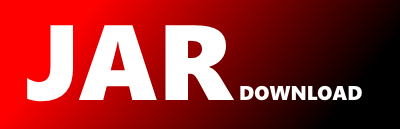
com.vendasta.sales.v1.generated.UserActionsProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: sales/v1/user_actions.proto
package com.vendasta.sales.v1.generated;
public final class UserActionsProto {
private UserActionsProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code sales.v1.ActionCategory}
*/
public enum ActionCategory
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ACTION_CATEGORY_UNKNOWN = 0;
*/
ACTION_CATEGORY_UNKNOWN(0),
/**
* ACTION_CATEGORY_CLICK = 1;
*/
ACTION_CATEGORY_CLICK(1),
/**
* ACTION_CATEGORY_VIEW = 2;
*/
ACTION_CATEGORY_VIEW(2),
/**
* ACTION_CATEGORY_LINGER = 3;
*/
ACTION_CATEGORY_LINGER(3),
/**
* ACTION_CATEGORY_TYPED = 4;
*/
ACTION_CATEGORY_TYPED(4),
/**
* ACTION_CATEGORY_PURCHASED = 5;
*/
ACTION_CATEGORY_PURCHASED(5),
/**
* ACTION_CATEGORY_ADDED_TO_CART = 6;
*/
ACTION_CATEGORY_ADDED_TO_CART(6),
UNRECOGNIZED(-1),
;
/**
* ACTION_CATEGORY_UNKNOWN = 0;
*/
public static final int ACTION_CATEGORY_UNKNOWN_VALUE = 0;
/**
* ACTION_CATEGORY_CLICK = 1;
*/
public static final int ACTION_CATEGORY_CLICK_VALUE = 1;
/**
* ACTION_CATEGORY_VIEW = 2;
*/
public static final int ACTION_CATEGORY_VIEW_VALUE = 2;
/**
* ACTION_CATEGORY_LINGER = 3;
*/
public static final int ACTION_CATEGORY_LINGER_VALUE = 3;
/**
* ACTION_CATEGORY_TYPED = 4;
*/
public static final int ACTION_CATEGORY_TYPED_VALUE = 4;
/**
* ACTION_CATEGORY_PURCHASED = 5;
*/
public static final int ACTION_CATEGORY_PURCHASED_VALUE = 5;
/**
* ACTION_CATEGORY_ADDED_TO_CART = 6;
*/
public static final int ACTION_CATEGORY_ADDED_TO_CART_VALUE = 6;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ActionCategory valueOf(int value) {
return forNumber(value);
}
public static ActionCategory forNumber(int value) {
switch (value) {
case 0: return ACTION_CATEGORY_UNKNOWN;
case 1: return ACTION_CATEGORY_CLICK;
case 2: return ACTION_CATEGORY_VIEW;
case 3: return ACTION_CATEGORY_LINGER;
case 4: return ACTION_CATEGORY_TYPED;
case 5: return ACTION_CATEGORY_PURCHASED;
case 6: return ACTION_CATEGORY_ADDED_TO_CART;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ActionCategory> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ActionCategory findValueByNumber(int number) {
return ActionCategory.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.getDescriptor().getEnumTypes().get(0);
}
private static final ActionCategory[] VALUES = values();
public static ActionCategory valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ActionCategory(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sales.v1.ActionCategory)
}
public interface UserActionsPagedResponseMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.UserActionsPagedResponseMetadata)
com.google.protobuf.MessageOrBuilder {
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
java.lang.String getNextCursor();
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
com.google.protobuf.ByteString
getNextCursorBytes();
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
boolean getHasMore();
/**
*
* Total results found by the operation
*
*
* int64 total_results = 3;
*/
long getTotalResults();
}
/**
*
* Contains metadata about the paged response
*
*
* Protobuf type {@code sales.v1.UserActionsPagedResponseMetadata}
*/
public static final class UserActionsPagedResponseMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.UserActionsPagedResponseMetadata)
UserActionsPagedResponseMetadataOrBuilder {
// Use UserActionsPagedResponseMetadata.newBuilder() to construct.
private UserActionsPagedResponseMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserActionsPagedResponseMetadata() {
nextCursor_ = "";
hasMore_ = false;
totalResults_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private UserActionsPagedResponseMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
nextCursor_ = s;
break;
}
case 16: {
hasMore_ = input.readBool();
break;
}
case 24: {
totalResults_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsPagedResponseMetadata_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsPagedResponseMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.class, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.Builder.class);
}
public static final int NEXT_CURSOR_FIELD_NUMBER = 1;
private volatile java.lang.Object nextCursor_;
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public java.lang.String getNextCursor() {
java.lang.Object ref = nextCursor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nextCursor_ = s;
return s;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public com.google.protobuf.ByteString
getNextCursorBytes() {
java.lang.Object ref = nextCursor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nextCursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HAS_MORE_FIELD_NUMBER = 2;
private boolean hasMore_;
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public boolean getHasMore() {
return hasMore_;
}
public static final int TOTAL_RESULTS_FIELD_NUMBER = 3;
private long totalResults_;
/**
*
* Total results found by the operation
*
*
* int64 total_results = 3;
*/
public long getTotalResults() {
return totalResults_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNextCursorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, nextCursor_);
}
if (hasMore_ != false) {
output.writeBool(2, hasMore_);
}
if (totalResults_ != 0L) {
output.writeInt64(3, totalResults_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNextCursorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, nextCursor_);
}
if (hasMore_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, hasMore_);
}
if (totalResults_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, totalResults_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata other = (com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata) obj;
boolean result = true;
result = result && getNextCursor()
.equals(other.getNextCursor());
result = result && (getHasMore()
== other.getHasMore());
result = result && (getTotalResults()
== other.getTotalResults());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NEXT_CURSOR_FIELD_NUMBER;
hash = (53 * hash) + getNextCursor().hashCode();
hash = (37 * hash) + HAS_MORE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasMore());
hash = (37 * hash) + TOTAL_RESULTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotalResults());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Contains metadata about the paged response
*
*
* Protobuf type {@code sales.v1.UserActionsPagedResponseMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.UserActionsPagedResponseMetadata)
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsPagedResponseMetadata_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsPagedResponseMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.class, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
nextCursor_ = "";
hasMore_ = false;
totalResults_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsPagedResponseMetadata_descriptor;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata build() {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata buildPartial() {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata result = new com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata(this);
result.nextCursor_ = nextCursor_;
result.hasMore_ = hasMore_;
result.totalResults_ = totalResults_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata) {
return mergeFrom((com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata other) {
if (other == com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.getDefaultInstance()) return this;
if (!other.getNextCursor().isEmpty()) {
nextCursor_ = other.nextCursor_;
onChanged();
}
if (other.getHasMore() != false) {
setHasMore(other.getHasMore());
}
if (other.getTotalResults() != 0L) {
setTotalResults(other.getTotalResults());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object nextCursor_ = "";
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public java.lang.String getNextCursor() {
java.lang.Object ref = nextCursor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nextCursor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public com.google.protobuf.ByteString
getNextCursorBytes() {
java.lang.Object ref = nextCursor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nextCursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder setNextCursor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nextCursor_ = value;
onChanged();
return this;
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder clearNextCursor() {
nextCursor_ = getDefaultInstance().getNextCursor();
onChanged();
return this;
}
/**
*
* A cursor that can be provided to retrieve the next page of results
*
*
* string next_cursor = 1;
*/
public Builder setNextCursorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nextCursor_ = value;
onChanged();
return this;
}
private boolean hasMore_ ;
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public boolean getHasMore() {
return hasMore_;
}
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public Builder setHasMore(boolean value) {
hasMore_ = value;
onChanged();
return this;
}
/**
*
* Whether or not more results exist
*
*
* bool has_more = 2;
*/
public Builder clearHasMore() {
hasMore_ = false;
onChanged();
return this;
}
private long totalResults_ ;
/**
*
* Total results found by the operation
*
*
* int64 total_results = 3;
*/
public long getTotalResults() {
return totalResults_;
}
/**
*
* Total results found by the operation
*
*
* int64 total_results = 3;
*/
public Builder setTotalResults(long value) {
totalResults_ = value;
onChanged();
return this;
}
/**
*
* Total results found by the operation
*
*
* int64 total_results = 3;
*/
public Builder clearTotalResults() {
totalResults_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.UserActionsPagedResponseMetadata)
}
// @@protoc_insertion_point(class_scope:sales.v1.UserActionsPagedResponseMetadata)
private static final com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata();
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public UserActionsPagedResponseMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UserActionsPagedResponseMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UserActionsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.UserActionsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
java.lang.String getAccountGroupId();
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
com.google.protobuf.ByteString
getAccountGroupIdBytes();
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
int getCategoryValue();
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory();
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
java.lang.String getDescription();
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
* Protobuf type {@code sales.v1.UserActionsRequest}
*/
public static final class UserActionsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.UserActionsRequest)
UserActionsRequestOrBuilder {
// Use UserActionsRequest.newBuilder() to construct.
private UserActionsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserActionsRequest() {
accountGroupId_ = "";
category_ = 0;
description_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private UserActionsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
accountGroupId_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
category_ = rawValue;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.class, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder.class);
}
public static final int ACCOUNT_GROUP_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object accountGroupId_;
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public java.lang.String getAccountGroupId() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountGroupId_ = s;
return s;
}
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public com.google.protobuf.ByteString
getAccountGroupIdBytes() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountGroupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CATEGORY_FIELD_NUMBER = 2;
private int category_;
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public int getCategoryValue() {
return category_;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory() {
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory result = com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.valueOf(category_);
return result == null ? com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.UNRECOGNIZED : result;
}
public static final int DESCRIPTION_FIELD_NUMBER = 3;
private volatile java.lang.Object description_;
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getAccountGroupIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, accountGroupId_);
}
if (category_ != com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.ACTION_CATEGORY_UNKNOWN.getNumber()) {
output.writeEnum(2, category_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, description_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getAccountGroupIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, accountGroupId_);
}
if (category_ != com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.ACTION_CATEGORY_UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, category_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, description_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest other = (com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest) obj;
boolean result = true;
result = result && getAccountGroupId()
.equals(other.getAccountGroupId());
result = result && category_ == other.category_;
result = result && getDescription()
.equals(other.getDescription());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ACCOUNT_GROUP_ID_FIELD_NUMBER;
hash = (53 * hash) + getAccountGroupId().hashCode();
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + category_;
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.UserActionsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.UserActionsRequest)
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.class, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
accountGroupId_ = "";
category_ = 0;
description_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsRequest_descriptor;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest build() {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest buildPartial() {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest result = new com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest(this);
result.accountGroupId_ = accountGroupId_;
result.category_ = category_;
result.description_ = description_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest other) {
if (other == com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.getDefaultInstance()) return this;
if (!other.getAccountGroupId().isEmpty()) {
accountGroupId_ = other.accountGroupId_;
onChanged();
}
if (other.category_ != 0) {
setCategoryValue(other.getCategoryValue());
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object accountGroupId_ = "";
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public java.lang.String getAccountGroupId() {
java.lang.Object ref = accountGroupId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountGroupId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public com.google.protobuf.ByteString
getAccountGroupIdBytes() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountGroupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public Builder setAccountGroupId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accountGroupId_ = value;
onChanged();
return this;
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public Builder clearAccountGroupId() {
accountGroupId_ = getDefaultInstance().getAccountGroupId();
onChanged();
return this;
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public Builder setAccountGroupIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accountGroupId_ = value;
onChanged();
return this;
}
private int category_ = 0;
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public int getCategoryValue() {
return category_;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public Builder setCategoryValue(int value) {
category_ = value;
onChanged();
return this;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory() {
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory result = com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.valueOf(category_);
return result == null ? com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.UNRECOGNIZED : result;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public Builder setCategory(com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory value) {
if (value == null) {
throw new NullPointerException();
}
category_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public Builder clearCategory() {
category_ = 0;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.UserActionsRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.UserActionsRequest)
private static final com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest();
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public UserActionsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UserActionsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UploadUserActionForCustomerIDRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.UploadUserActionForCustomerIDRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
java.lang.String getCustomerId();
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
com.google.protobuf.ByteString
getCustomerIdBytes();
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
java.lang.String getPartnerId();
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
com.google.protobuf.ByteString
getPartnerIdBytes();
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
int getCategoryValue();
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory();
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
java.lang.String getDescription();
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
* Protobuf type {@code sales.v1.UploadUserActionForCustomerIDRequest}
*/
public static final class UploadUserActionForCustomerIDRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.UploadUserActionForCustomerIDRequest)
UploadUserActionForCustomerIDRequestOrBuilder {
// Use UploadUserActionForCustomerIDRequest.newBuilder() to construct.
private UploadUserActionForCustomerIDRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UploadUserActionForCustomerIDRequest() {
customerId_ = "";
partnerId_ = "";
category_ = 0;
description_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private UploadUserActionForCustomerIDRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
customerId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
partnerId_ = s;
break;
}
case 24: {
int rawValue = input.readEnum();
category_ = rawValue;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UploadUserActionForCustomerIDRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UploadUserActionForCustomerIDRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest.class, com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest.Builder.class);
}
public static final int CUSTOMER_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object customerId_;
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
public java.lang.String getCustomerId() {
java.lang.Object ref = customerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerId_ = s;
return s;
}
}
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
public com.google.protobuf.ByteString
getCustomerIdBytes() {
java.lang.Object ref = customerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PARTNER_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object partnerId_;
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
}
}
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CATEGORY_FIELD_NUMBER = 3;
private int category_;
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
public int getCategoryValue() {
return category_;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory() {
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory result = com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.valueOf(category_);
return result == null ? com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.UNRECOGNIZED : result;
}
public static final int DESCRIPTION_FIELD_NUMBER = 4;
private volatile java.lang.Object description_;
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getCustomerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, customerId_);
}
if (!getPartnerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, partnerId_);
}
if (category_ != com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.ACTION_CATEGORY_UNKNOWN.getNumber()) {
output.writeEnum(3, category_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, description_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getCustomerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, customerId_);
}
if (!getPartnerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, partnerId_);
}
if (category_ != com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.ACTION_CATEGORY_UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, category_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, description_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest other = (com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest) obj;
boolean result = true;
result = result && getCustomerId()
.equals(other.getCustomerId());
result = result && getPartnerId()
.equals(other.getPartnerId());
result = result && category_ == other.category_;
result = result && getDescription()
.equals(other.getDescription());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CUSTOMER_ID_FIELD_NUMBER;
hash = (53 * hash) + getCustomerId().hashCode();
hash = (37 * hash) + PARTNER_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartnerId().hashCode();
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + category_;
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.UploadUserActionForCustomerIDRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.UploadUserActionForCustomerIDRequest)
com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UploadUserActionForCustomerIDRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UploadUserActionForCustomerIDRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest.class, com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
customerId_ = "";
partnerId_ = "";
category_ = 0;
description_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UploadUserActionForCustomerIDRequest_descriptor;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest build() {
com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest buildPartial() {
com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest result = new com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest(this);
result.customerId_ = customerId_;
result.partnerId_ = partnerId_;
result.category_ = category_;
result.description_ = description_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest other) {
if (other == com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest.getDefaultInstance()) return this;
if (!other.getCustomerId().isEmpty()) {
customerId_ = other.customerId_;
onChanged();
}
if (!other.getPartnerId().isEmpty()) {
partnerId_ = other.partnerId_;
onChanged();
}
if (other.category_ != 0) {
setCategoryValue(other.getCategoryValue());
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object customerId_ = "";
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
public java.lang.String getCustomerId() {
java.lang.Object ref = customerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
public com.google.protobuf.ByteString
getCustomerIdBytes() {
java.lang.Object ref = customerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
public Builder setCustomerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
customerId_ = value;
onChanged();
return this;
}
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
public Builder clearCustomerId() {
customerId_ = getDefaultInstance().getCustomerId();
onChanged();
return this;
}
/**
*
* The customer ID that the action is associated with
*
*
* string customer_id = 1;
*/
public Builder setCustomerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
customerId_ = value;
onChanged();
return this;
}
private java.lang.Object partnerId_ = "";
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
public java.lang.String getPartnerId() {
java.lang.Object ref = partnerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partnerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
public com.google.protobuf.ByteString
getPartnerIdBytes() {
java.lang.Object ref = partnerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partnerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
public Builder setPartnerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partnerId_ = value;
onChanged();
return this;
}
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
public Builder clearPartnerId() {
partnerId_ = getDefaultInstance().getPartnerId();
onChanged();
return this;
}
/**
*
* The partner ID that the customer ID belongs to
*
*
* string partner_id = 2;
*/
public Builder setPartnerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partnerId_ = value;
onChanged();
return this;
}
private int category_ = 0;
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
public int getCategoryValue() {
return category_;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
public Builder setCategoryValue(int value) {
category_ = value;
onChanged();
return this;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory() {
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory result = com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.valueOf(category_);
return result == null ? com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.UNRECOGNIZED : result;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
public Builder setCategory(com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory value) {
if (value == null) {
throw new NullPointerException();
}
category_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 3;
*/
public Builder clearCategory() {
category_ = 0;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* The description of the event that occurred
*
*
* string description = 4;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.UploadUserActionForCustomerIDRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.UploadUserActionForCustomerIDRequest)
private static final com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest();
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public UploadUserActionForCustomerIDRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UploadUserActionForCustomerIDRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UploadUserActionForCustomerIDRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetUserActionsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.GetUserActionsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
java.lang.String getAccountGroupId();
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
com.google.protobuf.ByteString
getAccountGroupIdBytes();
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
java.lang.String getCursor();
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
com.google.protobuf.ByteString
getCursorBytes();
/**
*
* The number of results to return.
*
*
* int64 page_size = 3;
*/
long getPageSize();
}
/**
* Protobuf type {@code sales.v1.GetUserActionsRequest}
*/
public static final class GetUserActionsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.GetUserActionsRequest)
GetUserActionsRequestOrBuilder {
// Use GetUserActionsRequest.newBuilder() to construct.
private GetUserActionsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetUserActionsRequest() {
accountGroupId_ = "";
cursor_ = "";
pageSize_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetUserActionsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
accountGroupId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
cursor_ = s;
break;
}
case 24: {
pageSize_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_GetUserActionsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_GetUserActionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest.class, com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest.Builder.class);
}
public static final int ACCOUNT_GROUP_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object accountGroupId_;
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public java.lang.String getAccountGroupId() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountGroupId_ = s;
return s;
}
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public com.google.protobuf.ByteString
getAccountGroupIdBytes() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountGroupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CURSOR_FIELD_NUMBER = 2;
private volatile java.lang.Object cursor_;
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
public java.lang.String getCursor() {
java.lang.Object ref = cursor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cursor_ = s;
return s;
}
}
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
public com.google.protobuf.ByteString
getCursorBytes() {
java.lang.Object ref = cursor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAGE_SIZE_FIELD_NUMBER = 3;
private long pageSize_;
/**
*
* The number of results to return.
*
*
* int64 page_size = 3;
*/
public long getPageSize() {
return pageSize_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getAccountGroupIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, accountGroupId_);
}
if (!getCursorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, cursor_);
}
if (pageSize_ != 0L) {
output.writeInt64(3, pageSize_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getAccountGroupIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, accountGroupId_);
}
if (!getCursorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, cursor_);
}
if (pageSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, pageSize_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest other = (com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest) obj;
boolean result = true;
result = result && getAccountGroupId()
.equals(other.getAccountGroupId());
result = result && getCursor()
.equals(other.getCursor());
result = result && (getPageSize()
== other.getPageSize());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ACCOUNT_GROUP_ID_FIELD_NUMBER;
hash = (53 * hash) + getAccountGroupId().hashCode();
hash = (37 * hash) + CURSOR_FIELD_NUMBER;
hash = (53 * hash) + getCursor().hashCode();
hash = (37 * hash) + PAGE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPageSize());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.GetUserActionsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.GetUserActionsRequest)
com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_GetUserActionsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_GetUserActionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest.class, com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
accountGroupId_ = "";
cursor_ = "";
pageSize_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_GetUserActionsRequest_descriptor;
}
public com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest build() {
com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest buildPartial() {
com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest result = new com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest(this);
result.accountGroupId_ = accountGroupId_;
result.cursor_ = cursor_;
result.pageSize_ = pageSize_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest other) {
if (other == com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest.getDefaultInstance()) return this;
if (!other.getAccountGroupId().isEmpty()) {
accountGroupId_ = other.accountGroupId_;
onChanged();
}
if (!other.getCursor().isEmpty()) {
cursor_ = other.cursor_;
onChanged();
}
if (other.getPageSize() != 0L) {
setPageSize(other.getPageSize());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object accountGroupId_ = "";
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public java.lang.String getAccountGroupId() {
java.lang.Object ref = accountGroupId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountGroupId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public com.google.protobuf.ByteString
getAccountGroupIdBytes() {
java.lang.Object ref = accountGroupId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountGroupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public Builder setAccountGroupId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accountGroupId_ = value;
onChanged();
return this;
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public Builder clearAccountGroupId() {
accountGroupId_ = getDefaultInstance().getAccountGroupId();
onChanged();
return this;
}
/**
*
* The account group that the action is associated with
*
*
* string account_group_id = 1;
*/
public Builder setAccountGroupIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accountGroupId_ = value;
onChanged();
return this;
}
private java.lang.Object cursor_ = "";
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
public java.lang.String getCursor() {
java.lang.Object ref = cursor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cursor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
public com.google.protobuf.ByteString
getCursorBytes() {
java.lang.Object ref = cursor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cursor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
public Builder setCursor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cursor_ = value;
onChanged();
return this;
}
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
public Builder clearCursor() {
cursor_ = getDefaultInstance().getCursor();
onChanged();
return this;
}
/**
*
* Cursor allows paging of results, the next_cursor returned by the last response is expected.
*
*
* string cursor = 2;
*/
public Builder setCursorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cursor_ = value;
onChanged();
return this;
}
private long pageSize_ ;
/**
*
* The number of results to return.
*
*
* int64 page_size = 3;
*/
public long getPageSize() {
return pageSize_;
}
/**
*
* The number of results to return.
*
*
* int64 page_size = 3;
*/
public Builder setPageSize(long value) {
pageSize_ = value;
onChanged();
return this;
}
/**
*
* The number of results to return.
*
*
* int64 page_size = 3;
*/
public Builder clearPageSize() {
pageSize_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.GetUserActionsRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.GetUserActionsRequest)
private static final com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest();
}
public static com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetUserActionsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetUserActionsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.UserActionsProto.GetUserActionsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UserActionOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.UserAction)
com.google.protobuf.MessageOrBuilder {
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
boolean hasCreated();
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
com.google.protobuf.Timestamp getCreated();
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
com.google.protobuf.TimestampOrBuilder getCreatedOrBuilder();
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
int getCategoryValue();
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory();
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
java.lang.String getDescription();
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
* Protobuf type {@code sales.v1.UserAction}
*/
public static final class UserAction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.UserAction)
UserActionOrBuilder {
// Use UserAction.newBuilder() to construct.
private UserAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserAction() {
category_ = 0;
description_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private UserAction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (created_ != null) {
subBuilder = created_.toBuilder();
}
created_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(created_);
created_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
category_ = rawValue;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserAction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UserAction.class, com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder.class);
}
public static final int CREATED_FIELD_NUMBER = 1;
private com.google.protobuf.Timestamp created_;
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public boolean hasCreated() {
return created_ != null;
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public com.google.protobuf.Timestamp getCreated() {
return created_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : created_;
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public com.google.protobuf.TimestampOrBuilder getCreatedOrBuilder() {
return getCreated();
}
public static final int CATEGORY_FIELD_NUMBER = 2;
private int category_;
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public int getCategoryValue() {
return category_;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory() {
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory result = com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.valueOf(category_);
return result == null ? com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.UNRECOGNIZED : result;
}
public static final int DESCRIPTION_FIELD_NUMBER = 3;
private volatile java.lang.Object description_;
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (created_ != null) {
output.writeMessage(1, getCreated());
}
if (category_ != com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.ACTION_CATEGORY_UNKNOWN.getNumber()) {
output.writeEnum(2, category_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, description_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (created_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getCreated());
}
if (category_ != com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.ACTION_CATEGORY_UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, category_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, description_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.UserActionsProto.UserAction)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.UserActionsProto.UserAction other = (com.vendasta.sales.v1.generated.UserActionsProto.UserAction) obj;
boolean result = true;
result = result && (hasCreated() == other.hasCreated());
if (hasCreated()) {
result = result && getCreated()
.equals(other.getCreated());
}
result = result && category_ == other.category_;
result = result && getDescription()
.equals(other.getDescription());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCreated()) {
hash = (37 * hash) + CREATED_FIELD_NUMBER;
hash = (53 * hash) + getCreated().hashCode();
}
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + category_;
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.UserActionsProto.UserAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.UserAction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.UserAction)
com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserAction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UserAction.class, com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.UserActionsProto.UserAction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (createdBuilder_ == null) {
created_ = null;
} else {
created_ = null;
createdBuilder_ = null;
}
category_ = 0;
description_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserAction_descriptor;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.UserAction.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction build() {
com.vendasta.sales.v1.generated.UserActionsProto.UserAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction buildPartial() {
com.vendasta.sales.v1.generated.UserActionsProto.UserAction result = new com.vendasta.sales.v1.generated.UserActionsProto.UserAction(this);
if (createdBuilder_ == null) {
result.created_ = created_;
} else {
result.created_ = createdBuilder_.build();
}
result.category_ = category_;
result.description_ = description_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.UserActionsProto.UserAction) {
return mergeFrom((com.vendasta.sales.v1.generated.UserActionsProto.UserAction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.UserActionsProto.UserAction other) {
if (other == com.vendasta.sales.v1.generated.UserActionsProto.UserAction.getDefaultInstance()) return this;
if (other.hasCreated()) {
mergeCreated(other.getCreated());
}
if (other.category_ != 0) {
setCategoryValue(other.getCategoryValue());
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.UserActionsProto.UserAction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.UserActionsProto.UserAction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.Timestamp created_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> createdBuilder_;
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public boolean hasCreated() {
return createdBuilder_ != null || created_ != null;
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public com.google.protobuf.Timestamp getCreated() {
if (createdBuilder_ == null) {
return created_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : created_;
} else {
return createdBuilder_.getMessage();
}
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public Builder setCreated(com.google.protobuf.Timestamp value) {
if (createdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
created_ = value;
onChanged();
} else {
createdBuilder_.setMessage(value);
}
return this;
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public Builder setCreated(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (createdBuilder_ == null) {
created_ = builderForValue.build();
onChanged();
} else {
createdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public Builder mergeCreated(com.google.protobuf.Timestamp value) {
if (createdBuilder_ == null) {
if (created_ != null) {
created_ =
com.google.protobuf.Timestamp.newBuilder(created_).mergeFrom(value).buildPartial();
} else {
created_ = value;
}
onChanged();
} else {
createdBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public Builder clearCreated() {
if (createdBuilder_ == null) {
created_ = null;
onChanged();
} else {
created_ = null;
createdBuilder_ = null;
}
return this;
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public com.google.protobuf.Timestamp.Builder getCreatedBuilder() {
onChanged();
return getCreatedFieldBuilder().getBuilder();
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
public com.google.protobuf.TimestampOrBuilder getCreatedOrBuilder() {
if (createdBuilder_ != null) {
return createdBuilder_.getMessageOrBuilder();
} else {
return created_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : created_;
}
}
/**
*
* The account group that the action is associated with
*
*
* .google.protobuf.Timestamp created = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getCreatedFieldBuilder() {
if (createdBuilder_ == null) {
createdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getCreated(),
getParentForChildren(),
isClean());
created_ = null;
}
return createdBuilder_;
}
private int category_ = 0;
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public int getCategoryValue() {
return category_;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public Builder setCategoryValue(int value) {
category_ = value;
onChanged();
return this;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory getCategory() {
com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory result = com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.valueOf(category_);
return result == null ? com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory.UNRECOGNIZED : result;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public Builder setCategory(com.vendasta.sales.v1.generated.UserActionsProto.ActionCategory value) {
if (value == null) {
throw new NullPointerException();
}
category_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The category that the action is included in
*
*
* .sales.v1.ActionCategory category = 2;
*/
public Builder clearCategory() {
category_ = 0;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* The description of the event that occurred
*
*
* string description = 3;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.UserAction)
}
// @@protoc_insertion_point(class_scope:sales.v1.UserAction)
private static final com.vendasta.sales.v1.generated.UserActionsProto.UserAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.UserActionsProto.UserAction();
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public UserAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UserAction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UserActionsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.UserActionsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
java.util.List
getUserActionsList();
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
com.vendasta.sales.v1.generated.UserActionsProto.UserAction getUserActions(int index);
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
int getUserActionsCount();
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
java.util.List extends com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder>
getUserActionsOrBuilderList();
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder getUserActionsOrBuilder(
int index);
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
boolean hasPagingMetadata();
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata getPagingMetadata();
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadataOrBuilder getPagingMetadataOrBuilder();
}
/**
* Protobuf type {@code sales.v1.UserActionsResponse}
*/
public static final class UserActionsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.UserActionsResponse)
UserActionsResponseOrBuilder {
// Use UserActionsResponse.newBuilder() to construct.
private UserActionsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserActionsResponse() {
userActions_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private UserActionsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
userActions_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
userActions_.add(
input.readMessage(com.vendasta.sales.v1.generated.UserActionsProto.UserAction.parser(), extensionRegistry));
break;
}
case 18: {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.Builder subBuilder = null;
if (pagingMetadata_ != null) {
subBuilder = pagingMetadata_.toBuilder();
}
pagingMetadata_ = input.readMessage(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagingMetadata_);
pagingMetadata_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
userActions_ = java.util.Collections.unmodifiableList(userActions_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse.class, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse.Builder.class);
}
private int bitField0_;
public static final int USER_ACTIONS_FIELD_NUMBER = 1;
private java.util.List userActions_;
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public java.util.List getUserActionsList() {
return userActions_;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder>
getUserActionsOrBuilderList() {
return userActions_;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public int getUserActionsCount() {
return userActions_.size();
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction getUserActions(int index) {
return userActions_.get(index);
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder getUserActionsOrBuilder(
int index) {
return userActions_.get(index);
}
public static final int PAGING_METADATA_FIELD_NUMBER = 2;
private com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata pagingMetadata_;
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public boolean hasPagingMetadata() {
return pagingMetadata_ != null;
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata getPagingMetadata() {
return pagingMetadata_ == null ? com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadataOrBuilder getPagingMetadataOrBuilder() {
return getPagingMetadata();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < userActions_.size(); i++) {
output.writeMessage(1, userActions_.get(i));
}
if (pagingMetadata_ != null) {
output.writeMessage(2, getPagingMetadata());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < userActions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, userActions_.get(i));
}
if (pagingMetadata_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagingMetadata());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse other = (com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse) obj;
boolean result = true;
result = result && getUserActionsList()
.equals(other.getUserActionsList());
result = result && (hasPagingMetadata() == other.hasPagingMetadata());
if (hasPagingMetadata()) {
result = result && getPagingMetadata()
.equals(other.getPagingMetadata());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getUserActionsCount() > 0) {
hash = (37 * hash) + USER_ACTIONS_FIELD_NUMBER;
hash = (53 * hash) + getUserActionsList().hashCode();
}
if (hasPagingMetadata()) {
hash = (37 * hash) + PAGING_METADATA_FIELD_NUMBER;
hash = (53 * hash) + getPagingMetadata().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.UserActionsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.UserActionsResponse)
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse.class, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getUserActionsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (userActionsBuilder_ == null) {
userActions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
userActionsBuilder_.clear();
}
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = null;
} else {
pagingMetadata_ = null;
pagingMetadataBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_UserActionsResponse_descriptor;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse build() {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse buildPartial() {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse result = new com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (userActionsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
userActions_ = java.util.Collections.unmodifiableList(userActions_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.userActions_ = userActions_;
} else {
result.userActions_ = userActionsBuilder_.build();
}
if (pagingMetadataBuilder_ == null) {
result.pagingMetadata_ = pagingMetadata_;
} else {
result.pagingMetadata_ = pagingMetadataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse) {
return mergeFrom((com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse other) {
if (other == com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse.getDefaultInstance()) return this;
if (userActionsBuilder_ == null) {
if (!other.userActions_.isEmpty()) {
if (userActions_.isEmpty()) {
userActions_ = other.userActions_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureUserActionsIsMutable();
userActions_.addAll(other.userActions_);
}
onChanged();
}
} else {
if (!other.userActions_.isEmpty()) {
if (userActionsBuilder_.isEmpty()) {
userActionsBuilder_.dispose();
userActionsBuilder_ = null;
userActions_ = other.userActions_;
bitField0_ = (bitField0_ & ~0x00000001);
userActionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getUserActionsFieldBuilder() : null;
} else {
userActionsBuilder_.addAllMessages(other.userActions_);
}
}
}
if (other.hasPagingMetadata()) {
mergePagingMetadata(other.getPagingMetadata());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List userActions_ =
java.util.Collections.emptyList();
private void ensureUserActionsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
userActions_ = new java.util.ArrayList(userActions_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserAction, com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder> userActionsBuilder_;
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public java.util.List getUserActionsList() {
if (userActionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(userActions_);
} else {
return userActionsBuilder_.getMessageList();
}
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public int getUserActionsCount() {
if (userActionsBuilder_ == null) {
return userActions_.size();
} else {
return userActionsBuilder_.getCount();
}
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction getUserActions(int index) {
if (userActionsBuilder_ == null) {
return userActions_.get(index);
} else {
return userActionsBuilder_.getMessage(index);
}
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder setUserActions(
int index, com.vendasta.sales.v1.generated.UserActionsProto.UserAction value) {
if (userActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserActionsIsMutable();
userActions_.set(index, value);
onChanged();
} else {
userActionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder setUserActions(
int index, com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder builderForValue) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
userActions_.set(index, builderForValue.build());
onChanged();
} else {
userActionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder addUserActions(com.vendasta.sales.v1.generated.UserActionsProto.UserAction value) {
if (userActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserActionsIsMutable();
userActions_.add(value);
onChanged();
} else {
userActionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder addUserActions(
int index, com.vendasta.sales.v1.generated.UserActionsProto.UserAction value) {
if (userActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserActionsIsMutable();
userActions_.add(index, value);
onChanged();
} else {
userActionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder addUserActions(
com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder builderForValue) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
userActions_.add(builderForValue.build());
onChanged();
} else {
userActionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder addUserActions(
int index, com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder builderForValue) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
userActions_.add(index, builderForValue.build());
onChanged();
} else {
userActionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder addAllUserActions(
java.lang.Iterable extends com.vendasta.sales.v1.generated.UserActionsProto.UserAction> values) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, userActions_);
onChanged();
} else {
userActionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder clearUserActions() {
if (userActionsBuilder_ == null) {
userActions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
userActionsBuilder_.clear();
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public Builder removeUserActions(int index) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
userActions_.remove(index);
onChanged();
} else {
userActionsBuilder_.remove(index);
}
return this;
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder getUserActionsBuilder(
int index) {
return getUserActionsFieldBuilder().getBuilder(index);
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder getUserActionsOrBuilder(
int index) {
if (userActionsBuilder_ == null) {
return userActions_.get(index); } else {
return userActionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder>
getUserActionsOrBuilderList() {
if (userActionsBuilder_ != null) {
return userActionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(userActions_);
}
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder addUserActionsBuilder() {
return getUserActionsFieldBuilder().addBuilder(
com.vendasta.sales.v1.generated.UserActionsProto.UserAction.getDefaultInstance());
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder addUserActionsBuilder(
int index) {
return getUserActionsFieldBuilder().addBuilder(
index, com.vendasta.sales.v1.generated.UserActionsProto.UserAction.getDefaultInstance());
}
/**
*
* A collection of UserActions returned
*
*
* repeated .sales.v1.UserAction user_actions = 1;
*/
public java.util.List
getUserActionsBuilderList() {
return getUserActionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserAction, com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder>
getUserActionsFieldBuilder() {
if (userActionsBuilder_ == null) {
userActionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserAction, com.vendasta.sales.v1.generated.UserActionsProto.UserAction.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionOrBuilder>(
userActions_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
userActions_ = null;
}
return userActionsBuilder_;
}
private com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata pagingMetadata_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadataOrBuilder> pagingMetadataBuilder_;
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public boolean hasPagingMetadata() {
return pagingMetadataBuilder_ != null || pagingMetadata_ != null;
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata getPagingMetadata() {
if (pagingMetadataBuilder_ == null) {
return pagingMetadata_ == null ? com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
} else {
return pagingMetadataBuilder_.getMessage();
}
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public Builder setPagingMetadata(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata value) {
if (pagingMetadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagingMetadata_ = value;
onChanged();
} else {
pagingMetadataBuilder_.setMessage(value);
}
return this;
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public Builder setPagingMetadata(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.Builder builderForValue) {
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = builderForValue.build();
onChanged();
} else {
pagingMetadataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public Builder mergePagingMetadata(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata value) {
if (pagingMetadataBuilder_ == null) {
if (pagingMetadata_ != null) {
pagingMetadata_ =
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.newBuilder(pagingMetadata_).mergeFrom(value).buildPartial();
} else {
pagingMetadata_ = value;
}
onChanged();
} else {
pagingMetadataBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public Builder clearPagingMetadata() {
if (pagingMetadataBuilder_ == null) {
pagingMetadata_ = null;
onChanged();
} else {
pagingMetadata_ = null;
pagingMetadataBuilder_ = null;
}
return this;
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.Builder getPagingMetadataBuilder() {
onChanged();
return getPagingMetadataFieldBuilder().getBuilder();
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadataOrBuilder getPagingMetadataOrBuilder() {
if (pagingMetadataBuilder_ != null) {
return pagingMetadataBuilder_.getMessageOrBuilder();
} else {
return pagingMetadata_ == null ?
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.getDefaultInstance() : pagingMetadata_;
}
}
/**
*
* Contains paging data returned from the query
*
*
* .sales.v1.UserActionsPagedResponseMetadata paging_metadata = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadataOrBuilder>
getPagingMetadataFieldBuilder() {
if (pagingMetadataBuilder_ == null) {
pagingMetadataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadata.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsPagedResponseMetadataOrBuilder>(
getPagingMetadata(),
getParentForChildren(),
isClean());
pagingMetadata_ = null;
}
return pagingMetadataBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.UserActionsResponse)
}
// @@protoc_insertion_point(class_scope:sales.v1.UserActionsResponse)
private static final com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse();
}
public static com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public UserActionsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UserActionsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BulkUploadUserActionsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sales.v1.BulkUploadUserActionsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
java.util.List
getUserActionsList();
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest getUserActions(int index);
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
int getUserActionsCount();
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
java.util.List extends com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder>
getUserActionsOrBuilderList();
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder getUserActionsOrBuilder(
int index);
}
/**
* Protobuf type {@code sales.v1.BulkUploadUserActionsRequest}
*/
public static final class BulkUploadUserActionsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:sales.v1.BulkUploadUserActionsRequest)
BulkUploadUserActionsRequestOrBuilder {
// Use BulkUploadUserActionsRequest.newBuilder() to construct.
private BulkUploadUserActionsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BulkUploadUserActionsRequest() {
userActions_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private BulkUploadUserActionsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
userActions_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
userActions_.add(
input.readMessage(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
userActions_ = java.util.Collections.unmodifiableList(userActions_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_BulkUploadUserActionsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_BulkUploadUserActionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest.class, com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest.Builder.class);
}
public static final int USER_ACTIONS_FIELD_NUMBER = 1;
private java.util.List userActions_;
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public java.util.List getUserActionsList() {
return userActions_;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder>
getUserActionsOrBuilderList() {
return userActions_;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public int getUserActionsCount() {
return userActions_.size();
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest getUserActions(int index) {
return userActions_.get(index);
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder getUserActionsOrBuilder(
int index) {
return userActions_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < userActions_.size(); i++) {
output.writeMessage(1, userActions_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < userActions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, userActions_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest)) {
return super.equals(obj);
}
com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest other = (com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest) obj;
boolean result = true;
result = result && getUserActionsList()
.equals(other.getUserActionsList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getUserActionsCount() > 0) {
hash = (37 * hash) + USER_ACTIONS_FIELD_NUMBER;
hash = (53 * hash) + getUserActionsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sales.v1.BulkUploadUserActionsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:sales.v1.BulkUploadUserActionsRequest)
com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_BulkUploadUserActionsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_BulkUploadUserActionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest.class, com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest.Builder.class);
}
// Construct using com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getUserActionsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (userActionsBuilder_ == null) {
userActions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
userActionsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.internal_static_sales_v1_BulkUploadUserActionsRequest_descriptor;
}
public com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest getDefaultInstanceForType() {
return com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest.getDefaultInstance();
}
public com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest build() {
com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest buildPartial() {
com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest result = new com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest(this);
int from_bitField0_ = bitField0_;
if (userActionsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
userActions_ = java.util.Collections.unmodifiableList(userActions_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.userActions_ = userActions_;
} else {
result.userActions_ = userActionsBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest) {
return mergeFrom((com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest other) {
if (other == com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest.getDefaultInstance()) return this;
if (userActionsBuilder_ == null) {
if (!other.userActions_.isEmpty()) {
if (userActions_.isEmpty()) {
userActions_ = other.userActions_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureUserActionsIsMutable();
userActions_.addAll(other.userActions_);
}
onChanged();
}
} else {
if (!other.userActions_.isEmpty()) {
if (userActionsBuilder_.isEmpty()) {
userActionsBuilder_.dispose();
userActionsBuilder_ = null;
userActions_ = other.userActions_;
bitField0_ = (bitField0_ & ~0x00000001);
userActionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getUserActionsFieldBuilder() : null;
} else {
userActionsBuilder_.addAllMessages(other.userActions_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List userActions_ =
java.util.Collections.emptyList();
private void ensureUserActionsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
userActions_ = new java.util.ArrayList(userActions_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder> userActionsBuilder_;
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public java.util.List getUserActionsList() {
if (userActionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(userActions_);
} else {
return userActionsBuilder_.getMessageList();
}
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public int getUserActionsCount() {
if (userActionsBuilder_ == null) {
return userActions_.size();
} else {
return userActionsBuilder_.getCount();
}
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest getUserActions(int index) {
if (userActionsBuilder_ == null) {
return userActions_.get(index);
} else {
return userActionsBuilder_.getMessage(index);
}
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder setUserActions(
int index, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest value) {
if (userActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserActionsIsMutable();
userActions_.set(index, value);
onChanged();
} else {
userActionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder setUserActions(
int index, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder builderForValue) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
userActions_.set(index, builderForValue.build());
onChanged();
} else {
userActionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder addUserActions(com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest value) {
if (userActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserActionsIsMutable();
userActions_.add(value);
onChanged();
} else {
userActionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder addUserActions(
int index, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest value) {
if (userActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserActionsIsMutable();
userActions_.add(index, value);
onChanged();
} else {
userActionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder addUserActions(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder builderForValue) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
userActions_.add(builderForValue.build());
onChanged();
} else {
userActionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder addUserActions(
int index, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder builderForValue) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
userActions_.add(index, builderForValue.build());
onChanged();
} else {
userActionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder addAllUserActions(
java.lang.Iterable extends com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest> values) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, userActions_);
onChanged();
} else {
userActionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder clearUserActions() {
if (userActionsBuilder_ == null) {
userActions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
userActionsBuilder_.clear();
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public Builder removeUserActions(int index) {
if (userActionsBuilder_ == null) {
ensureUserActionsIsMutable();
userActions_.remove(index);
onChanged();
} else {
userActionsBuilder_.remove(index);
}
return this;
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder getUserActionsBuilder(
int index) {
return getUserActionsFieldBuilder().getBuilder(index);
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder getUserActionsOrBuilder(
int index) {
if (userActionsBuilder_ == null) {
return userActions_.get(index); } else {
return userActionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public java.util.List extends com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder>
getUserActionsOrBuilderList() {
if (userActionsBuilder_ != null) {
return userActionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(userActions_);
}
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder addUserActionsBuilder() {
return getUserActionsFieldBuilder().addBuilder(
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.getDefaultInstance());
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder addUserActionsBuilder(
int index) {
return getUserActionsFieldBuilder().addBuilder(
index, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.getDefaultInstance());
}
/**
*
* An array of user actions
*
*
* repeated .sales.v1.UserActionsRequest user_actions = 1;
*/
public java.util.List
getUserActionsBuilderList() {
return getUserActionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder>
getUserActionsFieldBuilder() {
if (userActionsBuilder_ == null) {
userActionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequest.Builder, com.vendasta.sales.v1.generated.UserActionsProto.UserActionsRequestOrBuilder>(
userActions_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
userActions_ = null;
}
return userActionsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:sales.v1.BulkUploadUserActionsRequest)
}
// @@protoc_insertion_point(class_scope:sales.v1.BulkUploadUserActionsRequest)
private static final com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest();
}
public static com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public BulkUploadUserActionsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BulkUploadUserActionsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.vendasta.sales.v1.generated.UserActionsProto.BulkUploadUserActionsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_UserActionsPagedResponseMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_UserActionsPagedResponseMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_UserActionsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_UserActionsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_UploadUserActionForCustomerIDRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_UploadUserActionForCustomerIDRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_GetUserActionsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_GetUserActionsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_UserAction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_UserAction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_UserActionsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_UserActionsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_sales_v1_BulkUploadUserActionsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_sales_v1_BulkUploadUserActionsRequest_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\033sales/v1/user_actions.proto\022\010sales.v1\032" +
"\037google/protobuf/timestamp.proto\"`\n User" +
"ActionsPagedResponseMetadata\022\023\n\013next_cur" +
"sor\030\001 \001(\t\022\020\n\010has_more\030\002 \001(\010\022\025\n\rtotal_res" +
"ults\030\003 \001(\003\"o\n\022UserActionsRequest\022\030\n\020acco" +
"unt_group_id\030\001 \001(\t\022*\n\010category\030\002 \001(\0162\030.s" +
"ales.v1.ActionCategory\022\023\n\013description\030\003 " +
"\001(\t\"\220\001\n$UploadUserActionForCustomerIDReq" +
"uest\022\023\n\013customer_id\030\001 \001(\t\022\022\n\npartner_id\030" +
"\002 \001(\t\022*\n\010category\030\003 \001(\0162\030.sales.v1.Actio",
"nCategory\022\023\n\013description\030\004 \001(\t\"T\n\025GetUse" +
"rActionsRequest\022\030\n\020account_group_id\030\001 \001(" +
"\t\022\016\n\006cursor\030\002 \001(\t\022\021\n\tpage_size\030\003 \001(\003\"z\n\n" +
"UserAction\022+\n\007created\030\001 \001(\0132\032.google.pro" +
"tobuf.Timestamp\022*\n\010category\030\002 \001(\0162\030.sale" +
"s.v1.ActionCategory\022\023\n\013description\030\003 \001(\t" +
"\"\206\001\n\023UserActionsResponse\022*\n\014user_actions" +
"\030\001 \003(\0132\024.sales.v1.UserAction\022C\n\017paging_m" +
"etadata\030\002 \001(\0132*.sales.v1.UserActionsPage" +
"dResponseMetadata\"R\n\034BulkUploadUserActio",
"nsRequest\0222\n\014user_actions\030\001 \003(\0132\034.sales." +
"v1.UserActionsRequest*\333\001\n\016ActionCategory" +
"\022\033\n\027ACTION_CATEGORY_UNKNOWN\020\000\022\031\n\025ACTION_" +
"CATEGORY_CLICK\020\001\022\030\n\024ACTION_CATEGORY_VIEW" +
"\020\002\022\032\n\026ACTION_CATEGORY_LINGER\020\003\022\031\n\025ACTION" +
"_CATEGORY_TYPED\020\004\022\035\n\031ACTION_CATEGORY_PUR" +
"CHASED\020\005\022!\n\035ACTION_CATEGORY_ADDED_TO_CAR" +
"T\020\006Bn\n\037com.vendasta.sales.v1.generatedB\020" +
"UserActionsProtoZ9github.com/vendasta/ge" +
"nerated-protos-go/sales/v1;sales/v1b\006pro",
"to3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.TimestampProto.getDescriptor(),
}, assigner);
internal_static_sales_v1_UserActionsPagedResponseMetadata_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_sales_v1_UserActionsPagedResponseMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_UserActionsPagedResponseMetadata_descriptor,
new java.lang.String[] { "NextCursor", "HasMore", "TotalResults", });
internal_static_sales_v1_UserActionsRequest_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_sales_v1_UserActionsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_UserActionsRequest_descriptor,
new java.lang.String[] { "AccountGroupId", "Category", "Description", });
internal_static_sales_v1_UploadUserActionForCustomerIDRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_sales_v1_UploadUserActionForCustomerIDRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_UploadUserActionForCustomerIDRequest_descriptor,
new java.lang.String[] { "CustomerId", "PartnerId", "Category", "Description", });
internal_static_sales_v1_GetUserActionsRequest_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_sales_v1_GetUserActionsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_GetUserActionsRequest_descriptor,
new java.lang.String[] { "AccountGroupId", "Cursor", "PageSize", });
internal_static_sales_v1_UserAction_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_sales_v1_UserAction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_UserAction_descriptor,
new java.lang.String[] { "Created", "Category", "Description", });
internal_static_sales_v1_UserActionsResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_sales_v1_UserActionsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_UserActionsResponse_descriptor,
new java.lang.String[] { "UserActions", "PagingMetadata", });
internal_static_sales_v1_BulkUploadUserActionsRequest_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_sales_v1_BulkUploadUserActionsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_sales_v1_BulkUploadUserActionsRequest_descriptor,
new java.lang.String[] { "UserActions", });
com.google.protobuf.TimestampProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy