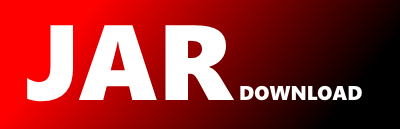
com.vendasta.sales.v1.internal.Salesperson Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.SalespersonProto;
/**
*
**/
public final class Salesperson {
private final String salespersonId;
private final String firstName;
private final String lastName;
private final String email;
private final List phoneNumber;
private Salesperson (
final String salespersonId,
final String firstName,
final String lastName,
final String email,
final List phoneNumber)
{
this.salespersonId = salespersonId;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
this.phoneNumber = phoneNumber;
}
/**
*
* @return The final value of salespersonId on the object
**/
public String getSalespersonId() {
return this.salespersonId;
}
/**
*
* @return The final value of firstName on the object
**/
public String getFirstName() {
return this.firstName;
}
/**
*
* @return The final value of lastName on the object
**/
public String getLastName() {
return this.lastName;
}
/**
*
* @return The final value of email on the object
**/
public String getEmail() {
return this.email;
}
/**
*
* @return The final value of phoneNumber on the object
**/
public List getPhoneNumber() {
return this.phoneNumber;
}
public static class Builder {
private String salespersonId;
private String firstName;
private String lastName;
private String email;
private List phoneNumber;
public Builder() {
this.salespersonId = "";
this.firstName = "";
this.lastName = "";
this.email = "";
this.phoneNumber = new ArrayList();
}
/**
* Adds a value to the builder for salespersonId
* @param salespersonId Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setSalespersonId(String salespersonId) {
this.salespersonId = salespersonId;
return this;
}
/**
* Adds a value to the builder for firstName
* @param firstName Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setFirstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* Adds a value to the builder for lastName
* @param lastName Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setLastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Adds a value to the builder for email
* @param email Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setEmail(String email) {
this.email = email;
return this;
}
/**
* Adds a value to the builder for phoneNumber
* @param phoneNumber Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setPhoneNumber(List phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the Salesperson class
* @return The instantiated final Salesperson
**/
public Salesperson build() {
return new Salesperson(
this.salespersonId,
this.firstName,
this.lastName,
this.email,
this.phoneNumber);
}
}
/**
* Returns a Builder for Salesperson, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable Salesperson object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the Salesperson instance
**/
public String toString() {
String result = "Salesperson\n";
result += "-> salespersonId: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.salespersonId).split("\n"))) + "\n";
result += "-> firstName: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.firstName).split("\n"))) + "\n";
result += "-> lastName: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.lastName).split("\n"))) + "\n";
result += "-> email: (String)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.email).split("\n"))) + "\n";
result += "-> phoneNumber: (List)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.phoneNumber).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* Salesperson, which is much more usable.
* @return An instance of Salesperson representing the input proto object
**/
public static Salesperson fromProto(SalespersonProto.Salesperson proto) {
Salesperson out = null;
if (proto != null) {
Salesperson.Builder outBuilder = Salesperson.newBuilder()
.setSalespersonId(proto.getSalespersonId())
.setFirstName(proto.getFirstName())
.setLastName(proto.getLastName())
.setEmail(proto.getEmail())
.setPhoneNumber(proto.getPhoneNumberList());
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of Salesperson instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(SalespersonProto.Salesperson proto : protos) {
out.add(Salesperson.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of SalespersonProto.Salesperson which is a proto object ready for wire transmission
**/
public SalespersonProto.Salesperson toProto() {
Salesperson obj = this;
SalespersonProto.Salesperson.Builder outBuilder = SalespersonProto.Salesperson.newBuilder();
outBuilder.setSalespersonId(obj.getSalespersonId());
outBuilder.setFirstName(obj.getFirstName());
outBuilder.setLastName(obj.getLastName());
outBuilder.setEmail(obj.getEmail());
outBuilder.addAllPhoneNumber(obj.getPhoneNumber());
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of SalespersonProto.Salesperson instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (Salesperson obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy