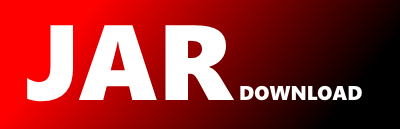
com.vendasta.sales.v1.generated.BusinessServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.generated;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.3.0)",
comments = "Source: sales/v1/api.proto")
public final class BusinessServiceGrpc {
private BusinessServiceGrpc() {}
public static final String SERVICE_NAME = "sales.v1.BusinessService";
// Static method descriptors that strictly reflect the proto.
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_SEARCH =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"sales.v1.BusinessService", "Search"),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_REQUEST_SUBSCRIPTION_CHANGE =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"sales.v1.BusinessService", "RequestSubscriptionChange"),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.google.protobuf.Empty.getDefaultInstance()));
/**
* Creates a new async stub that supports all call types for the service
*/
public static BusinessServiceStub newStub(io.grpc.Channel channel) {
return new BusinessServiceStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static BusinessServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new BusinessServiceBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary and streaming output calls on the service
*/
public static BusinessServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
return new BusinessServiceFutureStub(channel);
}
/**
*/
public static abstract class BusinessServiceImplBase implements io.grpc.BindableService {
/**
*
* BusinessSearch returns a set of businesses
*
*/
public void search(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_SEARCH, responseObserver);
}
/**
*
* RequestSubscriptionChange allows a business to send a request to the partner configured email address to change
* their subscription for a product. Most likely use case is to cancel.
*
*/
public void requestSubscriptionChange(com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_REQUEST_SUBSCRIPTION_CHANGE, responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
METHOD_SEARCH,
asyncUnaryCall(
new MethodHandlers<
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest,
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse>(
this, METHODID_SEARCH)))
.addMethod(
METHOD_REQUEST_SUBSCRIPTION_CHANGE,
asyncUnaryCall(
new MethodHandlers<
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest,
com.google.protobuf.Empty>(
this, METHODID_REQUEST_SUBSCRIPTION_CHANGE)))
.build();
}
}
/**
*/
public static final class BusinessServiceStub extends io.grpc.stub.AbstractStub {
private BusinessServiceStub(io.grpc.Channel channel) {
super(channel);
}
private BusinessServiceStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BusinessServiceStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new BusinessServiceStub(channel, callOptions);
}
/**
*
* BusinessSearch returns a set of businesses
*
*/
public void search(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_SEARCH, getCallOptions()), request, responseObserver);
}
/**
*
* RequestSubscriptionChange allows a business to send a request to the partner configured email address to change
* their subscription for a product. Most likely use case is to cancel.
*
*/
public void requestSubscriptionChange(com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_REQUEST_SUBSCRIPTION_CHANGE, getCallOptions()), request, responseObserver);
}
}
/**
*/
public static final class BusinessServiceBlockingStub extends io.grpc.stub.AbstractStub {
private BusinessServiceBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private BusinessServiceBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BusinessServiceBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new BusinessServiceBlockingStub(channel, callOptions);
}
/**
*
* BusinessSearch returns a set of businesses
*
*/
public com.vendasta.sales.v1.generated.ApiProto.BusinessSearchResponse search(com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_SEARCH, getCallOptions(), request);
}
/**
*
* RequestSubscriptionChange allows a business to send a request to the partner configured email address to change
* their subscription for a product. Most likely use case is to cancel.
*
*/
public com.google.protobuf.Empty requestSubscriptionChange(com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_REQUEST_SUBSCRIPTION_CHANGE, getCallOptions(), request);
}
}
/**
*/
public static final class BusinessServiceFutureStub extends io.grpc.stub.AbstractStub {
private BusinessServiceFutureStub(io.grpc.Channel channel) {
super(channel);
}
private BusinessServiceFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BusinessServiceFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new BusinessServiceFutureStub(channel, callOptions);
}
/**
*
* BusinessSearch returns a set of businesses
*
*/
public com.google.common.util.concurrent.ListenableFuture search(
com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_SEARCH, getCallOptions()), request);
}
/**
*
* RequestSubscriptionChange allows a business to send a request to the partner configured email address to change
* their subscription for a product. Most likely use case is to cancel.
*
*/
public com.google.common.util.concurrent.ListenableFuture requestSubscriptionChange(
com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_REQUEST_SUBSCRIPTION_CHANGE, getCallOptions()), request);
}
}
private static final int METHODID_SEARCH = 0;
private static final int METHODID_REQUEST_SUBSCRIPTION_CHANGE = 1;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final BusinessServiceImplBase serviceImpl;
private final int methodId;
MethodHandlers(BusinessServiceImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_SEARCH:
serviceImpl.search((com.vendasta.sales.v1.generated.ApiProto.BusinessSearchRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_REQUEST_SUBSCRIPTION_CHANGE:
serviceImpl.requestSubscriptionChange((com.vendasta.sales.v1.generated.ApiProto.RequestSubscriptionChangeRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static final class BusinessServiceDescriptorSupplier implements io.grpc.protobuf.ProtoFileDescriptorSupplier {
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.getDescriptor();
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (BusinessServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new BusinessServiceDescriptorSupplier())
.addMethod(METHOD_SEARCH)
.addMethod(METHOD_REQUEST_SUBSCRIPTION_CHANGE)
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy