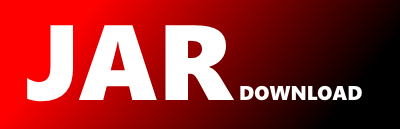
com.vendasta.sales.v1.internal.RequestSubscriptionChangeRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.ApiProto;
/**
*
**/
public final class RequestSubscriptionChangeRequest {
private final String businessId;
private final String message;
private final String phoneNumber;
private final String name;
private RequestSubscriptionChangeRequest (
final String businessId,
final String message,
final String phoneNumber,
final String name)
{
this.businessId = businessId;
this.message = message;
this.phoneNumber = phoneNumber;
this.name = name;
}
/**
* The id of the business that is making the request to change subscription
* @return The final value of businessId on the object
**/
public String getBusinessId() {
return this.businessId;
}
/**
* The message from the business to be sent, requesting the change
* @return The final value of message on the object
**/
public String getMessage() {
return this.message;
}
/**
* The phone number to be contacted about the subscription change
* @return The final value of phoneNumber on the object
**/
public String getPhoneNumber() {
return this.phoneNumber;
}
/**
* The person to contact about the subscription change
* @return The final value of name on the object
**/
public String getName() {
return this.name;
}
public static class Builder {
private String businessId;
private String message;
private String phoneNumber;
private String name;
public Builder() {
this.businessId = "";
this.message = "";
this.phoneNumber = "";
this.name = "";
}
/**
* Adds a value to the builder for businessId
* @param businessId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setBusinessId(String businessId) {
this.businessId = businessId;
return this;
}
/**
* Adds a value to the builder for message
* @param message Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setMessage(String message) {
this.message = message;
return this;
}
/**
* Adds a value to the builder for phoneNumber
* @param phoneNumber Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* Adds a value to the builder for name
* @param name Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the RequestSubscriptionChangeRequest class
* @return The instantiated final RequestSubscriptionChangeRequest
**/
public RequestSubscriptionChangeRequest build() {
return new RequestSubscriptionChangeRequest(
this.businessId,
this.message,
this.phoneNumber,
this.name);
}
}
/**
* Returns a Builder for RequestSubscriptionChangeRequest, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable RequestSubscriptionChangeRequest object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the RequestSubscriptionChangeRequest instance
**/
public String toString() {
String result = "RequestSubscriptionChangeRequest\n";
result += "-> businessId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.businessId).split("\n"))) + "\n";
result += "-> message: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.message).split("\n"))) + "\n";
result += "-> phoneNumber: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.phoneNumber).split("\n"))) + "\n";
result += "-> name: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.name).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* RequestSubscriptionChangeRequest, which is much more usable.
* @return An instance of RequestSubscriptionChangeRequest representing the input proto object
**/
public static RequestSubscriptionChangeRequest fromProto(ApiProto.RequestSubscriptionChangeRequest proto) {
RequestSubscriptionChangeRequest out = null;
if (proto != null) {
RequestSubscriptionChangeRequest.Builder outBuilder = RequestSubscriptionChangeRequest.newBuilder()
.setBusinessId(proto.getBusinessId())
.setMessage(proto.getMessage())
.setPhoneNumber(proto.getPhoneNumber())
.setName(proto.getName());
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of RequestSubscriptionChangeRequest instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(ApiProto.RequestSubscriptionChangeRequest proto : protos) {
out.add(RequestSubscriptionChangeRequest.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of ApiProto.RequestSubscriptionChangeRequest which is a proto object ready for wire transmission
**/
public ApiProto.RequestSubscriptionChangeRequest toProto() {
RequestSubscriptionChangeRequest obj = this;
ApiProto.RequestSubscriptionChangeRequest.Builder outBuilder = ApiProto.RequestSubscriptionChangeRequest.newBuilder();
outBuilder.setBusinessId(obj.getBusinessId());
outBuilder.setMessage(obj.getMessage());
outBuilder.setPhoneNumber(obj.getPhoneNumber());
outBuilder.setName(obj.getName());
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of ApiProto.RequestSubscriptionChangeRequest instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (RequestSubscriptionChangeRequest obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy