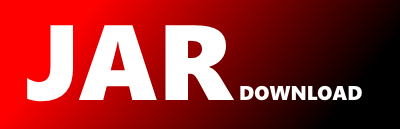
com.vendasta.sales.v1.generated.SalesTeamServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.generated;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.3.0)",
comments = "Source: sales/v1/api.proto")
public final class SalesTeamServiceGrpc {
private SalesTeamServiceGrpc() {}
public static final String SERVICE_NAME = "sales.v1.SalesTeamService";
// Static method descriptors that strictly reflect the proto.
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_LIST =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"sales.v1.SalesTeamService", "List"),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse.getDefaultInstance()));
/**
* Creates a new async stub that supports all call types for the service
*/
public static SalesTeamServiceStub newStub(io.grpc.Channel channel) {
return new SalesTeamServiceStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static SalesTeamServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new SalesTeamServiceBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary and streaming output calls on the service
*/
public static SalesTeamServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
return new SalesTeamServiceFutureStub(channel);
}
/**
*/
public static abstract class SalesTeamServiceImplBase implements io.grpc.BindableService {
/**
*/
public void list(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_LIST, responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
METHOD_LIST,
asyncUnaryCall(
new MethodHandlers<
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest,
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse>(
this, METHODID_LIST)))
.build();
}
}
/**
*/
public static final class SalesTeamServiceStub extends io.grpc.stub.AbstractStub {
private SalesTeamServiceStub(io.grpc.Channel channel) {
super(channel);
}
private SalesTeamServiceStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SalesTeamServiceStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new SalesTeamServiceStub(channel, callOptions);
}
/**
*/
public void list(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_LIST, getCallOptions()), request, responseObserver);
}
}
/**
*/
public static final class SalesTeamServiceBlockingStub extends io.grpc.stub.AbstractStub {
private SalesTeamServiceBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private SalesTeamServiceBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SalesTeamServiceBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new SalesTeamServiceBlockingStub(channel, callOptions);
}
/**
*/
public com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsResponse list(com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_LIST, getCallOptions(), request);
}
}
/**
*/
public static final class SalesTeamServiceFutureStub extends io.grpc.stub.AbstractStub {
private SalesTeamServiceFutureStub(io.grpc.Channel channel) {
super(channel);
}
private SalesTeamServiceFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SalesTeamServiceFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new SalesTeamServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture list(
com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_LIST, getCallOptions()), request);
}
}
private static final int METHODID_LIST = 0;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final SalesTeamServiceImplBase serviceImpl;
private final int methodId;
MethodHandlers(SalesTeamServiceImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_LIST:
serviceImpl.list((com.vendasta.sales.v1.generated.ApiProto.ListSalesTeamsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static final class SalesTeamServiceDescriptorSupplier implements io.grpc.protobuf.ProtoFileDescriptorSupplier {
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.vendasta.sales.v1.generated.ApiProto.getDescriptor();
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (SalesTeamServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new SalesTeamServiceDescriptorSupplier())
.addMethod(METHOD_LIST)
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy