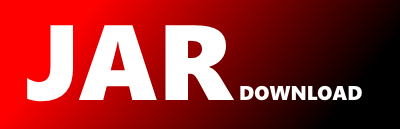
com.versionone.om.MessageReceipt Maven / Gradle / Ivy
package com.versionone.om;
@MetaDataAttribute("MessageReceipt")
public class MessageReceipt extends Entity {
MessageReceipt(AssetID id, V1Instance instance)
{
super(id, instance);
}
MessageReceipt(V1Instance instance)
{
super(instance);
}
///
/// Member this message is addressed to.
///
public Member getRecipient()
{
return getRelation(Member.class, "Recipient");
}
///
/// Member this message is addressed to.
///
public void setRecipient(Member value)
{
setRelation("Recipient", value);
}
///
/// Member this message is addressed to.
///
public Message getMessage()
{
return getRelation(Message.class, "Message");
}
///
/// Member this message is addressed to.
///
public void setMessage(Message value)
{
setRelation("Message", value);
}
///
/// True if the item has not been read
///
public boolean isUnread()
{
return (Boolean) get("IsUnread");
}
///
/// True if the item has been read
///
public boolean isRead()
{
return (Boolean) get("IsRead");
}
///
/// True if the item has not been archived
///
public boolean isUnarchived()
{
return (Boolean) get("IsUnarchived");
}
///
/// True if the item has been archived
///
public boolean isArchived()
{
return (Boolean) get("IsArchived");
}
///
/// Mark the message as having been read by the recipient.
///
public void markAsRead()
{
getInstance().executeOperation(MessageReceipt.class, this, "MarkAsRead");
}
///
/// Mark the message as having not been read by the recipient.
///
public void markAsUnread()
{
getInstance().executeOperation(MessageReceipt.class, this, "MarkAsUnread");
}
///
/// Mark the message as having been archived.
///
public void markAsArchived()
{
getInstance().executeOperation(MessageReceipt.class, this, "MarkAsArchived");
}
///
/// Mark the message as not having been archived.
///
public void markAsUnarchived()
{
getInstance().executeOperation(MessageReceipt.class, this, "MarkAsUnarchived");
}
///
/// Mark the message as having been archived.
///
public void delete()
{
getInstance().executeOperation(MessageReceipt.class, this, "Delete");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy