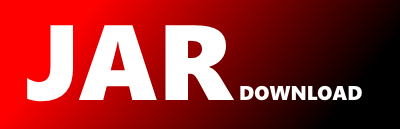
com.vendasta.sales.v1.generated.BusinessProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: sales/v1/business.proto
package com.vendasta.sales.v1.generated;
public final class BusinessProto {
private BusinessProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code sales.v1.Campaign}
*/
public enum Campaign
implements com.google.protobuf.ProtocolMessageEnum {
/**
* CAMPAIGN_ANY = 0;
*/
CAMPAIGN_ANY(0),
/**
* CAMPAIGN_ON = 1;
*/
CAMPAIGN_ON(1),
/**
* CAMPAIGN_OFF = 2;
*/
CAMPAIGN_OFF(2),
UNRECOGNIZED(-1),
;
/**
* CAMPAIGN_ANY = 0;
*/
public static final int CAMPAIGN_ANY_VALUE = 0;
/**
* CAMPAIGN_ON = 1;
*/
public static final int CAMPAIGN_ON_VALUE = 1;
/**
* CAMPAIGN_OFF = 2;
*/
public static final int CAMPAIGN_OFF_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Campaign valueOf(int value) {
return forNumber(value);
}
public static Campaign forNumber(int value) {
switch (value) {
case 0: return CAMPAIGN_ANY;
case 1: return CAMPAIGN_ON;
case 2: return CAMPAIGN_OFF;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Campaign> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Campaign findValueByNumber(int number) {
return Campaign.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.getDescriptor().getEnumTypes().get(0);
}
private static final Campaign[] VALUES = values();
public static Campaign valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Campaign(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sales.v1.Campaign)
}
/**
* Protobuf enum {@code sales.v1.Snapshot}
*/
public enum Snapshot
implements com.google.protobuf.ProtocolMessageEnum {
/**
* SNAPSHOT_ANY = 0;
*/
SNAPSHOT_ANY(0),
/**
* SNAPSHOT_SENT = 1;
*/
SNAPSHOT_SENT(1),
/**
* SNAPSHOT_NOT_SENT = 2;
*/
SNAPSHOT_NOT_SENT(2),
UNRECOGNIZED(-1),
;
/**
* SNAPSHOT_ANY = 0;
*/
public static final int SNAPSHOT_ANY_VALUE = 0;
/**
* SNAPSHOT_SENT = 1;
*/
public static final int SNAPSHOT_SENT_VALUE = 1;
/**
* SNAPSHOT_NOT_SENT = 2;
*/
public static final int SNAPSHOT_NOT_SENT_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Snapshot valueOf(int value) {
return forNumber(value);
}
public static Snapshot forNumber(int value) {
switch (value) {
case 0: return SNAPSHOT_ANY;
case 1: return SNAPSHOT_SENT;
case 2: return SNAPSHOT_NOT_SENT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Snapshot> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Snapshot findValueByNumber(int number) {
return Snapshot.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.getDescriptor().getEnumTypes().get(1);
}
private static final Snapshot[] VALUES = values();
public static Snapshot valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Snapshot(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sales.v1.Snapshot)
}
/**
* Protobuf enum {@code sales.v1.Archived}
*/
public enum Archived
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ARCHIVED_ANY = 0;
*/
ARCHIVED_ANY(0),
/**
* ARCHIVED_YES = 1;
*/
ARCHIVED_YES(1),
/**
* ARCHIVED_NO = 2;
*/
ARCHIVED_NO(2),
UNRECOGNIZED(-1),
;
/**
* ARCHIVED_ANY = 0;
*/
public static final int ARCHIVED_ANY_VALUE = 0;
/**
* ARCHIVED_YES = 1;
*/
public static final int ARCHIVED_YES_VALUE = 1;
/**
* ARCHIVED_NO = 2;
*/
public static final int ARCHIVED_NO_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Archived valueOf(int value) {
return forNumber(value);
}
public static Archived forNumber(int value) {
switch (value) {
case 0: return ARCHIVED_ANY;
case 1: return ARCHIVED_YES;
case 2: return ARCHIVED_NO;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Archived> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Archived findValueByNumber(int number) {
return Archived.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.getDescriptor().getEnumTypes().get(2);
}
private static final Archived[] VALUES = values();
public static Archived valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Archived(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sales.v1.Archived)
}
public interface BusinessPagedResponseMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.BusinessPagedResponseMetadata)
com.google.protobuf.MessageOrBuilder {
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
java.lang.String getNextCursor();
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
com.google.protobuf.ByteString
getNextCursorBytes();
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
boolean getHasMore();
/**
*
* The total number of results for this query across all pages
*
*
* int64 total_results = 3;
*/
long getTotalResults();
}
/**
*
* Contains metadata about the paged response
*
*
* Protobuf type {@code sales.v1.BusinessPagedResponseMetadata}
*/
public static final class BusinessPagedResponseMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.BusinessPagedResponseMetadata)
BusinessPagedResponseMetadataOrBuilder {
// Use BusinessPagedResponseMetadata.newBuilder() to construct.
private BusinessPagedResponseMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BusinessPagedResponseMetadata() {
nextCursor_ = "";
hasMore_ = false;
totalResults_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private BusinessPagedResponseMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
nextCursor_ = s;
break;
}
case 16: {
hasMore_ = input.readBool();
break;
}
case 24: {
totalResults_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessPagedResponseMetadata_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessPagedResponseMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.class, com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.Builder.class);
}
public static final int NEXT_CURSOR_FIELD_NUMBER = 1;
private volatile java.lang.Object nextCursor_;
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public java.lang.String getNextCursor() {
java.lang.Object ref = nextCursor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nextCursor_ = s;
return s;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public com.google.protobuf.ByteString
getNextCursorBytes() {
java.lang.Object ref = nextCursor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nextCursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HAS_MORE_FIELD_NUMBER = 2;
private boolean hasMore_;
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public boolean getHasMore() {
return hasMore_;
}
public static final int TOTAL_RESULTS_FIELD_NUMBER = 3;
private long totalResults_;
/**
*
* The total number of results for this query across all pages
*
*
* int64 total_results = 3;
*/
public long getTotalResults() {
return totalResults_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNextCursorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, nextCursor_);
}
if (hasMore_ != false) {
output.writeBool(2, hasMore_);
}
if (totalResults_ != 0L) {
output.writeInt64(3, totalResults_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNextCursorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, nextCursor_);
}
if (hasMore_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, hasMore_);
}
if (totalResults_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, totalResults_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata other = (com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata) obj;
boolean result = true;
result = result && getNextCursor()
.equals(other.getNextCursor());
result = result && (getHasMore()
== other.getHasMore());
result = result && (getTotalResults()
== other.getTotalResults());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NEXT_CURSOR_FIELD_NUMBER;
hash = (53 * hash) + getNextCursor().hashCode();
hash = (37 * hash) + HAS_MORE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasMore());
hash = (37 * hash) + TOTAL_RESULTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotalResults());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Contains metadata about the paged response
*
*
* Protobuf type {@code sales.v1.BusinessPagedResponseMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.BusinessPagedResponseMetadata)
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessPagedResponseMetadata_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessPagedResponseMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.class, com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
nextCursor_ = "";
hasMore_ = false;
totalResults_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessPagedResponseMetadata_descriptor;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata build() {
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata buildPartial() {
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata result = new com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata(this);
result.nextCursor_ = nextCursor_;
result.hasMore_ = hasMore_;
result.totalResults_ = totalResults_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata) {
return mergeFrom((com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata other) {
if (other == com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata.getDefaultInstance()) return this;
if (!other.getNextCursor().isEmpty()) {
nextCursor_ = other.nextCursor_;
onChanged();
}
if (other.getHasMore() != false) {
setHasMore(other.getHasMore());
}
if (other.getTotalResults() != 0L) {
setTotalResults(other.getTotalResults());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object nextCursor_ = "";
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public java.lang.String getNextCursor() {
java.lang.Object ref = nextCursor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nextCursor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public com.google.protobuf.ByteString
getNextCursorBytes() {
java.lang.Object ref = nextCursor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nextCursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder setNextCursor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nextCursor_ = value;
onChanged();
return this;
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder clearNextCursor() {
nextCursor_ = getDefaultInstance().getNextCursor();
onChanged();
return this;
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder setNextCursorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nextCursor_ = value;
onChanged();
return this;
}
private boolean hasMore_ ;
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public boolean getHasMore() {
return hasMore_;
}
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public Builder setHasMore(boolean value) {
hasMore_ = value;
onChanged();
return this;
}
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public Builder clearHasMore() {
hasMore_ = false;
onChanged();
return this;
}
private long totalResults_ ;
/**
*
* The total number of results for this query across all pages
*
*
* int64 total_results = 3;
*/
public long getTotalResults() {
return totalResults_;
}
/**
*
* The total number of results for this query across all pages
*
*
* int64 total_results = 3;
*/
public Builder setTotalResults(long value) {
totalResults_ = value;
onChanged();
return this;
}
/**
*
* The total number of results for this query across all pages
*
*
* int64 total_results = 3;
*/
public Builder clearTotalResults() {
totalResults_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.BusinessPagedResponseMetadata)
}
// @@protoc_insertion_point(class_scope:sales.v1.BusinessPagedResponseMetadata)
private static final com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata();
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public BusinessPagedResponseMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BusinessPagedResponseMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessPagedResponseMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BusinessSearchFiltersOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.BusinessSearchFilters)
com.google.protobuf.MessageOrBuilder {
/**
* string partner_id = 1;
*/
java.lang.String getPartnerId();
/**
* string partner_id = 1;
*/
com.google.protobuf.ByteString
getPartnerIdBytes();
/**
* string market_id = 2;
*/
java.lang.String getMarketId();
/**
* string market_id = 2;
*/
com.google.protobuf.ByteString
getMarketIdBytes();
/**
* repeated string sales_statuses = 3;
*/
java.util.List
getSalesStatusesList();
/**
* repeated string sales_statuses = 3;
*/
int getSalesStatusesCount();
/**
* repeated string sales_statuses = 3;
*/
java.lang.String getSalesStatuses(int index);
/**
* repeated string sales_statuses = 3;
*/
com.google.protobuf.ByteString
getSalesStatusesBytes(int index);
/**
* string sales_action = 4;
*/
java.lang.String getSalesAction();
/**
* string sales_action = 4;
*/
com.google.protobuf.ByteString
getSalesActionBytes();
/**
* .google.protobuf.Timestamp from_date = 5;
*/
boolean hasFromDate();
/**
* .google.protobuf.Timestamp from_date = 5;
*/
com.google.protobuf.Timestamp getFromDate();
/**
* .google.protobuf.Timestamp from_date = 5;
*/
com.google.protobuf.TimestampOrBuilder getFromDateOrBuilder();
/**
* .google.protobuf.Timestamp to_date = 6;
*/
boolean hasToDate();
/**
* .google.protobuf.Timestamp to_date = 6;
*/
com.google.protobuf.Timestamp getToDate();
/**
* .google.protobuf.Timestamp to_date = 6;
*/
com.google.protobuf.TimestampOrBuilder getToDateOrBuilder();
/**
* .sales.v1.Archived archived = 7;
*/
int getArchivedValue();
/**
* .sales.v1.Archived archived = 7;
*/
com.vendasta.sales.v1.generated.BusinessProto.Archived getArchived();
/**
* repeated string account_tags = 8;
*/
java.util.List
getAccountTagsList();
/**
* repeated string account_tags = 8;
*/
int getAccountTagsCount();
/**
* repeated string account_tags = 8;
*/
java.lang.String getAccountTags(int index);
/**
* repeated string account_tags = 8;
*/
com.google.protobuf.ByteString
getAccountTagsBytes(int index);
/**
* repeated string business_categories = 9;
*/
java.util.List
getBusinessCategoriesList();
/**
* repeated string business_categories = 9;
*/
int getBusinessCategoriesCount();
/**
* repeated string business_categories = 9;
*/
java.lang.String getBusinessCategories(int index);
/**
* repeated string business_categories = 9;
*/
com.google.protobuf.ByteString
getBusinessCategoriesBytes(int index);
/**
* .sales.v1.Campaign on_campaign = 10;
*/
int getOnCampaignValue();
/**
* .sales.v1.Campaign on_campaign = 10;
*/
com.vendasta.sales.v1.generated.BusinessProto.Campaign getOnCampaign();
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
int getSnapshotSentValue();
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
com.vendasta.sales.v1.generated.BusinessProto.Snapshot getSnapshotSent();
/**
* string query = 12;
*/
java.lang.String getQuery();
/**
* string query = 12;
*/
com.google.protobuf.ByteString
getQueryBytes();
/**
* repeated string assignees = 13;
*/
java.util.List
getAssigneesList();
/**
* repeated string assignees = 13;
*/
int getAssigneesCount();
/**
* repeated string assignees = 13;
*/
java.lang.String getAssignees(int index);
/**
* repeated string assignees = 13;
*/
com.google.protobuf.ByteString
getAssigneesBytes(int index);
/**
* string goal = 14;
*/
java.lang.String getGoal();
/**
* string goal = 14;
*/
com.google.protobuf.ByteString
getGoalBytes();
/**
* string training_priorities = 15;
*/
java.lang.String getTrainingPriorities();
/**
* string training_priorities = 15;
*/
com.google.protobuf.ByteString
getTrainingPrioritiesBytes();
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
java.lang.String getCustomFieldPartnerId();
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
com.google.protobuf.ByteString
getCustomFieldPartnerIdBytes();
}
/**
* Protobuf type {@code sales.v1.BusinessSearchFilters}
*/
public static final class BusinessSearchFilters extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.BusinessSearchFilters)
BusinessSearchFiltersOrBuilder {
// Use BusinessSearchFilters.newBuilder() to construct.
private BusinessSearchFilters(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BusinessSearchFilters() {
partnerId_ = "";
marketId_ = "";
salesStatuses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
salesAction_ = "";
archived_ = 0;
accountTags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
businessCategories_ = com.google.protobuf.LazyStringArrayList.EMPTY;
onCampaign_ = 0;
snapshotSent_ = 0;
query_ = "";
assignees_ = com.google.protobuf.LazyStringArrayList.EMPTY;
goal_ = "";
trainingPriorities_ = "";
customFieldPartnerId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private BusinessSearchFilters(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
partnerId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
marketId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
salesStatuses_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000004;
}
salesStatuses_.add(s);
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
salesAction_ = s;
break;
}
case 42: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (fromDate_ != null) {
subBuilder = fromDate_.toBuilder();
}
fromDate_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(fromDate_);
fromDate_ = subBuilder.buildPartial();
}
break;
}
case 50: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (toDate_ != null) {
subBuilder = toDate_.toBuilder();
}
toDate_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(toDate_);
toDate_ = subBuilder.buildPartial();
}
break;
}
case 56: {
int rawValue = input.readEnum();
archived_ = rawValue;
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
accountTags_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000080;
}
accountTags_.add(s);
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
businessCategories_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000100;
}
businessCategories_.add(s);
break;
}
case 80: {
int rawValue = input.readEnum();
onCampaign_ = rawValue;
break;
}
case 88: {
int rawValue = input.readEnum();
snapshotSent_ = rawValue;
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
query_ = s;
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00001000) == 0x00001000)) {
assignees_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00001000;
}
assignees_.add(s);
break;
}
case 114: {
java.lang.String s = input.readStringRequireUtf8();
goal_ = s;
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
trainingPriorities_ = s;
break;
}
case 130: {
java.lang.String s = input.readStringRequireUtf8();
customFieldPartnerId_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
salesStatuses_ = salesStatuses_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
accountTags_ = accountTags_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
businessCategories_ = businessCategories_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00001000) == 0x00001000)) {
assignees_ = assignees_.getUnmodifiableView();
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchFilters_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchFilters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.class, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.Builder.class);
}
private int bitField0_;
public static final int PARTNER_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object partnerId_;
/**
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
}
}
/**
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MARKET_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object marketId_;
/**
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
}
}
/**
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SALES_STATUSES_FIELD_NUMBER = 3;
private com.google.protobuf.LazyStringList salesStatuses_;
/**
* repeated string sales_statuses = 3;
*/
public com.google.protobuf.ProtocolStringList
getSalesStatusesList() {
return salesStatuses_;
}
/**
* repeated string sales_statuses = 3;
*/
public int getSalesStatusesCount() {
return salesStatuses_.size();
}
/**
* repeated string sales_statuses = 3;
*/
public java.lang.String getSalesStatuses(int index) {
return salesStatuses_.get(index);
}
/**
* repeated string sales_statuses = 3;
*/
public com.google.protobuf.ByteString
getSalesStatusesBytes(int index) {
return salesStatuses_.getByteString(index);
}
public static final int SALES_ACTION_FIELD_NUMBER = 4;
private volatile java.lang.Object salesAction_;
/**
* string sales_action = 4;
*/
public java.lang.String getSalesAction() {
java.lang.Object ref = salesAction_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
salesAction_ = s;
return s;
}
}
/**
* string sales_action = 4;
*/
public com.google.protobuf.ByteString
getSalesActionBytes() {
java.lang.Object ref = salesAction_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
salesAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FROM_DATE_FIELD_NUMBER = 5;
private com.google.protobuf.Timestamp fromDate_;
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public boolean hasFromDate() {
return fromDate_ != null;
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public com.google.protobuf.Timestamp getFromDate() {
return fromDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : fromDate_;
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public com.google.protobuf.TimestampOrBuilder getFromDateOrBuilder() {
return getFromDate();
}
public static final int TO_DATE_FIELD_NUMBER = 6;
private com.google.protobuf.Timestamp toDate_;
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public boolean hasToDate() {
return toDate_ != null;
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public com.google.protobuf.Timestamp getToDate() {
return toDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : toDate_;
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public com.google.protobuf.TimestampOrBuilder getToDateOrBuilder() {
return getToDate();
}
public static final int ARCHIVED_FIELD_NUMBER = 7;
private int archived_;
/**
* .sales.v1.Archived archived = 7;
*/
public int getArchivedValue() {
return archived_;
}
/**
* .sales.v1.Archived archived = 7;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Archived getArchived() {
com.vendasta.sales.v1.generated.BusinessProto.Archived result = com.vendasta.sales.v1.generated.BusinessProto.Archived.valueOf(archived_);
return result == null ? com.vendasta.sales.v1.generated.BusinessProto.Archived.UNRECOGNIZED : result;
}
public static final int ACCOUNT_TAGS_FIELD_NUMBER = 8;
private com.google.protobuf.LazyStringList accountTags_;
/**
* repeated string account_tags = 8;
*/
public com.google.protobuf.ProtocolStringList
getAccountTagsList() {
return accountTags_;
}
/**
* repeated string account_tags = 8;
*/
public int getAccountTagsCount() {
return accountTags_.size();
}
/**
* repeated string account_tags = 8;
*/
public java.lang.String getAccountTags(int index) {
return accountTags_.get(index);
}
/**
* repeated string account_tags = 8;
*/
public com.google.protobuf.ByteString
getAccountTagsBytes(int index) {
return accountTags_.getByteString(index);
}
public static final int BUSINESS_CATEGORIES_FIELD_NUMBER = 9;
private com.google.protobuf.LazyStringList businessCategories_;
/**
* repeated string business_categories = 9;
*/
public com.google.protobuf.ProtocolStringList
getBusinessCategoriesList() {
return businessCategories_;
}
/**
* repeated string business_categories = 9;
*/
public int getBusinessCategoriesCount() {
return businessCategories_.size();
}
/**
* repeated string business_categories = 9;
*/
public java.lang.String getBusinessCategories(int index) {
return businessCategories_.get(index);
}
/**
* repeated string business_categories = 9;
*/
public com.google.protobuf.ByteString
getBusinessCategoriesBytes(int index) {
return businessCategories_.getByteString(index);
}
public static final int ON_CAMPAIGN_FIELD_NUMBER = 10;
private int onCampaign_;
/**
* .sales.v1.Campaign on_campaign = 10;
*/
public int getOnCampaignValue() {
return onCampaign_;
}
/**
* .sales.v1.Campaign on_campaign = 10;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Campaign getOnCampaign() {
com.vendasta.sales.v1.generated.BusinessProto.Campaign result = com.vendasta.sales.v1.generated.BusinessProto.Campaign.valueOf(onCampaign_);
return result == null ? com.vendasta.sales.v1.generated.BusinessProto.Campaign.UNRECOGNIZED : result;
}
public static final int SNAPSHOT_SENT_FIELD_NUMBER = 11;
private int snapshotSent_;
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
public int getSnapshotSentValue() {
return snapshotSent_;
}
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Snapshot getSnapshotSent() {
com.vendasta.sales.v1.generated.BusinessProto.Snapshot result = com.vendasta.sales.v1.generated.BusinessProto.Snapshot.valueOf(snapshotSent_);
return result == null ? com.vendasta.sales.v1.generated.BusinessProto.Snapshot.UNRECOGNIZED : result;
}
public static final int QUERY_FIELD_NUMBER = 12;
private volatile java.lang.Object query_;
/**
* string query = 12;
*/
public java.lang.String getQuery() {
java.lang.Object ref = query_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
query_ = s;
return s;
}
}
/**
* string query = 12;
*/
public com.google.protobuf.ByteString
getQueryBytes() {
java.lang.Object ref = query_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
query_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ASSIGNEES_FIELD_NUMBER = 13;
private com.google.protobuf.LazyStringList assignees_;
/**
* repeated string assignees = 13;
*/
public com.google.protobuf.ProtocolStringList
getAssigneesList() {
return assignees_;
}
/**
* repeated string assignees = 13;
*/
public int getAssigneesCount() {
return assignees_.size();
}
/**
* repeated string assignees = 13;
*/
public java.lang.String getAssignees(int index) {
return assignees_.get(index);
}
/**
* repeated string assignees = 13;
*/
public com.google.protobuf.ByteString
getAssigneesBytes(int index) {
return assignees_.getByteString(index);
}
public static final int GOAL_FIELD_NUMBER = 14;
private volatile java.lang.Object goal_;
/**
* string goal = 14;
*/
public java.lang.String getGoal() {
java.lang.Object ref = goal_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
goal_ = s;
return s;
}
}
/**
* string goal = 14;
*/
public com.google.protobuf.ByteString
getGoalBytes() {
java.lang.Object ref = goal_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
goal_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRAINING_PRIORITIES_FIELD_NUMBER = 15;
private volatile java.lang.Object trainingPriorities_;
/**
* string training_priorities = 15;
*/
public java.lang.String getTrainingPriorities() {
java.lang.Object ref = trainingPriorities_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
trainingPriorities_ = s;
return s;
}
}
/**
* string training_priorities = 15;
*/
public com.google.protobuf.ByteString
getTrainingPrioritiesBytes() {
java.lang.Object ref = trainingPriorities_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
trainingPriorities_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CUSTOM_FIELD_PARTNER_ID_FIELD_NUMBER = 16;
private volatile java.lang.Object customFieldPartnerId_;
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
public java.lang.String getCustomFieldPartnerId() {
java.lang.Object ref = customFieldPartnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customFieldPartnerId_ = s;
return s;
}
}
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
public com.google.protobuf.ByteString
getCustomFieldPartnerIdBytes() {
java.lang.Object ref = customFieldPartnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customFieldPartnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, marketId_);
}
for (int i = 0; i < salesStatuses_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, salesStatuses_.getRaw(i));
}
if (!getSalesActionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, salesAction_);
}
if (fromDate_ != null) {
output.writeMessage(5, getFromDate());
}
if (toDate_ != null) {
output.writeMessage(6, getToDate());
}
if (archived_ != com.vendasta.sales.v1.generated.BusinessProto.Archived.ARCHIVED_ANY.getNumber()) {
output.writeEnum(7, archived_);
}
for (int i = 0; i < accountTags_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, accountTags_.getRaw(i));
}
for (int i = 0; i < businessCategories_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, businessCategories_.getRaw(i));
}
if (onCampaign_ != com.vendasta.sales.v1.generated.BusinessProto.Campaign.CAMPAIGN_ANY.getNumber()) {
output.writeEnum(10, onCampaign_);
}
if (snapshotSent_ != com.vendasta.sales.v1.generated.BusinessProto.Snapshot.SNAPSHOT_ANY.getNumber()) {
output.writeEnum(11, snapshotSent_);
}
if (!getQueryBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, query_);
}
for (int i = 0; i < assignees_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, assignees_.getRaw(i));
}
if (!getGoalBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, goal_);
}
if (!getTrainingPrioritiesBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, trainingPriorities_);
}
if (!getCustomFieldPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, customFieldPartnerId_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, marketId_);
}
{
int dataSize = 0;
for (int i = 0; i < salesStatuses_.size(); i++) {
dataSize += computeStringSizeNoTag(salesStatuses_.getRaw(i));
}
size += dataSize;
size += 1 * getSalesStatusesList().size();
}
if (!getSalesActionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, salesAction_);
}
if (fromDate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getFromDate());
}
if (toDate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getToDate());
}
if (archived_ != com.vendasta.sales.v1.generated.BusinessProto.Archived.ARCHIVED_ANY.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, archived_);
}
{
int dataSize = 0;
for (int i = 0; i < accountTags_.size(); i++) {
dataSize += computeStringSizeNoTag(accountTags_.getRaw(i));
}
size += dataSize;
size += 1 * getAccountTagsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < businessCategories_.size(); i++) {
dataSize += computeStringSizeNoTag(businessCategories_.getRaw(i));
}
size += dataSize;
size += 1 * getBusinessCategoriesList().size();
}
if (onCampaign_ != com.vendasta.sales.v1.generated.BusinessProto.Campaign.CAMPAIGN_ANY.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, onCampaign_);
}
if (snapshotSent_ != com.vendasta.sales.v1.generated.BusinessProto.Snapshot.SNAPSHOT_ANY.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, snapshotSent_);
}
if (!getQueryBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, query_);
}
{
int dataSize = 0;
for (int i = 0; i < assignees_.size(); i++) {
dataSize += computeStringSizeNoTag(assignees_.getRaw(i));
}
size += dataSize;
size += 1 * getAssigneesList().size();
}
if (!getGoalBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, goal_);
}
if (!getTrainingPrioritiesBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, trainingPriorities_);
}
if (!getCustomFieldPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, customFieldPartnerId_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters other = (com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters) obj;
boolean result = true;
result = result && getPartnerId()
.equals(other.getPartnerId());
result = result && getMarketId()
.equals(other.getMarketId());
result = result && getSalesStatusesList()
.equals(other.getSalesStatusesList());
result = result && getSalesAction()
.equals(other.getSalesAction());
result = result && (hasFromDate() == other.hasFromDate());
if (hasFromDate()) {
result = result && getFromDate()
.equals(other.getFromDate());
}
result = result && (hasToDate() == other.hasToDate());
if (hasToDate()) {
result = result && getToDate()
.equals(other.getToDate());
}
result = result && archived_ == other.archived_;
result = result && getAccountTagsList()
.equals(other.getAccountTagsList());
result = result && getBusinessCategoriesList()
.equals(other.getBusinessCategoriesList());
result = result && onCampaign_ == other.onCampaign_;
result = result && snapshotSent_ == other.snapshotSent_;
result = result && getQuery()
.equals(other.getQuery());
result = result && getAssigneesList()
.equals(other.getAssigneesList());
result = result && getGoal()
.equals(other.getGoal());
result = result && getTrainingPriorities()
.equals(other.getTrainingPriorities());
result = result && getCustomFieldPartnerId()
.equals(other.getCustomFieldPartnerId());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartnerId().hashCode();
hash = (37 * hash) + MARKET_ID_FIELD_NUMBER;
hash = (53 * hash) + getMarketId().hashCode();
if (getSalesStatusesCount() > 0) {
hash = (37 * hash) + SALES_STATUSES_FIELD_NUMBER;
hash = (53 * hash) + getSalesStatusesList().hashCode();
}
hash = (37 * hash) + SALES_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getSalesAction().hashCode();
if (hasFromDate()) {
hash = (37 * hash) + FROM_DATE_FIELD_NUMBER;
hash = (53 * hash) + getFromDate().hashCode();
}
if (hasToDate()) {
hash = (37 * hash) + TO_DATE_FIELD_NUMBER;
hash = (53 * hash) + getToDate().hashCode();
}
hash = (37 * hash) + ARCHIVED_FIELD_NUMBER;
hash = (53 * hash) + archived_;
if (getAccountTagsCount() > 0) {
hash = (37 * hash) + ACCOUNT_TAGS_FIELD_NUMBER;
hash = (53 * hash) + getAccountTagsList().hashCode();
}
if (getBusinessCategoriesCount() > 0) {
hash = (37 * hash) + BUSINESS_CATEGORIES_FIELD_NUMBER;
hash = (53 * hash) + getBusinessCategoriesList().hashCode();
}
hash = (37 * hash) + ON_CAMPAIGN_FIELD_NUMBER;
hash = (53 * hash) + onCampaign_;
hash = (37 * hash) + SNAPSHOT_SENT_FIELD_NUMBER;
hash = (53 * hash) + snapshotSent_;
hash = (37 * hash) + QUERY_FIELD_NUMBER;
hash = (53 * hash) + getQuery().hashCode();
if (getAssigneesCount() > 0) {
hash = (37 * hash) + ASSIGNEES_FIELD_NUMBER;
hash = (53 * hash) + getAssigneesList().hashCode();
}
hash = (37 * hash) + GOAL_FIELD_NUMBER;
hash = (53 * hash) + getGoal().hashCode();
hash = (37 * hash) + TRAINING_PRIORITIES_FIELD_NUMBER;
hash = (53 * hash) + getTrainingPriorities().hashCode();
hash = (37 * hash) + CUSTOM_FIELD_PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getCustomFieldPartnerId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.BusinessSearchFilters}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.BusinessSearchFilters)
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFiltersOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchFilters_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchFilters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.class, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
partnerId_ = "";
marketId_ = "";
salesStatuses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
salesAction_ = "";
if (fromDateBuilder_ == null) {
fromDate_ = null;
} else {
fromDate_ = null;
fromDateBuilder_ = null;
}
if (toDateBuilder_ == null) {
toDate_ = null;
} else {
toDate_ = null;
toDateBuilder_ = null;
}
archived_ = 0;
accountTags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
businessCategories_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
onCampaign_ = 0;
snapshotSent_ = 0;
query_ = "";
assignees_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00001000);
goal_ = "";
trainingPriorities_ = "";
customFieldPartnerId_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchFilters_descriptor;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters build() {
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters buildPartial() {
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters result = new com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.partnerId_ = partnerId_;
result.marketId_ = marketId_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
salesStatuses_ = salesStatuses_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.salesStatuses_ = salesStatuses_;
result.salesAction_ = salesAction_;
if (fromDateBuilder_ == null) {
result.fromDate_ = fromDate_;
} else {
result.fromDate_ = fromDateBuilder_.build();
}
if (toDateBuilder_ == null) {
result.toDate_ = toDate_;
} else {
result.toDate_ = toDateBuilder_.build();
}
result.archived_ = archived_;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
accountTags_ = accountTags_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000080);
}
result.accountTags_ = accountTags_;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
businessCategories_ = businessCategories_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000100);
}
result.businessCategories_ = businessCategories_;
result.onCampaign_ = onCampaign_;
result.snapshotSent_ = snapshotSent_;
result.query_ = query_;
if (((bitField0_ & 0x00001000) == 0x00001000)) {
assignees_ = assignees_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00001000);
}
result.assignees_ = assignees_;
result.goal_ = goal_;
result.trainingPriorities_ = trainingPriorities_;
result.customFieldPartnerId_ = customFieldPartnerId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters) {
return mergeFrom((com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters other) {
if (other == com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters.getDefaultInstance()) return this;
if (!other.getPartnerId().isEmpty()) {
partnerId_ = other.partnerId_;
onChanged();
}
if (!other.getMarketId().isEmpty()) {
marketId_ = other.marketId_;
onChanged();
}
if (!other.salesStatuses_.isEmpty()) {
if (salesStatuses_.isEmpty()) {
salesStatuses_ = other.salesStatuses_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureSalesStatusesIsMutable();
salesStatuses_.addAll(other.salesStatuses_);
}
onChanged();
}
if (!other.getSalesAction().isEmpty()) {
salesAction_ = other.salesAction_;
onChanged();
}
if (other.hasFromDate()) {
mergeFromDate(other.getFromDate());
}
if (other.hasToDate()) {
mergeToDate(other.getToDate());
}
if (other.archived_ != 0) {
setArchivedValue(other.getArchivedValue());
}
if (!other.accountTags_.isEmpty()) {
if (accountTags_.isEmpty()) {
accountTags_ = other.accountTags_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureAccountTagsIsMutable();
accountTags_.addAll(other.accountTags_);
}
onChanged();
}
if (!other.businessCategories_.isEmpty()) {
if (businessCategories_.isEmpty()) {
businessCategories_ = other.businessCategories_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureBusinessCategoriesIsMutable();
businessCategories_.addAll(other.businessCategories_);
}
onChanged();
}
if (other.onCampaign_ != 0) {
setOnCampaignValue(other.getOnCampaignValue());
}
if (other.snapshotSent_ != 0) {
setSnapshotSentValue(other.getSnapshotSentValue());
}
if (!other.getQuery().isEmpty()) {
query_ = other.query_;
onChanged();
}
if (!other.assignees_.isEmpty()) {
if (assignees_.isEmpty()) {
assignees_ = other.assignees_;
bitField0_ = (bitField0_ & ~0x00001000);
} else {
ensureAssigneesIsMutable();
assignees_.addAll(other.assignees_);
}
onChanged();
}
if (!other.getGoal().isEmpty()) {
goal_ = other.goal_;
onChanged();
}
if (!other.getTrainingPriorities().isEmpty()) {
trainingPriorities_ = other.trainingPriorities_;
onChanged();
}
if (!other.getCustomFieldPartnerId().isEmpty()) {
customFieldPartnerId_ = other.customFieldPartnerId_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object partnerId_ = "";
/**
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string partner_id = 1;
*/
public Builder setPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partnerId_ = value;
onChanged();
return this;
}
/**
* string partner_id = 1;
*/
public Builder clearPartnerId() {
partnerId_ = getDefaultInstance().getPartnerId();
onChanged();
return this;
}
/**
* string partner_id = 1;
*/
public Builder setPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partnerId_ = value;
onChanged();
return this;
}
private java.lang.Object marketId_ = "";
/**
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string market_id = 2;
*/
public Builder setMarketId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
marketId_ = value;
onChanged();
return this;
}
/**
* string market_id = 2;
*/
public Builder clearMarketId() {
marketId_ = getDefaultInstance().getMarketId();
onChanged();
return this;
}
/**
* string market_id = 2;
*/
public Builder setMarketIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
marketId_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList salesStatuses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSalesStatusesIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
salesStatuses_ = new com.google.protobuf.LazyStringArrayList(salesStatuses_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated string sales_statuses = 3;
*/
public com.google.protobuf.ProtocolStringList
getSalesStatusesList() {
return salesStatuses_.getUnmodifiableView();
}
/**
* repeated string sales_statuses = 3;
*/
public int getSalesStatusesCount() {
return salesStatuses_.size();
}
/**
* repeated string sales_statuses = 3;
*/
public java.lang.String getSalesStatuses(int index) {
return salesStatuses_.get(index);
}
/**
* repeated string sales_statuses = 3;
*/
public com.google.protobuf.ByteString
getSalesStatusesBytes(int index) {
return salesStatuses_.getByteString(index);
}
/**
* repeated string sales_statuses = 3;
*/
public Builder setSalesStatuses(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSalesStatusesIsMutable();
salesStatuses_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sales_statuses = 3;
*/
public Builder addSalesStatuses(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSalesStatusesIsMutable();
salesStatuses_.add(value);
onChanged();
return this;
}
/**
* repeated string sales_statuses = 3;
*/
public Builder addAllSalesStatuses(
java.lang.Iterable values) {
ensureSalesStatusesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, salesStatuses_);
onChanged();
return this;
}
/**
* repeated string sales_statuses = 3;
*/
public Builder clearSalesStatuses() {
salesStatuses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* repeated string sales_statuses = 3;
*/
public Builder addSalesStatusesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSalesStatusesIsMutable();
salesStatuses_.add(value);
onChanged();
return this;
}
private java.lang.Object salesAction_ = "";
/**
* string sales_action = 4;
*/
public java.lang.String getSalesAction() {
java.lang.Object ref = salesAction_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
salesAction_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sales_action = 4;
*/
public com.google.protobuf.ByteString
getSalesActionBytes() {
java.lang.Object ref = salesAction_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
salesAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sales_action = 4;
*/
public Builder setSalesAction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
salesAction_ = value;
onChanged();
return this;
}
/**
* string sales_action = 4;
*/
public Builder clearSalesAction() {
salesAction_ = getDefaultInstance().getSalesAction();
onChanged();
return this;
}
/**
* string sales_action = 4;
*/
public Builder setSalesActionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
salesAction_ = value;
onChanged();
return this;
}
private com.google.protobuf.Timestamp fromDate_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> fromDateBuilder_;
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public boolean hasFromDate() {
return fromDateBuilder_ != null || fromDate_ != null;
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public com.google.protobuf.Timestamp getFromDate() {
if (fromDateBuilder_ == null) {
return fromDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : fromDate_;
} else {
return fromDateBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public Builder setFromDate(com.google.protobuf.Timestamp value) {
if (fromDateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
fromDate_ = value;
onChanged();
} else {
fromDateBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public Builder setFromDate(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (fromDateBuilder_ == null) {
fromDate_ = builderForValue.build();
onChanged();
} else {
fromDateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public Builder mergeFromDate(com.google.protobuf.Timestamp value) {
if (fromDateBuilder_ == null) {
if (fromDate_ != null) {
fromDate_ =
com.google.protobuf.Timestamp.newBuilder(fromDate_).mergeFrom(value).buildPartial();
} else {
fromDate_ = value;
}
onChanged();
} else {
fromDateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public Builder clearFromDate() {
if (fromDateBuilder_ == null) {
fromDate_ = null;
onChanged();
} else {
fromDate_ = null;
fromDateBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public com.google.protobuf.Timestamp.Builder getFromDateBuilder() {
onChanged();
return getFromDateFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
public com.google.protobuf.TimestampOrBuilder getFromDateOrBuilder() {
if (fromDateBuilder_ != null) {
return fromDateBuilder_.getMessageOrBuilder();
} else {
return fromDate_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : fromDate_;
}
}
/**
* .google.protobuf.Timestamp from_date = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getFromDateFieldBuilder() {
if (fromDateBuilder_ == null) {
fromDateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getFromDate(),
getParentForChildren(),
isClean());
fromDate_ = null;
}
return fromDateBuilder_;
}
private com.google.protobuf.Timestamp toDate_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> toDateBuilder_;
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public boolean hasToDate() {
return toDateBuilder_ != null || toDate_ != null;
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public com.google.protobuf.Timestamp getToDate() {
if (toDateBuilder_ == null) {
return toDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : toDate_;
} else {
return toDateBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public Builder setToDate(com.google.protobuf.Timestamp value) {
if (toDateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
toDate_ = value;
onChanged();
} else {
toDateBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public Builder setToDate(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (toDateBuilder_ == null) {
toDate_ = builderForValue.build();
onChanged();
} else {
toDateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public Builder mergeToDate(com.google.protobuf.Timestamp value) {
if (toDateBuilder_ == null) {
if (toDate_ != null) {
toDate_ =
com.google.protobuf.Timestamp.newBuilder(toDate_).mergeFrom(value).buildPartial();
} else {
toDate_ = value;
}
onChanged();
} else {
toDateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public Builder clearToDate() {
if (toDateBuilder_ == null) {
toDate_ = null;
onChanged();
} else {
toDate_ = null;
toDateBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public com.google.protobuf.Timestamp.Builder getToDateBuilder() {
onChanged();
return getToDateFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
public com.google.protobuf.TimestampOrBuilder getToDateOrBuilder() {
if (toDateBuilder_ != null) {
return toDateBuilder_.getMessageOrBuilder();
} else {
return toDate_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : toDate_;
}
}
/**
* .google.protobuf.Timestamp to_date = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getToDateFieldBuilder() {
if (toDateBuilder_ == null) {
toDateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getToDate(),
getParentForChildren(),
isClean());
toDate_ = null;
}
return toDateBuilder_;
}
private int archived_ = 0;
/**
* .sales.v1.Archived archived = 7;
*/
public int getArchivedValue() {
return archived_;
}
/**
* .sales.v1.Archived archived = 7;
*/
public Builder setArchivedValue(int value) {
archived_ = value;
onChanged();
return this;
}
/**
* .sales.v1.Archived archived = 7;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Archived getArchived() {
com.vendasta.sales.v1.generated.BusinessProto.Archived result = com.vendasta.sales.v1.generated.BusinessProto.Archived.valueOf(archived_);
return result == null ? com.vendasta.sales.v1.generated.BusinessProto.Archived.UNRECOGNIZED : result;
}
/**
* .sales.v1.Archived archived = 7;
*/
public Builder setArchived(com.vendasta.sales.v1.generated.BusinessProto.Archived value) {
if (value == null) {
throw new NullPointerException();
}
archived_ = value.getNumber();
onChanged();
return this;
}
/**
* .sales.v1.Archived archived = 7;
*/
public Builder clearArchived() {
archived_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList accountTags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureAccountTagsIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
accountTags_ = new com.google.protobuf.LazyStringArrayList(accountTags_);
bitField0_ |= 0x00000080;
}
}
/**
* repeated string account_tags = 8;
*/
public com.google.protobuf.ProtocolStringList
getAccountTagsList() {
return accountTags_.getUnmodifiableView();
}
/**
* repeated string account_tags = 8;
*/
public int getAccountTagsCount() {
return accountTags_.size();
}
/**
* repeated string account_tags = 8;
*/
public java.lang.String getAccountTags(int index) {
return accountTags_.get(index);
}
/**
* repeated string account_tags = 8;
*/
public com.google.protobuf.ByteString
getAccountTagsBytes(int index) {
return accountTags_.getByteString(index);
}
/**
* repeated string account_tags = 8;
*/
public Builder setAccountTags(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountTagsIsMutable();
accountTags_.set(index, value);
onChanged();
return this;
}
/**
* repeated string account_tags = 8;
*/
public Builder addAccountTags(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountTagsIsMutable();
accountTags_.add(value);
onChanged();
return this;
}
/**
* repeated string account_tags = 8;
*/
public Builder addAllAccountTags(
java.lang.Iterable values) {
ensureAccountTagsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, accountTags_);
onChanged();
return this;
}
/**
* repeated string account_tags = 8;
*/
public Builder clearAccountTags() {
accountTags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
* repeated string account_tags = 8;
*/
public Builder addAccountTagsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureAccountTagsIsMutable();
accountTags_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList businessCategories_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureBusinessCategoriesIsMutable() {
if (!((bitField0_ & 0x00000100) == 0x00000100)) {
businessCategories_ = new com.google.protobuf.LazyStringArrayList(businessCategories_);
bitField0_ |= 0x00000100;
}
}
/**
* repeated string business_categories = 9;
*/
public com.google.protobuf.ProtocolStringList
getBusinessCategoriesList() {
return businessCategories_.getUnmodifiableView();
}
/**
* repeated string business_categories = 9;
*/
public int getBusinessCategoriesCount() {
return businessCategories_.size();
}
/**
* repeated string business_categories = 9;
*/
public java.lang.String getBusinessCategories(int index) {
return businessCategories_.get(index);
}
/**
* repeated string business_categories = 9;
*/
public com.google.protobuf.ByteString
getBusinessCategoriesBytes(int index) {
return businessCategories_.getByteString(index);
}
/**
* repeated string business_categories = 9;
*/
public Builder setBusinessCategories(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureBusinessCategoriesIsMutable();
businessCategories_.set(index, value);
onChanged();
return this;
}
/**
* repeated string business_categories = 9;
*/
public Builder addBusinessCategories(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureBusinessCategoriesIsMutable();
businessCategories_.add(value);
onChanged();
return this;
}
/**
* repeated string business_categories = 9;
*/
public Builder addAllBusinessCategories(
java.lang.Iterable values) {
ensureBusinessCategoriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, businessCategories_);
onChanged();
return this;
}
/**
* repeated string business_categories = 9;
*/
public Builder clearBusinessCategories() {
businessCategories_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
* repeated string business_categories = 9;
*/
public Builder addBusinessCategoriesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureBusinessCategoriesIsMutable();
businessCategories_.add(value);
onChanged();
return this;
}
private int onCampaign_ = 0;
/**
* .sales.v1.Campaign on_campaign = 10;
*/
public int getOnCampaignValue() {
return onCampaign_;
}
/**
* .sales.v1.Campaign on_campaign = 10;
*/
public Builder setOnCampaignValue(int value) {
onCampaign_ = value;
onChanged();
return this;
}
/**
* .sales.v1.Campaign on_campaign = 10;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Campaign getOnCampaign() {
com.vendasta.sales.v1.generated.BusinessProto.Campaign result = com.vendasta.sales.v1.generated.BusinessProto.Campaign.valueOf(onCampaign_);
return result == null ? com.vendasta.sales.v1.generated.BusinessProto.Campaign.UNRECOGNIZED : result;
}
/**
* .sales.v1.Campaign on_campaign = 10;
*/
public Builder setOnCampaign(com.vendasta.sales.v1.generated.BusinessProto.Campaign value) {
if (value == null) {
throw new NullPointerException();
}
onCampaign_ = value.getNumber();
onChanged();
return this;
}
/**
* .sales.v1.Campaign on_campaign = 10;
*/
public Builder clearOnCampaign() {
onCampaign_ = 0;
onChanged();
return this;
}
private int snapshotSent_ = 0;
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
public int getSnapshotSentValue() {
return snapshotSent_;
}
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
public Builder setSnapshotSentValue(int value) {
snapshotSent_ = value;
onChanged();
return this;
}
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
public com.vendasta.sales.v1.generated.BusinessProto.Snapshot getSnapshotSent() {
com.vendasta.sales.v1.generated.BusinessProto.Snapshot result = com.vendasta.sales.v1.generated.BusinessProto.Snapshot.valueOf(snapshotSent_);
return result == null ? com.vendasta.sales.v1.generated.BusinessProto.Snapshot.UNRECOGNIZED : result;
}
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
public Builder setSnapshotSent(com.vendasta.sales.v1.generated.BusinessProto.Snapshot value) {
if (value == null) {
throw new NullPointerException();
}
snapshotSent_ = value.getNumber();
onChanged();
return this;
}
/**
* .sales.v1.Snapshot snapshot_sent = 11;
*/
public Builder clearSnapshotSent() {
snapshotSent_ = 0;
onChanged();
return this;
}
private java.lang.Object query_ = "";
/**
* string query = 12;
*/
public java.lang.String getQuery() {
java.lang.Object ref = query_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
query_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string query = 12;
*/
public com.google.protobuf.ByteString
getQueryBytes() {
java.lang.Object ref = query_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
query_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string query = 12;
*/
public Builder setQuery(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
query_ = value;
onChanged();
return this;
}
/**
* string query = 12;
*/
public Builder clearQuery() {
query_ = getDefaultInstance().getQuery();
onChanged();
return this;
}
/**
* string query = 12;
*/
public Builder setQueryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
query_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList assignees_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureAssigneesIsMutable() {
if (!((bitField0_ & 0x00001000) == 0x00001000)) {
assignees_ = new com.google.protobuf.LazyStringArrayList(assignees_);
bitField0_ |= 0x00001000;
}
}
/**
* repeated string assignees = 13;
*/
public com.google.protobuf.ProtocolStringList
getAssigneesList() {
return assignees_.getUnmodifiableView();
}
/**
* repeated string assignees = 13;
*/
public int getAssigneesCount() {
return assignees_.size();
}
/**
* repeated string assignees = 13;
*/
public java.lang.String getAssignees(int index) {
return assignees_.get(index);
}
/**
* repeated string assignees = 13;
*/
public com.google.protobuf.ByteString
getAssigneesBytes(int index) {
return assignees_.getByteString(index);
}
/**
* repeated string assignees = 13;
*/
public Builder setAssignees(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAssigneesIsMutable();
assignees_.set(index, value);
onChanged();
return this;
}
/**
* repeated string assignees = 13;
*/
public Builder addAssignees(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAssigneesIsMutable();
assignees_.add(value);
onChanged();
return this;
}
/**
* repeated string assignees = 13;
*/
public Builder addAllAssignees(
java.lang.Iterable values) {
ensureAssigneesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, assignees_);
onChanged();
return this;
}
/**
* repeated string assignees = 13;
*/
public Builder clearAssignees() {
assignees_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
* repeated string assignees = 13;
*/
public Builder addAssigneesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureAssigneesIsMutable();
assignees_.add(value);
onChanged();
return this;
}
private java.lang.Object goal_ = "";
/**
* string goal = 14;
*/
public java.lang.String getGoal() {
java.lang.Object ref = goal_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
goal_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string goal = 14;
*/
public com.google.protobuf.ByteString
getGoalBytes() {
java.lang.Object ref = goal_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
goal_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string goal = 14;
*/
public Builder setGoal(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
goal_ = value;
onChanged();
return this;
}
/**
* string goal = 14;
*/
public Builder clearGoal() {
goal_ = getDefaultInstance().getGoal();
onChanged();
return this;
}
/**
* string goal = 14;
*/
public Builder setGoalBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
goal_ = value;
onChanged();
return this;
}
private java.lang.Object trainingPriorities_ = "";
/**
* string training_priorities = 15;
*/
public java.lang.String getTrainingPriorities() {
java.lang.Object ref = trainingPriorities_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
trainingPriorities_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string training_priorities = 15;
*/
public com.google.protobuf.ByteString
getTrainingPrioritiesBytes() {
java.lang.Object ref = trainingPriorities_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
trainingPriorities_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string training_priorities = 15;
*/
public Builder setTrainingPriorities(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
trainingPriorities_ = value;
onChanged();
return this;
}
/**
* string training_priorities = 15;
*/
public Builder clearTrainingPriorities() {
trainingPriorities_ = getDefaultInstance().getTrainingPriorities();
onChanged();
return this;
}
/**
* string training_priorities = 15;
*/
public Builder setTrainingPrioritiesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
trainingPriorities_ = value;
onChanged();
return this;
}
private java.lang.Object customFieldPartnerId_ = "";
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
public java.lang.String getCustomFieldPartnerId() {
java.lang.Object ref = customFieldPartnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customFieldPartnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
public com.google.protobuf.ByteString
getCustomFieldPartnerIdBytes() {
java.lang.Object ref = customFieldPartnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customFieldPartnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
public Builder setCustomFieldPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
customFieldPartnerId_ = value;
onChanged();
return this;
}
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
public Builder clearCustomFieldPartnerId() {
customFieldPartnerId_ = getDefaultInstance().getCustomFieldPartnerId();
onChanged();
return this;
}
/**
*
* Special case to allow a must query on the custom field partner id (only used by VMF currently)
*
*
* string custom_field_partner_id = 16;
*/
public Builder setCustomFieldPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
customFieldPartnerId_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.BusinessSearchFilters)
}
// @@protoc_insertion_point(class_scope:sales.v1.BusinessSearchFilters)
private static final com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters();
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public BusinessSearchFilters parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BusinessSearchFilters(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchFilters getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BusinessSearchSortOptionsOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.BusinessSearchSortOptions)
com.google.protobuf.MessageOrBuilder {
/**
* string sort_key = 1;
*/
java.lang.String getSortKey();
/**
* string sort_key = 1;
*/
com.google.protobuf.ByteString
getSortKeyBytes();
/**
* bool sort_ascending = 2;
*/
boolean getSortAscending();
}
/**
* Protobuf type {@code sales.v1.BusinessSearchSortOptions}
*/
public static final class BusinessSearchSortOptions extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.BusinessSearchSortOptions)
BusinessSearchSortOptionsOrBuilder {
// Use BusinessSearchSortOptions.newBuilder() to construct.
private BusinessSearchSortOptions(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BusinessSearchSortOptions() {
sortKey_ = "";
sortAscending_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private BusinessSearchSortOptions(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
sortKey_ = s;
break;
}
case 16: {
sortAscending_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchSortOptions_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchSortOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.class, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.Builder.class);
}
public static final int SORT_KEY_FIELD_NUMBER = 1;
private volatile java.lang.Object sortKey_;
/**
* string sort_key = 1;
*/
public java.lang.String getSortKey() {
java.lang.Object ref = sortKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sortKey_ = s;
return s;
}
}
/**
* string sort_key = 1;
*/
public com.google.protobuf.ByteString
getSortKeyBytes() {
java.lang.Object ref = sortKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sortKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SORT_ASCENDING_FIELD_NUMBER = 2;
private boolean sortAscending_;
/**
* bool sort_ascending = 2;
*/
public boolean getSortAscending() {
return sortAscending_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getSortKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, sortKey_);
}
if (sortAscending_ != false) {
output.writeBool(2, sortAscending_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getSortKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, sortKey_);
}
if (sortAscending_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, sortAscending_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions other = (com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions) obj;
boolean result = true;
result = result && getSortKey()
.equals(other.getSortKey());
result = result && (getSortAscending()
== other.getSortAscending());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SORT_KEY_FIELD_NUMBER;
hash = (53 * hash) + getSortKey().hashCode();
hash = (37 * hash) + SORT_ASCENDING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSortAscending());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.BusinessSearchSortOptions}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.BusinessSearchSortOptions)
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptionsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchSortOptions_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchSortOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.class, com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
sortKey_ = "";
sortAscending_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_BusinessSearchSortOptions_descriptor;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions build() {
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions buildPartial() {
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions result = new com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions(this);
result.sortKey_ = sortKey_;
result.sortAscending_ = sortAscending_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions) {
return mergeFrom((com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions other) {
if (other == com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions.getDefaultInstance()) return this;
if (!other.getSortKey().isEmpty()) {
sortKey_ = other.sortKey_;
onChanged();
}
if (other.getSortAscending() != false) {
setSortAscending(other.getSortAscending());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object sortKey_ = "";
/**
* string sort_key = 1;
*/
public java.lang.String getSortKey() {
java.lang.Object ref = sortKey_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sortKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sort_key = 1;
*/
public com.google.protobuf.ByteString
getSortKeyBytes() {
java.lang.Object ref = sortKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sortKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sort_key = 1;
*/
public Builder setSortKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sortKey_ = value;
onChanged();
return this;
}
/**
* string sort_key = 1;
*/
public Builder clearSortKey() {
sortKey_ = getDefaultInstance().getSortKey();
onChanged();
return this;
}
/**
* string sort_key = 1;
*/
public Builder setSortKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sortKey_ = value;
onChanged();
return this;
}
private boolean sortAscending_ ;
/**
* bool sort_ascending = 2;
*/
public boolean getSortAscending() {
return sortAscending_;
}
/**
* bool sort_ascending = 2;
*/
public Builder setSortAscending(boolean value) {
sortAscending_ = value;
onChanged();
return this;
}
/**
* bool sort_ascending = 2;
*/
public Builder clearSortAscending() {
sortAscending_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.BusinessSearchSortOptions)
}
// @@protoc_insertion_point(class_scope:sales.v1.BusinessSearchSortOptions)
private static final com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions();
}
public static com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public BusinessSearchSortOptions parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BusinessSearchSortOptions(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.BusinessProto.BusinessSearchSortOptions getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BusinessOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.Business)
com.google.protobuf.MessageOrBuilder {
/**
* string partner_id = 1;
*/
java.lang.String getPartnerId();
/**
* string partner_id = 1;
*/
com.google.protobuf.ByteString
getPartnerIdBytes();
/**
* string market_id = 2;
*/
java.lang.String getMarketId();
/**
* string market_id = 2;
*/
com.google.protobuf.ByteString
getMarketIdBytes();
/**
* string name = 3;
*/
java.lang.String getName();
/**
* string name = 3;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string account_group_id = 4;
*/
java.lang.String getAccountGroupId();
/**
* string account_group_id = 4;
*/
com.google.protobuf.ByteString
getAccountGroupIdBytes();
/**
* int64 hotness = 5;
*/
long getHotness();
/**
* string city = 6;
*/
java.lang.String getCity();
/**
* string city = 6;
*/
com.google.protobuf.ByteString
getCityBytes();
/**
* string zip = 7;
*/
java.lang.String getZip();
/**
* string zip = 7;
*/
com.google.protobuf.ByteString
getZipBytes();
/**
* string address = 8;
*/
java.lang.String getAddress();
/**
* string address = 8;
*/
com.google.protobuf.ByteString
getAddressBytes();
/**
* string state = 9;
*/
java.lang.String getState();
/**
* string state = 9;
*/
com.google.protobuf.ByteString
getStateBytes();
/**
* string country = 10;
*/
java.lang.String getCountry();
/**
* string country = 10;
*/
com.google.protobuf.ByteString
getCountryBytes();
/**
* string phone_number = 11;
*/
java.lang.String getPhoneNumber();
/**
* string phone_number = 11;
*/
com.google.protobuf.ByteString
getPhoneNumberBytes();
/**
* string salesperson_id = 12;
*/
java.lang.String getSalespersonId();
/**
* string salesperson_id = 12;
*/
com.google.protobuf.ByteString
getSalespersonIdBytes();
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
boolean hasLastSalesActivityDate();
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
com.google.protobuf.Timestamp getLastSalesActivityDate();
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
com.google.protobuf.TimestampOrBuilder getLastSalesActivityDateOrBuilder();
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
boolean hasLastCustomerActivityDate();
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
com.google.protobuf.Timestamp getLastCustomerActivityDate();
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
com.google.protobuf.TimestampOrBuilder getLastCustomerActivityDateOrBuilder();
/**
* string sales_status = 15;
*/
java.lang.String getSalesStatus();
/**
* string sales_status = 15;
*/
com.google.protobuf.ByteString
getSalesStatusBytes();
/**
* bool is_read = 16;
*/
boolean getIsRead();
/**
* string sales_person_action = 17;
*/
java.lang.String getSalesPersonAction();
/**
* string sales_person_action = 17;
*/
com.google.protobuf.ByteString
getSalesPersonActionBytes();
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
boolean hasLatestSnapshotExpiry();
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
com.google.protobuf.Timestamp getLatestSnapshotExpiry();
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
com.google.protobuf.TimestampOrBuilder getLatestSnapshotExpiryOrBuilder();
/**
* bool snapshot_or_campaign_email_status = 19;
*/
boolean getSnapshotOrCampaignEmailStatus();
/**
* string activity_type = 20;
*/
java.lang.String getActivityType();
/**
* string activity_type = 20;
*/
com.google.protobuf.ByteString
getActivityTypeBytes();
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
boolean hasLastConnectedDate();
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
com.google.protobuf.Timestamp getLastConnectedDate();
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
com.google.protobuf.TimestampOrBuilder getLastConnectedDateOrBuilder();
}
/**
* Protobuf type {@code sales.v1.Business}
*/
public static final class Business extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.Business)
BusinessOrBuilder {
// Use Business.newBuilder() to construct.
private Business(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Business() {
partnerId_ = "";
marketId_ = "";
name_ = "";
accountGroupId_ = "";
hotness_ = 0L;
city_ = "";
zip_ = "";
address_ = "";
state_ = "";
country_ = "";
phoneNumber_ = "";
salespersonId_ = "";
salesStatus_ = "";
isRead_ = false;
salesPersonAction_ = "";
snapshotOrCampaignEmailStatus_ = false;
activityType_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Business(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
partnerId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
marketId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
accountGroupId_ = s;
break;
}
case 40: {
hotness_ = input.readInt64();
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
city_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
zip_ = s;
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
address_ = s;
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
state_ = s;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
country_ = s;
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
phoneNumber_ = s;
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
salespersonId_ = s;
break;
}
case 106: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (lastSalesActivityDate_ != null) {
subBuilder = lastSalesActivityDate_.toBuilder();
}
lastSalesActivityDate_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(lastSalesActivityDate_);
lastSalesActivityDate_ = subBuilder.buildPartial();
}
break;
}
case 114: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (lastCustomerActivityDate_ != null) {
subBuilder = lastCustomerActivityDate_.toBuilder();
}
lastCustomerActivityDate_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(lastCustomerActivityDate_);
lastCustomerActivityDate_ = subBuilder.buildPartial();
}
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
salesStatus_ = s;
break;
}
case 128: {
isRead_ = input.readBool();
break;
}
case 138: {
java.lang.String s = input.readStringRequireUtf8();
salesPersonAction_ = s;
break;
}
case 146: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (latestSnapshotExpiry_ != null) {
subBuilder = latestSnapshotExpiry_.toBuilder();
}
latestSnapshotExpiry_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(latestSnapshotExpiry_);
latestSnapshotExpiry_ = subBuilder.buildPartial();
}
break;
}
case 152: {
snapshotOrCampaignEmailStatus_ = input.readBool();
break;
}
case 162: {
java.lang.String s = input.readStringRequireUtf8();
activityType_ = s;
break;
}
case 170: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (lastConnectedDate_ != null) {
subBuilder = lastConnectedDate_.toBuilder();
}
lastConnectedDate_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(lastConnectedDate_);
lastConnectedDate_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_Business_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_Business_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.BusinessProto.Business.class, com.vendasta.sales.v1.generated.BusinessProto.Business.Builder.class);
}
public static final int PARTNER_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object partnerId_;
/**
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
}
}
/**
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MARKET_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object marketId_;
/**
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
}
}
/**
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object name_;
/**
* string name = 3;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 3;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_GROUP_ID_FIELD_NUMBER = 4;
private volatile java.lang.Object accountGroupId_;
/**
* string account_group_id = 4;
*/
public java.lang.String getAccountGroupId() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountGroupId_ = s;
return s;
}
}
/**
* string account_group_id = 4;
*/
public com.google.protobuf.ByteString
getAccountGroupIdBytes() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountGroupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HOTNESS_FIELD_NUMBER = 5;
private long hotness_;
/**
* int64 hotness = 5;
*/
public long getHotness() {
return hotness_;
}
public static final int CITY_FIELD_NUMBER = 6;
private volatile java.lang.Object city_;
/**
* string city = 6;
*/
public java.lang.String getCity() {
java.lang.Object ref = city_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
city_ = s;
return s;
}
}
/**
* string city = 6;
*/
public com.google.protobuf.ByteString
getCityBytes() {
java.lang.Object ref = city_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
city_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ZIP_FIELD_NUMBER = 7;
private volatile java.lang.Object zip_;
/**
* string zip = 7;
*/
public java.lang.String getZip() {
java.lang.Object ref = zip_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
zip_ = s;
return s;
}
}
/**
* string zip = 7;
*/
public com.google.protobuf.ByteString
getZipBytes() {
java.lang.Object ref = zip_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
zip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADDRESS_FIELD_NUMBER = 8;
private volatile java.lang.Object address_;
/**
* string address = 8;
*/
public java.lang.String getAddress() {
java.lang.Object ref = address_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
address_ = s;
return s;
}
}
/**
* string address = 8;
*/
public com.google.protobuf.ByteString
getAddressBytes() {
java.lang.Object ref = address_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATE_FIELD_NUMBER = 9;
private volatile java.lang.Object state_;
/**
* string state = 9;
*/
public java.lang.String getState() {
java.lang.Object ref = state_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
state_ = s;
return s;
}
}
/**
* string state = 9;
*/
public com.google.protobuf.ByteString
getStateBytes() {
java.lang.Object ref = state_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
state_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COUNTRY_FIELD_NUMBER = 10;
private volatile java.lang.Object country_;
/**
* string country = 10;
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
country_ = s;
return s;
}
}
/**
* string country = 10;
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PHONE_NUMBER_FIELD_NUMBER = 11;
private volatile java.lang.Object phoneNumber_;
/**
* string phone_number = 11;
*/
public java.lang.String getPhoneNumber() {
java.lang.Object ref = phoneNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
phoneNumber_ = s;
return s;
}
}
/**
* string phone_number = 11;
*/
public com.google.protobuf.ByteString
getPhoneNumberBytes() {
java.lang.Object ref = phoneNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
phoneNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SALESPERSON_ID_FIELD_NUMBER = 12;
private volatile java.lang.Object salespersonId_;
/**
* string salesperson_id = 12;
*/
public java.lang.String getSalespersonId() {
java.lang.Object ref = salespersonId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
salespersonId_ = s;
return s;
}
}
/**
* string salesperson_id = 12;
*/
public com.google.protobuf.ByteString
getSalespersonIdBytes() {
java.lang.Object ref = salespersonId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
salespersonId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LAST_SALES_ACTIVITY_DATE_FIELD_NUMBER = 13;
private com.google.protobuf.Timestamp lastSalesActivityDate_;
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public boolean hasLastSalesActivityDate() {
return lastSalesActivityDate_ != null;
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public com.google.protobuf.Timestamp getLastSalesActivityDate() {
return lastSalesActivityDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastSalesActivityDate_;
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public com.google.protobuf.TimestampOrBuilder getLastSalesActivityDateOrBuilder() {
return getLastSalesActivityDate();
}
public static final int LAST_CUSTOMER_ACTIVITY_DATE_FIELD_NUMBER = 14;
private com.google.protobuf.Timestamp lastCustomerActivityDate_;
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public boolean hasLastCustomerActivityDate() {
return lastCustomerActivityDate_ != null;
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public com.google.protobuf.Timestamp getLastCustomerActivityDate() {
return lastCustomerActivityDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastCustomerActivityDate_;
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public com.google.protobuf.TimestampOrBuilder getLastCustomerActivityDateOrBuilder() {
return getLastCustomerActivityDate();
}
public static final int SALES_STATUS_FIELD_NUMBER = 15;
private volatile java.lang.Object salesStatus_;
/**
* string sales_status = 15;
*/
public java.lang.String getSalesStatus() {
java.lang.Object ref = salesStatus_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
salesStatus_ = s;
return s;
}
}
/**
* string sales_status = 15;
*/
public com.google.protobuf.ByteString
getSalesStatusBytes() {
java.lang.Object ref = salesStatus_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
salesStatus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IS_READ_FIELD_NUMBER = 16;
private boolean isRead_;
/**
* bool is_read = 16;
*/
public boolean getIsRead() {
return isRead_;
}
public static final int SALES_PERSON_ACTION_FIELD_NUMBER = 17;
private volatile java.lang.Object salesPersonAction_;
/**
* string sales_person_action = 17;
*/
public java.lang.String getSalesPersonAction() {
java.lang.Object ref = salesPersonAction_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
salesPersonAction_ = s;
return s;
}
}
/**
* string sales_person_action = 17;
*/
public com.google.protobuf.ByteString
getSalesPersonActionBytes() {
java.lang.Object ref = salesPersonAction_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
salesPersonAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LATEST_SNAPSHOT_EXPIRY_FIELD_NUMBER = 18;
private com.google.protobuf.Timestamp latestSnapshotExpiry_;
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public boolean hasLatestSnapshotExpiry() {
return latestSnapshotExpiry_ != null;
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public com.google.protobuf.Timestamp getLatestSnapshotExpiry() {
return latestSnapshotExpiry_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : latestSnapshotExpiry_;
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public com.google.protobuf.TimestampOrBuilder getLatestSnapshotExpiryOrBuilder() {
return getLatestSnapshotExpiry();
}
public static final int SNAPSHOT_OR_CAMPAIGN_EMAIL_STATUS_FIELD_NUMBER = 19;
private boolean snapshotOrCampaignEmailStatus_;
/**
* bool snapshot_or_campaign_email_status = 19;
*/
public boolean getSnapshotOrCampaignEmailStatus() {
return snapshotOrCampaignEmailStatus_;
}
public static final int ACTIVITY_TYPE_FIELD_NUMBER = 20;
private volatile java.lang.Object activityType_;
/**
* string activity_type = 20;
*/
public java.lang.String getActivityType() {
java.lang.Object ref = activityType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
activityType_ = s;
return s;
}
}
/**
* string activity_type = 20;
*/
public com.google.protobuf.ByteString
getActivityTypeBytes() {
java.lang.Object ref = activityType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
activityType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LAST_CONNECTED_DATE_FIELD_NUMBER = 21;
private com.google.protobuf.Timestamp lastConnectedDate_;
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public boolean hasLastConnectedDate() {
return lastConnectedDate_ != null;
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public com.google.protobuf.Timestamp getLastConnectedDate() {
return lastConnectedDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastConnectedDate_;
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public com.google.protobuf.TimestampOrBuilder getLastConnectedDateOrBuilder() {
return getLastConnectedDate();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, marketId_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, name_);
}
if (!getAccountGroupIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, accountGroupId_);
}
if (hotness_ != 0L) {
output.writeInt64(5, hotness_);
}
if (!getCityBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, city_);
}
if (!getZipBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, zip_);
}
if (!getAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, address_);
}
if (!getStateBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, state_);
}
if (!getCountryBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, country_);
}
if (!getPhoneNumberBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, phoneNumber_);
}
if (!getSalespersonIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, salespersonId_);
}
if (lastSalesActivityDate_ != null) {
output.writeMessage(13, getLastSalesActivityDate());
}
if (lastCustomerActivityDate_ != null) {
output.writeMessage(14, getLastCustomerActivityDate());
}
if (!getSalesStatusBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, salesStatus_);
}
if (isRead_ != false) {
output.writeBool(16, isRead_);
}
if (!getSalesPersonActionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, salesPersonAction_);
}
if (latestSnapshotExpiry_ != null) {
output.writeMessage(18, getLatestSnapshotExpiry());
}
if (snapshotOrCampaignEmailStatus_ != false) {
output.writeBool(19, snapshotOrCampaignEmailStatus_);
}
if (!getActivityTypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, activityType_);
}
if (lastConnectedDate_ != null) {
output.writeMessage(21, getLastConnectedDate());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, partnerId_);
}
if (!getMarketIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, marketId_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, name_);
}
if (!getAccountGroupIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, accountGroupId_);
}
if (hotness_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, hotness_);
}
if (!getCityBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, city_);
}
if (!getZipBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, zip_);
}
if (!getAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, address_);
}
if (!getStateBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, state_);
}
if (!getCountryBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, country_);
}
if (!getPhoneNumberBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, phoneNumber_);
}
if (!getSalespersonIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, salespersonId_);
}
if (lastSalesActivityDate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getLastSalesActivityDate());
}
if (lastCustomerActivityDate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getLastCustomerActivityDate());
}
if (!getSalesStatusBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, salesStatus_);
}
if (isRead_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, isRead_);
}
if (!getSalesPersonActionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, salesPersonAction_);
}
if (latestSnapshotExpiry_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, getLatestSnapshotExpiry());
}
if (snapshotOrCampaignEmailStatus_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(19, snapshotOrCampaignEmailStatus_);
}
if (!getActivityTypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, activityType_);
}
if (lastConnectedDate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, getLastConnectedDate());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.BusinessProto.Business)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.BusinessProto.Business other = (com.vendasta.sales.v1.generated.BusinessProto.Business) obj;
boolean result = true;
result = result && getPartnerId()
.equals(other.getPartnerId());
result = result && getMarketId()
.equals(other.getMarketId());
result = result && getName()
.equals(other.getName());
result = result && getAccountGroupId()
.equals(other.getAccountGroupId());
result = result && (getHotness()
== other.getHotness());
result = result && getCity()
.equals(other.getCity());
result = result && getZip()
.equals(other.getZip());
result = result && getAddress()
.equals(other.getAddress());
result = result && getState()
.equals(other.getState());
result = result && getCountry()
.equals(other.getCountry());
result = result && getPhoneNumber()
.equals(other.getPhoneNumber());
result = result && getSalespersonId()
.equals(other.getSalespersonId());
result = result && (hasLastSalesActivityDate() == other.hasLastSalesActivityDate());
if (hasLastSalesActivityDate()) {
result = result && getLastSalesActivityDate()
.equals(other.getLastSalesActivityDate());
}
result = result && (hasLastCustomerActivityDate() == other.hasLastCustomerActivityDate());
if (hasLastCustomerActivityDate()) {
result = result && getLastCustomerActivityDate()
.equals(other.getLastCustomerActivityDate());
}
result = result && getSalesStatus()
.equals(other.getSalesStatus());
result = result && (getIsRead()
== other.getIsRead());
result = result && getSalesPersonAction()
.equals(other.getSalesPersonAction());
result = result && (hasLatestSnapshotExpiry() == other.hasLatestSnapshotExpiry());
if (hasLatestSnapshotExpiry()) {
result = result && getLatestSnapshotExpiry()
.equals(other.getLatestSnapshotExpiry());
}
result = result && (getSnapshotOrCampaignEmailStatus()
== other.getSnapshotOrCampaignEmailStatus());
result = result && getActivityType()
.equals(other.getActivityType());
result = result && (hasLastConnectedDate() == other.hasLastConnectedDate());
if (hasLastConnectedDate()) {
result = result && getLastConnectedDate()
.equals(other.getLastConnectedDate());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartnerId().hashCode();
hash = (37 * hash) + MARKET_ID_FIELD_NUMBER;
hash = (53 * hash) + getMarketId().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + ACCOUNT_GROUP_ID_FIELD_NUMBER;
hash = (53 * hash) + getAccountGroupId().hashCode();
hash = (37 * hash) + HOTNESS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getHotness());
hash = (37 * hash) + CITY_FIELD_NUMBER;
hash = (53 * hash) + getCity().hashCode();
hash = (37 * hash) + ZIP_FIELD_NUMBER;
hash = (53 * hash) + getZip().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + getState().hashCode();
hash = (37 * hash) + COUNTRY_FIELD_NUMBER;
hash = (53 * hash) + getCountry().hashCode();
hash = (37 * hash) + PHONE_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getPhoneNumber().hashCode();
hash = (37 * hash) + SALESPERSON_ID_FIELD_NUMBER;
hash = (53 * hash) + getSalespersonId().hashCode();
if (hasLastSalesActivityDate()) {
hash = (37 * hash) + LAST_SALES_ACTIVITY_DATE_FIELD_NUMBER;
hash = (53 * hash) + getLastSalesActivityDate().hashCode();
}
if (hasLastCustomerActivityDate()) {
hash = (37 * hash) + LAST_CUSTOMER_ACTIVITY_DATE_FIELD_NUMBER;
hash = (53 * hash) + getLastCustomerActivityDate().hashCode();
}
hash = (37 * hash) + SALES_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getSalesStatus().hashCode();
hash = (37 * hash) + IS_READ_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsRead());
hash = (37 * hash) + SALES_PERSON_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getSalesPersonAction().hashCode();
if (hasLatestSnapshotExpiry()) {
hash = (37 * hash) + LATEST_SNAPSHOT_EXPIRY_FIELD_NUMBER;
hash = (53 * hash) + getLatestSnapshotExpiry().hashCode();
}
hash = (37 * hash) + SNAPSHOT_OR_CAMPAIGN_EMAIL_STATUS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSnapshotOrCampaignEmailStatus());
hash = (37 * hash) + ACTIVITY_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getActivityType().hashCode();
if (hasLastConnectedDate()) {
hash = (37 * hash) + LAST_CONNECTED_DATE_FIELD_NUMBER;
hash = (53 * hash) + getLastConnectedDate().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.BusinessProto.Business prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.Business}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.Business)
com.vendasta.sales.v1.generated.BusinessProto.BusinessOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_Business_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_Business_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.BusinessProto.Business.class, com.vendasta.sales.v1.generated.BusinessProto.Business.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.BusinessProto.Business.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
partnerId_ = "";
marketId_ = "";
name_ = "";
accountGroupId_ = "";
hotness_ = 0L;
city_ = "";
zip_ = "";
address_ = "";
state_ = "";
country_ = "";
phoneNumber_ = "";
salespersonId_ = "";
if (lastSalesActivityDateBuilder_ == null) {
lastSalesActivityDate_ = null;
} else {
lastSalesActivityDate_ = null;
lastSalesActivityDateBuilder_ = null;
}
if (lastCustomerActivityDateBuilder_ == null) {
lastCustomerActivityDate_ = null;
} else {
lastCustomerActivityDate_ = null;
lastCustomerActivityDateBuilder_ = null;
}
salesStatus_ = "";
isRead_ = false;
salesPersonAction_ = "";
if (latestSnapshotExpiryBuilder_ == null) {
latestSnapshotExpiry_ = null;
} else {
latestSnapshotExpiry_ = null;
latestSnapshotExpiryBuilder_ = null;
}
snapshotOrCampaignEmailStatus_ = false;
activityType_ = "";
if (lastConnectedDateBuilder_ == null) {
lastConnectedDate_ = null;
} else {
lastConnectedDate_ = null;
lastConnectedDateBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.BusinessProto.internal_static_sales_v1_Business_descriptor;
}
public com.vendasta.sales.v1.generated.BusinessProto.Business getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.BusinessProto.Business.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.BusinessProto.Business build() {
com.vendasta.sales.v1.generated.BusinessProto.Business result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.BusinessProto.Business buildPartial() {
com.vendasta.sales.v1.generated.BusinessProto.Business result = new com.vendasta.sales.v1.generated.BusinessProto.Business(this);
result.partnerId_ = partnerId_;
result.marketId_ = marketId_;
result.name_ = name_;
result.accountGroupId_ = accountGroupId_;
result.hotness_ = hotness_;
result.city_ = city_;
result.zip_ = zip_;
result.address_ = address_;
result.state_ = state_;
result.country_ = country_;
result.phoneNumber_ = phoneNumber_;
result.salespersonId_ = salespersonId_;
if (lastSalesActivityDateBuilder_ == null) {
result.lastSalesActivityDate_ = lastSalesActivityDate_;
} else {
result.lastSalesActivityDate_ = lastSalesActivityDateBuilder_.build();
}
if (lastCustomerActivityDateBuilder_ == null) {
result.lastCustomerActivityDate_ = lastCustomerActivityDate_;
} else {
result.lastCustomerActivityDate_ = lastCustomerActivityDateBuilder_.build();
}
result.salesStatus_ = salesStatus_;
result.isRead_ = isRead_;
result.salesPersonAction_ = salesPersonAction_;
if (latestSnapshotExpiryBuilder_ == null) {
result.latestSnapshotExpiry_ = latestSnapshotExpiry_;
} else {
result.latestSnapshotExpiry_ = latestSnapshotExpiryBuilder_.build();
}
result.snapshotOrCampaignEmailStatus_ = snapshotOrCampaignEmailStatus_;
result.activityType_ = activityType_;
if (lastConnectedDateBuilder_ == null) {
result.lastConnectedDate_ = lastConnectedDate_;
} else {
result.lastConnectedDate_ = lastConnectedDateBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.BusinessProto.Business) {
return mergeFrom((com.vendasta.sales.v1.generated.BusinessProto.Business)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.BusinessProto.Business other) {
if (other == com.vendasta.sales.v1.generated.BusinessProto.Business.getDefaultInstance()) return this;
if (!other.getPartnerId().isEmpty()) {
partnerId_ = other.partnerId_;
onChanged();
}
if (!other.getMarketId().isEmpty()) {
marketId_ = other.marketId_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getAccountGroupId().isEmpty()) {
accountGroupId_ = other.accountGroupId_;
onChanged();
}
if (other.getHotness() != 0L) {
setHotness(other.getHotness());
}
if (!other.getCity().isEmpty()) {
city_ = other.city_;
onChanged();
}
if (!other.getZip().isEmpty()) {
zip_ = other.zip_;
onChanged();
}
if (!other.getAddress().isEmpty()) {
address_ = other.address_;
onChanged();
}
if (!other.getState().isEmpty()) {
state_ = other.state_;
onChanged();
}
if (!other.getCountry().isEmpty()) {
country_ = other.country_;
onChanged();
}
if (!other.getPhoneNumber().isEmpty()) {
phoneNumber_ = other.phoneNumber_;
onChanged();
}
if (!other.getSalespersonId().isEmpty()) {
salespersonId_ = other.salespersonId_;
onChanged();
}
if (other.hasLastSalesActivityDate()) {
mergeLastSalesActivityDate(other.getLastSalesActivityDate());
}
if (other.hasLastCustomerActivityDate()) {
mergeLastCustomerActivityDate(other.getLastCustomerActivityDate());
}
if (!other.getSalesStatus().isEmpty()) {
salesStatus_ = other.salesStatus_;
onChanged();
}
if (other.getIsRead() != false) {
setIsRead(other.getIsRead());
}
if (!other.getSalesPersonAction().isEmpty()) {
salesPersonAction_ = other.salesPersonAction_;
onChanged();
}
if (other.hasLatestSnapshotExpiry()) {
mergeLatestSnapshotExpiry(other.getLatestSnapshotExpiry());
}
if (other.getSnapshotOrCampaignEmailStatus() != false) {
setSnapshotOrCampaignEmailStatus(other.getSnapshotOrCampaignEmailStatus());
}
if (!other.getActivityType().isEmpty()) {
activityType_ = other.activityType_;
onChanged();
}
if (other.hasLastConnectedDate()) {
mergeLastConnectedDate(other.getLastConnectedDate());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.BusinessProto.Business parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.BusinessProto.Business) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object partnerId_ = "";
/**
* string partner_id = 1;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string partner_id = 1;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string partner_id = 1;
*/
public Builder setPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partnerId_ = value;
onChanged();
return this;
}
/**
* string partner_id = 1;
*/
public Builder clearPartnerId() {
partnerId_ = getDefaultInstance().getPartnerId();
onChanged();
return this;
}
/**
* string partner_id = 1;
*/
public Builder setPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partnerId_ = value;
onChanged();
return this;
}
private java.lang.Object marketId_ = "";
/**
* string market_id = 2;
*/
public java.lang.String getMarketId() {
java.lang.Object ref = marketId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string market_id = 2;
*/
public com.google.protobuf.ByteString
getMarketIdBytes() {
java.lang.Object ref = marketId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string market_id = 2;
*/
public Builder setMarketId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
marketId_ = value;
onChanged();
return this;
}
/**
* string market_id = 2;
*/
public Builder clearMarketId() {
marketId_ = getDefaultInstance().getMarketId();
onChanged();
return this;
}
/**
* string market_id = 2;
*/
public Builder setMarketIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
marketId_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 3;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 3;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 3;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 3;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 3;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object accountGroupId_ = "";
/**
* string account_group_id = 4;
*/
public java.lang.String getAccountGroupId() {
java.lang.Object ref = accountGroupId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountGroupId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string account_group_id = 4;
*/
public com.google.protobuf.ByteString
getAccountGroupIdBytes() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountGroupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string account_group_id = 4;
*/
public Builder setAccountGroupId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accountGroupId_ = value;
onChanged();
return this;
}
/**
* string account_group_id = 4;
*/
public Builder clearAccountGroupId() {
accountGroupId_ = getDefaultInstance().getAccountGroupId();
onChanged();
return this;
}
/**
* string account_group_id = 4;
*/
public Builder setAccountGroupIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accountGroupId_ = value;
onChanged();
return this;
}
private long hotness_ ;
/**
* int64 hotness = 5;
*/
public long getHotness() {
return hotness_;
}
/**
* int64 hotness = 5;
*/
public Builder setHotness(long value) {
hotness_ = value;
onChanged();
return this;
}
/**
* int64 hotness = 5;
*/
public Builder clearHotness() {
hotness_ = 0L;
onChanged();
return this;
}
private java.lang.Object city_ = "";
/**
* string city = 6;
*/
public java.lang.String getCity() {
java.lang.Object ref = city_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
city_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string city = 6;
*/
public com.google.protobuf.ByteString
getCityBytes() {
java.lang.Object ref = city_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
city_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string city = 6;
*/
public Builder setCity(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
city_ = value;
onChanged();
return this;
}
/**
* string city = 6;
*/
public Builder clearCity() {
city_ = getDefaultInstance().getCity();
onChanged();
return this;
}
/**
* string city = 6;
*/
public Builder setCityBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
city_ = value;
onChanged();
return this;
}
private java.lang.Object zip_ = "";
/**
* string zip = 7;
*/
public java.lang.String getZip() {
java.lang.Object ref = zip_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
zip_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string zip = 7;
*/
public com.google.protobuf.ByteString
getZipBytes() {
java.lang.Object ref = zip_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
zip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string zip = 7;
*/
public Builder setZip(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
zip_ = value;
onChanged();
return this;
}
/**
* string zip = 7;
*/
public Builder clearZip() {
zip_ = getDefaultInstance().getZip();
onChanged();
return this;
}
/**
* string zip = 7;
*/
public Builder setZipBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
zip_ = value;
onChanged();
return this;
}
private java.lang.Object address_ = "";
/**
* string address = 8;
*/
public java.lang.String getAddress() {
java.lang.Object ref = address_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
address_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string address = 8;
*/
public com.google.protobuf.ByteString
getAddressBytes() {
java.lang.Object ref = address_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string address = 8;
*/
public Builder setAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
address_ = value;
onChanged();
return this;
}
/**
* string address = 8;
*/
public Builder clearAddress() {
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
/**
* string address = 8;
*/
public Builder setAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
address_ = value;
onChanged();
return this;
}
private java.lang.Object state_ = "";
/**
* string state = 9;
*/
public java.lang.String getState() {
java.lang.Object ref = state_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
state_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string state = 9;
*/
public com.google.protobuf.ByteString
getStateBytes() {
java.lang.Object ref = state_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
state_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string state = 9;
*/
public Builder setState(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
state_ = value;
onChanged();
return this;
}
/**
* string state = 9;
*/
public Builder clearState() {
state_ = getDefaultInstance().getState();
onChanged();
return this;
}
/**
* string state = 9;
*/
public Builder setStateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
state_ = value;
onChanged();
return this;
}
private java.lang.Object country_ = "";
/**
* string country = 10;
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
country_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string country = 10;
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string country = 10;
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
country_ = value;
onChanged();
return this;
}
/**
* string country = 10;
*/
public Builder clearCountry() {
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* string country = 10;
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
country_ = value;
onChanged();
return this;
}
private java.lang.Object phoneNumber_ = "";
/**
* string phone_number = 11;
*/
public java.lang.String getPhoneNumber() {
java.lang.Object ref = phoneNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
phoneNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string phone_number = 11;
*/
public com.google.protobuf.ByteString
getPhoneNumberBytes() {
java.lang.Object ref = phoneNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
phoneNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string phone_number = 11;
*/
public Builder setPhoneNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
phoneNumber_ = value;
onChanged();
return this;
}
/**
* string phone_number = 11;
*/
public Builder clearPhoneNumber() {
phoneNumber_ = getDefaultInstance().getPhoneNumber();
onChanged();
return this;
}
/**
* string phone_number = 11;
*/
public Builder setPhoneNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
phoneNumber_ = value;
onChanged();
return this;
}
private java.lang.Object salespersonId_ = "";
/**
* string salesperson_id = 12;
*/
public java.lang.String getSalespersonId() {
java.lang.Object ref = salespersonId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
salespersonId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string salesperson_id = 12;
*/
public com.google.protobuf.ByteString
getSalespersonIdBytes() {
java.lang.Object ref = salespersonId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
salespersonId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string salesperson_id = 12;
*/
public Builder setSalespersonId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
salespersonId_ = value;
onChanged();
return this;
}
/**
* string salesperson_id = 12;
*/
public Builder clearSalespersonId() {
salespersonId_ = getDefaultInstance().getSalespersonId();
onChanged();
return this;
}
/**
* string salesperson_id = 12;
*/
public Builder setSalespersonIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
salespersonId_ = value;
onChanged();
return this;
}
private com.google.protobuf.Timestamp lastSalesActivityDate_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastSalesActivityDateBuilder_;
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public boolean hasLastSalesActivityDate() {
return lastSalesActivityDateBuilder_ != null || lastSalesActivityDate_ != null;
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public com.google.protobuf.Timestamp getLastSalesActivityDate() {
if (lastSalesActivityDateBuilder_ == null) {
return lastSalesActivityDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastSalesActivityDate_;
} else {
return lastSalesActivityDateBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public Builder setLastSalesActivityDate(com.google.protobuf.Timestamp value) {
if (lastSalesActivityDateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastSalesActivityDate_ = value;
onChanged();
} else {
lastSalesActivityDateBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public Builder setLastSalesActivityDate(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastSalesActivityDateBuilder_ == null) {
lastSalesActivityDate_ = builderForValue.build();
onChanged();
} else {
lastSalesActivityDateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public Builder mergeLastSalesActivityDate(com.google.protobuf.Timestamp value) {
if (lastSalesActivityDateBuilder_ == null) {
if (lastSalesActivityDate_ != null) {
lastSalesActivityDate_ =
com.google.protobuf.Timestamp.newBuilder(lastSalesActivityDate_).mergeFrom(value).buildPartial();
} else {
lastSalesActivityDate_ = value;
}
onChanged();
} else {
lastSalesActivityDateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public Builder clearLastSalesActivityDate() {
if (lastSalesActivityDateBuilder_ == null) {
lastSalesActivityDate_ = null;
onChanged();
} else {
lastSalesActivityDate_ = null;
lastSalesActivityDateBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public com.google.protobuf.Timestamp.Builder getLastSalesActivityDateBuilder() {
onChanged();
return getLastSalesActivityDateFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
public com.google.protobuf.TimestampOrBuilder getLastSalesActivityDateOrBuilder() {
if (lastSalesActivityDateBuilder_ != null) {
return lastSalesActivityDateBuilder_.getMessageOrBuilder();
} else {
return lastSalesActivityDate_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : lastSalesActivityDate_;
}
}
/**
* .google.protobuf.Timestamp last_sales_activity_date = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastSalesActivityDateFieldBuilder() {
if (lastSalesActivityDateBuilder_ == null) {
lastSalesActivityDateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLastSalesActivityDate(),
getParentForChildren(),
isClean());
lastSalesActivityDate_ = null;
}
return lastSalesActivityDateBuilder_;
}
private com.google.protobuf.Timestamp lastCustomerActivityDate_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastCustomerActivityDateBuilder_;
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public boolean hasLastCustomerActivityDate() {
return lastCustomerActivityDateBuilder_ != null || lastCustomerActivityDate_ != null;
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public com.google.protobuf.Timestamp getLastCustomerActivityDate() {
if (lastCustomerActivityDateBuilder_ == null) {
return lastCustomerActivityDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastCustomerActivityDate_;
} else {
return lastCustomerActivityDateBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public Builder setLastCustomerActivityDate(com.google.protobuf.Timestamp value) {
if (lastCustomerActivityDateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastCustomerActivityDate_ = value;
onChanged();
} else {
lastCustomerActivityDateBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public Builder setLastCustomerActivityDate(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastCustomerActivityDateBuilder_ == null) {
lastCustomerActivityDate_ = builderForValue.build();
onChanged();
} else {
lastCustomerActivityDateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public Builder mergeLastCustomerActivityDate(com.google.protobuf.Timestamp value) {
if (lastCustomerActivityDateBuilder_ == null) {
if (lastCustomerActivityDate_ != null) {
lastCustomerActivityDate_ =
com.google.protobuf.Timestamp.newBuilder(lastCustomerActivityDate_).mergeFrom(value).buildPartial();
} else {
lastCustomerActivityDate_ = value;
}
onChanged();
} else {
lastCustomerActivityDateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public Builder clearLastCustomerActivityDate() {
if (lastCustomerActivityDateBuilder_ == null) {
lastCustomerActivityDate_ = null;
onChanged();
} else {
lastCustomerActivityDate_ = null;
lastCustomerActivityDateBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public com.google.protobuf.Timestamp.Builder getLastCustomerActivityDateBuilder() {
onChanged();
return getLastCustomerActivityDateFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
public com.google.protobuf.TimestampOrBuilder getLastCustomerActivityDateOrBuilder() {
if (lastCustomerActivityDateBuilder_ != null) {
return lastCustomerActivityDateBuilder_.getMessageOrBuilder();
} else {
return lastCustomerActivityDate_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : lastCustomerActivityDate_;
}
}
/**
* .google.protobuf.Timestamp last_customer_activity_date = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastCustomerActivityDateFieldBuilder() {
if (lastCustomerActivityDateBuilder_ == null) {
lastCustomerActivityDateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLastCustomerActivityDate(),
getParentForChildren(),
isClean());
lastCustomerActivityDate_ = null;
}
return lastCustomerActivityDateBuilder_;
}
private java.lang.Object salesStatus_ = "";
/**
* string sales_status = 15;
*/
public java.lang.String getSalesStatus() {
java.lang.Object ref = salesStatus_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
salesStatus_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sales_status = 15;
*/
public com.google.protobuf.ByteString
getSalesStatusBytes() {
java.lang.Object ref = salesStatus_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
salesStatus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sales_status = 15;
*/
public Builder setSalesStatus(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
salesStatus_ = value;
onChanged();
return this;
}
/**
* string sales_status = 15;
*/
public Builder clearSalesStatus() {
salesStatus_ = getDefaultInstance().getSalesStatus();
onChanged();
return this;
}
/**
* string sales_status = 15;
*/
public Builder setSalesStatusBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
salesStatus_ = value;
onChanged();
return this;
}
private boolean isRead_ ;
/**
* bool is_read = 16;
*/
public boolean getIsRead() {
return isRead_;
}
/**
* bool is_read = 16;
*/
public Builder setIsRead(boolean value) {
isRead_ = value;
onChanged();
return this;
}
/**
* bool is_read = 16;
*/
public Builder clearIsRead() {
isRead_ = false;
onChanged();
return this;
}
private java.lang.Object salesPersonAction_ = "";
/**
* string sales_person_action = 17;
*/
public java.lang.String getSalesPersonAction() {
java.lang.Object ref = salesPersonAction_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
salesPersonAction_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sales_person_action = 17;
*/
public com.google.protobuf.ByteString
getSalesPersonActionBytes() {
java.lang.Object ref = salesPersonAction_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
salesPersonAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sales_person_action = 17;
*/
public Builder setSalesPersonAction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
salesPersonAction_ = value;
onChanged();
return this;
}
/**
* string sales_person_action = 17;
*/
public Builder clearSalesPersonAction() {
salesPersonAction_ = getDefaultInstance().getSalesPersonAction();
onChanged();
return this;
}
/**
* string sales_person_action = 17;
*/
public Builder setSalesPersonActionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
salesPersonAction_ = value;
onChanged();
return this;
}
private com.google.protobuf.Timestamp latestSnapshotExpiry_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> latestSnapshotExpiryBuilder_;
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public boolean hasLatestSnapshotExpiry() {
return latestSnapshotExpiryBuilder_ != null || latestSnapshotExpiry_ != null;
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public com.google.protobuf.Timestamp getLatestSnapshotExpiry() {
if (latestSnapshotExpiryBuilder_ == null) {
return latestSnapshotExpiry_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : latestSnapshotExpiry_;
} else {
return latestSnapshotExpiryBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public Builder setLatestSnapshotExpiry(com.google.protobuf.Timestamp value) {
if (latestSnapshotExpiryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
latestSnapshotExpiry_ = value;
onChanged();
} else {
latestSnapshotExpiryBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public Builder setLatestSnapshotExpiry(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (latestSnapshotExpiryBuilder_ == null) {
latestSnapshotExpiry_ = builderForValue.build();
onChanged();
} else {
latestSnapshotExpiryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public Builder mergeLatestSnapshotExpiry(com.google.protobuf.Timestamp value) {
if (latestSnapshotExpiryBuilder_ == null) {
if (latestSnapshotExpiry_ != null) {
latestSnapshotExpiry_ =
com.google.protobuf.Timestamp.newBuilder(latestSnapshotExpiry_).mergeFrom(value).buildPartial();
} else {
latestSnapshotExpiry_ = value;
}
onChanged();
} else {
latestSnapshotExpiryBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public Builder clearLatestSnapshotExpiry() {
if (latestSnapshotExpiryBuilder_ == null) {
latestSnapshotExpiry_ = null;
onChanged();
} else {
latestSnapshotExpiry_ = null;
latestSnapshotExpiryBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public com.google.protobuf.Timestamp.Builder getLatestSnapshotExpiryBuilder() {
onChanged();
return getLatestSnapshotExpiryFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
public com.google.protobuf.TimestampOrBuilder getLatestSnapshotExpiryOrBuilder() {
if (latestSnapshotExpiryBuilder_ != null) {
return latestSnapshotExpiryBuilder_.getMessageOrBuilder();
} else {
return latestSnapshotExpiry_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : latestSnapshotExpiry_;
}
}
/**
* .google.protobuf.Timestamp latest_snapshot_expiry = 18;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLatestSnapshotExpiryFieldBuilder() {
if (latestSnapshotExpiryBuilder_ == null) {
latestSnapshotExpiryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLatestSnapshotExpiry(),
getParentForChildren(),
isClean());
latestSnapshotExpiry_ = null;
}
return latestSnapshotExpiryBuilder_;
}
private boolean snapshotOrCampaignEmailStatus_ ;
/**
* bool snapshot_or_campaign_email_status = 19;
*/
public boolean getSnapshotOrCampaignEmailStatus() {
return snapshotOrCampaignEmailStatus_;
}
/**
* bool snapshot_or_campaign_email_status = 19;
*/
public Builder setSnapshotOrCampaignEmailStatus(boolean value) {
snapshotOrCampaignEmailStatus_ = value;
onChanged();
return this;
}
/**
* bool snapshot_or_campaign_email_status = 19;
*/
public Builder clearSnapshotOrCampaignEmailStatus() {
snapshotOrCampaignEmailStatus_ = false;
onChanged();
return this;
}
private java.lang.Object activityType_ = "";
/**
* string activity_type = 20;
*/
public java.lang.String getActivityType() {
java.lang.Object ref = activityType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
activityType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string activity_type = 20;
*/
public com.google.protobuf.ByteString
getActivityTypeBytes() {
java.lang.Object ref = activityType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
activityType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string activity_type = 20;
*/
public Builder setActivityType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
activityType_ = value;
onChanged();
return this;
}
/**
* string activity_type = 20;
*/
public Builder clearActivityType() {
activityType_ = getDefaultInstance().getActivityType();
onChanged();
return this;
}
/**
* string activity_type = 20;
*/
public Builder setActivityTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
activityType_ = value;
onChanged();
return this;
}
private com.google.protobuf.Timestamp lastConnectedDate_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastConnectedDateBuilder_;
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public boolean hasLastConnectedDate() {
return lastConnectedDateBuilder_ != null || lastConnectedDate_ != null;
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public com.google.protobuf.Timestamp getLastConnectedDate() {
if (lastConnectedDateBuilder_ == null) {
return lastConnectedDate_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastConnectedDate_;
} else {
return lastConnectedDateBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public Builder setLastConnectedDate(com.google.protobuf.Timestamp value) {
if (lastConnectedDateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastConnectedDate_ = value;
onChanged();
} else {
lastConnectedDateBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public Builder setLastConnectedDate(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastConnectedDateBuilder_ == null) {
lastConnectedDate_ = builderForValue.build();
onChanged();
} else {
lastConnectedDateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public Builder mergeLastConnectedDate(com.google.protobuf.Timestamp value) {
if (lastConnectedDateBuilder_ == null) {
if (lastConnectedDate_ != null) {
lastConnectedDate_ =
com.google.protobuf.Timestamp.newBuilder(lastConnectedDate_).mergeFrom(value).buildPartial();
} else {
lastConnectedDate_ = value;
}
onChanged();
} else {
lastConnectedDateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public Builder clearLastConnectedDate() {
if (lastConnectedDateBuilder_ == null) {
lastConnectedDate_ = null;
onChanged();
} else {
lastConnectedDate_ = null;
lastConnectedDateBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public com.google.protobuf.Timestamp.Builder getLastConnectedDateBuilder() {
onChanged();
return getLastConnectedDateFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
public com.google.protobuf.TimestampOrBuilder getLastConnectedDateOrBuilder() {
if (lastConnectedDateBuilder_ != null) {
return lastConnectedDateBuilder_.getMessageOrBuilder();
} else {
return lastConnectedDate_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : lastConnectedDate_;
}
}
/**
* .google.protobuf.Timestamp last_connected_date = 21;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastConnectedDateFieldBuilder() {
if (lastConnectedDateBuilder_ == null) {
lastConnectedDateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLastConnectedDate(),
getParentForChildren(),
isClean());
lastConnectedDate_ = null;
}
return lastConnectedDateBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.Business)
}
// @@protoc_insertion_point(class_scope:sales.v1.Business)
private static final com.vendasta.sales.v1.generated.BusinessProto.Business DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.BusinessProto.Business();
}
public static com.vendasta.sales.v1.generated.BusinessProto.Business getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Business parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Business(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.BusinessProto.Business getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_BusinessPagedResponseMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_BusinessPagedResponseMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_BusinessSearchFilters_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_BusinessSearchFilters_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_BusinessSearchSortOptions_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_BusinessSearchSortOptions_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_Business_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_Business_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\027sales/v1/business.proto\022\010sales.v1\032\037goo" +
"gle/protobuf/timestamp.proto\"]\n\035Business" +
"PagedResponseMetadata\022\023\n\013next_cursor\030\001 \001" +
"(\t\022\020\n\010has_more\030\002 \001(\010\022\025\n\rtotal_results\030\003 " +
"\001(\003\"\343\003\n\025BusinessSearchFilters\022\022\n\npartner" +
"_id\030\001 \001(\t\022\021\n\tmarket_id\030\002 \001(\t\022\026\n\016sales_st" +
"atuses\030\003 \003(\t\022\024\n\014sales_action\030\004 \001(\t\022-\n\tfr" +
"om_date\030\005 \001(\0132\032.google.protobuf.Timestam" +
"p\022+\n\007to_date\030\006 \001(\0132\032.google.protobuf.Tim" +
"estamp\022$\n\010archived\030\007 \001(\0162\022.sales.v1.Arch",
"ived\022\024\n\014account_tags\030\010 \003(\t\022\033\n\023business_c" +
"ategories\030\t \003(\t\022\'\n\013on_campaign\030\n \001(\0162\022.s" +
"ales.v1.Campaign\022)\n\rsnapshot_sent\030\013 \001(\0162" +
"\022.sales.v1.Snapshot\022\r\n\005query\030\014 \001(\t\022\021\n\tas" +
"signees\030\r \003(\t\022\014\n\004goal\030\016 \001(\t\022\033\n\023training_" +
"priorities\030\017 \001(\t\022\037\n\027custom_field_partner" +
"_id\030\020 \001(\t\"E\n\031BusinessSearchSortOptions\022\020" +
"\n\010sort_key\030\001 \001(\t\022\026\n\016sort_ascending\030\002 \001(\010" +
"\"\336\004\n\010Business\022\022\n\npartner_id\030\001 \001(\t\022\021\n\tmar" +
"ket_id\030\002 \001(\t\022\014\n\004name\030\003 \001(\t\022\030\n\020account_gr",
"oup_id\030\004 \001(\t\022\017\n\007hotness\030\005 \001(\003\022\014\n\004city\030\006 " +
"\001(\t\022\013\n\003zip\030\007 \001(\t\022\017\n\007address\030\010 \001(\t\022\r\n\005sta" +
"te\030\t \001(\t\022\017\n\007country\030\n \001(\t\022\024\n\014phone_numbe" +
"r\030\013 \001(\t\022\026\n\016salesperson_id\030\014 \001(\t\022<\n\030last_" +
"sales_activity_date\030\r \001(\0132\032.google.proto" +
"buf.Timestamp\022?\n\033last_customer_activity_" +
"date\030\016 \001(\0132\032.google.protobuf.Timestamp\022\024" +
"\n\014sales_status\030\017 \001(\t\022\017\n\007is_read\030\020 \001(\010\022\033\n" +
"\023sales_person_action\030\021 \001(\t\022:\n\026latest_sna" +
"pshot_expiry\030\022 \001(\0132\032.google.protobuf.Tim",
"estamp\022)\n!snapshot_or_campaign_email_sta" +
"tus\030\023 \001(\010\022\025\n\ractivity_type\030\024 \001(\t\0227\n\023last" +
"_connected_date\030\025 \001(\0132\032.google.protobuf." +
"Timestamp*?\n\010Campaign\022\020\n\014CAMPAIGN_ANY\020\000\022" +
"\017\n\013CAMPAIGN_ON\020\001\022\020\n\014CAMPAIGN_OFF\020\002*F\n\010Sn" +
"apshot\022\020\n\014SNAPSHOT_ANY\020\000\022\021\n\rSNAPSHOT_SEN" +
"T\020\001\022\025\n\021SNAPSHOT_NOT_SENT\020\002*?\n\010Archived\022\020" +
"\n\014ARCHIVED_ANY\020\000\022\020\n\014ARCHIVED_YES\020\001\022\017\n\013AR" +
"CHIVED_NO\020\002Bk\n\037com.vendasta.sales.v1.gen" +
"eratedB\rBusinessProtoZ9github.com/vendas",
"ta/generated-protos-go/sales/v1;sales/v1" +
"b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.TimestampProto.getDescriptor(),
}, assigner);
internal_static_sales_v1_BusinessPagedResponseMetadata_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_sales_v1_BusinessPagedResponseMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_BusinessPagedResponseMetadata_descriptor,
new java.lang.String[] { "NextCursor", "HasMore", "TotalResults", });
internal_static_sales_v1_BusinessSearchFilters_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_sales_v1_BusinessSearchFilters_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_BusinessSearchFilters_descriptor,
new java.lang.String[] { "PartnerId", "MarketId", "SalesStatuses", "SalesAction", "FromDate", "ToDate", "Archived", "AccountTags", "BusinessCategories", "OnCampaign", "SnapshotSent", "Query", "Assignees", "Goal", "TrainingPriorities", "CustomFieldPartnerId", });
internal_static_sales_v1_BusinessSearchSortOptions_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_sales_v1_BusinessSearchSortOptions_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_BusinessSearchSortOptions_descriptor,
new java.lang.String[] { "SortKey", "SortAscending", });
internal_static_sales_v1_Business_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_sales_v1_Business_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_Business_descriptor,
new java.lang.String[] { "PartnerId", "MarketId", "Name", "AccountGroupId", "Hotness", "City", "Zip", "Address", "State", "Country", "PhoneNumber", "SalespersonId", "LastSalesActivityDate", "LastCustomerActivityDate", "SalesStatus", "IsRead", "SalesPersonAction", "LatestSnapshotExpiry", "SnapshotOrCampaignEmailStatus", "ActivityType", "LastConnectedDate", });
com.google.protobuf.TimestampProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy