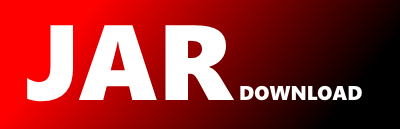
com.vendasta.sales.v1.internal.LineItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.WorkflowProto;
/**
*
**/
public final class LineItem {
/**
*
**/
public static final class AppKey {
private final String appId;
private final String editionId;
private AppKey (
final String appId,
final String editionId)
{
this.appId = appId;
this.editionId = editionId;
}
/**
*
* @return The final value of appId on the object
**/
public String getAppId() {
return this.appId;
}
/**
*
* @return The final value of editionId on the object
**/
public String getEditionId() {
return this.editionId;
}
public static class Builder {
private String appId;
private String editionId;
public Builder() {
this.appId = "";
this.editionId = "";
}
/**
* Adds a value to the builder for appId
* @param appId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setAppId(String appId) {
this.appId = appId;
return this;
}
/**
* Adds a value to the builder for editionId
* @param editionId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setEditionId(String editionId) {
this.editionId = editionId;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the AppKey class
* @return The instantiated final AppKey
**/
public AppKey build() {
return new AppKey(
this.appId,
this.editionId);
}
}
/**
* Returns a Builder for AppKey, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable AppKey object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the AppKey instance
**/
public String toString() {
String result = "AppKey\n";
result += "-> appId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.appId).split("\n"))) + "\n";
result += "-> editionId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.editionId).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* AppKey, which is much more usable.
* @return An instance of AppKey representing the input proto object
**/
public static AppKey fromProto(WorkflowProto.LineItem.AppKey proto) {
AppKey out = null;
if (proto != null) {
AppKey.Builder outBuilder = AppKey.newBuilder()
.setAppId(proto.getAppId())
.setEditionId(proto.getEditionId());
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of AppKey instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(WorkflowProto.LineItem.AppKey proto : protos) {
out.add(AppKey.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of WorkflowProto.LineItem.AppKey which is a proto object ready for wire transmission
**/
public WorkflowProto.LineItem.AppKey toProto() {
AppKey obj = this;
WorkflowProto.LineItem.AppKey.Builder outBuilder = WorkflowProto.LineItem.AppKey.newBuilder();
outBuilder.setAppId(obj.getAppId());
outBuilder.setEditionId(obj.getEditionId());
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of WorkflowProto.LineItem.AppKey instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (AppKey obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
private final LineItem.AppKey appKey;
private final String packageId;
private final Currency currency;
private final Revenue initialRevenue;
private final Revenue currentRevenue;
private final long quantity;
private final boolean isTrial;
private final String currencyCode;
private LineItem (
final LineItem.AppKey appKey,
final String packageId,
final Currency currency,
final Revenue initialRevenue,
final Revenue currentRevenue,
final long quantity,
final boolean isTrial,
final String currencyCode)
{
this.appKey = appKey;
this.packageId = packageId;
this.currency = currency;
this.initialRevenue = initialRevenue;
this.currentRevenue = currentRevenue;
this.quantity = quantity;
this.isTrial = isTrial;
this.currencyCode = currencyCode;
}
/**
*
* @return The final value of appKey on the object
**/
public LineItem.AppKey getAppKey() {
return this.appKey;
}
/**
*
* @return The final value of packageId on the object
**/
public String getPackageId() {
return this.packageId;
}
/**
* While this is not deprecated, more currencies than the value of the enum are supported. To use those currencies use currency_code instead
* @return The final value of currency on the object
**/
public Currency getCurrency() {
return this.currency;
}
/**
* DEPRECATED: not important now that there is a draft state for sales orders, history of the order should be used instead
* @return The final value of initialRevenue on the object
**/
public Revenue getInitialRevenue() {
return this.initialRevenue;
}
/**
*
* @return The final value of currentRevenue on the object
**/
public Revenue getCurrentRevenue() {
return this.currentRevenue;
}
/**
*
* @return The final value of quantity on the object
**/
public long getQuantity() {
return this.quantity;
}
/**
* Only used when AppKey is the identifier
* @return The final value of isTrial on the object
**/
public boolean getIsTrial() {
return this.isTrial;
}
/**
* The 3-letter currency code defined in ISO 4217.
* @return The final value of currencyCode on the object
**/
public String getCurrencyCode() {
return this.currencyCode;
}
public static class Builder {
private LineItem.AppKey appKey;
private String packageId;
private Currency currency;
private Revenue initialRevenue;
private Revenue currentRevenue;
private long quantity;
private boolean isTrial;
private String currencyCode;
public Builder() {
this.appKey = null;
this.packageId = "";
this.currency = null;
this.initialRevenue = null;
this.currentRevenue = null;
this.quantity = 0;
this.isTrial = false;
this.currencyCode = "";
}
/**
* Adds a value to the builder for appKey
* @param appKey Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setAppKey(LineItem.AppKey appKey) {
this.appKey = appKey;
return this;
}
/**
* Adds a value to the builder for packageId
* @param packageId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setPackageId(String packageId) {
this.packageId = packageId;
return this;
}
/**
* Adds a value to the builder for currency
* @param currency Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCurrency(Currency currency) {
this.currency = currency;
return this;
}
/**
* Adds a value to the builder for initialRevenue
* @param initialRevenue Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setInitialRevenue(Revenue initialRevenue) {
this.initialRevenue = initialRevenue;
return this;
}
/**
* Adds a value to the builder for currentRevenue
* @param currentRevenue Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCurrentRevenue(Revenue currentRevenue) {
this.currentRevenue = currentRevenue;
return this;
}
/**
* Adds a value to the builder for quantity
* @param quantity Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setQuantity(long quantity) {
this.quantity = quantity;
return this;
}
/**
* Adds a value to the builder for isTrial
* @param isTrial Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setIsTrial(boolean isTrial) {
this.isTrial = isTrial;
return this;
}
/**
* Adds a value to the builder for currencyCode
* @param currencyCode Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setCurrencyCode(String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the LineItem class
* @return The instantiated final LineItem
**/
public LineItem build() {
return new LineItem(
this.appKey,
this.packageId,
this.currency,
this.initialRevenue,
this.currentRevenue,
this.quantity,
this.isTrial,
this.currencyCode);
}
}
/**
* Returns a Builder for LineItem, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable LineItem object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the LineItem instance
**/
public String toString() {
String result = "LineItem\n";
result += "-> appKey: (LineItem.AppKey)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.appKey).split("\n"))) + "\n";
result += "-> packageId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.packageId).split("\n"))) + "\n";
result += "-> currency: (Currency)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.currency).split("\n"))) + "\n";
result += "-> initialRevenue: (Revenue)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.initialRevenue).split("\n"))) + "\n";
result += "-> currentRevenue: (Revenue)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.currentRevenue).split("\n"))) + "\n";
result += "-> quantity: (long)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.quantity).split("\n"))) + "\n";
result += "-> isTrial: (boolean)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.isTrial).split("\n"))) + "\n";
result += "-> currencyCode: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.currencyCode).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* LineItem, which is much more usable.
* @return An instance of LineItem representing the input proto object
**/
public static LineItem fromProto(WorkflowProto.LineItem proto) {
LineItem out = null;
if (proto != null) {
LineItem.Builder outBuilder = LineItem.newBuilder()
.setAppKey(LineItem.AppKey.fromProto(proto.getAppKey()))
.setPackageId(proto.getPackageId())
.setCurrency(Currency.fromProto(proto.getCurrency()))
.setInitialRevenue(Revenue.fromProto(proto.getInitialRevenue()))
.setCurrentRevenue(Revenue.fromProto(proto.getCurrentRevenue()))
.setQuantity(proto.getQuantity())
.setIsTrial(proto.getIsTrial())
.setCurrencyCode(proto.getCurrencyCode());
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of LineItem instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(WorkflowProto.LineItem proto : protos) {
out.add(LineItem.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of WorkflowProto.LineItem which is a proto object ready for wire transmission
**/
public WorkflowProto.LineItem toProto() {
LineItem obj = this;
WorkflowProto.LineItem.Builder outBuilder = WorkflowProto.LineItem.newBuilder();
if(obj.getAppKey() != null){outBuilder.setAppKey(obj.getAppKey().toProto());}
outBuilder.setPackageId(obj.getPackageId());
outBuilder.setCurrency(obj.getCurrency() != null?obj.getCurrency().toProto():null);
if(obj.getInitialRevenue() != null){outBuilder.setInitialRevenue(obj.getInitialRevenue().toProto());}
if(obj.getCurrentRevenue() != null){outBuilder.setCurrentRevenue(obj.getCurrentRevenue().toProto());}
outBuilder.setQuantity(obj.getQuantity());
outBuilder.setIsTrial(obj.getIsTrial());
outBuilder.setCurrencyCode(obj.getCurrencyCode());
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of WorkflowProto.LineItem instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (LineItem obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy