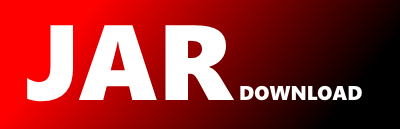
com.vendasta.sales.v1.internal.Business Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.BusinessProto;
/**
*
**/
public final class Business {
private final String partnerId;
private final String marketId;
private final String name;
private final String accountGroupId;
private final long hotness;
private final String city;
private final String zip;
private final String address;
private final String state;
private final String country;
private final String phoneNumber;
private final String salespersonId;
private final Date lastSalesActivityDate;
private final Date lastCustomerActivityDate;
private final String salesStatus;
private final boolean isRead;
private final String salesPersonAction;
private final Date latestSnapshotExpiry;
private final boolean snapshotOrCampaignEmailStatus;
private final String activityType;
private final Date lastConnectedDate;
private Business (
final String partnerId,
final String marketId,
final String name,
final String accountGroupId,
final long hotness,
final String city,
final String zip,
final String address,
final String state,
final String country,
final String phoneNumber,
final String salespersonId,
final Date lastSalesActivityDate,
final Date lastCustomerActivityDate,
final String salesStatus,
final boolean isRead,
final String salesPersonAction,
final Date latestSnapshotExpiry,
final boolean snapshotOrCampaignEmailStatus,
final String activityType,
final Date lastConnectedDate)
{
this.partnerId = partnerId;
this.marketId = marketId;
this.name = name;
this.accountGroupId = accountGroupId;
this.hotness = hotness;
this.city = city;
this.zip = zip;
this.address = address;
this.state = state;
this.country = country;
this.phoneNumber = phoneNumber;
this.salespersonId = salespersonId;
this.lastSalesActivityDate = lastSalesActivityDate;
this.lastCustomerActivityDate = lastCustomerActivityDate;
this.salesStatus = salesStatus;
this.isRead = isRead;
this.salesPersonAction = salesPersonAction;
this.latestSnapshotExpiry = latestSnapshotExpiry;
this.snapshotOrCampaignEmailStatus = snapshotOrCampaignEmailStatus;
this.activityType = activityType;
this.lastConnectedDate = lastConnectedDate;
}
/**
*
* @return The final value of partnerId on the object
**/
public String getPartnerId() {
return this.partnerId;
}
/**
*
* @return The final value of marketId on the object
**/
public String getMarketId() {
return this.marketId;
}
/**
*
* @return The final value of name on the object
**/
public String getName() {
return this.name;
}
/**
*
* @return The final value of accountGroupId on the object
**/
public String getAccountGroupId() {
return this.accountGroupId;
}
/**
*
* @return The final value of hotness on the object
**/
public long getHotness() {
return this.hotness;
}
/**
*
* @return The final value of city on the object
**/
public String getCity() {
return this.city;
}
/**
*
* @return The final value of zip on the object
**/
public String getZip() {
return this.zip;
}
/**
*
* @return The final value of address on the object
**/
public String getAddress() {
return this.address;
}
/**
*
* @return The final value of state on the object
**/
public String getState() {
return this.state;
}
/**
*
* @return The final value of country on the object
**/
public String getCountry() {
return this.country;
}
/**
*
* @return The final value of phoneNumber on the object
**/
public String getPhoneNumber() {
return this.phoneNumber;
}
/**
*
* @return The final value of salespersonId on the object
**/
public String getSalespersonId() {
return this.salespersonId;
}
/**
*
* @return The final value of lastSalesActivityDate on the object
**/
public Date getLastSalesActivityDate() {
return this.lastSalesActivityDate;
}
/**
*
* @return The final value of lastCustomerActivityDate on the object
**/
public Date getLastCustomerActivityDate() {
return this.lastCustomerActivityDate;
}
/**
*
* @return The final value of salesStatus on the object
**/
public String getSalesStatus() {
return this.salesStatus;
}
/**
*
* @return The final value of isRead on the object
**/
public boolean getIsRead() {
return this.isRead;
}
/**
*
* @return The final value of salesPersonAction on the object
**/
public String getSalesPersonAction() {
return this.salesPersonAction;
}
/**
*
* @return The final value of latestSnapshotExpiry on the object
**/
public Date getLatestSnapshotExpiry() {
return this.latestSnapshotExpiry;
}
/**
*
* @return The final value of snapshotOrCampaignEmailStatus on the object
**/
public boolean getSnapshotOrCampaignEmailStatus() {
return this.snapshotOrCampaignEmailStatus;
}
/**
*
* @return The final value of activityType on the object
**/
public String getActivityType() {
return this.activityType;
}
/**
*
* @return The final value of lastConnectedDate on the object
**/
public Date getLastConnectedDate() {
return this.lastConnectedDate;
}
public static class Builder {
private String partnerId;
private String marketId;
private String name;
private String accountGroupId;
private long hotness;
private String city;
private String zip;
private String address;
private String state;
private String country;
private String phoneNumber;
private String salespersonId;
private Date lastSalesActivityDate;
private Date lastCustomerActivityDate;
private String salesStatus;
private boolean isRead;
private String salesPersonAction;
private Date latestSnapshotExpiry;
private boolean snapshotOrCampaignEmailStatus;
private String activityType;
private Date lastConnectedDate;
public Builder() {
this.partnerId = "";
this.marketId = "";
this.name = "";
this.accountGroupId = "";
this.hotness = 0;
this.city = "";
this.zip = "";
this.address = "";
this.state = "";
this.country = "";
this.phoneNumber = "";
this.salespersonId = "";
this.lastSalesActivityDate = null;
this.lastCustomerActivityDate = null;
this.salesStatus = "";
this.isRead = false;
this.salesPersonAction = "";
this.latestSnapshotExpiry = null;
this.snapshotOrCampaignEmailStatus = false;
this.activityType = "";
this.lastConnectedDate = null;
}
/**
* Adds a value to the builder for partnerId
* @param partnerId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setPartnerId(String partnerId) {
this.partnerId = partnerId;
return this;
}
/**
* Adds a value to the builder for marketId
* @param marketId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setMarketId(String marketId) {
this.marketId = marketId;
return this;
}
/**
* Adds a value to the builder for name
* @param name Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Adds a value to the builder for accountGroupId
* @param accountGroupId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setAccountGroupId(String accountGroupId) {
this.accountGroupId = accountGroupId;
return this;
}
/**
* Adds a value to the builder for hotness
* @param hotness Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setHotness(long hotness) {
this.hotness = hotness;
return this;
}
/**
* Adds a value to the builder for city
* @param city Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCity(String city) {
this.city = city;
return this;
}
/**
* Adds a value to the builder for zip
* @param zip Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setZip(String zip) {
this.zip = zip;
return this;
}
/**
* Adds a value to the builder for address
* @param address Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setAddress(String address) {
this.address = address;
return this;
}
/**
* Adds a value to the builder for state
* @param state Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setState(String state) {
this.state = state;
return this;
}
/**
* Adds a value to the builder for country
* @param country Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCountry(String country) {
this.country = country;
return this;
}
/**
* Adds a value to the builder for phoneNumber
* @param phoneNumber Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* Adds a value to the builder for salespersonId
* @param salespersonId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setSalespersonId(String salespersonId) {
this.salespersonId = salespersonId;
return this;
}
/**
* Adds a value to the builder for lastSalesActivityDate
* @param lastSalesActivityDate Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setLastSalesActivityDate(Date lastSalesActivityDate) {
this.lastSalesActivityDate = lastSalesActivityDate;
return this;
}
/**
* Adds a value to the builder for lastCustomerActivityDate
* @param lastCustomerActivityDate Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setLastCustomerActivityDate(Date lastCustomerActivityDate) {
this.lastCustomerActivityDate = lastCustomerActivityDate;
return this;
}
/**
* Adds a value to the builder for salesStatus
* @param salesStatus Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setSalesStatus(String salesStatus) {
this.salesStatus = salesStatus;
return this;
}
/**
* Adds a value to the builder for isRead
* @param isRead Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setIsRead(boolean isRead) {
this.isRead = isRead;
return this;
}
/**
* Adds a value to the builder for salesPersonAction
* @param salesPersonAction Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setSalesPersonAction(String salesPersonAction) {
this.salesPersonAction = salesPersonAction;
return this;
}
/**
* Adds a value to the builder for latestSnapshotExpiry
* @param latestSnapshotExpiry Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setLatestSnapshotExpiry(Date latestSnapshotExpiry) {
this.latestSnapshotExpiry = latestSnapshotExpiry;
return this;
}
/**
* Adds a value to the builder for snapshotOrCampaignEmailStatus
* @param snapshotOrCampaignEmailStatus Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setSnapshotOrCampaignEmailStatus(boolean snapshotOrCampaignEmailStatus) {
this.snapshotOrCampaignEmailStatus = snapshotOrCampaignEmailStatus;
return this;
}
/**
* Adds a value to the builder for activityType
* @param activityType Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setActivityType(String activityType) {
this.activityType = activityType;
return this;
}
/**
* Adds a value to the builder for lastConnectedDate
* @param lastConnectedDate Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setLastConnectedDate(Date lastConnectedDate) {
this.lastConnectedDate = lastConnectedDate;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the Business class
* @return The instantiated final Business
**/
public Business build() {
return new Business(
this.partnerId,
this.marketId,
this.name,
this.accountGroupId,
this.hotness,
this.city,
this.zip,
this.address,
this.state,
this.country,
this.phoneNumber,
this.salespersonId,
this.lastSalesActivityDate,
this.lastCustomerActivityDate,
this.salesStatus,
this.isRead,
this.salesPersonAction,
this.latestSnapshotExpiry,
this.snapshotOrCampaignEmailStatus,
this.activityType,
this.lastConnectedDate);
}
}
/**
* Returns a Builder for Business, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable Business object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the Business instance
**/
public String toString() {
String result = "Business\n";
result += "-> partnerId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.partnerId).split("\n"))) + "\n";
result += "-> marketId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.marketId).split("\n"))) + "\n";
result += "-> name: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.name).split("\n"))) + "\n";
result += "-> accountGroupId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.accountGroupId).split("\n"))) + "\n";
result += "-> hotness: (long)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.hotness).split("\n"))) + "\n";
result += "-> city: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.city).split("\n"))) + "\n";
result += "-> zip: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.zip).split("\n"))) + "\n";
result += "-> address: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.address).split("\n"))) + "\n";
result += "-> state: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.state).split("\n"))) + "\n";
result += "-> country: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.country).split("\n"))) + "\n";
result += "-> phoneNumber: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.phoneNumber).split("\n"))) + "\n";
result += "-> salespersonId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.salespersonId).split("\n"))) + "\n";
result += "-> lastSalesActivityDate: (Date)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.lastSalesActivityDate).split("\n"))) + "\n";
result += "-> lastCustomerActivityDate: (Date)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.lastCustomerActivityDate).split("\n"))) + "\n";
result += "-> salesStatus: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.salesStatus).split("\n"))) + "\n";
result += "-> isRead: (boolean)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.isRead).split("\n"))) + "\n";
result += "-> salesPersonAction: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.salesPersonAction).split("\n"))) + "\n";
result += "-> latestSnapshotExpiry: (Date)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.latestSnapshotExpiry).split("\n"))) + "\n";
result += "-> snapshotOrCampaignEmailStatus: (boolean)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.snapshotOrCampaignEmailStatus).split("\n"))) + "\n";
result += "-> activityType: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.activityType).split("\n"))) + "\n";
result += "-> lastConnectedDate: (Date)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.lastConnectedDate).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* Business, which is much more usable.
* @return An instance of Business representing the input proto object
**/
public static Business fromProto(BusinessProto.Business proto) {
Business out = null;
if (proto != null) {
Business.Builder outBuilder = Business.newBuilder()
.setPartnerId(proto.getPartnerId())
.setMarketId(proto.getMarketId())
.setName(proto.getName())
.setAccountGroupId(proto.getAccountGroupId())
.setHotness(proto.getHotness())
.setCity(proto.getCity())
.setZip(proto.getZip())
.setAddress(proto.getAddress())
.setState(proto.getState())
.setCountry(proto.getCountry())
.setPhoneNumber(proto.getPhoneNumber())
.setSalespersonId(proto.getSalespersonId())
.setLastSalesActivityDate(proto.hasLastSalesActivityDate()?new Date(proto.getLastSalesActivityDate().getSeconds() * 1000):null)
.setLastCustomerActivityDate(proto.hasLastCustomerActivityDate()?new Date(proto.getLastCustomerActivityDate().getSeconds() * 1000):null)
.setSalesStatus(proto.getSalesStatus())
.setIsRead(proto.getIsRead())
.setSalesPersonAction(proto.getSalesPersonAction())
.setLatestSnapshotExpiry(proto.hasLatestSnapshotExpiry()?new Date(proto.getLatestSnapshotExpiry().getSeconds() * 1000):null)
.setSnapshotOrCampaignEmailStatus(proto.getSnapshotOrCampaignEmailStatus())
.setActivityType(proto.getActivityType())
.setLastConnectedDate(proto.hasLastConnectedDate()?new Date(proto.getLastConnectedDate().getSeconds() * 1000):null);
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of Business instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(BusinessProto.Business proto : protos) {
out.add(Business.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of BusinessProto.Business which is a proto object ready for wire transmission
**/
public BusinessProto.Business toProto() {
Business obj = this;
BusinessProto.Business.Builder outBuilder = BusinessProto.Business.newBuilder();
outBuilder.setPartnerId(obj.getPartnerId());
outBuilder.setMarketId(obj.getMarketId());
outBuilder.setName(obj.getName());
outBuilder.setAccountGroupId(obj.getAccountGroupId());
outBuilder.setHotness(obj.getHotness());
outBuilder.setCity(obj.getCity());
outBuilder.setZip(obj.getZip());
outBuilder.setAddress(obj.getAddress());
outBuilder.setState(obj.getState());
outBuilder.setCountry(obj.getCountry());
outBuilder.setPhoneNumber(obj.getPhoneNumber());
outBuilder.setSalespersonId(obj.getSalespersonId());
if(obj.getLastSalesActivityDate()!=null){outBuilder.setLastSalesActivityDate(com.google.protobuf.Timestamp.newBuilder().setSeconds(obj.getLastSalesActivityDate().getTime() / 1000).build());}
if(obj.getLastCustomerActivityDate()!=null){outBuilder.setLastCustomerActivityDate(com.google.protobuf.Timestamp.newBuilder().setSeconds(obj.getLastCustomerActivityDate().getTime() / 1000).build());}
outBuilder.setSalesStatus(obj.getSalesStatus());
outBuilder.setIsRead(obj.getIsRead());
outBuilder.setSalesPersonAction(obj.getSalesPersonAction());
if(obj.getLatestSnapshotExpiry()!=null){outBuilder.setLatestSnapshotExpiry(com.google.protobuf.Timestamp.newBuilder().setSeconds(obj.getLatestSnapshotExpiry().getTime() / 1000).build());}
outBuilder.setSnapshotOrCampaignEmailStatus(obj.getSnapshotOrCampaignEmailStatus());
outBuilder.setActivityType(obj.getActivityType());
if(obj.getLastConnectedDate()!=null){outBuilder.setLastConnectedDate(com.google.protobuf.Timestamp.newBuilder().setSeconds(obj.getLastConnectedDate().getTime() / 1000).build());}
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of BusinessProto.Business instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (Business obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy