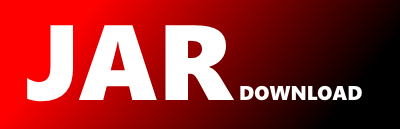
com.vendasta.sales.v1.internal.GetSalespersonRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.ApiProto;
/**
*
**/
public final class GetSalespersonRequest {
private final String partnerId;
private final String salespersonId;
private final String userId;
private GetSalespersonRequest (
final String partnerId,
final String salespersonId,
final String userId)
{
this.partnerId = partnerId;
this.salespersonId = salespersonId;
this.userId = userId;
}
/**
* partner_id is the namespace the salesperson is in
* @return The final value of partnerId on the object
**/
public String getPartnerId() {
return this.partnerId;
}
/**
* salesperson_id is the IAM SubjectID of the matching IAM Persona
* @return The final value of salespersonId on the object
**/
public String getSalespersonId() {
return this.salespersonId;
}
/**
* user_id is the IAM UserID the Subject belongs to
* @return The final value of userId on the object
**/
public String getUserId() {
return this.userId;
}
public static class Builder {
private String partnerId;
private String salespersonId;
private String userId;
public Builder() {
this.partnerId = "";
this.salespersonId = "";
this.userId = "";
}
/**
* Adds a value to the builder for partnerId
* @param partnerId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setPartnerId(String partnerId) {
this.partnerId = partnerId;
return this;
}
/**
* Adds a value to the builder for salespersonId
* @param salespersonId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setSalespersonId(String salespersonId) {
this.salespersonId = salespersonId;
return this;
}
/**
* Adds a value to the builder for userId
* @param userId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setUserId(String userId) {
this.userId = userId;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the GetSalespersonRequest class
* @return The instantiated final GetSalespersonRequest
**/
public GetSalespersonRequest build() {
return new GetSalespersonRequest(
this.partnerId,
this.salespersonId,
this.userId);
}
}
/**
* Returns a Builder for GetSalespersonRequest, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable GetSalespersonRequest object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the GetSalespersonRequest instance
**/
public String toString() {
String result = "GetSalespersonRequest\n";
result += "-> partnerId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.partnerId).split("\n"))) + "\n";
result += "-> salespersonId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.salespersonId).split("\n"))) + "\n";
result += "-> userId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.userId).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* GetSalespersonRequest, which is much more usable.
* @return An instance of GetSalespersonRequest representing the input proto object
**/
public static GetSalespersonRequest fromProto(ApiProto.GetSalespersonRequest proto) {
GetSalespersonRequest out = null;
if (proto != null) {
GetSalespersonRequest.Builder outBuilder = GetSalespersonRequest.newBuilder()
.setPartnerId(proto.getPartnerId())
.setSalespersonId(proto.getSalespersonId())
.setUserId(proto.getUserId());
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of GetSalespersonRequest instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(ApiProto.GetSalespersonRequest proto : protos) {
out.add(GetSalespersonRequest.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of ApiProto.GetSalespersonRequest which is a proto object ready for wire transmission
**/
public ApiProto.GetSalespersonRequest toProto() {
GetSalespersonRequest obj = this;
ApiProto.GetSalespersonRequest.Builder outBuilder = ApiProto.GetSalespersonRequest.newBuilder();
outBuilder.setPartnerId(obj.getPartnerId());
outBuilder.setSalespersonId(obj.getSalespersonId());
outBuilder.setUserId(obj.getUserId());
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of ApiProto.GetSalespersonRequest instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (GetSalespersonRequest obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy