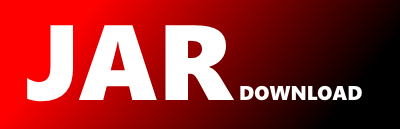
com.vendasta.sales.v1.internal.Duration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.WorkflowProto;
/**
*
**/
public final class Duration {
private final long value;
private final DurationPeriod duration;
private Duration (
final long value,
final DurationPeriod duration)
{
this.value = value;
this.duration = duration;
}
/**
* Value for duration of time of a given operation.
* @return The final value of value on the object
**/
public long getValue() {
return this.value;
}
/**
* Unit for measuring time.
* @return The final value of duration on the object
**/
public DurationPeriod getDuration() {
return this.duration;
}
public static class Builder {
private long value;
private DurationPeriod duration;
public Builder() {
this.value = 0;
this.duration = null;
}
/**
* Adds a value to the builder for value
* @param value Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setValue(long value) {
this.value = value;
return this;
}
/**
* Adds a value to the builder for duration
* @param duration Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setDuration(DurationPeriod duration) {
this.duration = duration;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the Duration class
* @return The instantiated final Duration
**/
public Duration build() {
return new Duration(
this.value,
this.duration);
}
}
/**
* Returns a Builder for Duration, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable Duration object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the Duration instance
**/
public String toString() {
String result = "Duration\n";
result += "-> value: (long)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.value).split("\n"))) + "\n";
result += "-> duration: (DurationPeriod)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.duration).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* Duration, which is much more usable.
* @return An instance of Duration representing the input proto object
**/
public static Duration fromProto(WorkflowProto.Duration proto) {
Duration out = null;
if (proto != null) {
Duration.Builder outBuilder = Duration.newBuilder()
.setValue(proto.getValue())
.setDuration(DurationPeriod.fromProto(proto.getDuration()));
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of Duration instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(WorkflowProto.Duration proto : protos) {
out.add(Duration.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of WorkflowProto.Duration which is a proto object ready for wire transmission
**/
public WorkflowProto.Duration toProto() {
Duration obj = this;
WorkflowProto.Duration.Builder outBuilder = WorkflowProto.Duration.newBuilder();
outBuilder.setValue(obj.getValue());
outBuilder.setDuration(obj.getDuration() != null?obj.getDuration().toProto():null);
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of WorkflowProto.Duration instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (Duration obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy