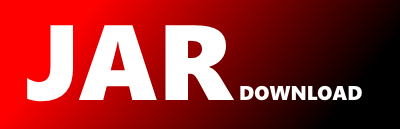
com.venmo.cursor.IterableMergeCursor Maven / Gradle / Ivy
package com.venmo.cursor;
import android.database.MergeCursor;
import java.util.Iterator;
import static com.venmo.cursor.CursorUtils.nextDocumentHelper;
import static com.venmo.cursor.CursorUtils.previousDocumentHelper;
public class IterableMergeCursor extends MergeCursor implements IterableCursor {
private final IterableCursor[] mCursors;
private IterableCursor mCurrent;
public IterableMergeCursor(IterableCursor cursor) {
this(asArray(cursor));
}
@SuppressWarnings("unchecked")
public IterableMergeCursor(IterableCursor first, IterableCursor second) {
this(IterableMergeCursor.asArray(first, second));
}
@SuppressWarnings("unchecked")
public IterableMergeCursor(IterableCursor first, IterableCursor second,
IterableCursor third) {
this(IterableMergeCursor.asArray(first, second, third));
}
@SuppressWarnings("unchecked")
public IterableMergeCursor(IterableCursor first, IterableCursor second,
IterableCursor third, IterableCursor fourth) {
this(IterableMergeCursor.asArray(first, second, third, fourth));
}
@SuppressWarnings("unchecked")
public IterableMergeCursor(IterableCursor first, IterableCursor second,
IterableCursor third, IterableCursor fourth, IterableCursor fifth) {
this(IterableMergeCursor.asArray(first, second, third, fourth, fifth));
}
public IterableMergeCursor(IterableCursor... cursors) {
super(cursors);
mCursors = cursors;
moveToFirst();
}
@SuppressWarnings("unchecked")
private static IterableCursor[] asArray(IterableCursor cursor) {
return (IterableCursor[]) new IterableCursor[]{cursor};
}
@SuppressWarnings("unchecked")
private static IterableCursor[] asArray(IterableCursor... cursor) {
return (IterableCursor[]) cursor;
}
@Override
public boolean onMove(int oldPosition, int newPosition) {
int newPositionCopy = newPosition;
for (IterableCursor cursor : mCursors) {
if (cursor != null) {
if (newPositionCopy < cursor.getCount()) {
mCurrent = cursor;
break;
} else {
newPositionCopy -= cursor.getCount();
}
}
}
return super.onMove(oldPosition, newPosition);
}
@Override
public T peek() {
return mCurrent.peek();
}
@Override
public T nextDocument() {
return nextDocumentHelper(this);
}
@Override
public T previousDocument() {
return previousDocumentHelper(this);
}
@Override
public Iterator iterator() {
return new CursorIterator(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy