase_ = 0;
criteria_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignCriteria_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria build() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria result = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria(this);
if (criteriaCase_ == 1) {
if (locationCriteriaBuilder_ == null) {
result.criteria_ = criteria_;
} else {
result.criteria_ = locationCriteriaBuilder_.build();
}
}
if (criteriaCase_ == 2) {
if (categoryCriteriaBuilder_ == null) {
result.criteria_ = criteria_;
} else {
result.criteria_ = categoryCriteriaBuilder_.build();
}
}
if (criteriaCase_ == 3) {
if (tagCriteriaBuilder_ == null) {
result.criteria_ = criteria_;
} else {
result.criteria_ = tagCriteriaBuilder_.build();
}
}
if (criteriaCase_ == 4) {
if (conversionPointCriteriaBuilder_ == null) {
result.criteria_ = criteria_;
} else {
result.criteria_ = conversionPointCriteriaBuilder_.build();
}
}
result.criteriaCase_ = criteriaCase_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.getDefaultInstance()) return this;
switch (other.getCriteriaCase()) {
case LOCATION_CRITERIA: {
mergeLocationCriteria(other.getLocationCriteria());
break;
}
case CATEGORY_CRITERIA: {
mergeCategoryCriteria(other.getCategoryCriteria());
break;
}
case TAG_CRITERIA: {
mergeTagCriteria(other.getTagCriteria());
break;
}
case CONVERSION_POINT_CRITERIA: {
mergeConversionPointCriteria(other.getConversionPointCriteria());
break;
}
case CRITERIA_NOT_SET: {
break;
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int criteriaCase_ = 0;
private java.lang.Object criteria_;
public CriteriaCase
getCriteriaCase() {
return CriteriaCase.forNumber(
criteriaCase_);
}
public Builder clearCriteria() {
criteriaCase_ = 0;
criteria_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteriaOrBuilder> locationCriteriaBuilder_;
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria getLocationCriteria() {
if (locationCriteriaBuilder_ == null) {
if (criteriaCase_ == 1) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance();
} else {
if (criteriaCase_ == 1) {
return locationCriteriaBuilder_.getMessage();
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance();
}
}
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public Builder setLocationCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria value) {
if (locationCriteriaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
criteria_ = value;
onChanged();
} else {
locationCriteriaBuilder_.setMessage(value);
}
criteriaCase_ = 1;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public Builder setLocationCriteria(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.Builder builderForValue) {
if (locationCriteriaBuilder_ == null) {
criteria_ = builderForValue.build();
onChanged();
} else {
locationCriteriaBuilder_.setMessage(builderForValue.build());
}
criteriaCase_ = 1;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public Builder mergeLocationCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria value) {
if (locationCriteriaBuilder_ == null) {
if (criteriaCase_ == 1 &&
criteria_ != com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance()) {
criteria_ = com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.newBuilder((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_)
.mergeFrom(value).buildPartial();
} else {
criteria_ = value;
}
onChanged();
} else {
if (criteriaCase_ == 1) {
locationCriteriaBuilder_.mergeFrom(value);
}
locationCriteriaBuilder_.setMessage(value);
}
criteriaCase_ = 1;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public Builder clearLocationCriteria() {
if (locationCriteriaBuilder_ == null) {
if (criteriaCase_ == 1) {
criteriaCase_ = 0;
criteria_ = null;
onChanged();
}
} else {
if (criteriaCase_ == 1) {
criteriaCase_ = 0;
criteria_ = null;
}
locationCriteriaBuilder_.clear();
}
return this;
}
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.Builder getLocationCriteriaBuilder() {
return getLocationCriteriaFieldBuilder().getBuilder();
}
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteriaOrBuilder getLocationCriteriaOrBuilder() {
if ((criteriaCase_ == 1) && (locationCriteriaBuilder_ != null)) {
return locationCriteriaBuilder_.getMessageOrBuilder();
} else {
if (criteriaCase_ == 1) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance();
}
}
/**
* .sales.v1.AutoAssignCriteria.LocationCriteria location_criteria = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteriaOrBuilder>
getLocationCriteriaFieldBuilder() {
if (locationCriteriaBuilder_ == null) {
if (!(criteriaCase_ == 1)) {
criteria_ = com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.getDefaultInstance();
}
locationCriteriaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteriaOrBuilder>(
(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.LocationCriteria) criteria_,
getParentForChildren(),
isClean());
criteria_ = null;
}
criteriaCase_ = 1;
onChanged();;
return locationCriteriaBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteriaOrBuilder> categoryCriteriaBuilder_;
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria getCategoryCriteria() {
if (categoryCriteriaBuilder_ == null) {
if (criteriaCase_ == 2) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance();
} else {
if (criteriaCase_ == 2) {
return categoryCriteriaBuilder_.getMessage();
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance();
}
}
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public Builder setCategoryCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria value) {
if (categoryCriteriaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
criteria_ = value;
onChanged();
} else {
categoryCriteriaBuilder_.setMessage(value);
}
criteriaCase_ = 2;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public Builder setCategoryCriteria(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.Builder builderForValue) {
if (categoryCriteriaBuilder_ == null) {
criteria_ = builderForValue.build();
onChanged();
} else {
categoryCriteriaBuilder_.setMessage(builderForValue.build());
}
criteriaCase_ = 2;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public Builder mergeCategoryCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria value) {
if (categoryCriteriaBuilder_ == null) {
if (criteriaCase_ == 2 &&
criteria_ != com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance()) {
criteria_ = com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.newBuilder((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_)
.mergeFrom(value).buildPartial();
} else {
criteria_ = value;
}
onChanged();
} else {
if (criteriaCase_ == 2) {
categoryCriteriaBuilder_.mergeFrom(value);
}
categoryCriteriaBuilder_.setMessage(value);
}
criteriaCase_ = 2;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public Builder clearCategoryCriteria() {
if (categoryCriteriaBuilder_ == null) {
if (criteriaCase_ == 2) {
criteriaCase_ = 0;
criteria_ = null;
onChanged();
}
} else {
if (criteriaCase_ == 2) {
criteriaCase_ = 0;
criteria_ = null;
}
categoryCriteriaBuilder_.clear();
}
return this;
}
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.Builder getCategoryCriteriaBuilder() {
return getCategoryCriteriaFieldBuilder().getBuilder();
}
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteriaOrBuilder getCategoryCriteriaOrBuilder() {
if ((criteriaCase_ == 2) && (categoryCriteriaBuilder_ != null)) {
return categoryCriteriaBuilder_.getMessageOrBuilder();
} else {
if (criteriaCase_ == 2) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance();
}
}
/**
* .sales.v1.AutoAssignCriteria.CategoryCriteria category_criteria = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteriaOrBuilder>
getCategoryCriteriaFieldBuilder() {
if (categoryCriteriaBuilder_ == null) {
if (!(criteriaCase_ == 2)) {
criteria_ = com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.getDefaultInstance();
}
categoryCriteriaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteriaOrBuilder>(
(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.CategoryCriteria) criteria_,
getParentForChildren(),
isClean());
criteria_ = null;
}
criteriaCase_ = 2;
onChanged();;
return categoryCriteriaBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteriaOrBuilder> tagCriteriaBuilder_;
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria getTagCriteria() {
if (tagCriteriaBuilder_ == null) {
if (criteriaCase_ == 3) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance();
} else {
if (criteriaCase_ == 3) {
return tagCriteriaBuilder_.getMessage();
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance();
}
}
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public Builder setTagCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria value) {
if (tagCriteriaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
criteria_ = value;
onChanged();
} else {
tagCriteriaBuilder_.setMessage(value);
}
criteriaCase_ = 3;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public Builder setTagCriteria(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.Builder builderForValue) {
if (tagCriteriaBuilder_ == null) {
criteria_ = builderForValue.build();
onChanged();
} else {
tagCriteriaBuilder_.setMessage(builderForValue.build());
}
criteriaCase_ = 3;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public Builder mergeTagCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria value) {
if (tagCriteriaBuilder_ == null) {
if (criteriaCase_ == 3 &&
criteria_ != com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance()) {
criteria_ = com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.newBuilder((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_)
.mergeFrom(value).buildPartial();
} else {
criteria_ = value;
}
onChanged();
} else {
if (criteriaCase_ == 3) {
tagCriteriaBuilder_.mergeFrom(value);
}
tagCriteriaBuilder_.setMessage(value);
}
criteriaCase_ = 3;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public Builder clearTagCriteria() {
if (tagCriteriaBuilder_ == null) {
if (criteriaCase_ == 3) {
criteriaCase_ = 0;
criteria_ = null;
onChanged();
}
} else {
if (criteriaCase_ == 3) {
criteriaCase_ = 0;
criteria_ = null;
}
tagCriteriaBuilder_.clear();
}
return this;
}
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.Builder getTagCriteriaBuilder() {
return getTagCriteriaFieldBuilder().getBuilder();
}
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteriaOrBuilder getTagCriteriaOrBuilder() {
if ((criteriaCase_ == 3) && (tagCriteriaBuilder_ != null)) {
return tagCriteriaBuilder_.getMessageOrBuilder();
} else {
if (criteriaCase_ == 3) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance();
}
}
/**
* .sales.v1.AutoAssignCriteria.TagCriteria tag_criteria = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteriaOrBuilder>
getTagCriteriaFieldBuilder() {
if (tagCriteriaBuilder_ == null) {
if (!(criteriaCase_ == 3)) {
criteria_ = com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.getDefaultInstance();
}
tagCriteriaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteriaOrBuilder>(
(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.TagCriteria) criteria_,
getParentForChildren(),
isClean());
criteria_ = null;
}
criteriaCase_ = 3;
onChanged();;
return tagCriteriaBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteriaOrBuilder> conversionPointCriteriaBuilder_;
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria getConversionPointCriteria() {
if (conversionPointCriteriaBuilder_ == null) {
if (criteriaCase_ == 4) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance();
} else {
if (criteriaCase_ == 4) {
return conversionPointCriteriaBuilder_.getMessage();
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance();
}
}
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public Builder setConversionPointCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria value) {
if (conversionPointCriteriaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
criteria_ = value;
onChanged();
} else {
conversionPointCriteriaBuilder_.setMessage(value);
}
criteriaCase_ = 4;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public Builder setConversionPointCriteria(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder builderForValue) {
if (conversionPointCriteriaBuilder_ == null) {
criteria_ = builderForValue.build();
onChanged();
} else {
conversionPointCriteriaBuilder_.setMessage(builderForValue.build());
}
criteriaCase_ = 4;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public Builder mergeConversionPointCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria value) {
if (conversionPointCriteriaBuilder_ == null) {
if (criteriaCase_ == 4 &&
criteria_ != com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance()) {
criteria_ = com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.newBuilder((com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_)
.mergeFrom(value).buildPartial();
} else {
criteria_ = value;
}
onChanged();
} else {
if (criteriaCase_ == 4) {
conversionPointCriteriaBuilder_.mergeFrom(value);
}
conversionPointCriteriaBuilder_.setMessage(value);
}
criteriaCase_ = 4;
return this;
}
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public Builder clearConversionPointCriteria() {
if (conversionPointCriteriaBuilder_ == null) {
if (criteriaCase_ == 4) {
criteriaCase_ = 0;
criteria_ = null;
onChanged();
}
} else {
if (criteriaCase_ == 4) {
criteriaCase_ = 0;
criteria_ = null;
}
conversionPointCriteriaBuilder_.clear();
}
return this;
}
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder getConversionPointCriteriaBuilder() {
return getConversionPointCriteriaFieldBuilder().getBuilder();
}
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteriaOrBuilder getConversionPointCriteriaOrBuilder() {
if ((criteriaCase_ == 4) && (conversionPointCriteriaBuilder_ != null)) {
return conversionPointCriteriaBuilder_.getMessageOrBuilder();
} else {
if (criteriaCase_ == 4) {
return (com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_;
}
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance();
}
}
/**
* .sales.v1.AutoAssignCriteria.ConversionPointCriteria conversion_point_criteria = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteriaOrBuilder>
getConversionPointCriteriaFieldBuilder() {
if (conversionPointCriteriaBuilder_ == null) {
if (!(criteriaCase_ == 4)) {
criteria_ = com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.getDefaultInstance();
}
conversionPointCriteriaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteriaOrBuilder>(
(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.ConversionPointCriteria) criteria_,
getParentForChildren(),
isClean());
criteria_ = null;
}
criteriaCase_ = 4;
onChanged();;
return conversionPointCriteriaBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.AutoAssignCriteria)
}
// @@protoc_insertion_point(class_scope:sales.v1.AutoAssignCriteria)
private static final com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria();
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AutoAssignCriteria parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AutoAssignCriteria(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AutoAssignRuleOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.AutoAssignRule)
com.google.protobuf.MessageOrBuilder {
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
java.util.List
getCriteriaList();
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria getCriteria(int index);
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
int getCriteriaCount();
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder>
getCriteriaOrBuilderList();
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder getCriteriaOrBuilder(
int index);
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
java.util.List
getSalespersonIdsList();
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
int getSalespersonIdsCount();
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
java.lang.String getSalespersonIds(int index);
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
com.google.protobuf.ByteString
getSalespersonIdsBytes(int index);
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
int getCriteriaMatchTypeValue();
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType getCriteriaMatchType();
}
/**
* Protobuf type {@code sales.v1.AutoAssignRule}
*/
public static final class AutoAssignRule extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.AutoAssignRule)
AutoAssignRuleOrBuilder {
// Use AutoAssignRule.newBuilder() to construct.
private AutoAssignRule(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AutoAssignRule() {
criteria_ = java.util.Collections.emptyList();
salespersonIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
criteriaMatchType_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private AutoAssignRule(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
criteria_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
criteria_.add(
input.readMessage(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.parser(), extensionRegistry));
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
salespersonIds_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
salespersonIds_.add(s);
break;
}
case 24: {
int rawValue = input.readEnum();
criteriaMatchType_ = rawValue;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
criteria_ = java.util.Collections.unmodifiableList(criteria_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
salespersonIds_ = salespersonIds_.getUnmodifiableView();
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignRule_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder.class);
}
private int bitField0_;
public static final int CRITERIA_FIELD_NUMBER = 1;
private java.util.List criteria_;
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public java.util.List getCriteriaList() {
return criteria_;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder>
getCriteriaOrBuilderList() {
return criteria_;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public int getCriteriaCount() {
return criteria_.size();
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria getCriteria(int index) {
return criteria_.get(index);
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder getCriteriaOrBuilder(
int index) {
return criteria_.get(index);
}
public static final int SALESPERSON_IDS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList salespersonIds_;
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public com.google.protobuf.ProtocolStringList
getSalespersonIdsList() {
return salespersonIds_;
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public int getSalespersonIdsCount() {
return salespersonIds_.size();
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public java.lang.String getSalespersonIds(int index) {
return salespersonIds_.get(index);
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public com.google.protobuf.ByteString
getSalespersonIdsBytes(int index) {
return salespersonIds_.getByteString(index);
}
public static final int CRITERIA_MATCH_TYPE_FIELD_NUMBER = 3;
private int criteriaMatchType_;
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
public int getCriteriaMatchTypeValue() {
return criteriaMatchType_;
}
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType getCriteriaMatchType() {
com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType result = com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType.valueOf(criteriaMatchType_);
return result == null ? com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < criteria_.size(); i++) {
output.writeMessage(1, criteria_.get(i));
}
for (int i = 0; i < salespersonIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, salespersonIds_.getRaw(i));
}
if (criteriaMatchType_ != com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType.CRITERIA_MATCH_TYPE_ALL.getNumber()) {
output.writeEnum(3, criteriaMatchType_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < criteria_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, criteria_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < salespersonIds_.size(); i++) {
dataSize += computeStringSizeNoTag(salespersonIds_.getRaw(i));
}
size += dataSize;
size += 1 * getSalespersonIdsList().size();
}
if (criteriaMatchType_ != com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType.CRITERIA_MATCH_TYPE_ALL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, criteriaMatchType_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule other = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule) obj;
boolean result = true;
result = result && getCriteriaList()
.equals(other.getCriteriaList());
result = result && getSalespersonIdsList()
.equals(other.getSalespersonIdsList());
result = result && criteriaMatchType_ == other.criteriaMatchType_;
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCriteriaCount() > 0) {
hash = (37 * hash) + CRITERIA_FIELD_NUMBER;
hash = (53 * hash) + getCriteriaList().hashCode();
}
if (getSalespersonIdsCount() > 0) {
hash = (37 * hash) + SALESPERSON_IDS_FIELD_NUMBER;
hash = (53 * hash) + getSalespersonIdsList().hashCode();
}
hash = (37 * hash) + CRITERIA_MATCH_TYPE_FIELD_NUMBER;
hash = (53 * hash) + criteriaMatchType_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.AutoAssignRule}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.AutoAssignRule)
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignRule_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.class, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCriteriaFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (criteriaBuilder_ == null) {
criteria_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
criteriaBuilder_.clear();
}
salespersonIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
criteriaMatchType_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_AutoAssignRule_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule build() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule result = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (criteriaBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
criteria_ = java.util.Collections.unmodifiableList(criteria_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.criteria_ = criteria_;
} else {
result.criteria_ = criteriaBuilder_.build();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
salespersonIds_ = salespersonIds_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.salespersonIds_ = salespersonIds_;
result.criteriaMatchType_ = criteriaMatchType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.getDefaultInstance()) return this;
if (criteriaBuilder_ == null) {
if (!other.criteria_.isEmpty()) {
if (criteria_.isEmpty()) {
criteria_ = other.criteria_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCriteriaIsMutable();
criteria_.addAll(other.criteria_);
}
onChanged();
}
} else {
if (!other.criteria_.isEmpty()) {
if (criteriaBuilder_.isEmpty()) {
criteriaBuilder_.dispose();
criteriaBuilder_ = null;
criteria_ = other.criteria_;
bitField0_ = (bitField0_ & ~0x00000001);
criteriaBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCriteriaFieldBuilder() : null;
} else {
criteriaBuilder_.addAllMessages(other.criteria_);
}
}
}
if (!other.salespersonIds_.isEmpty()) {
if (salespersonIds_.isEmpty()) {
salespersonIds_ = other.salespersonIds_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSalespersonIdsIsMutable();
salespersonIds_.addAll(other.salespersonIds_);
}
onChanged();
}
if (other.criteriaMatchType_ != 0) {
setCriteriaMatchTypeValue(other.getCriteriaMatchTypeValue());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List criteria_ =
java.util.Collections.emptyList();
private void ensureCriteriaIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
criteria_ = new java.util.ArrayList(criteria_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder> criteriaBuilder_;
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public java.util.List getCriteriaList() {
if (criteriaBuilder_ == null) {
return java.util.Collections.unmodifiableList(criteria_);
} else {
return criteriaBuilder_.getMessageList();
}
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public int getCriteriaCount() {
if (criteriaBuilder_ == null) {
return criteria_.size();
} else {
return criteriaBuilder_.getCount();
}
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria getCriteria(int index) {
if (criteriaBuilder_ == null) {
return criteria_.get(index);
} else {
return criteriaBuilder_.getMessage(index);
}
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder setCriteria(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria value) {
if (criteriaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCriteriaIsMutable();
criteria_.set(index, value);
onChanged();
} else {
criteriaBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder setCriteria(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder builderForValue) {
if (criteriaBuilder_ == null) {
ensureCriteriaIsMutable();
criteria_.set(index, builderForValue.build());
onChanged();
} else {
criteriaBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder addCriteria(com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria value) {
if (criteriaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCriteriaIsMutable();
criteria_.add(value);
onChanged();
} else {
criteriaBuilder_.addMessage(value);
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder addCriteria(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria value) {
if (criteriaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCriteriaIsMutable();
criteria_.add(index, value);
onChanged();
} else {
criteriaBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder addCriteria(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder builderForValue) {
if (criteriaBuilder_ == null) {
ensureCriteriaIsMutable();
criteria_.add(builderForValue.build());
onChanged();
} else {
criteriaBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder addCriteria(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder builderForValue) {
if (criteriaBuilder_ == null) {
ensureCriteriaIsMutable();
criteria_.add(index, builderForValue.build());
onChanged();
} else {
criteriaBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder addAllCriteria(
java.lang.Iterable extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria> values) {
if (criteriaBuilder_ == null) {
ensureCriteriaIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, criteria_);
onChanged();
} else {
criteriaBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder clearCriteria() {
if (criteriaBuilder_ == null) {
criteria_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
criteriaBuilder_.clear();
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public Builder removeCriteria(int index) {
if (criteriaBuilder_ == null) {
ensureCriteriaIsMutable();
criteria_.remove(index);
onChanged();
} else {
criteriaBuilder_.remove(index);
}
return this;
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder getCriteriaBuilder(
int index) {
return getCriteriaFieldBuilder().getBuilder(index);
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder getCriteriaOrBuilder(
int index) {
if (criteriaBuilder_ == null) {
return criteria_.get(index); } else {
return criteriaBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder>
getCriteriaOrBuilderList() {
if (criteriaBuilder_ != null) {
return criteriaBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(criteria_);
}
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder addCriteriaBuilder() {
return getCriteriaFieldBuilder().addBuilder(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.getDefaultInstance());
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder addCriteriaBuilder(
int index) {
return getCriteriaFieldBuilder().addBuilder(
index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.getDefaultInstance());
}
/**
*
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
*
*
* repeated .sales.v1.AutoAssignCriteria criteria = 1;
*/
public java.util.List
getCriteriaBuilderList() {
return getCriteriaFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder>
getCriteriaFieldBuilder() {
if (criteriaBuilder_ == null) {
criteriaBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteria.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignCriteriaOrBuilder>(
criteria_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
criteria_ = null;
}
return criteriaBuilder_;
}
private com.google.protobuf.LazyStringList salespersonIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSalespersonIdsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
salespersonIds_ = new com.google.protobuf.LazyStringArrayList(salespersonIds_);
bitField0_ |= 0x00000002;
}
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public com.google.protobuf.ProtocolStringList
getSalespersonIdsList() {
return salespersonIds_.getUnmodifiableView();
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public int getSalespersonIdsCount() {
return salespersonIds_.size();
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public java.lang.String getSalespersonIds(int index) {
return salespersonIds_.get(index);
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public com.google.protobuf.ByteString
getSalespersonIdsBytes(int index) {
return salespersonIds_.getByteString(index);
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public Builder setSalespersonIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSalespersonIdsIsMutable();
salespersonIds_.set(index, value);
onChanged();
return this;
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public Builder addSalespersonIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSalespersonIdsIsMutable();
salespersonIds_.add(value);
onChanged();
return this;
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public Builder addAllSalespersonIds(
java.lang.Iterable values) {
ensureSalespersonIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, salespersonIds_);
onChanged();
return this;
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public Builder clearSalespersonIds() {
salespersonIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* The salespeople that are eligible to be assigned accounts that meet the criteria.
*
*
* repeated string salesperson_ids = 2;
*/
public Builder addSalespersonIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSalespersonIdsIsMutable();
salespersonIds_.add(value);
onChanged();
return this;
}
private int criteriaMatchType_ = 0;
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
public int getCriteriaMatchTypeValue() {
return criteriaMatchType_;
}
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
public Builder setCriteriaMatchTypeValue(int value) {
criteriaMatchType_ = value;
onChanged();
return this;
}
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType getCriteriaMatchType() {
com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType result = com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType.valueOf(criteriaMatchType_);
return result == null ? com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType.UNRECOGNIZED : result;
}
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
public Builder setCriteriaMatchType(com.vendasta.sales.v1.generated.ApiProto.CriteriaMatchType value) {
if (value == null) {
throw new NullPointerException();
}
criteriaMatchType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
*
*
* .sales.v1.CriteriaMatchType criteria_match_type = 3;
*/
public Builder clearCriteriaMatchType() {
criteriaMatchType_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.AutoAssignRule)
}
// @@protoc_insertion_point(class_scope:sales.v1.AutoAssignRule)
private static final com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule();
}
public static com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AutoAssignRule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AutoAssignRule(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetAutoAssignConfigRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.GetAutoAssignConfigRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
java.lang.String getPartnerId();
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
com.google.protobuf.ByteString
getPartnerIdBytes();
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
java.lang.String getMarketId();
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
com.google.protobuf.ByteString
getMarketIdBytes();
}
/**
* Protobuf type {@code sales.v1.GetAutoAssignConfigRequest}
*/
public static final class GetAutoAssignConfigRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.GetAutoAssignConfigRequest)
GetAutoAssignConfigRequestOrBuilder {
// Use GetAutoAssignConfigRequest.newBuilder() to construct.
private GetAutoAssignConfigRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetAutoAssignConfigRequest() {
partnerId_ = "";
marketId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetAutoAssignConfigRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
partnerId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
marketId_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest.class, com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest.Builder.class);
}
public static final int PARTNER_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object partnerId_;
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MARKET_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object marketId_;
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, marketId_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, marketId_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest other = (com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest) obj;
boolean result = true;
result = result && getPartnerId()
.equals(other.getPartnerId());
result = result && getMarketId()
.equals(other.getMarketId());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartnerId().hashCode();
hash = (37 * hash) + MARKET_ID_FIELD_NUMBER;
hash = (53 * hash) + getMarketId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.GetAutoAssignConfigRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.GetAutoAssignConfigRequest)
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest.class, com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
partnerId_ = "";
marketId_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigRequest_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest build() {
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest result = new com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest(this);
result.partnerId_ = partnerId_;
result.marketId_ = marketId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest.getDefaultInstance()) return this;
if (!other.getPartnerId().isEmpty()) {
partnerId_ = other.partnerId_;
onChanged();
}
if (!other.getMarketId().isEmpty()) {
marketId_ = other.marketId_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object partnerId_ = "";
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder setPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partnerId_ = value;
onChanged();
return this;
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder clearPartnerId() {
partnerId_ = getDefaultInstance().getPartnerId();
onChanged();
return this;
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder setPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partnerId_ = value;
onChanged();
return this;
}
private java.lang.Object marketId_ = "";
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder setMarketId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
marketId_ = value;
onChanged();
return this;
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder clearMarketId() {
marketId_ = getDefaultInstance().getMarketId();
onChanged();
return this;
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder setMarketIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
marketId_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.GetAutoAssignConfigRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.GetAutoAssignConfigRequest)
private static final com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest();
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetAutoAssignConfigRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetAutoAssignConfigRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetAutoAssignConfigResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.GetAutoAssignConfigResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
java.lang.String getPartnerId();
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
com.google.protobuf.ByteString
getPartnerIdBytes();
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
java.lang.String getMarketId();
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
com.google.protobuf.ByteString
getMarketIdBytes();
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
java.util.List
getRulesList();
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getRules(int index);
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
int getRulesCount();
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>
getRulesOrBuilderList();
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder getRulesOrBuilder(
int index);
}
/**
* Protobuf type {@code sales.v1.GetAutoAssignConfigResponse}
*/
public static final class GetAutoAssignConfigResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.GetAutoAssignConfigResponse)
GetAutoAssignConfigResponseOrBuilder {
// Use GetAutoAssignConfigResponse.newBuilder() to construct.
private GetAutoAssignConfigResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetAutoAssignConfigResponse() {
partnerId_ = "";
marketId_ = "";
rules_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetAutoAssignConfigResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
partnerId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
marketId_ = s;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
rules_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
rules_.add(
input.readMessage(com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
rules_ = java.util.Collections.unmodifiableList(rules_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse.class, com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse.Builder.class);
}
private int bitField0_;
public static final int PARTNER_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object partnerId_;
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MARKET_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object marketId_;
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RULES_FIELD_NUMBER = 3;
private java.util.List rules_;
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List getRulesList() {
return rules_;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>
getRulesOrBuilderList() {
return rules_;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public int getRulesCount() {
return rules_.size();
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getRules(int index) {
return rules_.get(index);
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder getRulesOrBuilder(
int index) {
return rules_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, marketId_);
}
for (int i = 0; i < rules_.size(); i++) {
output.writeMessage(3, rules_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, marketId_);
}
for (int i = 0; i < rules_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, rules_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse other = (com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse) obj;
boolean result = true;
result = result && getPartnerId()
.equals(other.getPartnerId());
result = result && getMarketId()
.equals(other.getMarketId());
result = result && getRulesList()
.equals(other.getRulesList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartnerId().hashCode();
hash = (37 * hash) + MARKET_ID_FIELD_NUMBER;
hash = (53 * hash) + getMarketId().hashCode();
if (getRulesCount() > 0) {
hash = (37 * hash) + RULES_FIELD_NUMBER;
hash = (53 * hash) + getRulesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.GetAutoAssignConfigResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.GetAutoAssignConfigResponse)
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse.class, com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRulesFieldBuilder();
}
}
public Builder clear() {
super.clear();
partnerId_ = "";
marketId_ = "";
if (rulesBuilder_ == null) {
rules_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
rulesBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetAutoAssignConfigResponse_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse build() {
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse result = new com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.partnerId_ = partnerId_;
result.marketId_ = marketId_;
if (rulesBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
rules_ = java.util.Collections.unmodifiableList(rules_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.rules_ = rules_;
} else {
result.rules_ = rulesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse.getDefaultInstance()) return this;
if (!other.getPartnerId().isEmpty()) {
partnerId_ = other.partnerId_;
onChanged();
}
if (!other.getMarketId().isEmpty()) {
marketId_ = other.marketId_;
onChanged();
}
if (rulesBuilder_ == null) {
if (!other.rules_.isEmpty()) {
if (rules_.isEmpty()) {
rules_ = other.rules_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureRulesIsMutable();
rules_.addAll(other.rules_);
}
onChanged();
}
} else {
if (!other.rules_.isEmpty()) {
if (rulesBuilder_.isEmpty()) {
rulesBuilder_.dispose();
rulesBuilder_ = null;
rules_ = other.rules_;
bitField0_ = (bitField0_ & ~0x00000004);
rulesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRulesFieldBuilder() : null;
} else {
rulesBuilder_.addAllMessages(other.rules_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object partnerId_ = "";
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder setPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partnerId_ = value;
onChanged();
return this;
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder clearPartnerId() {
partnerId_ = getDefaultInstance().getPartnerId();
onChanged();
return this;
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder setPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partnerId_ = value;
onChanged();
return this;
}
private java.lang.Object marketId_ = "";
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder setMarketId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
marketId_ = value;
onChanged();
return this;
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder clearMarketId() {
marketId_ = getDefaultInstance().getMarketId();
onChanged();
return this;
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder setMarketIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
marketId_ = value;
onChanged();
return this;
}
private java.util.List rules_ =
java.util.Collections.emptyList();
private void ensureRulesIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
rules_ = new java.util.ArrayList(rules_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder> rulesBuilder_;
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List getRulesList() {
if (rulesBuilder_ == null) {
return java.util.Collections.unmodifiableList(rules_);
} else {
return rulesBuilder_.getMessageList();
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public int getRulesCount() {
if (rulesBuilder_ == null) {
return rules_.size();
} else {
return rulesBuilder_.getCount();
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getRules(int index) {
if (rulesBuilder_ == null) {
return rules_.get(index);
} else {
return rulesBuilder_.getMessage(index);
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder setRules(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.set(index, value);
onChanged();
} else {
rulesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder setRules(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.set(index, builderForValue.build());
onChanged();
} else {
rulesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addRules(com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.add(value);
onChanged();
} else {
rulesBuilder_.addMessage(value);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addRules(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.add(index, value);
onChanged();
} else {
rulesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addRules(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.add(builderForValue.build());
onChanged();
} else {
rulesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addRules(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.add(index, builderForValue.build());
onChanged();
} else {
rulesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addAllRules(
java.lang.Iterable extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule> values) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rules_);
onChanged();
} else {
rulesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder clearRules() {
if (rulesBuilder_ == null) {
rules_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
rulesBuilder_.clear();
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder removeRules(int index) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.remove(index);
onChanged();
} else {
rulesBuilder_.remove(index);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder getRulesBuilder(
int index) {
return getRulesFieldBuilder().getBuilder(index);
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder getRulesOrBuilder(
int index) {
if (rulesBuilder_ == null) {
return rules_.get(index); } else {
return rulesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>
getRulesOrBuilderList() {
if (rulesBuilder_ != null) {
return rulesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rules_);
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder addRulesBuilder() {
return getRulesFieldBuilder().addBuilder(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.getDefaultInstance());
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder addRulesBuilder(
int index) {
return getRulesFieldBuilder().addBuilder(
index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.getDefaultInstance());
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List
getRulesBuilderList() {
return getRulesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>
getRulesFieldBuilder() {
if (rulesBuilder_ == null) {
rulesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>(
rules_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
rules_ = null;
}
return rulesBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.GetAutoAssignConfigResponse)
}
// @@protoc_insertion_point(class_scope:sales.v1.GetAutoAssignConfigResponse)
private static final com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse();
}
public static com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetAutoAssignConfigResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetAutoAssignConfigResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateOrUpdateAutoAssignConfigRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.CreateOrUpdateAutoAssignConfigRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
java.lang.String getPartnerId();
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
com.google.protobuf.ByteString
getPartnerIdBytes();
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
java.lang.String getMarketId();
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
com.google.protobuf.ByteString
getMarketIdBytes();
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
java.util.List
getRulesList();
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getRules(int index);
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
int getRulesCount();
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>
getRulesOrBuilderList();
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder getRulesOrBuilder(
int index);
}
/**
* Protobuf type {@code sales.v1.CreateOrUpdateAutoAssignConfigRequest}
*/
public static final class CreateOrUpdateAutoAssignConfigRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.CreateOrUpdateAutoAssignConfigRequest)
CreateOrUpdateAutoAssignConfigRequestOrBuilder {
// Use CreateOrUpdateAutoAssignConfigRequest.newBuilder() to construct.
private CreateOrUpdateAutoAssignConfigRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateOrUpdateAutoAssignConfigRequest() {
partnerId_ = "";
marketId_ = "";
rules_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private CreateOrUpdateAutoAssignConfigRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
partnerId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
marketId_ = s;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
rules_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
rules_.add(
input.readMessage(com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
rules_ = java.util.Collections.unmodifiableList(rules_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest.class, com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest.Builder.class);
}
private int bitField0_;
public static final int PARTNER_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object partnerId_;
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MARKET_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object marketId_;
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RULES_FIELD_NUMBER = 3;
private java.util.List rules_;
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List getRulesList() {
return rules_;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>
getRulesOrBuilderList() {
return rules_;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public int getRulesCount() {
return rules_.size();
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getRules(int index) {
return rules_.get(index);
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder getRulesOrBuilder(
int index) {
return rules_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, marketId_);
}
for (int i = 0; i < rules_.size(); i++) {
output.writeMessage(3, rules_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, marketId_);
}
for (int i = 0; i < rules_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, rules_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest other = (com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest) obj;
boolean result = true;
result = result && getPartnerId()
.equals(other.getPartnerId());
result = result && getMarketId()
.equals(other.getMarketId());
result = result && getRulesList()
.equals(other.getRulesList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartnerId().hashCode();
hash = (37 * hash) + MARKET_ID_FIELD_NUMBER;
hash = (53 * hash) + getMarketId().hashCode();
if (getRulesCount() > 0) {
hash = (37 * hash) + RULES_FIELD_NUMBER;
hash = (53 * hash) + getRulesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.CreateOrUpdateAutoAssignConfigRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.CreateOrUpdateAutoAssignConfigRequest)
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest.class, com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRulesFieldBuilder();
}
}
public Builder clear() {
super.clear();
partnerId_ = "";
marketId_ = "";
if (rulesBuilder_ == null) {
rules_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
rulesBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest build() {
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest result = new com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.partnerId_ = partnerId_;
result.marketId_ = marketId_;
if (rulesBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
rules_ = java.util.Collections.unmodifiableList(rules_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.rules_ = rules_;
} else {
result.rules_ = rulesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest.getDefaultInstance()) return this;
if (!other.getPartnerId().isEmpty()) {
partnerId_ = other.partnerId_;
onChanged();
}
if (!other.getMarketId().isEmpty()) {
marketId_ = other.marketId_;
onChanged();
}
if (rulesBuilder_ == null) {
if (!other.rules_.isEmpty()) {
if (rules_.isEmpty()) {
rules_ = other.rules_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureRulesIsMutable();
rules_.addAll(other.rules_);
}
onChanged();
}
} else {
if (!other.rules_.isEmpty()) {
if (rulesBuilder_.isEmpty()) {
rulesBuilder_.dispose();
rulesBuilder_ = null;
rules_ = other.rules_;
bitField0_ = (bitField0_ & ~0x00000004);
rulesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRulesFieldBuilder() : null;
} else {
rulesBuilder_.addAllMessages(other.rules_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object partnerId_ = "";
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder setPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partnerId_ = value;
onChanged();
return this;
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder clearPartnerId() {
partnerId_ = getDefaultInstance().getPartnerId();
onChanged();
return this;
}
/**
*
* The unique partner ID.
*
*
* string partner_id = 1;
*/
public Builder setPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partnerId_ = value;
onChanged();
return this;
}
private java.lang.Object marketId_ = "";
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder setMarketId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
marketId_ = value;
onChanged();
return this;
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder clearMarketId() {
marketId_ = getDefaultInstance().getMarketId();
onChanged();
return this;
}
/**
*
* The unique market ID.
*
*
* string market_id = 2;
*/
public Builder setMarketIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
marketId_ = value;
onChanged();
return this;
}
private java.util.List rules_ =
java.util.Collections.emptyList();
private void ensureRulesIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
rules_ = new java.util.ArrayList(rules_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder> rulesBuilder_;
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List getRulesList() {
if (rulesBuilder_ == null) {
return java.util.Collections.unmodifiableList(rules_);
} else {
return rulesBuilder_.getMessageList();
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public int getRulesCount() {
if (rulesBuilder_ == null) {
return rules_.size();
} else {
return rulesBuilder_.getCount();
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule getRules(int index) {
if (rulesBuilder_ == null) {
return rules_.get(index);
} else {
return rulesBuilder_.getMessage(index);
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder setRules(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.set(index, value);
onChanged();
} else {
rulesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder setRules(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.set(index, builderForValue.build());
onChanged();
} else {
rulesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addRules(com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.add(value);
onChanged();
} else {
rulesBuilder_.addMessage(value);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addRules(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.add(index, value);
onChanged();
} else {
rulesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addRules(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.add(builderForValue.build());
onChanged();
} else {
rulesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addRules(
int index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.add(index, builderForValue.build());
onChanged();
} else {
rulesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder addAllRules(
java.lang.Iterable extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule> values) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rules_);
onChanged();
} else {
rulesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder clearRules() {
if (rulesBuilder_ == null) {
rules_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
rulesBuilder_.clear();
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public Builder removeRules(int index) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.remove(index);
onChanged();
} else {
rulesBuilder_.remove(index);
}
return this;
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder getRulesBuilder(
int index) {
return getRulesFieldBuilder().getBuilder(index);
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder getRulesOrBuilder(
int index) {
if (rulesBuilder_ == null) {
return rules_.get(index); } else {
return rulesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List extends com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>
getRulesOrBuilderList() {
if (rulesBuilder_ != null) {
return rulesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rules_);
}
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder addRulesBuilder() {
return getRulesFieldBuilder().addBuilder(
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.getDefaultInstance());
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder addRulesBuilder(
int index) {
return getRulesFieldBuilder().addBuilder(
index, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.getDefaultInstance());
}
/**
*
* The rules the define which accounts get assigned to which salespeople.
* These rules will be checked, in order, against an account using the rule's criteria, and the first matching rule (if any) is applicable.
*
*
* repeated .sales.v1.AutoAssignRule rules = 3;
*/
public java.util.List
getRulesBuilderList() {
return getRulesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>
getRulesFieldBuilder() {
if (rulesBuilder_ == null) {
rulesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRule.Builder, com.vendasta.sales.v1.generated.ApiProto.AutoAssignRuleOrBuilder>(
rules_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
rules_ = null;
}
return rulesBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.CreateOrUpdateAutoAssignConfigRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.CreateOrUpdateAutoAssignConfigRequest)
private static final com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest();
}
public static com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CreateOrUpdateAutoAssignConfigRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CreateOrUpdateAutoAssignConfigRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetSalespersonRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.GetSalespersonRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
java.lang.String getPartnerId();
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
com.google.protobuf.ByteString
getPartnerIdBytes();
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
java.lang.String getSalespersonId();
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
com.google.protobuf.ByteString
getSalespersonIdBytes();
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
java.lang.String getUserId();
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
com.google.protobuf.ByteString
getUserIdBytes();
public com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.IdCase getIdCase();
}
/**
* Protobuf type {@code sales.v1.GetSalespersonRequest}
*/
public static final class GetSalespersonRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.GetSalespersonRequest)
GetSalespersonRequestOrBuilder {
// Use GetSalespersonRequest.newBuilder() to construct.
private GetSalespersonRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetSalespersonRequest() {
partnerId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetSalespersonRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
partnerId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
idCase_ = 2;
id_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
idCase_ = 3;
id_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetSalespersonRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetSalespersonRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.class, com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.Builder.class);
}
private int idCase_ = 0;
private java.lang.Object id_;
public enum IdCase
implements com.google.protobuf.Internal.EnumLite {
SALESPERSON_ID(2),
USER_ID(3),
ID_NOT_SET(0);
private final int value;
private IdCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static IdCase valueOf(int value) {
return forNumber(value);
}
public static IdCase forNumber(int value) {
switch (value) {
case 2: return SALESPERSON_ID;
case 3: return USER_ID;
case 0: return ID_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public IdCase
getIdCase() {
return IdCase.forNumber(
idCase_);
}
public static final int PARTNER_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object partnerId_;
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
}
}
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SALESPERSON_ID_FIELD_NUMBER = 2;
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
public java.lang.String getSalespersonId() {
java.lang.Object ref = "";
if (idCase_ == 2) {
ref = id_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (idCase_ == 2) {
id_ = s;
}
return s;
}
}
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
public com.google.protobuf.ByteString
getSalespersonIdBytes() {
java.lang.Object ref = "";
if (idCase_ == 2) {
ref = id_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (idCase_ == 2) {
id_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USER_ID_FIELD_NUMBER = 3;
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
public java.lang.String getUserId() {
java.lang.Object ref = "";
if (idCase_ == 3) {
ref = id_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (idCase_ == 3) {
id_ = s;
}
return s;
}
}
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
public com.google.protobuf.ByteString
getUserIdBytes() {
java.lang.Object ref = "";
if (idCase_ == 3) {
ref = id_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (idCase_ == 3) {
id_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, partnerId_);
}
if (idCase_ == 2) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, id_);
}
if (idCase_ == 3) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, id_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, partnerId_);
}
if (idCase_ == 2) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, id_);
}
if (idCase_ == 3) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, id_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest other = (com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest) obj;
boolean result = true;
result = result && getPartnerId()
.equals(other.getPartnerId());
result = result && getIdCase().equals(
other.getIdCase());
if (!result) return false;
switch (idCase_) {
case 2:
result = result && getSalespersonId()
.equals(other.getSalespersonId());
break;
case 3:
result = result && getUserId()
.equals(other.getUserId());
break;
case 0:
default:
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartnerId().hashCode();
switch (idCase_) {
case 2:
hash = (37 * hash) + SALESPERSON_ID_FIELD_NUMBER;
hash = (53 * hash) + getSalespersonId().hashCode();
break;
case 3:
hash = (37 * hash) + USER_ID_FIELD_NUMBER;
hash = (53 * hash) + getUserId().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.GetSalespersonRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.GetSalespersonRequest)
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetSalespersonRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetSalespersonRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.class, com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
partnerId_ = "";
idCase_ = 0;
id_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_GetSalespersonRequest_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest build() {
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest result = new com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest(this);
result.partnerId_ = partnerId_;
if (idCase_ == 2) {
result.id_ = id_;
}
if (idCase_ == 3) {
result.id_ = id_;
}
result.idCase_ = idCase_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.getDefaultInstance()) return this;
if (!other.getPartnerId().isEmpty()) {
partnerId_ = other.partnerId_;
onChanged();
}
switch (other.getIdCase()) {
case SALESPERSON_ID: {
idCase_ = 2;
id_ = other.id_;
onChanged();
break;
}
case USER_ID: {
idCase_ = 3;
id_ = other.id_;
onChanged();
break;
}
case ID_NOT_SET: {
break;
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int idCase_ = 0;
private java.lang.Object id_;
public IdCase
getIdCase() {
return IdCase.forNumber(
idCase_);
}
public Builder clearId() {
idCase_ = 0;
id_ = null;
onChanged();
return this;
}
private java.lang.Object partnerId_ = "";
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
public Builder setPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partnerId_ = value;
onChanged();
return this;
}
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
public Builder clearPartnerId() {
partnerId_ = getDefaultInstance().getPartnerId();
onChanged();
return this;
}
/**
*
* partner_id is the namespace the salesperson is in
*
*
* string partner_id = 1;
*/
public Builder setPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partnerId_ = value;
onChanged();
return this;
}
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
public java.lang.String getSalespersonId() {
java.lang.Object ref = "";
if (idCase_ == 2) {
ref = id_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (idCase_ == 2) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
public com.google.protobuf.ByteString
getSalespersonIdBytes() {
java.lang.Object ref = "";
if (idCase_ == 2) {
ref = id_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (idCase_ == 2) {
id_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
public Builder setSalespersonId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
idCase_ = 2;
id_ = value;
onChanged();
return this;
}
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
public Builder clearSalespersonId() {
if (idCase_ == 2) {
idCase_ = 0;
id_ = null;
onChanged();
}
return this;
}
/**
*
* salesperson_id is the IAM SubjectID of the matching IAM Persona
*
*
* string salesperson_id = 2;
*/
public Builder setSalespersonIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
idCase_ = 2;
id_ = value;
onChanged();
return this;
}
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
public java.lang.String getUserId() {
java.lang.Object ref = "";
if (idCase_ == 3) {
ref = id_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (idCase_ == 3) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
public com.google.protobuf.ByteString
getUserIdBytes() {
java.lang.Object ref = "";
if (idCase_ == 3) {
ref = id_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (idCase_ == 3) {
id_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
public Builder setUserId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
idCase_ = 3;
id_ = value;
onChanged();
return this;
}
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
public Builder clearUserId() {
if (idCase_ == 3) {
idCase_ = 0;
id_ = null;
onChanged();
}
return this;
}
/**
*
* user_id is the IAM UserID the Subject belongs to
*
*
* string user_id = 3;
*/
public Builder setUserIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
idCase_ = 3;
id_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.GetSalespersonRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.GetSalespersonRequest)
private static final com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest();
}
public static com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetSalespersonRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetSalespersonRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PagedResponseMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.PagedResponseMetadata)
com.google.protobuf.MessageOrBuilder {
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
java.lang.String getNextCursor();
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
com.google.protobuf.ByteString
getNextCursorBytes();
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
boolean getHasMore();
}
/**
*
* Contains metadata about the paged response
*
*
* Protobuf type {@code sales.v1.PagedResponseMetadata}
*/
public static final class PagedResponseMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.PagedResponseMetadata)
PagedResponseMetadataOrBuilder {
// Use PagedResponseMetadata.newBuilder() to construct.
private PagedResponseMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PagedResponseMetadata() {
nextCursor_ = "";
hasMore_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PagedResponseMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
nextCursor_ = s;
break;
}
case 16: {
hasMore_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedResponseMetadata_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedResponseMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.class, com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.Builder.class);
}
public static final int NEXT_CURSOR_FIELD_NUMBER = 1;
private volatile java.lang.Object nextCursor_;
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public java.lang.String getNextCursor() {
java.lang.Object ref = nextCursor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nextCursor_ = s;
return s;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public com.google.protobuf.ByteString
getNextCursorBytes() {
java.lang.Object ref = nextCursor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nextCursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HAS_MORE_FIELD_NUMBER = 2;
private boolean hasMore_;
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public boolean getHasMore() {
return hasMore_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNextCursorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, nextCursor_);
}
if (hasMore_ != false) {
output.writeBool(2, hasMore_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNextCursorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, nextCursor_);
}
if (hasMore_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, hasMore_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata other = (com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata) obj;
boolean result = true;
result = result && getNextCursor()
.equals(other.getNextCursor());
result = result && (getHasMore()
== other.getHasMore());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NEXT_CURSOR_FIELD_NUMBER;
hash = (53 * hash) + getNextCursor().hashCode();
hash = (37 * hash) + HAS_MORE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasMore());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Contains metadata about the paged response
*
*
* Protobuf type {@code sales.v1.PagedResponseMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.PagedResponseMetadata)
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedResponseMetadata_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedResponseMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.class, com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
nextCursor_ = "";
hasMore_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedResponseMetadata_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata build() {
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata result = new com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata(this);
result.nextCursor_ = nextCursor_;
result.hasMore_ = hasMore_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.getDefaultInstance()) return this;
if (!other.getNextCursor().isEmpty()) {
nextCursor_ = other.nextCursor_;
onChanged();
}
if (other.getHasMore() != false) {
setHasMore(other.getHasMore());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object nextCursor_ = "";
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public java.lang.String getNextCursor() {
java.lang.Object ref = nextCursor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nextCursor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public com.google.protobuf.ByteString
getNextCursorBytes() {
java.lang.Object ref = nextCursor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nextCursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder setNextCursor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nextCursor_ = value;
onChanged();
return this;
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder clearNextCursor() {
nextCursor_ = getDefaultInstance().getNextCursor();
onChanged();
return this;
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder setNextCursorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nextCursor_ = value;
onChanged();
return this;
}
private boolean hasMore_ ;
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public boolean getHasMore() {
return hasMore_;
}
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public Builder setHasMore(boolean value) {
hasMore_ = value;
onChanged();
return this;
}
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public Builder clearHasMore() {
hasMore_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.PagedResponseMetadata)
}
// @@protoc_insertion_point(class_scope:sales.v1.PagedResponseMetadata)
private static final com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata();
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PagedResponseMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PagedResponseMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PagedRequestOptionsOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.PagedRequestOptions)
com.google.protobuf.MessageOrBuilder {
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
java.lang.String getCursor();
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
com.google.protobuf.ByteString
getCursorBytes();
/**
*
* page_size specifies the number of items to return in the next page
*
*
* int64 page_size = 2;
*/
long getPageSize();
}
/**
*
* To provide options for the paged request
*
*
* Protobuf type {@code sales.v1.PagedRequestOptions}
*/
public static final class PagedRequestOptions extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.PagedRequestOptions)
PagedRequestOptionsOrBuilder {
// Use PagedRequestOptions.newBuilder() to construct.
private PagedRequestOptions(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PagedRequestOptions() {
cursor_ = "";
pageSize_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private PagedRequestOptions(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
cursor_ = s;
break;
}
case 16: {
pageSize_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedRequestOptions_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedRequestOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.class, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder.class);
}
public static final int CURSOR_FIELD_NUMBER = 1;
private volatile java.lang.Object cursor_;
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
public java.lang.String getCursor() {
java.lang.Object ref = cursor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cursor_ = s;
return s;
}
}
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
public com.google.protobuf.ByteString
getCursorBytes() {
java.lang.Object ref = cursor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAGE_SIZE_FIELD_NUMBER = 2;
private long pageSize_;
/**
*
* page_size specifies the number of items to return in the next page
*
*
* int64 page_size = 2;
*/
public long getPageSize() {
return pageSize_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getCursorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cursor_);
}
if (pageSize_ != 0L) {
output.writeInt64(2, pageSize_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getCursorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cursor_);
}
if (pageSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, pageSize_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions other = (com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions) obj;
boolean result = true;
result = result && getCursor()
.equals(other.getCursor());
result = result && (getPageSize()
== other.getPageSize());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CURSOR_FIELD_NUMBER;
hash = (53 * hash) + getCursor().hashCode();
hash = (37 * hash) + PAGE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPageSize());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* To provide options for the paged request
*
*
* Protobuf type {@code sales.v1.PagedRequestOptions}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.PagedRequestOptions)
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedRequestOptions_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedRequestOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.class, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
cursor_ = "";
pageSize_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_PagedRequestOptions_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions build() {
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions result = new com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions(this);
result.cursor_ = cursor_;
result.pageSize_ = pageSize_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.getDefaultInstance()) return this;
if (!other.getCursor().isEmpty()) {
cursor_ = other.cursor_;
onChanged();
}
if (other.getPageSize() != 0L) {
setPageSize(other.getPageSize());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object cursor_ = "";
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
public java.lang.String getCursor() {
java.lang.Object ref = cursor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cursor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
public com.google.protobuf.ByteString
getCursorBytes() {
java.lang.Object ref = cursor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
public Builder setCursor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cursor_ = value;
onChanged();
return this;
}
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
public Builder clearCursor() {
cursor_ = getDefaultInstance().getCursor();
onChanged();
return this;
}
/**
*
* cursor can be passed to retrieve the next page of results keyed by the cursor
*
*
* string cursor = 1;
*/
public Builder setCursorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cursor_ = value;
onChanged();
return this;
}
private long pageSize_ ;
/**
*
* page_size specifies the number of items to return in the next page
*
*
* int64 page_size = 2;
*/
public long getPageSize() {
return pageSize_;
}
/**
*
* page_size specifies the number of items to return in the next page
*
*
* int64 page_size = 2;
*/
public Builder setPageSize(long value) {
pageSize_ = value;
onChanged();
return this;
}
/**
*
* page_size specifies the number of items to return in the next page
*
*
* int64 page_size = 2;
*/
public Builder clearPageSize() {
pageSize_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.PagedRequestOptions)
}
// @@protoc_insertion_point(class_scope:sales.v1.PagedRequestOptions)
private static final com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions();
}
public static com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PagedRequestOptions parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PagedRequestOptions(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListSalesTeamsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.ListSalesTeamsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
boolean hasFilters();
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters getFilters();
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFiltersOrBuilder getFiltersOrBuilder();
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
boolean hasPagingOptions();
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getPagingOptions();
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder getPagingOptionsOrBuilder();
}
/**
* Protobuf type {@code sales.v1.ListSalesTeamsRequest}
*/
public static final class ListSalesTeamsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.ListSalesTeamsRequest)
ListSalesTeamsRequestOrBuilder {
// Use ListSalesTeamsRequest.newBuilder() to construct.
private ListSalesTeamsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListSalesTeamsRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ListSalesTeamsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.Builder subBuilder = null;
if (filters_ != null) {
subBuilder = filters_.toBuilder();
}
filters_ = input.readMessage(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(filters_);
filters_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder subBuilder = null;
if (pagingOptions_ != null) {
subBuilder = pagingOptions_.toBuilder();
}
pagingOptions_ = input.readMessage(com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagingOptions_);
pagingOptions_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.class, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.Builder.class);
}
public interface ListSalesTeamsFiltersOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters)
com.google.protobuf.MessageOrBuilder {
/**
* string partner_id = 1;
*/
java.lang.String getPartnerId();
/**
* string partner_id = 1;
*/
com.google.protobuf.ByteString
getPartnerIdBytes();
/**
* string market_id = 2;
*/
java.lang.String getMarketId();
/**
* string market_id = 2;
*/
com.google.protobuf.ByteString
getMarketIdBytes();
}
/**
* Protobuf type {@code sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters}
*/
public static final class ListSalesTeamsFilters extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters)
ListSalesTeamsFiltersOrBuilder {
// Use ListSalesTeamsFilters.newBuilder() to construct.
private ListSalesTeamsFilters(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListSalesTeamsFilters() {
partnerId_ = "";
marketId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ListSalesTeamsFilters(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
partnerId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
marketId_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.class, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.Builder.class);
}
public static final int PARTNER_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object partnerId_;
/**
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
}
}
/**
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MARKET_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object marketId_;
/**
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
}
}
/**
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, marketId_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, marketId_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters other = (com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters) obj;
boolean result = true;
result = result && getPartnerId()
.equals(other.getPartnerId());
result = result && getMarketId()
.equals(other.getMarketId());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartnerId().hashCode();
hash = (37 * hash) + MARKET_ID_FIELD_NUMBER;
hash = (53 * hash) + getMarketId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters)
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFiltersOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.class, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
partnerId_ = "";
marketId_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters build() {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters result = new com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters(this);
result.partnerId_ = partnerId_;
result.marketId_ = marketId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.getDefaultInstance()) return this;
if (!other.getPartnerId().isEmpty()) {
partnerId_ = other.partnerId_;
onChanged();
}
if (!other.getMarketId().isEmpty()) {
marketId_ = other.marketId_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object partnerId_ = "";
/**
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string partner_id = 1;
*/
public Builder setPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partnerId_ = value;
onChanged();
return this;
}
/**
* string partner_id = 1;
*/
public Builder clearPartnerId() {
partnerId_ = getDefaultInstance().getPartnerId();
onChanged();
return this;
}
/**
* string partner_id = 1;
*/
public Builder setPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partnerId_ = value;
onChanged();
return this;
}
private java.lang.Object marketId_ = "";
/**
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string market_id = 2;
*/
public Builder setMarketId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
marketId_ = value;
onChanged();
return this;
}
/**
* string market_id = 2;
*/
public Builder clearMarketId() {
marketId_ = getDefaultInstance().getMarketId();
onChanged();
return this;
}
/**
* string market_id = 2;
*/
public Builder setMarketIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
marketId_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters)
}
// @@protoc_insertion_point(class_scope:sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters)
private static final com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters();
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ListSalesTeamsFilters parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListSalesTeamsFilters(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int FILTERS_FIELD_NUMBER = 1;
private com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters filters_;
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public boolean hasFilters() {
return filters_ != null;
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters getFilters() {
return filters_ == null ? com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.getDefaultInstance() : filters_;
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFiltersOrBuilder getFiltersOrBuilder() {
return getFilters();
}
public static final int PAGING_OPTIONS_FIELD_NUMBER = 2;
private com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions pagingOptions_;
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public boolean hasPagingOptions() {
return pagingOptions_ != null;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getPagingOptions() {
return pagingOptions_ == null ? com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.getDefaultInstance() : pagingOptions_;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder getPagingOptionsOrBuilder() {
return getPagingOptions();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (filters_ != null) {
output.writeMessage(1, getFilters());
}
if (pagingOptions_ != null) {
output.writeMessage(2, getPagingOptions());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (filters_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFilters());
}
if (pagingOptions_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagingOptions());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest other = (com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest) obj;
boolean result = true;
result = result && (hasFilters() == other.hasFilters());
if (hasFilters()) {
result = result && getFilters()
.equals(other.getFilters());
}
result = result && (hasPagingOptions() == other.hasPagingOptions());
if (hasPagingOptions()) {
result = result && getPagingOptions()
.equals(other.getPagingOptions());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFilters()) {
hash = (37 * hash) + FILTERS_FIELD_NUMBER;
hash = (53 * hash) + getFilters().hashCode();
}
if (hasPagingOptions()) {
hash = (37 * hash) + PAGING_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getPagingOptions().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.ListSalesTeamsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.ListSalesTeamsRequest)
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.class, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (filtersBuilder_ == null) {
filters_ = null;
} else {
filters_ = null;
filtersBuilder_ = null;
}
if (pagingOptionsBuilder_ == null) {
pagingOptions_ = null;
} else {
pagingOptions_ = null;
pagingOptionsBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsRequest_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest build() {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest result = new com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest(this);
if (filtersBuilder_ == null) {
result.filters_ = filters_;
} else {
result.filters_ = filtersBuilder_.build();
}
if (pagingOptionsBuilder_ == null) {
result.pagingOptions_ = pagingOptions_;
} else {
result.pagingOptions_ = pagingOptionsBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.getDefaultInstance()) return this;
if (other.hasFilters()) {
mergeFilters(other.getFilters());
}
if (other.hasPagingOptions()) {
mergePagingOptions(other.getPagingOptions());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters filters_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.Builder, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFiltersOrBuilder> filtersBuilder_;
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public boolean hasFilters() {
return filtersBuilder_ != null || filters_ != null;
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters getFilters() {
if (filtersBuilder_ == null) {
return filters_ == null ? com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.getDefaultInstance() : filters_;
} else {
return filtersBuilder_.getMessage();
}
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public Builder setFilters(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters value) {
if (filtersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
filters_ = value;
onChanged();
} else {
filtersBuilder_.setMessage(value);
}
return this;
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public Builder setFilters(
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.Builder builderForValue) {
if (filtersBuilder_ == null) {
filters_ = builderForValue.build();
onChanged();
} else {
filtersBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public Builder mergeFilters(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters value) {
if (filtersBuilder_ == null) {
if (filters_ != null) {
filters_ =
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.newBuilder(filters_).mergeFrom(value).buildPartial();
} else {
filters_ = value;
}
onChanged();
} else {
filtersBuilder_.mergeFrom(value);
}
return this;
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public Builder clearFilters() {
if (filtersBuilder_ == null) {
filters_ = null;
onChanged();
} else {
filters_ = null;
filtersBuilder_ = null;
}
return this;
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.Builder getFiltersBuilder() {
onChanged();
return getFiltersFieldBuilder().getBuilder();
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFiltersOrBuilder getFiltersOrBuilder() {
if (filtersBuilder_ != null) {
return filtersBuilder_.getMessageOrBuilder();
} else {
return filters_ == null ?
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.getDefaultInstance() : filters_;
}
}
/**
* .sales.v1.ListSalesTeamsRequest.ListSalesTeamsFilters filters = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.Builder, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFiltersOrBuilder>
getFiltersFieldBuilder() {
if (filtersBuilder_ == null) {
filtersBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFilters.Builder, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.ListSalesTeamsFiltersOrBuilder>(
getFilters(),
getParentForChildren(),
isClean());
filters_ = null;
}
return filtersBuilder_;
}
private com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions pagingOptions_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder> pagingOptionsBuilder_;
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public boolean hasPagingOptions() {
return pagingOptionsBuilder_ != null || pagingOptions_ != null;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getPagingOptions() {
if (pagingOptionsBuilder_ == null) {
return pagingOptions_ == null ? com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.getDefaultInstance() : pagingOptions_;
} else {
return pagingOptionsBuilder_.getMessage();
}
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public Builder setPagingOptions(com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions value) {
if (pagingOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagingOptions_ = value;
onChanged();
} else {
pagingOptionsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public Builder setPagingOptions(
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder builderForValue) {
if (pagingOptionsBuilder_ == null) {
pagingOptions_ = builderForValue.build();
onChanged();
} else {
pagingOptionsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public Builder mergePagingOptions(com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions value) {
if (pagingOptionsBuilder_ == null) {
if (pagingOptions_ != null) {
pagingOptions_ =
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.newBuilder(pagingOptions_).mergeFrom(value).buildPartial();
} else {
pagingOptions_ = value;
}
onChanged();
} else {
pagingOptionsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public Builder clearPagingOptions() {
if (pagingOptionsBuilder_ == null) {
pagingOptions_ = null;
onChanged();
} else {
pagingOptions_ = null;
pagingOptionsBuilder_ = null;
}
return this;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder getPagingOptionsBuilder() {
onChanged();
return getPagingOptionsFieldBuilder().getBuilder();
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder getPagingOptionsOrBuilder() {
if (pagingOptionsBuilder_ != null) {
return pagingOptionsBuilder_.getMessageOrBuilder();
} else {
return pagingOptions_ == null ?
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.getDefaultInstance() : pagingOptions_;
}
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder>
getPagingOptionsFieldBuilder() {
if (pagingOptionsBuilder_ == null) {
pagingOptionsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder>(
getPagingOptions(),
getParentForChildren(),
isClean());
pagingOptions_ = null;
}
return pagingOptionsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.ListSalesTeamsRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.ListSalesTeamsRequest)
private static final com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest();
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ListSalesTeamsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListSalesTeamsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListSalesTeamsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.ListSalesTeamsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
java.util.List
getSalesTeamsList();
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam getSalesTeams(int index);
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
int getSalesTeamsCount();
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
java.util.List extends com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder>
getSalesTeamsOrBuilderList();
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder getSalesTeamsOrBuilder(
int index);
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
boolean hasPagingMetadata();
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata getPagingMetadata();
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadataOrBuilder getPagingMetadataOrBuilder();
}
/**
* Protobuf type {@code sales.v1.ListSalesTeamsResponse}
*/
public static final class ListSalesTeamsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.ListSalesTeamsResponse)
ListSalesTeamsResponseOrBuilder {
// Use ListSalesTeamsResponse.newBuilder() to construct.
private ListSalesTeamsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListSalesTeamsResponse() {
salesTeams_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ListSalesTeamsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
salesTeams_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
salesTeams_.add(
input.readMessage(com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.parser(), extensionRegistry));
break;
}
case 18: {
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.Builder subBuilder = null;
if (pagingMetadata_ != null) {
subBuilder = pagingMetadata_.toBuilder();
}
pagingMetadata_ = input.readMessage(com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagingMetadata_);
pagingMetadata_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
salesTeams_ = java.util.Collections.unmodifiableList(salesTeams_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse.class, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse.Builder.class);
}
private int bitField0_;
public static final int SALES_TEAMS_FIELD_NUMBER = 1;
private java.util.List salesTeams_;
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public java.util.List getSalesTeamsList() {
return salesTeams_;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder>
getSalesTeamsOrBuilderList() {
return salesTeams_;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public int getSalesTeamsCount() {
return salesTeams_.size();
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam getSalesTeams(int index) {
return salesTeams_.get(index);
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder getSalesTeamsOrBuilder(
int index) {
return salesTeams_.get(index);
}
public static final int PAGING_METADATA_FIELD_NUMBER = 2;
private com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata pagingMetadata_;
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public boolean hasPagingMetadata() {
return pagingMetadata_ != null;
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata getPagingMetadata() {
return pagingMetadata_ == null ? com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadataOrBuilder getPagingMetadataOrBuilder() {
return getPagingMetadata();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < salesTeams_.size(); i++) {
output.writeMessage(1, salesTeams_.get(i));
}
if (pagingMetadata_ != null) {
output.writeMessage(2, getPagingMetadata());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < salesTeams_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, salesTeams_.get(i));
}
if (pagingMetadata_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagingMetadata());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse other = (com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse) obj;
boolean result = true;
result = result && getSalesTeamsList()
.equals(other.getSalesTeamsList());
result = result && (hasPagingMetadata() == other.hasPagingMetadata());
if (hasPagingMetadata()) {
result = result && getPagingMetadata()
.equals(other.getPagingMetadata());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSalesTeamsCount() > 0) {
hash = (37 * hash) + SALES_TEAMS_FIELD_NUMBER;
hash = (53 * hash) + getSalesTeamsList().hashCode();
}
if (hasPagingMetadata()) {
hash = (37 * hash) + PAGING_METADATA_FIELD_NUMBER;
hash = (53 * hash) + getPagingMetadata().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.ListSalesTeamsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.ListSalesTeamsResponse)
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse.class, com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getSalesTeamsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (salesTeamsBuilder_ == null) {
salesTeams_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
salesTeamsBuilder_.clear();
}
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = null;
} else {
pagingMetadata_ = null;
pagingMetadataBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_ListSalesTeamsResponse_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse build() {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse result = new com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (salesTeamsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
salesTeams_ = java.util.Collections.unmodifiableList(salesTeams_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.salesTeams_ = salesTeams_;
} else {
result.salesTeams_ = salesTeamsBuilder_.build();
}
if (pagingMetadataBuilder_ == null) {
result.pagingMetadata_ = pagingMetadata_;
} else {
result.pagingMetadata_ = pagingMetadataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse.getDefaultInstance()) return this;
if (salesTeamsBuilder_ == null) {
if (!other.salesTeams_.isEmpty()) {
if (salesTeams_.isEmpty()) {
salesTeams_ = other.salesTeams_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSalesTeamsIsMutable();
salesTeams_.addAll(other.salesTeams_);
}
onChanged();
}
} else {
if (!other.salesTeams_.isEmpty()) {
if (salesTeamsBuilder_.isEmpty()) {
salesTeamsBuilder_.dispose();
salesTeamsBuilder_ = null;
salesTeams_ = other.salesTeams_;
bitField0_ = (bitField0_ & ~0x00000001);
salesTeamsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSalesTeamsFieldBuilder() : null;
} else {
salesTeamsBuilder_.addAllMessages(other.salesTeams_);
}
}
}
if (other.hasPagingMetadata()) {
mergePagingMetadata(other.getPagingMetadata());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List salesTeams_ =
java.util.Collections.emptyList();
private void ensureSalesTeamsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
salesTeams_ = new java.util.ArrayList(salesTeams_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder> salesTeamsBuilder_;
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public java.util.List getSalesTeamsList() {
if (salesTeamsBuilder_ == null) {
return java.util.Collections.unmodifiableList(salesTeams_);
} else {
return salesTeamsBuilder_.getMessageList();
}
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public int getSalesTeamsCount() {
if (salesTeamsBuilder_ == null) {
return salesTeams_.size();
} else {
return salesTeamsBuilder_.getCount();
}
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam getSalesTeams(int index) {
if (salesTeamsBuilder_ == null) {
return salesTeams_.get(index);
} else {
return salesTeamsBuilder_.getMessage(index);
}
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder setSalesTeams(
int index, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam value) {
if (salesTeamsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSalesTeamsIsMutable();
salesTeams_.set(index, value);
onChanged();
} else {
salesTeamsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder setSalesTeams(
int index, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder builderForValue) {
if (salesTeamsBuilder_ == null) {
ensureSalesTeamsIsMutable();
salesTeams_.set(index, builderForValue.build());
onChanged();
} else {
salesTeamsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder addSalesTeams(com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam value) {
if (salesTeamsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSalesTeamsIsMutable();
salesTeams_.add(value);
onChanged();
} else {
salesTeamsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder addSalesTeams(
int index, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam value) {
if (salesTeamsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSalesTeamsIsMutable();
salesTeams_.add(index, value);
onChanged();
} else {
salesTeamsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder addSalesTeams(
com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder builderForValue) {
if (salesTeamsBuilder_ == null) {
ensureSalesTeamsIsMutable();
salesTeams_.add(builderForValue.build());
onChanged();
} else {
salesTeamsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder addSalesTeams(
int index, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder builderForValue) {
if (salesTeamsBuilder_ == null) {
ensureSalesTeamsIsMutable();
salesTeams_.add(index, builderForValue.build());
onChanged();
} else {
salesTeamsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder addAllSalesTeams(
java.lang.Iterable extends com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam> values) {
if (salesTeamsBuilder_ == null) {
ensureSalesTeamsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, salesTeams_);
onChanged();
} else {
salesTeamsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder clearSalesTeams() {
if (salesTeamsBuilder_ == null) {
salesTeams_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
salesTeamsBuilder_.clear();
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public Builder removeSalesTeams(int index) {
if (salesTeamsBuilder_ == null) {
ensureSalesTeamsIsMutable();
salesTeams_.remove(index);
onChanged();
} else {
salesTeamsBuilder_.remove(index);
}
return this;
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder getSalesTeamsBuilder(
int index) {
return getSalesTeamsFieldBuilder().getBuilder(index);
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder getSalesTeamsOrBuilder(
int index) {
if (salesTeamsBuilder_ == null) {
return salesTeams_.get(index); } else {
return salesTeamsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder>
getSalesTeamsOrBuilderList() {
if (salesTeamsBuilder_ != null) {
return salesTeamsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(salesTeams_);
}
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder addSalesTeamsBuilder() {
return getSalesTeamsFieldBuilder().addBuilder(
com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.getDefaultInstance());
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder addSalesTeamsBuilder(
int index) {
return getSalesTeamsFieldBuilder().addBuilder(
index, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.getDefaultInstance());
}
/**
* repeated .sales.v1.SalesTeam sales_teams = 1;
*/
public java.util.List
getSalesTeamsBuilderList() {
return getSalesTeamsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder>
getSalesTeamsFieldBuilder() {
if (salesTeamsBuilder_ == null) {
salesTeamsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeam.Builder, com.vendasta.sales.v1.generated.SalesTeamProto.SalesTeamOrBuilder>(
salesTeams_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
salesTeams_ = null;
}
return salesTeamsBuilder_;
}
private com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata pagingMetadata_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata, com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadataOrBuilder> pagingMetadataBuilder_;
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public boolean hasPagingMetadata() {
return pagingMetadataBuilder_ != null || pagingMetadata_ != null;
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata getPagingMetadata() {
if (pagingMetadataBuilder_ == null) {
return pagingMetadata_ == null ? com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
} else {
return pagingMetadataBuilder_.getMessage();
}
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public Builder setPagingMetadata(com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata value) {
if (pagingMetadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagingMetadata_ = value;
onChanged();
} else {
pagingMetadataBuilder_.setMessage(value);
}
return this;
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public Builder setPagingMetadata(
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.Builder builderForValue) {
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = builderForValue.build();
onChanged();
} else {
pagingMetadataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public Builder mergePagingMetadata(com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata value) {
if (pagingMetadataBuilder_ == null) {
if (pagingMetadata_ != null) {
pagingMetadata_ =
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.newBuilder(pagingMetadata_).mergeFrom(value).buildPartial();
} else {
pagingMetadata_ = value;
}
onChanged();
} else {
pagingMetadataBuilder_.mergeFrom(value);
}
return this;
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public Builder clearPagingMetadata() {
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = null;
onChanged();
} else {
pagingMetadata_ = null;
pagingMetadataBuilder_ = null;
}
return this;
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.Builder getPagingMetadataBuilder() {
onChanged();
return getPagingMetadataFieldBuilder().getBuilder();
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadataOrBuilder getPagingMetadataOrBuilder() {
if (pagingMetadataBuilder_ != null) {
return pagingMetadataBuilder_.getMessageOrBuilder();
} else {
return pagingMetadata_ == null ?
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
}
}
/**
* .sales.v1.PagedResponseMetadata paging_metadata = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata, com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadataOrBuilder>
getPagingMetadataFieldBuilder() {
if (pagingMetadataBuilder_ == null) {
pagingMetadataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata, com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedResponseMetadataOrBuilder>(
getPagingMetadata(),
getParentForChildren(),
isClean());
pagingMetadata_ = null;
}
return pagingMetadataBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.ListSalesTeamsResponse)
}
// @@protoc_insertion_point(class_scope:sales.v1.ListSalesTeamsResponse)
private static final com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse();
}
public static com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ListSalesTeamsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListSalesTeamsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BusinessSearchRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.BusinessSearchRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
boolean hasFilters();
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters getFilters();
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFiltersOrBuilder getFiltersOrBuilder();
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
boolean hasPagingOptions();
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getPagingOptions();
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder getPagingOptionsOrBuilder();
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
boolean hasSortOptions();
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions getSortOptions();
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptionsOrBuilder getSortOptionsOrBuilder();
}
/**
* Protobuf type {@code sales.v1.BusinessSearchRequest}
*/
public static final class BusinessSearchRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.BusinessSearchRequest)
BusinessSearchRequestOrBuilder {
// Use BusinessSearchRequest.newBuilder() to construct.
private BusinessSearchRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BusinessSearchRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private BusinessSearchRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.Builder subBuilder = null;
if (filters_ != null) {
subBuilder = filters_.toBuilder();
}
filters_ = input.readMessage(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(filters_);
filters_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder subBuilder = null;
if (pagingOptions_ != null) {
subBuilder = pagingOptions_.toBuilder();
}
pagingOptions_ = input.readMessage(com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagingOptions_);
pagingOptions_ = subBuilder.buildPartial();
}
break;
}
case 26: {
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.Builder subBuilder = null;
if (sortOptions_ != null) {
subBuilder = sortOptions_.toBuilder();
}
sortOptions_ = input.readMessage(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(sortOptions_);
sortOptions_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest.class, com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest.Builder.class);
}
public static final int FILTERS_FIELD_NUMBER = 1;
private com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters filters_;
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public boolean hasFilters() {
return filters_ != null;
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters getFilters() {
return filters_ == null ? com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.getDefaultInstance() : filters_;
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFiltersOrBuilder getFiltersOrBuilder() {
return getFilters();
}
public static final int PAGING_OPTIONS_FIELD_NUMBER = 2;
private com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions pagingOptions_;
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public boolean hasPagingOptions() {
return pagingOptions_ != null;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getPagingOptions() {
return pagingOptions_ == null ? com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.getDefaultInstance() : pagingOptions_;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder getPagingOptionsOrBuilder() {
return getPagingOptions();
}
public static final int SORT_OPTIONS_FIELD_NUMBER = 3;
private com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions sortOptions_;
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public boolean hasSortOptions() {
return sortOptions_ != null;
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions getSortOptions() {
return sortOptions_ == null ? com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.getDefaultInstance() : sortOptions_;
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptionsOrBuilder getSortOptionsOrBuilder() {
return getSortOptions();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (filters_ != null) {
output.writeMessage(1, getFilters());
}
if (pagingOptions_ != null) {
output.writeMessage(2, getPagingOptions());
}
if (sortOptions_ != null) {
output.writeMessage(3, getSortOptions());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (filters_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFilters());
}
if (pagingOptions_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagingOptions());
}
if (sortOptions_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getSortOptions());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest other = (com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest) obj;
boolean result = true;
result = result && (hasFilters() == other.hasFilters());
if (hasFilters()) {
result = result && getFilters()
.equals(other.getFilters());
}
result = result && (hasPagingOptions() == other.hasPagingOptions());
if (hasPagingOptions()) {
result = result && getPagingOptions()
.equals(other.getPagingOptions());
}
result = result && (hasSortOptions() == other.hasSortOptions());
if (hasSortOptions()) {
result = result && getSortOptions()
.equals(other.getSortOptions());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFilters()) {
hash = (37 * hash) + FILTERS_FIELD_NUMBER;
hash = (53 * hash) + getFilters().hashCode();
}
if (hasPagingOptions()) {
hash = (37 * hash) + PAGING_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getPagingOptions().hashCode();
}
if (hasSortOptions()) {
hash = (37 * hash) + SORT_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getSortOptions().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.BusinessSearchRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.BusinessSearchRequest)
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest.class, com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (filtersBuilder_ == null) {
filters_ = null;
} else {
filters_ = null;
filtersBuilder_ = null;
}
if (pagingOptionsBuilder_ == null) {
pagingOptions_ = null;
} else {
pagingOptions_ = null;
pagingOptionsBuilder_ = null;
}
if (sortOptionsBuilder_ == null) {
sortOptions_ = null;
} else {
sortOptions_ = null;
sortOptionsBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchRequest_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest build() {
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest result = new com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest(this);
if (filtersBuilder_ == null) {
result.filters_ = filters_;
} else {
result.filters_ = filtersBuilder_.build();
}
if (pagingOptionsBuilder_ == null) {
result.pagingOptions_ = pagingOptions_;
} else {
result.pagingOptions_ = pagingOptionsBuilder_.build();
}
if (sortOptionsBuilder_ == null) {
result.sortOptions_ = sortOptions_;
} else {
result.sortOptions_ = sortOptionsBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest.getDefaultInstance()) return this;
if (other.hasFilters()) {
mergeFilters(other.getFilters());
}
if (other.hasPagingOptions()) {
mergePagingOptions(other.getPagingOptions());
}
if (other.hasSortOptions()) {
mergeSortOptions(other.getSortOptions());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters filters_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFiltersOrBuilder> filtersBuilder_;
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public boolean hasFilters() {
return filtersBuilder_ != null || filters_ != null;
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters getFilters() {
if (filtersBuilder_ == null) {
return filters_ == null ? com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.getDefaultInstance() : filters_;
} else {
return filtersBuilder_.getMessage();
}
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public Builder setFilters(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters value) {
if (filtersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
filters_ = value;
onChanged();
} else {
filtersBuilder_.setMessage(value);
}
return this;
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public Builder setFilters(
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.Builder builderForValue) {
if (filtersBuilder_ == null) {
filters_ = builderForValue.build();
onChanged();
} else {
filtersBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public Builder mergeFilters(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters value) {
if (filtersBuilder_ == null) {
if (filters_ != null) {
filters_ =
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.newBuilder(filters_).mergeFrom(value).buildPartial();
} else {
filters_ = value;
}
onChanged();
} else {
filtersBuilder_.mergeFrom(value);
}
return this;
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public Builder clearFilters() {
if (filtersBuilder_ == null) {
filters_ = null;
onChanged();
} else {
filters_ = null;
filtersBuilder_ = null;
}
return this;
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.Builder getFiltersBuilder() {
onChanged();
return getFiltersFieldBuilder().getBuilder();
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFiltersOrBuilder getFiltersOrBuilder() {
if (filtersBuilder_ != null) {
return filtersBuilder_.getMessageOrBuilder();
} else {
return filters_ == null ?
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.getDefaultInstance() : filters_;
}
}
/**
* .sales.v1.BusinessSearchFilters filters = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFiltersOrBuilder>
getFiltersFieldBuilder() {
if (filtersBuilder_ == null) {
filtersBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFiltersOrBuilder>(
getFilters(),
getParentForChildren(),
isClean());
filters_ = null;
}
return filtersBuilder_;
}
private com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions pagingOptions_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder> pagingOptionsBuilder_;
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public boolean hasPagingOptions() {
return pagingOptionsBuilder_ != null || pagingOptions_ != null;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions getPagingOptions() {
if (pagingOptionsBuilder_ == null) {
return pagingOptions_ == null ? com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.getDefaultInstance() : pagingOptions_;
} else {
return pagingOptionsBuilder_.getMessage();
}
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public Builder setPagingOptions(com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions value) {
if (pagingOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagingOptions_ = value;
onChanged();
} else {
pagingOptionsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public Builder setPagingOptions(
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder builderForValue) {
if (pagingOptionsBuilder_ == null) {
pagingOptions_ = builderForValue.build();
onChanged();
} else {
pagingOptionsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public Builder mergePagingOptions(com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions value) {
if (pagingOptionsBuilder_ == null) {
if (pagingOptions_ != null) {
pagingOptions_ =
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.newBuilder(pagingOptions_).mergeFrom(value).buildPartial();
} else {
pagingOptions_ = value;
}
onChanged();
} else {
pagingOptionsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public Builder clearPagingOptions() {
if (pagingOptionsBuilder_ == null) {
pagingOptions_ = null;
onChanged();
} else {
pagingOptions_ = null;
pagingOptionsBuilder_ = null;
}
return this;
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder getPagingOptionsBuilder() {
onChanged();
return getPagingOptionsFieldBuilder().getBuilder();
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
public com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder getPagingOptionsOrBuilder() {
if (pagingOptionsBuilder_ != null) {
return pagingOptionsBuilder_.getMessageOrBuilder();
} else {
return pagingOptions_ == null ?
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.getDefaultInstance() : pagingOptions_;
}
}
/**
*
* Options for how to page the response for this request
*
*
* .sales.v1.PagedRequestOptions paging_options = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder>
getPagingOptionsFieldBuilder() {
if (pagingOptionsBuilder_ == null) {
pagingOptionsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptions.Builder, com.vendasta.sales.v1.generated.ApiProto.PagedRequestOptionsOrBuilder>(
getPagingOptions(),
getParentForChildren(),
isClean());
pagingOptions_ = null;
}
return pagingOptionsBuilder_;
}
private com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions sortOptions_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptionsOrBuilder> sortOptionsBuilder_;
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public boolean hasSortOptions() {
return sortOptionsBuilder_ != null || sortOptions_ != null;
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions getSortOptions() {
if (sortOptionsBuilder_ == null) {
return sortOptions_ == null ? com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.getDefaultInstance() : sortOptions_;
} else {
return sortOptionsBuilder_.getMessage();
}
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public Builder setSortOptions(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions value) {
if (sortOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sortOptions_ = value;
onChanged();
} else {
sortOptionsBuilder_.setMessage(value);
}
return this;
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public Builder setSortOptions(
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.Builder builderForValue) {
if (sortOptionsBuilder_ == null) {
sortOptions_ = builderForValue.build();
onChanged();
} else {
sortOptionsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public Builder mergeSortOptions(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions value) {
if (sortOptionsBuilder_ == null) {
if (sortOptions_ != null) {
sortOptions_ =
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.newBuilder(sortOptions_).mergeFrom(value).buildPartial();
} else {
sortOptions_ = value;
}
onChanged();
} else {
sortOptionsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public Builder clearSortOptions() {
if (sortOptionsBuilder_ == null) {
sortOptions_ = null;
onChanged();
} else {
sortOptions_ = null;
sortOptionsBuilder_ = null;
}
return this;
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.Builder getSortOptionsBuilder() {
onChanged();
return getSortOptionsFieldBuilder().getBuilder();
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptionsOrBuilder getSortOptionsOrBuilder() {
if (sortOptionsBuilder_ != null) {
return sortOptionsBuilder_.getMessageOrBuilder();
} else {
return sortOptions_ == null ?
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.getDefaultInstance() : sortOptions_;
}
}
/**
* .sales.v1.BusinessSearchSortOptions sort_options = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptionsOrBuilder>
getSortOptionsFieldBuilder() {
if (sortOptionsBuilder_ == null) {
sortOptionsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptionsOrBuilder>(
getSortOptions(),
getParentForChildren(),
isClean());
sortOptions_ = null;
}
return sortOptionsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.BusinessSearchRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.BusinessSearchRequest)
private static final com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest();
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public BusinessSearchRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BusinessSearchRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BusinessSearchResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.BusinessSearchResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .sales.v1.Business businesses = 1;
*/
java.util.List
getBusinessesList();
/**
* repeated .sales.v1.Business businesses = 1;
*/
com.vendasta.sales.v1.generated.BusinessProto.Business getBusinesses(int index);
/**
* repeated .sales.v1.Business businesses = 1;
*/
int getBusinessesCount();
/**
* repeated .sales.v1.Business businesses = 1;
*/
java.util.List extends com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder>
getBusinessesOrBuilderList();
/**
* repeated .sales.v1.Business businesses = 1;
*/
com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder getBusinessesOrBuilder(
int index);
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
boolean hasPagingMetadata();
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata getPagingMetadata();
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadataOrBuilder getPagingMetadataOrBuilder();
}
/**
* Protobuf type {@code sales.v1.BusinessSearchResponse}
*/
public static final class BusinessSearchResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.BusinessSearchResponse)
BusinessSearchResponseOrBuilder {
// Use BusinessSearchResponse.newBuilder() to construct.
private BusinessSearchResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BusinessSearchResponse() {
businesses_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private BusinessSearchResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
businesses_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
businesses_.add(
input.readMessage(com.vendasta.sales.v1.generated.BusinessProto.Business.parser(), extensionRegistry));
break;
}
case 18: {
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.Builder subBuilder = null;
if (pagingMetadata_ != null) {
subBuilder = pagingMetadata_.toBuilder();
}
pagingMetadata_ = input.readMessage(com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagingMetadata_);
pagingMetadata_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
businesses_ = java.util.Collections.unmodifiableList(businesses_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse.class, com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse.Builder.class);
}
private int bitField0_;
public static final int BUSINESSES_FIELD_NUMBER = 1;
private java.util.List businesses_;
/**
* repeated .sales.v1.Business businesses = 1;
*/
public java.util.List getBusinessesList() {
return businesses_;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder>
getBusinessesOrBuilderList() {
return businesses_;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public int getBusinessesCount() {
return businesses_.size();
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Business getBusinesses(int index) {
return businesses_.get(index);
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder getBusinessesOrBuilder(
int index) {
return businesses_.get(index);
}
public static final int PAGING_METADATA_FIELD_NUMBER = 2;
private com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata pagingMetadata_;
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public boolean hasPagingMetadata() {
return pagingMetadata_ != null;
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata getPagingMetadata() {
return pagingMetadata_ == null ? com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadataOrBuilder getPagingMetadataOrBuilder() {
return getPagingMetadata();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < businesses_.size(); i++) {
output.writeMessage(1, businesses_.get(i));
}
if (pagingMetadata_ != null) {
output.writeMessage(2, getPagingMetadata());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < businesses_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, businesses_.get(i));
}
if (pagingMetadata_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagingMetadata());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse other = (com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse) obj;
boolean result = true;
result = result && getBusinessesList()
.equals(other.getBusinessesList());
result = result && (hasPagingMetadata() == other.hasPagingMetadata());
if (hasPagingMetadata()) {
result = result && getPagingMetadata()
.equals(other.getPagingMetadata());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getBusinessesCount() > 0) {
hash = (37 * hash) + BUSINESSES_FIELD_NUMBER;
hash = (53 * hash) + getBusinessesList().hashCode();
}
if (hasPagingMetadata()) {
hash = (37 * hash) + PAGING_METADATA_FIELD_NUMBER;
hash = (53 * hash) + getPagingMetadata().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.BusinessSearchResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.BusinessSearchResponse)
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse.class, com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getBusinessesFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (businessesBuilder_ == null) {
businesses_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
businessesBuilder_.clear();
}
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = null;
} else {
pagingMetadata_ = null;
pagingMetadataBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_BusinessSearchResponse_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse build() {
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse result = new com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (businessesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
businesses_ = java.util.Collections.unmodifiableList(businesses_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.businesses_ = businesses_;
} else {
result.businesses_ = businessesBuilder_.build();
}
if (pagingMetadataBuilder_ == null) {
result.pagingMetadata_ = pagingMetadata_;
} else {
result.pagingMetadata_ = pagingMetadataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse.getDefaultInstance()) return this;
if (businessesBuilder_ == null) {
if (!other.businesses_.isEmpty()) {
if (businesses_.isEmpty()) {
businesses_ = other.businesses_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureBusinessesIsMutable();
businesses_.addAll(other.businesses_);
}
onChanged();
}
} else {
if (!other.businesses_.isEmpty()) {
if (businessesBuilder_.isEmpty()) {
businessesBuilder_.dispose();
businessesBuilder_ = null;
businesses_ = other.businesses_;
bitField0_ = (bitField0_ & ~0x00000001);
businessesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getBusinessesFieldBuilder() : null;
} else {
businessesBuilder_.addAllMessages(other.businesses_);
}
}
}
if (other.hasPagingMetadata()) {
mergePagingMetadata(other.getPagingMetadata());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List businesses_ =
java.util.Collections.emptyList();
private void ensureBusinessesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
businesses_ = new java.util.ArrayList(businesses_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.Business, com.vendasta.sales.v1.generated.BusinessProto.Business.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder> businessesBuilder_;
/**
* repeated .sales.v1.Business businesses = 1;
*/
public java.util.List getBusinessesList() {
if (businessesBuilder_ == null) {
return java.util.Collections.unmodifiableList(businesses_);
} else {
return businessesBuilder_.getMessageList();
}
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public int getBusinessesCount() {
if (businessesBuilder_ == null) {
return businesses_.size();
} else {
return businessesBuilder_.getCount();
}
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Business getBusinesses(int index) {
if (businessesBuilder_ == null) {
return businesses_.get(index);
} else {
return businessesBuilder_.getMessage(index);
}
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder setBusinesses(
int index, com.vendasta.sales.v1.generated.BusinessProto.Business value) {
if (businessesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBusinessesIsMutable();
businesses_.set(index, value);
onChanged();
} else {
businessesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder setBusinesses(
int index, com.vendasta.sales.v1.generated.BusinessProto.Business.Builder builderForValue) {
if (businessesBuilder_ == null) {
ensureBusinessesIsMutable();
businesses_.set(index, builderForValue.build());
onChanged();
} else {
businessesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder addBusinesses(com.vendasta.sales.v1.generated.BusinessProto.Business value) {
if (businessesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBusinessesIsMutable();
businesses_.add(value);
onChanged();
} else {
businessesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder addBusinesses(
int index, com.vendasta.sales.v1.generated.BusinessProto.Business value) {
if (businessesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBusinessesIsMutable();
businesses_.add(index, value);
onChanged();
} else {
businessesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder addBusinesses(
com.vendasta.sales.v1.generated.BusinessProto.Business.Builder builderForValue) {
if (businessesBuilder_ == null) {
ensureBusinessesIsMutable();
businesses_.add(builderForValue.build());
onChanged();
} else {
businessesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder addBusinesses(
int index, com.vendasta.sales.v1.generated.BusinessProto.Business.Builder builderForValue) {
if (businessesBuilder_ == null) {
ensureBusinessesIsMutable();
businesses_.add(index, builderForValue.build());
onChanged();
} else {
businessesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder addAllBusinesses(
java.lang.Iterable extends com.vendasta.sales.v1.generated.BusinessProto.Business> values) {
if (businessesBuilder_ == null) {
ensureBusinessesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, businesses_);
onChanged();
} else {
businessesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder clearBusinesses() {
if (businessesBuilder_ == null) {
businesses_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
businessesBuilder_.clear();
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public Builder removeBusinesses(int index) {
if (businessesBuilder_ == null) {
ensureBusinessesIsMutable();
businesses_.remove(index);
onChanged();
} else {
businessesBuilder_.remove(index);
}
return this;
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Business.Builder getBusinessesBuilder(
int index) {
return getBusinessesFieldBuilder().getBuilder(index);
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder getBusinessesOrBuilder(
int index) {
if (businessesBuilder_ == null) {
return businesses_.get(index); } else {
return businessesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder>
getBusinessesOrBuilderList() {
if (businessesBuilder_ != null) {
return businessesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(businesses_);
}
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Business.Builder addBusinessesBuilder() {
return getBusinessesFieldBuilder().addBuilder(
com.vendasta.sales.v1.generated.BusinessProto.Business.getDefaultInstance());
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Business.Builder addBusinessesBuilder(
int index) {
return getBusinessesFieldBuilder().addBuilder(
index, com.vendasta.sales.v1.generated.BusinessProto.Business.getDefaultInstance());
}
/**
* repeated .sales.v1.Business businesses = 1;
*/
public java.util.List
getBusinessesBuilderList() {
return getBusinessesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.Business, com.vendasta.sales.v1.generated.BusinessProto.Business.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder>
getBusinessesFieldBuilder() {
if (businessesBuilder_ == null) {
businessesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.Business, com.vendasta.sales.v1.generated.BusinessProto.Business.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder>(
businesses_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
businesses_ = null;
}
return businessesBuilder_;
}
private com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata pagingMetadata_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata, com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadataOrBuilder> pagingMetadataBuilder_;
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public boolean hasPagingMetadata() {
return pagingMetadataBuilder_ != null || pagingMetadata_ != null;
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata getPagingMetadata() {
if (pagingMetadataBuilder_ == null) {
return pagingMetadata_ == null ? com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
} else {
return pagingMetadataBuilder_.getMessage();
}
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public Builder setPagingMetadata(com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata value) {
if (pagingMetadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagingMetadata_ = value;
onChanged();
} else {
pagingMetadataBuilder_.setMessage(value);
}
return this;
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public Builder setPagingMetadata(
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.Builder builderForValue) {
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = builderForValue.build();
onChanged();
} else {
pagingMetadataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public Builder mergePagingMetadata(com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata value) {
if (pagingMetadataBuilder_ == null) {
if (pagingMetadata_ != null) {
pagingMetadata_ =
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.newBuilder(pagingMetadata_).mergeFrom(value).buildPartial();
} else {
pagingMetadata_ = value;
}
onChanged();
} else {
pagingMetadataBuilder_.mergeFrom(value);
}
return this;
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public Builder clearPagingMetadata() {
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = null;
onChanged();
} else {
pagingMetadata_ = null;
pagingMetadataBuilder_ = null;
}
return this;
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.Builder getPagingMetadataBuilder() {
onChanged();
return getPagingMetadataFieldBuilder().getBuilder();
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadataOrBuilder getPagingMetadataOrBuilder() {
if (pagingMetadataBuilder_ != null) {
return pagingMetadataBuilder_.getMessageOrBuilder();
} else {
return pagingMetadata_ == null ?
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
}
}
/**
* .sales.v1.BusinessPagedResponseMetadata paging_metadata = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata, com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadataOrBuilder>
getPagingMetadataFieldBuilder() {
if (pagingMetadataBuilder_ == null) {
pagingMetadataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata, com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadataOrBuilder>(
getPagingMetadata(),
getParentForChildren(),
isClean());
pagingMetadata_ = null;
}
return pagingMetadataBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.BusinessSearchResponse)
}
// @@protoc_insertion_point(class_scope:sales.v1.BusinessSearchResponse)
private static final com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse();
}
public static com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public BusinessSearchResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BusinessSearchResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RequestSubscriptionChangeRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.RequestSubscriptionChangeRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
java.lang.String getBusinessId();
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
com.google.protobuf.ByteString
getBusinessIdBytes();
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
java.lang.String getMessage();
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
java.lang.String getPhoneNumber();
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
com.google.protobuf.ByteString
getPhoneNumberBytes();
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
java.lang.String getName();
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code sales.v1.RequestSubscriptionChangeRequest}
*/
public static final class RequestSubscriptionChangeRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.RequestSubscriptionChangeRequest)
RequestSubscriptionChangeRequestOrBuilder {
// Use RequestSubscriptionChangeRequest.newBuilder() to construct.
private RequestSubscriptionChangeRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RequestSubscriptionChangeRequest() {
businessId_ = "";
message_ = "";
phoneNumber_ = "";
name_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private RequestSubscriptionChangeRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
businessId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
phoneNumber_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_RequestSubscriptionChangeRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_RequestSubscriptionChangeRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest.class, com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest.Builder.class);
}
public static final int BUSINESS_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object businessId_;
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
public java.lang.String getBusinessId() {
java.lang.Object ref = businessId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
businessId_ = s;
return s;
}
}
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
public com.google.protobuf.ByteString
getBusinessIdBytes() {
java.lang.Object ref = businessId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
businessId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MESSAGE_FIELD_NUMBER = 2;
private volatile java.lang.Object message_;
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PHONE_NUMBER_FIELD_NUMBER = 3;
private volatile java.lang.Object phoneNumber_;
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
public java.lang.String getPhoneNumber() {
java.lang.Object ref = phoneNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
phoneNumber_ = s;
return s;
}
}
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
public com.google.protobuf.ByteString
getPhoneNumberBytes() {
java.lang.Object ref = phoneNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
phoneNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object name_;
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getBusinessIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, businessId_);
}
if (!getMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, message_);
}
if (!getPhoneNumberBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, phoneNumber_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, name_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getBusinessIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, businessId_);
}
if (!getMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, message_);
}
if (!getPhoneNumberBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, phoneNumber_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, name_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest other = (com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest) obj;
boolean result = true;
result = result && getBusinessId()
.equals(other.getBusinessId());
result = result && getMessage()
.equals(other.getMessage());
result = result && getPhoneNumber()
.equals(other.getPhoneNumber());
result = result && getName()
.equals(other.getName());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + BUSINESS_ID_FIELD_NUMBER;
hash = (53 * hash) + getBusinessId().hashCode();
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (37 * hash) + PHONE_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getPhoneNumber().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.RequestSubscriptionChangeRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.RequestSubscriptionChangeRequest)
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_RequestSubscriptionChangeRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_RequestSubscriptionChangeRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest.class, com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
businessId_ = "";
message_ = "";
phoneNumber_ = "";
name_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.ApiProto.internal_static_sales_v1_RequestSubscriptionChangeRequest_descriptor;
}
public com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest build() {
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest buildPartial() {
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest result = new com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest(this);
result.businessId_ = businessId_;
result.message_ = message_;
result.phoneNumber_ = phoneNumber_;
result.name_ = name_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest other) {
if (other == com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest.getDefaultInstance()) return this;
if (!other.getBusinessId().isEmpty()) {
businessId_ = other.businessId_;
onChanged();
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
if (!other.getPhoneNumber().isEmpty()) {
phoneNumber_ = other.phoneNumber_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object businessId_ = "";
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
public java.lang.String getBusinessId() {
java.lang.Object ref = businessId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
businessId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
public com.google.protobuf.ByteString
getBusinessIdBytes() {
java.lang.Object ref = businessId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
businessId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
public Builder setBusinessId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
businessId_ = value;
onChanged();
return this;
}
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
public Builder clearBusinessId() {
businessId_ = getDefaultInstance().getBusinessId();
onChanged();
return this;
}
/**
*
* The id of the business that is making the request to change subscription
*
*
* string business_id = 1;
*/
public Builder setBusinessIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
businessId_ = value;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
*
* The message from the business to be sent, requesting the change
*
*
* string message = 2;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
private java.lang.Object phoneNumber_ = "";
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
public java.lang.String getPhoneNumber() {
java.lang.Object ref = phoneNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
phoneNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
public com.google.protobuf.ByteString
getPhoneNumberBytes() {
java.lang.Object ref = phoneNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
phoneNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
public Builder setPhoneNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
phoneNumber_ = value;
onChanged();
return this;
}
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
public Builder clearPhoneNumber() {
phoneNumber_ = getDefaultInstance().getPhoneNumber();
onChanged();
return this;
}
/**
*
* The phone number to be contacted about the subscription change
*
*
* string phone_number = 3;
*/
public Builder setPhoneNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
phoneNumber_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* The person to contact about the subscription change
*
*
* string name = 4;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.RequestSubscriptionChangeRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.RequestSubscriptionChangeRequest)
private static final com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest();
}
public static com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RequestSubscriptionChangeRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RequestSubscriptionChangeRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_AutoAssignCriteria_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_AutoAssignCriteria_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_AutoAssignCriteria_TagCriteria_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_AutoAssignCriteria_TagCriteria_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_AutoAssignRule_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_AutoAssignRule_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_GetAutoAssignConfigRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_GetAutoAssignConfigRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_GetAutoAssignConfigResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_GetAutoAssignConfigResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_GetSalespersonRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_GetSalespersonRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_PagedResponseMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_PagedResponseMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_PagedRequestOptions_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_PagedRequestOptions_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_ListSalesTeamsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_ListSalesTeamsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_ListSalesTeamsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_ListSalesTeamsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_BusinessSearchRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_BusinessSearchRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_BusinessSearchResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_BusinessSearchResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_RequestSubscriptionChangeRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_RequestSubscriptionChangeRequest_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\022sales/v1/api.proto\022\010sales.v1\032\033google/p" +
"rotobuf/empty.proto\032\032sales/v1/salesperso" +
"n.proto\032\031sales/v1/sales_team.proto\032\027sale" +
"s/v1/business.proto\032\033sales/v1/user_actio" +
"ns.proto\"\275\004\n\022AutoAssignCriteria\022J\n\021locat" +
"ion_criteria\030\001 \001(\0132-.sales.v1.AutoAssign" +
"Criteria.LocationCriteriaH\000\022J\n\021category_" +
"criteria\030\002 \001(\0132-.sales.v1.AutoAssignCrit" +
"eria.CategoryCriteriaH\000\022@\n\014tag_criteria\030" +
"\003 \001(\0132(.sales.v1.AutoAssignCriteria.TagC",
"riteriaH\000\022Y\n\031conversion_point_criteria\030\004" +
" \001(\01324.sales.v1.AutoAssignCriteria.Conve" +
"rsionPointCriteriaH\000\032@\n\020LocationCriteria" +
"\022\017\n\007country\030\001 \001(\t\022\r\n\005state\030\002 \001(\t\022\014\n\004city" +
"\030\003 \001(\t\032&\n\020CategoryCriteria\022\022\n\ncategories" +
"\030\002 \003(\t\032G\n\013TagCriteria\022\014\n\004tags\030\001 \003(\t\022*\n\nm" +
"atch_type\030\002 \001(\0162\026.sales.v1.TagMatchType\032" +
"3\n\027ConversionPointCriteria\022\030\n\020conversion" +
"_point\030\001 \003(\tB\n\n\010criteria\"\223\001\n\016AutoAssignR" +
"ule\022.\n\010criteria\030\001 \003(\0132\034.sales.v1.AutoAss",
"ignCriteria\022\027\n\017salesperson_ids\030\002 \003(\t\0228\n\023" +
"criteria_match_type\030\003 \001(\0162\033.sales.v1.Cri" +
"teriaMatchType\"C\n\032GetAutoAssignConfigReq" +
"uest\022\022\n\npartner_id\030\001 \001(\t\022\021\n\tmarket_id\030\002 " +
"\001(\t\"m\n\033GetAutoAssignConfigResponse\022\022\n\npa" +
"rtner_id\030\001 \001(\t\022\021\n\tmarket_id\030\002 \001(\t\022\'\n\005rul" +
"es\030\003 \003(\0132\030.sales.v1.AutoAssignRule\"w\n%Cr" +
"eateOrUpdateAutoAssignConfigRequest\022\022\n\np" +
"artner_id\030\001 \001(\t\022\021\n\tmarket_id\030\002 \001(\t\022\'\n\005ru" +
"les\030\003 \003(\0132\030.sales.v1.AutoAssignRule\"^\n\025G",
"etSalespersonRequest\022\022\n\npartner_id\030\001 \001(\t" +
"\022\030\n\016salesperson_id\030\002 \001(\tH\000\022\021\n\007user_id\030\003 " +
"\001(\tH\000B\004\n\002id\">\n\025PagedResponseMetadata\022\023\n\013" +
"next_cursor\030\001 \001(\t\022\020\n\010has_more\030\002 \001(\010\"8\n\023P" +
"agedRequestOptions\022\016\n\006cursor\030\001 \001(\t\022\021\n\tpa" +
"ge_size\030\002 \001(\003\"\326\001\n\025ListSalesTeamsRequest\022" +
"F\n\007filters\030\001 \001(\01325.sales.v1.ListSalesTea" +
"msRequest.ListSalesTeamsFilters\0225\n\016pagin" +
"g_options\030\002 \001(\0132\035.sales.v1.PagedRequestO" +
"ptions\032>\n\025ListSalesTeamsFilters\022\022\n\npartn",
"er_id\030\001 \001(\t\022\021\n\tmarket_id\030\002 \001(\t\"|\n\026ListSa" +
"lesTeamsResponse\022(\n\013sales_teams\030\001 \003(\0132\023." +
"sales.v1.SalesTeam\0228\n\017paging_metadata\030\002 " +
"\001(\0132\037.sales.v1.PagedResponseMetadata\"\273\001\n" +
"\025BusinessSearchRequest\0220\n\007filters\030\001 \001(\0132" +
"\037.sales.v1.BusinessSearchFilters\0225\n\016pagi" +
"ng_options\030\002 \001(\0132\035.sales.v1.PagedRequest" +
"Options\0229\n\014sort_options\030\003 \001(\0132#.sales.v1" +
".BusinessSearchSortOptions\"\202\001\n\026BusinessS" +
"earchResponse\022&\n\nbusinesses\030\001 \003(\0132\022.sale",
"s.v1.Business\022@\n\017paging_metadata\030\002 \001(\0132\'" +
".sales.v1.BusinessPagedResponseMetadata\"" +
"l\n RequestSubscriptionChangeRequest\022\023\n\013b" +
"usiness_id\030\001 \001(\t\022\017\n\007message\030\002 \001(\t\022\024\n\014pho" +
"ne_number\030\003 \001(\t\022\014\n\004name\030\004 \001(\t*>\n\014TagMatc" +
"hType\022\026\n\022TAG_MATCH_TYPE_ALL\020\000\022\026\n\022TAG_MAT" +
"CH_TYPE_ANY\020\001*M\n\021CriteriaMatchType\022\033\n\027CR" +
"ITERIA_MATCH_TYPE_ALL\020\000\022\033\n\027CRITERIA_MATC" +
"H_TYPE_ANY\020\0012\240\002\n\005Sales\022b\n\023GetAutoAssignC" +
"onfig\022$.sales.v1.GetAutoAssignConfigRequ",
"est\032%.sales.v1.GetAutoAssignConfigRespon" +
"se\022i\n\036CreateOrUpdateAutoAssignConfig\022/.s" +
"ales.v1.CreateOrUpdateAutoAssignConfigRe" +
"quest\032\026.google.protobuf.Empty\022H\n\016GetSale" +
"sperson\022\037.sales.v1.GetSalespersonRequest" +
"\032\025.sales.v1.Salesperson2]\n\020SalesTeamServ" +
"ice\022I\n\004List\022\037.sales.v1.ListSalesTeamsReq" +
"uest\032 .sales.v1.ListSalesTeamsResponse2\277" +
"\001\n\017BusinessService\022K\n\006Search\022\037.sales.v1." +
"BusinessSearchRequest\032 .sales.v1.Busines",
"sSearchResponse\022_\n\031RequestSubscriptionCh" +
"ange\022*.sales.v1.RequestSubscriptionChang" +
"eRequest\032\026.google.protobuf.Empty2\364\002\n\023Use" +
"rActionsProvider\022H\n\020UploadUserAction\022\034.s" +
"ales.v1.UserActionsRequest\032\026.google.prot" +
"obuf.Empty\022g\n\035UploadUserActionForCustome" +
"rID\022..sales.v1.UploadUserActionForCustom" +
"erIDRequest\032\026.google.protobuf.Empty\022W\n\025B" +
"ulkUploadUserActions\022&.sales.v1.BulkUplo" +
"adUserActionsRequest\032\026.google.protobuf.E",
"mpty\022Q\n\017ListUserActions\022\037.sales.v1.GetUs" +
"erActionsRequest\032\035.sales.v1.UserActionsR" +
"esponseBf\n\037com.vendasta.sales.v1.generat" +
"edB\010ApiProtoZ9github.com/vendasta/genera" +
"ted-protos-go/sales/v1;sales/v1b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.EmptyProto.getDescriptor(),
com.vendasta.sales.v1.generated.SalespersonProto.getDescriptor(),
com.vendasta.sales.v1.generated.SalesTeamProto.getDescriptor(),
com.vendasta.sales.v1.generated.BusinessProto.getDescriptor(),
com.vendasta.sales.v1.generated.UserActionsProto.getDescriptor(),
}, assigner);
internal_static_sales_v1_AutoAssignCriteria_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_sales_v1_AutoAssignCriteria_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_AutoAssignCriteria_descriptor,
new java.lang.String[] { "LocationCriteria", "CategoryCriteria", "TagCriteria", "ConversionPointCriteria", "Criteria", });
internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_descriptor =
internal_static_sales_v1_AutoAssignCriteria_descriptor.getNestedTypes().get(0);
internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_AutoAssignCriteria_LocationCriteria_descriptor,
new java.lang.String[] { "Country", "State", "City", });
internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_descriptor =
internal_static_sales_v1_AutoAssignCriteria_descriptor.getNestedTypes().get(1);
internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_AutoAssignCriteria_CategoryCriteria_descriptor,
new java.lang.String[] { "Categories", });
internal_static_sales_v1_AutoAssignCriteria_TagCriteria_descriptor =
internal_static_sales_v1_AutoAssignCriteria_descriptor.getNestedTypes().get(2);
internal_static_sales_v1_AutoAssignCriteria_TagCriteria_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_AutoAssignCriteria_TagCriteria_descriptor,
new java.lang.String[] { "Tags", "MatchType", });
internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_descriptor =
internal_static_sales_v1_AutoAssignCriteria_descriptor.getNestedTypes().get(3);
internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_AutoAssignCriteria_ConversionPointCriteria_descriptor,
new java.lang.String[] { "ConversionPoint", });
internal_static_sales_v1_AutoAssignRule_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_sales_v1_AutoAssignRule_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_AutoAssignRule_descriptor,
new java.lang.String[] { "Criteria", "SalespersonIds", "CriteriaMatchType", });
internal_static_sales_v1_GetAutoAssignConfigRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_sales_v1_GetAutoAssignConfigRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_GetAutoAssignConfigRequest_descriptor,
new java.lang.String[] { "PartnerId", "MarketId", });
internal_static_sales_v1_GetAutoAssignConfigResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_sales_v1_GetAutoAssignConfigResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_GetAutoAssignConfigResponse_descriptor,
new java.lang.String[] { "PartnerId", "MarketId", "Rules", });
internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_CreateOrUpdateAutoAssignConfigRequest_descriptor,
new java.lang.String[] { "PartnerId", "MarketId", "Rules", });
internal_static_sales_v1_GetSalespersonRequest_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_sales_v1_GetSalespersonRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_GetSalespersonRequest_descriptor,
new java.lang.String[] { "PartnerId", "SalespersonId", "UserId", "Id", });
internal_static_sales_v1_PagedResponseMetadata_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_sales_v1_PagedResponseMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_PagedResponseMetadata_descriptor,
new java.lang.String[] { "NextCursor", "HasMore", });
internal_static_sales_v1_PagedRequestOptions_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_sales_v1_PagedRequestOptions_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_PagedRequestOptions_descriptor,
new java.lang.String[] { "Cursor", "PageSize", });
internal_static_sales_v1_ListSalesTeamsRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_sales_v1_ListSalesTeamsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_ListSalesTeamsRequest_descriptor,
new java.lang.String[] { "Filters", "PagingOptions", });
internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_descriptor =
internal_static_sales_v1_ListSalesTeamsRequest_descriptor.getNestedTypes().get(0);
internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_ListSalesTeamsRequest_ListSalesTeamsFilters_descriptor,
new java.lang.String[] { "PartnerId", "MarketId", });
internal_static_sales_v1_ListSalesTeamsResponse_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_sales_v1_ListSalesTeamsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_ListSalesTeamsResponse_descriptor,
new java.lang.String[] { "SalesTeams", "PagingMetadata", });
internal_static_sales_v1_BusinessSearchRequest_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_sales_v1_BusinessSearchRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_BusinessSearchRequest_descriptor,
new java.lang.String[] { "Filters", "PagingOptions", "SortOptions", });
internal_static_sales_v1_BusinessSearchResponse_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_sales_v1_BusinessSearchResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_BusinessSearchResponse_descriptor,
new java.lang.String[] { "Businesses", "PagingMetadata", });
internal_static_sales_v1_RequestSubscriptionChangeRequest_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_sales_v1_RequestSubscriptionChangeRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_RequestSubscriptionChangeRequest_descriptor,
new java.lang.String[] { "BusinessId", "Message", "PhoneNumber", "Name", });
com.google.protobuf.EmptyProto.getDescriptor();
com.vendasta.sales.v1.generated.SalespersonProto.getDescriptor();
com.vendasta.sales.v1.generated.SalesTeamProto.getDescriptor();
com.vendasta.sales.v1.generated.BusinessProto.getDescriptor();
com.vendasta.sales.v1.generated.UserActionsProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy