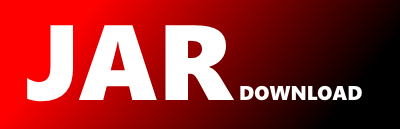
com.vendasta.sales.v1.internal.AutoAssignRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.ApiProto;
/**
*
**/
public final class AutoAssignRule {
private final List criteria;
private final List salespersonIds;
private final CriteriaMatchType criteriaMatchType;
private AutoAssignRule (
final List criteria,
final List salespersonIds,
final CriteriaMatchType criteriaMatchType)
{
this.criteria = criteria;
this.salespersonIds = salespersonIds;
this.criteriaMatchType = criteriaMatchType;
}
/**
* The criteria that defines what accounts match this rule.
* More than one criteria can be defined.
* The account must match all or any of them to be a match for the rule, depending on the criteria match type.
* @return The final value of criteria on the object
**/
public List getCriteria() {
return this.criteria;
}
/**
* The salespeople that are eligible to be assigned accounts that meet the criteria.
* @return The final value of salespersonIds on the object
**/
public List getSalespersonIds() {
return this.salespersonIds;
}
/**
* Controls whether we must match all ot any of the criteria, default behavior is to match all criteria.
* @return The final value of criteriaMatchType on the object
**/
public CriteriaMatchType getCriteriaMatchType() {
return this.criteriaMatchType;
}
public static class Builder {
private List criteria;
private List salespersonIds;
private CriteriaMatchType criteriaMatchType;
public Builder() {
this.criteria = null;
this.salespersonIds = new ArrayList();
this.criteriaMatchType = null;
}
/**
* Adds a value to the builder for criteria
* @param criteria Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCriteria(List criteria) {
this.criteria = criteria;
return this;
}
/**
* Adds a value to the builder for salespersonIds
* @param salespersonIds Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setSalespersonIds(List salespersonIds) {
this.salespersonIds = salespersonIds;
return this;
}
/**
* Adds a value to the builder for criteriaMatchType
* @param criteriaMatchType Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCriteriaMatchType(CriteriaMatchType criteriaMatchType) {
this.criteriaMatchType = criteriaMatchType;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the AutoAssignRule class
* @return The instantiated final AutoAssignRule
**/
public AutoAssignRule build() {
return new AutoAssignRule(
this.criteria,
this.salespersonIds,
this.criteriaMatchType);
}
}
/**
* Returns a Builder for AutoAssignRule, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable AutoAssignRule object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the AutoAssignRule instance
**/
public String toString() {
String result = "AutoAssignRule\n";
result += "-> criteria: (List)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.criteria).split("\n"))) + "\n";
result += "-> salespersonIds: (List)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.salespersonIds).split("\n"))) + "\n";
result += "-> criteriaMatchType: (CriteriaMatchType)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.criteriaMatchType).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* AutoAssignRule, which is much more usable.
* @return An instance of AutoAssignRule representing the input proto object
**/
public static AutoAssignRule fromProto(ApiProto.AutoAssignRule proto) {
AutoAssignRule out = null;
if (proto != null) {
AutoAssignRule.Builder outBuilder = AutoAssignRule.newBuilder()
.setCriteria(AutoAssignCriteria.fromProtos(proto.getCriteriaList()))
.setSalespersonIds(proto.getSalespersonIdsList())
.setCriteriaMatchType(CriteriaMatchType.fromProto(proto.getCriteriaMatchType()));
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of AutoAssignRule instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(ApiProto.AutoAssignRule proto : protos) {
out.add(AutoAssignRule.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of ApiProto.AutoAssignRule which is a proto object ready for wire transmission
**/
public ApiProto.AutoAssignRule toProto() {
AutoAssignRule obj = this;
ApiProto.AutoAssignRule.Builder outBuilder = ApiProto.AutoAssignRule.newBuilder();
outBuilder.addAllCriteria(AutoAssignCriteria.toProtos(obj.getCriteria()));
outBuilder.addAllSalespersonIds(obj.getSalespersonIds());
outBuilder.setCriteriaMatchType(obj.getCriteriaMatchType() != null?obj.getCriteriaMatchType().toProto():null);
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of ApiProto.AutoAssignRule instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (AutoAssignRule obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy