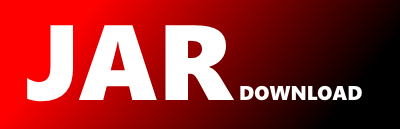
com.vendasta.sales.v1.internal.UserActionsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sales.v1 Show documentation
Show all versions of sales.v1 Show documentation
Java SDK for service sales
The newest version!
package com.vendasta.sales.v1.internal;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Collections;
import java.util.Arrays;
import org.apache.commons.lang3.StringUtils;
import com.vendasta.sales.v1.generated.UserActionsProto;
/**
*
**/
public final class UserActionsResponse {
private final List userActions;
private final UserActionsPagedResponseMetadata pagingMetadata;
private UserActionsResponse (
final List userActions,
final UserActionsPagedResponseMetadata pagingMetadata)
{
this.userActions = userActions;
this.pagingMetadata = pagingMetadata;
}
/**
* A collection of UserActions returned
* @return The final value of userActions on the object
**/
public List getUserActions() {
return this.userActions;
}
/**
* Contains paging data returned from the query
* @return The final value of pagingMetadata on the object
**/
public UserActionsPagedResponseMetadata getPagingMetadata() {
return this.pagingMetadata;
}
public static class Builder {
private List userActions;
private UserActionsPagedResponseMetadata pagingMetadata;
public Builder() {
this.userActions = null;
this.pagingMetadata = null;
}
/**
* Adds a value to the builder for userActions
* @param userActions Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setUserActions(List userActions) {
this.userActions = userActions;
return this;
}
/**
* Adds a value to the builder for pagingMetadata
* @param pagingMetadata Value to assign to the mutable Builder
* @return The Builder instance so that call chaining works
**/
public Builder setPagingMetadata(UserActionsPagedResponseMetadata pagingMetadata) {
this.pagingMetadata = pagingMetadata;
return this;
}
/**
* Takes the configuration in the mutable Builder and uses it to instantiate a final instance
* of the UserActionsResponse class
* @return The instantiated final UserActionsResponse
**/
public UserActionsResponse build() {
return new UserActionsResponse(
this.userActions,
this.pagingMetadata);
}
}
/**
* Returns a Builder for UserActionsResponse, which is a mutable representation of the object. Once the
* client has built up an object they can then create an immutable UserActionsResponse object using the
* build function.
* @return A fresh Builder instance with no values set
**/
public static Builder newBuilder() {
return new Builder();
}
/**
* Provides a human-readable representation of this object. Useful for debugging.
* @return A string representation of the UserActionsResponse instance
**/
public String toString() {
String result = "UserActionsResponse\n";
result += "-> userActions: (List)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.userActions).split("\n"))) + "\n";
result += "-> pagingMetadata: (UserActionsPagedResponseMetadata)"
+ StringUtils.join("\n ", Arrays.asList(String.valueOf(this.pagingMetadata).split("\n"))) + "\n";
return result;
}
/**
* Allows for simple conversion between the low-level generated protobuf object to
* UserActionsResponse, which is much more usable.
* @return An instance of UserActionsResponse representing the input proto object
**/
public static UserActionsResponse fromProto(UserActionsProto.UserActionsResponse proto) {
UserActionsResponse out = null;
if (proto != null) {
UserActionsResponse.Builder outBuilder = UserActionsResponse.newBuilder()
.setUserActions(UserAction.fromProtos(proto.getUserActionsList()))
.setPagingMetadata(UserActionsPagedResponseMetadata.fromProto(proto.getPagingMetadata()));
out = outBuilder.build();
}
return out;
}
/**
* Convenience method for handling lists of proto objects. It calls .fromProto on each one
* and returns a list of the converted results.
* @return A list of UserActionsResponse instances representing the input proto objects
**/
public static List fromProtos(List protos) {
List out = new ArrayList();
for(UserActionsProto.UserActionsResponse proto : protos) {
out.add(UserActionsResponse.fromProto(proto));
}
return out;
}
/**
* Allows for simple conversion of an object to the low-level generated protobuf object.
* @return An instance of UserActionsProto.UserActionsResponse which is a proto object ready for wire transmission
**/
public UserActionsProto.UserActionsResponse toProto() {
UserActionsResponse obj = this;
UserActionsProto.UserActionsResponse.Builder outBuilder = UserActionsProto.UserActionsResponse.newBuilder();
outBuilder.addAllUserActions(UserAction.toProtos(obj.getUserActions()));
if(obj.getPagingMetadata() != null){outBuilder.setPagingMetadata(obj.getPagingMetadata().toProto());}
return outBuilder.build();
}
/**
* Convenience method for handling lists of objects. It calls .toProto on each one and
* returns a list of the converted results.
* @return A list of UserActionsProto.UserActionsResponse instances representing the input objects.
*/
public static List toProtos(List objects) {
List out = new ArrayList();
if(objects != null) {
for (UserActionsResponse obj : objects) {
out.add(obj!=null?obj.toProto():null);
}
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy