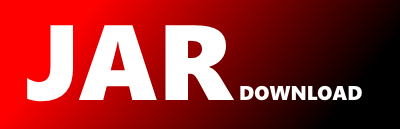
com.vendasta.sales.v1.generated.SalesGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.generated;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
/**
*
* The sales service is the backend for anything generically related to sales that struggles to fit in a more specific domain.
* Note that there are currently separate, more specific domains for sales orders and opportunities.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.3.0)",
comments = "Source: sales/v1/api.proto")
public final class SalesGrpc {
private SalesGrpc() {}
public static final String SERVICE_NAME = "sales.v1.Sales";
// Static method descriptors that strictly reflect the proto.
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_GET_AUTO_ASSIGN_CONFIG =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"sales.v1.Sales", "GetAutoAssignConfig"),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"sales.v1.Sales", "CreateOrUpdateAutoAssignConfig"),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.google.protobuf.Empty.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_GET_SALESPERSON =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"sales.v1.Sales", "GetSalesperson"),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.SalespersonProto.Salesperson.getDefaultInstance()));
/**
* Creates a new async stub that supports all call types for the service
*/
public static SalesStub newStub(io.grpc.Channel channel) {
return new SalesStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static SalesBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new SalesBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary and streaming output calls on the service
*/
public static SalesFutureStub newFutureStub(
io.grpc.Channel channel) {
return new SalesFutureStub(channel);
}
/**
*
* The sales service is the backend for anything generically related to sales that struggles to fit in a more specific domain.
* Note that there are currently separate, more specific domains for sales orders and opportunities.
*
*/
public static abstract class SalesImplBase implements io.grpc.BindableService {
/**
*
* GetAutoAssignConfig gets the auto assign account configuration for market.
*
*/
public void getAutoAssignConfig(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_GET_AUTO_ASSIGN_CONFIG, responseObserver);
}
/**
*
* CreateOrUpdateAutoAssignConfig creates or updates the auto assign account configuration for the market.
*
*/
public void createOrUpdateAutoAssignConfig(com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG, responseObserver);
}
/**
*
* GetSalesperson gets a salesperson
*
*/
public void getSalesperson(com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_GET_SALESPERSON, responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
METHOD_GET_AUTO_ASSIGN_CONFIG,
asyncUnaryCall(
new MethodHandlers<
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest,
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse>(
this, METHODID_GET_AUTO_ASSIGN_CONFIG)))
.addMethod(
METHOD_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG,
asyncUnaryCall(
new MethodHandlers<
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest,
com.google.protobuf.Empty>(
this, METHODID_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG)))
.addMethod(
METHOD_GET_SALESPERSON,
asyncUnaryCall(
new MethodHandlers<
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest,
com.vendasta.sales.v1.generated.SalespersonProto.Salesperson>(
this, METHODID_GET_SALESPERSON)))
.build();
}
}
/**
*
* The sales service is the backend for anything generically related to sales that struggles to fit in a more specific domain.
* Note that there are currently separate, more specific domains for sales orders and opportunities.
*
*/
public static final class SalesStub extends io.grpc.stub.AbstractStub {
private SalesStub(io.grpc.Channel channel) {
super(channel);
}
private SalesStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SalesStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new SalesStub(channel, callOptions);
}
/**
*
* GetAutoAssignConfig gets the auto assign account configuration for market.
*
*/
public void getAutoAssignConfig(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_GET_AUTO_ASSIGN_CONFIG, getCallOptions()), request, responseObserver);
}
/**
*
* CreateOrUpdateAutoAssignConfig creates or updates the auto assign account configuration for the market.
*
*/
public void createOrUpdateAutoAssignConfig(com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG, getCallOptions()), request, responseObserver);
}
/**
*
* GetSalesperson gets a salesperson
*
*/
public void getSalesperson(com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_GET_SALESPERSON, getCallOptions()), request, responseObserver);
}
}
/**
*
* The sales service is the backend for anything generically related to sales that struggles to fit in a more specific domain.
* Note that there are currently separate, more specific domains for sales orders and opportunities.
*
*/
public static final class SalesBlockingStub extends io.grpc.stub.AbstractStub {
private SalesBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private SalesBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SalesBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new SalesBlockingStub(channel, callOptions);
}
/**
*
* GetAutoAssignConfig gets the auto assign account configuration for market.
*
*/
public com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigResponse getAutoAssignConfig(com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_GET_AUTO_ASSIGN_CONFIG, getCallOptions(), request);
}
/**
*
* CreateOrUpdateAutoAssignConfig creates or updates the auto assign account configuration for the market.
*
*/
public com.google.protobuf.Empty createOrUpdateAutoAssignConfig(com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG, getCallOptions(), request);
}
/**
*
* GetSalesperson gets a salesperson
*
*/
public com.vendasta.sales.v1.generated.SalespersonProto.Salesperson getSalesperson(com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_GET_SALESPERSON, getCallOptions(), request);
}
}
/**
*
* The sales service is the backend for anything generically related to sales that struggles to fit in a more specific domain.
* Note that there are currently separate, more specific domains for sales orders and opportunities.
*
*/
public static final class SalesFutureStub extends io.grpc.stub.AbstractStub {
private SalesFutureStub(io.grpc.Channel channel) {
super(channel);
}
private SalesFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SalesFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new SalesFutureStub(channel, callOptions);
}
/**
*
* GetAutoAssignConfig gets the auto assign account configuration for market.
*
*/
public com.google.common.util.concurrent.ListenableFuture getAutoAssignConfig(
com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_GET_AUTO_ASSIGN_CONFIG, getCallOptions()), request);
}
/**
*
* CreateOrUpdateAutoAssignConfig creates or updates the auto assign account configuration for the market.
*
*/
public com.google.common.util.concurrent.ListenableFuture createOrUpdateAutoAssignConfig(
com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG, getCallOptions()), request);
}
/**
*
* GetSalesperson gets a salesperson
*
*/
public com.google.common.util.concurrent.ListenableFuture getSalesperson(
com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_GET_SALESPERSON, getCallOptions()), request);
}
}
private static final int METHODID_GET_AUTO_ASSIGN_CONFIG = 0;
private static final int METHODID_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG = 1;
private static final int METHODID_GET_SALESPERSON = 2;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final SalesImplBase serviceImpl;
private final int methodId;
MethodHandlers(SalesImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_GET_AUTO_ASSIGN_CONFIG:
serviceImpl.getAutoAssignConfig((com.vendasta.sales.v1.generated.ApiProto.GetAutoAssignConfigRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG:
serviceImpl.createOrUpdateAutoAssignConfig((com.vendasta.sales.v1.generated.ApiProto.CreateOrUpdateAutoAssignConfigRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_SALESPERSON:
serviceImpl.getSalesperson((com.vendasta.sales.v1.generated.ApiProto.GetSalespersonRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static final class SalesDescriptorSupplier implements io.grpc.protobuf.ProtoFileDescriptorSupplier {
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.getDescriptor();
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (SalesGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new SalesDescriptorSupplier())
.addMethod(METHOD_GET_AUTO_ASSIGN_CONFIG)
.addMethod(METHOD_CREATE_OR_UPDATE_AUTO_ASSIGN_CONFIG)
.addMethod(METHOD_GET_SALESPERSON)
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy