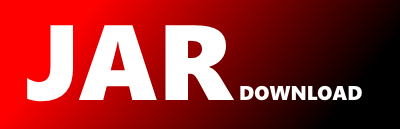
com.vendasta.sales.v1.internal.SalesTeam Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.SalesTeamProto;
/**
*
**/
public final class SalesTeam {
private final String teamName;
private final String groupId;
private final String partnerId;
private final String marketId;
private final Date updated;
private SalesTeam (
final String teamName,
final String groupId,
final String partnerId,
final String marketId,
final Date updated)
{
this.teamName = teamName;
this.groupId = groupId;
this.partnerId = partnerId;
this.marketId = marketId;
this.updated = updated;
}
/**
*
* @return The final value of teamName on the object
**/
public String getTeamName() {
return this.teamName;
}
/**
*
* @return The final value of groupId on the object
**/
public String getGroupId() {
return this.groupId;
}
/**
*
* @return The final value of partnerId on the object
**/
public String getPartnerId() {
return this.partnerId;
}
/**
*
* @return The final value of marketId on the object
**/
public String getMarketId() {
return this.marketId;
}
/**
*
* @return The final value of updated on the object
**/
public Date getUpdated() {
return this.updated;
}
public static class Builder {
private String teamName;
private String groupId;
private String partnerId;
private String marketId;
private Date updated;
public Builder() {
this.teamName = "";
this.groupId = "";
this.partnerId = "";
this.marketId = "";
this.updated = null;
}
/**
* Adds a value to the builder for teamName
* @param teamName Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setTeamName(String teamName) {
this.teamName = teamName;
return this;
}
/**
* Adds a value to the builder for groupId
* @param groupId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setGroupId(String groupId) {
this.groupId = groupId;
return this;
}
/**
* Adds a value to the builder for partnerId
* @param partnerId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setPartnerId(String partnerId) {
this.partnerId = partnerId;
return this;
}
/**
* Adds a value to the builder for marketId
* @param marketId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setMarketId(String marketId) {
this.marketId = marketId;
return this;
}
/**
* Adds a value to the builder for updated
* @param updated Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setUpdated(Date updated) {
this.updated = updated;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the SalesTeam class
* @return The instantiated final SalesTeam
**/
public SalesTeam build() {
return new SalesTeam(
this.teamName,
this.groupId,
this.partnerId,
this.marketId,
this.updated);
}
}
/**
* Returns a Builder for SalesTeam, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable SalesTeam object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the SalesTeam instance
**/
public String toString() {
String result = "SalesTeam\n";
result += "-> teamName: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.teamName).split("\n"))) + "\n";
result += "-> groupId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.groupId).split("\n"))) + "\n";
result += "-> partnerId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.partnerId).split("\n"))) + "\n";
result += "-> marketId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.marketId).split("\n"))) + "\n";
result += "-> updated: (Date)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.updated).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* SalesTeam, which is much more usable.
* @return An instance of SalesTeam representing the input proto object
**/
public static SalesTeam fromProto(SalesTeamProto.SalesTeam proto) {
SalesTeam out = null;
if (proto != null) {
SalesTeam.Builder outBuilder = SalesTeam.newBuilder()
.setTeamName(proto.getTeamName())
.setGroupId(proto.getGroupId())
.setPartnerId(proto.getPartnerId())
.setMarketId(proto.getMarketId())
.setUpdated(proto.hasUpdated()?new Date(proto.getUpdated().getSeconds() * 1000):null);
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of SalesTeam instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(SalesTeamProto.SalesTeam proto : protos) {
out.add(SalesTeam.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of SalesTeamProto.SalesTeam which is a proto object ready for wire transmission
**/
public SalesTeamProto.SalesTeam toProto() {
SalesTeam obj = this;
SalesTeamProto.SalesTeam.Builder outBuilder = SalesTeamProto.SalesTeam.newBuilder();
outBuilder.setTeamName(obj.getTeamName());
outBuilder.setGroupId(obj.getGroupId());
outBuilder.setPartnerId(obj.getPartnerId());
outBuilder.setMarketId(obj.getMarketId());
if(obj.getUpdated()!=null){outBuilder.setUpdated(com.google.protobuf.Timestamp.newBuilder().setSeconds(obj.getUpdated().getTime() / 1000).build());}
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of SalesTeamProto.SalesTeam instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (SalesTeam obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy