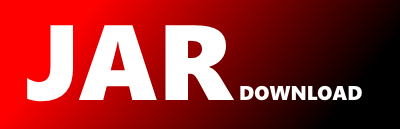
com.vendasta.sales.v1.internal.AutoAssignCriteria Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.ApiProto;
/**
*
**/
public final class AutoAssignCriteria {
/**
* Matches accounts based on their geographical location.
**/
public static final class LocationCriteria {
private final String country;
private final String state;
private final String city;
private LocationCriteria (
final String country,
final String state,
final String city)
{
this.country = country;
this.state = state;
this.city = city;
}
/**
*
* @return The final value of country on the object
**/
public String getCountry() {
return this.country;
}
/**
*
* @return The final value of state on the object
**/
public String getState() {
return this.state;
}
/**
*
* @return The final value of city on the object
**/
public String getCity() {
return this.city;
}
public static class Builder {
private String country;
private String state;
private String city;
public Builder() {
this.country = "";
this.state = "";
this.city = "";
}
/**
* Adds a value to the builder for country
* @param country Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCountry(String country) {
this.country = country;
return this;
}
/**
* Adds a value to the builder for state
* @param state Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setState(String state) {
this.state = state;
return this;
}
/**
* Adds a value to the builder for city
* @param city Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCity(String city) {
this.city = city;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the LocationCriteria class
* @return The instantiated final LocationCriteria
**/
public LocationCriteria build() {
return new LocationCriteria(
this.country,
this.state,
this.city);
}
}
/**
* Returns a Builder for LocationCriteria, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable LocationCriteria object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the LocationCriteria instance
**/
public String toString() {
String result = "LocationCriteria\n";
result += "-> country: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.country).split("\n"))) + "\n";
result += "-> state: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.state).split("\n"))) + "\n";
result += "-> city: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.city).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* LocationCriteria, which is much more usable.
* @return An instance of LocationCriteria representing the input proto object
**/
public static LocationCriteria fromProto(ApiProto.AutoAssignCriteria.LocationCriteria proto) {
LocationCriteria out = null;
if (proto != null) {
LocationCriteria.Builder outBuilder = LocationCriteria.newBuilder()
.setCountry(proto.getCountry())
.setState(proto.getState())
.setCity(proto.getCity());
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of LocationCriteria instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(ApiProto.AutoAssignCriteria.LocationCriteria proto : protos) {
out.add(LocationCriteria.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of ApiProto.AutoAssignCriteria.LocationCriteria which is a proto object ready for wire transmission
**/
public ApiProto.AutoAssignCriteria.LocationCriteria toProto() {
LocationCriteria obj = this;
ApiProto.AutoAssignCriteria.LocationCriteria.Builder outBuilder = ApiProto.AutoAssignCriteria.LocationCriteria.newBuilder();
outBuilder.setCountry(obj.getCountry());
outBuilder.setState(obj.getState());
outBuilder.setCity(obj.getCity());
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of ApiProto.AutoAssignCriteria.LocationCriteria instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (LocationCriteria obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
/**
* Matches accounts based on the business categories (also known as the business taxonomy).
**/
public static final class CategoryCriteria {
private final List categories;
private CategoryCriteria (
final List categories)
{
this.categories = categories;
}
/**
*
* @return The final value of categories on the object
**/
public List getCategories() {
return this.categories;
}
public static class Builder {
private List categories;
public Builder() {
this.categories = new ArrayList();
}
/**
* Adds a value to the builder for categories
* @param categories Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCategories(List categories) {
this.categories = categories;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the CategoryCriteria class
* @return The instantiated final CategoryCriteria
**/
public CategoryCriteria build() {
return new CategoryCriteria(
this.categories);
}
}
/**
* Returns a Builder for CategoryCriteria, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable CategoryCriteria object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the CategoryCriteria instance
**/
public String toString() {
String result = "CategoryCriteria\n";
result += "-> categories: (List)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.categories).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* CategoryCriteria, which is much more usable.
* @return An instance of CategoryCriteria representing the input proto object
**/
public static CategoryCriteria fromProto(ApiProto.AutoAssignCriteria.CategoryCriteria proto) {
CategoryCriteria out = null;
if (proto != null) {
CategoryCriteria.Builder outBuilder = CategoryCriteria.newBuilder()
.setCategories(proto.getCategoriesList());
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of CategoryCriteria instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(ApiProto.AutoAssignCriteria.CategoryCriteria proto : protos) {
out.add(CategoryCriteria.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of ApiProto.AutoAssignCriteria.CategoryCriteria which is a proto object ready for wire transmission
**/
public ApiProto.AutoAssignCriteria.CategoryCriteria toProto() {
CategoryCriteria obj = this;
ApiProto.AutoAssignCriteria.CategoryCriteria.Builder outBuilder = ApiProto.AutoAssignCriteria.CategoryCriteria.newBuilder();
outBuilder.addAllCategories(obj.getCategories());
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of ApiProto.AutoAssignCriteria.CategoryCriteria instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (CategoryCriteria obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
/**
* Matches accounts based on the generic tags applied.
**/
public static final class TagCriteria {
private final List tags;
private final TagMatchType matchType;
private TagCriteria (
final List tags,
final TagMatchType matchType)
{
this.tags = tags;
this.matchType = matchType;
}
/**
*
* @return The final value of tags on the object
**/
public List getTags() {
return this.tags;
}
/**
*
* @return The final value of matchType on the object
**/
public TagMatchType getMatchType() {
return this.matchType;
}
public static class Builder {
private List tags;
private TagMatchType matchType;
public Builder() {
this.tags = new ArrayList();
this.matchType = null;
}
/**
* Adds a value to the builder for tags
* @param tags Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setTags(List tags) {
this.tags = tags;
return this;
}
/**
* Adds a value to the builder for matchType
* @param matchType Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setMatchType(TagMatchType matchType) {
this.matchType = matchType;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the TagCriteria class
* @return The instantiated final TagCriteria
**/
public TagCriteria build() {
return new TagCriteria(
this.tags,
this.matchType);
}
}
/**
* Returns a Builder for TagCriteria, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable TagCriteria object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the TagCriteria instance
**/
public String toString() {
String result = "TagCriteria\n";
result += "-> tags: (List)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.tags).split("\n"))) + "\n";
result += "-> matchType: (TagMatchType)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.matchType).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* TagCriteria, which is much more usable.
* @return An instance of TagCriteria representing the input proto object
**/
public static TagCriteria fromProto(ApiProto.AutoAssignCriteria.TagCriteria proto) {
TagCriteria out = null;
if (proto != null) {
TagCriteria.Builder outBuilder = TagCriteria.newBuilder()
.setTags(proto.getTagsList())
.setMatchType(TagMatchType.fromProto(proto.getMatchType()));
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of TagCriteria instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(ApiProto.AutoAssignCriteria.TagCriteria proto : protos) {
out.add(TagCriteria.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of ApiProto.AutoAssignCriteria.TagCriteria which is a proto object ready for wire transmission
**/
public ApiProto.AutoAssignCriteria.TagCriteria toProto() {
TagCriteria obj = this;
ApiProto.AutoAssignCriteria.TagCriteria.Builder outBuilder = ApiProto.AutoAssignCriteria.TagCriteria.newBuilder();
outBuilder.addAllTags(obj.getTags());
outBuilder.setMatchType(obj.getMatchType() != null?obj.getMatchType().toProto():null);
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of ApiProto.AutoAssignCriteria.TagCriteria instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (TagCriteria obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
/**
* Matches accounts based on the conversion_point custom field applied. (VMF Only as of Sep 2019)
**/
public static final class ConversionPointCriteria {
private final List conversionPoint;
private ConversionPointCriteria (
final List conversionPoint)
{
this.conversionPoint = conversionPoint;
}
/**
*
* @return The final value of conversionPoint on the object
**/
public List getConversionPoint() {
return this.conversionPoint;
}
public static class Builder {
private List conversionPoint;
public Builder() {
this.conversionPoint = new ArrayList();
}
/**
* Adds a value to the builder for conversionPoint
* @param conversionPoint Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setConversionPoint(List conversionPoint) {
this.conversionPoint = conversionPoint;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the ConversionPointCriteria class
* @return The instantiated final ConversionPointCriteria
**/
public ConversionPointCriteria build() {
return new ConversionPointCriteria(
this.conversionPoint);
}
}
/**
* Returns a Builder for ConversionPointCriteria, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable ConversionPointCriteria object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the ConversionPointCriteria instance
**/
public String toString() {
String result = "ConversionPointCriteria\n";
result += "-> conversionPoint: (List)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.conversionPoint).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* ConversionPointCriteria, which is much more usable.
* @return An instance of ConversionPointCriteria representing the input proto object
**/
public static ConversionPointCriteria fromProto(ApiProto.AutoAssignCriteria.ConversionPointCriteria proto) {
ConversionPointCriteria out = null;
if (proto != null) {
ConversionPointCriteria.Builder outBuilder = ConversionPointCriteria.newBuilder()
.setConversionPoint(proto.getConversionPointList());
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of ConversionPointCriteria instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(ApiProto.AutoAssignCriteria.ConversionPointCriteria proto : protos) {
out.add(ConversionPointCriteria.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of ApiProto.AutoAssignCriteria.ConversionPointCriteria which is a proto object ready for wire transmission
**/
public ApiProto.AutoAssignCriteria.ConversionPointCriteria toProto() {
ConversionPointCriteria obj = this;
ApiProto.AutoAssignCriteria.ConversionPointCriteria.Builder outBuilder = ApiProto.AutoAssignCriteria.ConversionPointCriteria.newBuilder();
outBuilder.addAllConversionPoint(obj.getConversionPoint());
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of ApiProto.AutoAssignCriteria.ConversionPointCriteria instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (ConversionPointCriteria obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
private final AutoAssignCriteria.LocationCriteria locationCriteria;
private final AutoAssignCriteria.CategoryCriteria categoryCriteria;
private final AutoAssignCriteria.TagCriteria tagCriteria;
private final AutoAssignCriteria.ConversionPointCriteria conversionPointCriteria;
private AutoAssignCriteria (
final AutoAssignCriteria.LocationCriteria locationCriteria,
final AutoAssignCriteria.CategoryCriteria categoryCriteria,
final AutoAssignCriteria.TagCriteria tagCriteria,
final AutoAssignCriteria.ConversionPointCriteria conversionPointCriteria)
{
this.locationCriteria = locationCriteria;
this.categoryCriteria = categoryCriteria;
this.tagCriteria = tagCriteria;
this.conversionPointCriteria = conversionPointCriteria;
}
/**
*
* @return The final value of locationCriteria on the object
**/
public AutoAssignCriteria.LocationCriteria getLocationCriteria() {
return this.locationCriteria;
}
/**
*
* @return The final value of categoryCriteria on the object
**/
public AutoAssignCriteria.CategoryCriteria getCategoryCriteria() {
return this.categoryCriteria;
}
/**
*
* @return The final value of tagCriteria on the object
**/
public AutoAssignCriteria.TagCriteria getTagCriteria() {
return this.tagCriteria;
}
/**
*
* @return The final value of conversionPointCriteria on the object
**/
public AutoAssignCriteria.ConversionPointCriteria getConversionPointCriteria() {
return this.conversionPointCriteria;
}
public static class Builder {
private AutoAssignCriteria.LocationCriteria locationCriteria;
private AutoAssignCriteria.CategoryCriteria categoryCriteria;
private AutoAssignCriteria.TagCriteria tagCriteria;
private AutoAssignCriteria.ConversionPointCriteria conversionPointCriteria;
public Builder() {
this.locationCriteria = null;
this.categoryCriteria = null;
this.tagCriteria = null;
this.conversionPointCriteria = null;
}
/**
* Adds a value to the builder for locationCriteria
* @param locationCriteria Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setLocationCriteria(AutoAssignCriteria.LocationCriteria locationCriteria) {
this.locationCriteria = locationCriteria;
return this;
}
/**
* Adds a value to the builder for categoryCriteria
* @param categoryCriteria Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCategoryCriteria(AutoAssignCriteria.CategoryCriteria categoryCriteria) {
this.categoryCriteria = categoryCriteria;
return this;
}
/**
* Adds a value to the builder for tagCriteria
* @param tagCriteria Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setTagCriteria(AutoAssignCriteria.TagCriteria tagCriteria) {
this.tagCriteria = tagCriteria;
return this;
}
/**
* Adds a value to the builder for conversionPointCriteria
* @param conversionPointCriteria Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setConversionPointCriteria(AutoAssignCriteria.ConversionPointCriteria conversionPointCriteria) {
this.conversionPointCriteria = conversionPointCriteria;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the AutoAssignCriteria class
* @return The instantiated final AutoAssignCriteria
**/
public AutoAssignCriteria build() {
return new AutoAssignCriteria(
this.locationCriteria,
this.categoryCriteria,
this.tagCriteria,
this.conversionPointCriteria);
}
}
/**
* Returns a Builder for AutoAssignCriteria, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable AutoAssignCriteria object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the AutoAssignCriteria instance
**/
public String toString() {
String result = "AutoAssignCriteria\n";
result += "-> locationCriteria: (AutoAssignCriteria.LocationCriteria)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.locationCriteria).split("\n"))) + "\n";
result += "-> categoryCriteria: (AutoAssignCriteria.CategoryCriteria)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.categoryCriteria).split("\n"))) + "\n";
result += "-> tagCriteria: (AutoAssignCriteria.TagCriteria)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.tagCriteria).split("\n"))) + "\n";
result += "-> conversionPointCriteria: (AutoAssignCriteria.ConversionPointCriteria)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.conversionPointCriteria).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* AutoAssignCriteria, which is much more usable.
* @return An instance of AutoAssignCriteria representing the input proto object
**/
public static AutoAssignCriteria fromProto(ApiProto.AutoAssignCriteria proto) {
AutoAssignCriteria out = null;
if (proto != null) {
AutoAssignCriteria.Builder outBuilder = AutoAssignCriteria.newBuilder()
.setLocationCriteria(AutoAssignCriteria.LocationCriteria.fromProto(proto.getLocationCriteria()))
.setCategoryCriteria(AutoAssignCriteria.CategoryCriteria.fromProto(proto.getCategoryCriteria()))
.setTagCriteria(AutoAssignCriteria.TagCriteria.fromProto(proto.getTagCriteria()))
.setConversionPointCriteria(AutoAssignCriteria.ConversionPointCriteria.fromProto(proto.getConversionPointCriteria()));
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of AutoAssignCriteria instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(ApiProto.AutoAssignCriteria proto : protos) {
out.add(AutoAssignCriteria.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of ApiProto.AutoAssignCriteria which is a proto object ready for wire transmission
**/
public ApiProto.AutoAssignCriteria toProto() {
AutoAssignCriteria obj = this;
ApiProto.AutoAssignCriteria.Builder outBuilder = ApiProto.AutoAssignCriteria.newBuilder();
if(obj.getLocationCriteria() != null){outBuilder.setLocationCriteria(obj.getLocationCriteria().toProto());}
if(obj.getCategoryCriteria() != null){outBuilder.setCategoryCriteria(obj.getCategoryCriteria().toProto());}
if(obj.getTagCriteria() != null){outBuilder.setTagCriteria(obj.getTagCriteria().toProto());}
if(obj.getConversionPointCriteria() != null){outBuilder.setConversionPointCriteria(obj.getConversionPointCriteria().toProto());}
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of ApiProto.AutoAssignCriteria instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (AutoAssignCriteria obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy