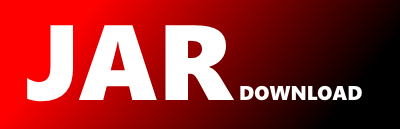
com.versionone.apiclient.services.QueryVariable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of VersionOne.SDK.Java.APIClient Show documentation
Show all versions of VersionOne.SDK.Java.APIClient Show documentation
A library for custom Java development against the VersionOne Development Platform's REST API.
package com.versionone.apiclient.services;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import com.versionone.apiclient.interfaces.IValueProvider;
public class QueryVariable implements IValueProvider {
public final String name;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy