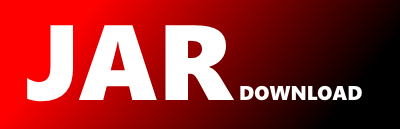
com.viae.maven.sonar.mojos.SonarMavenSetGitBranchMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-maven-plugin Show documentation
Show all versions of sonar-maven-plugin Show documentation
This plugin will make maven interact with SONAR.
/*
* Copyright (c) 2016 by VIAE (http///viae-it.com)
*/
package com.viae.maven.sonar.mojos;
import com.viae.maven.sonar.config.SonarStrings;
import com.viae.maven.sonar.exceptions.GitException;
import com.viae.maven.sonar.services.GitService;
import com.viae.maven.sonar.services.GitServiceImpl;
import org.apache.commons.lang3.StringUtils;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.project.MavenProject;
/**
* Mojo to set the sonar.branch property to the git branch name (if the property is not defined).
*
* Created by Vandeperre Maarten on 29/04/2016.
*/
@Mojo(name = SonarStrings.MOJO_NAME_SET_GIT_BRANCH, aggregator = true)
public class SonarMavenSetGitBranchMojo extends AbstractMojo {
@Component
protected MavenProject project;
private final GitService gitService = new GitServiceImpl(getLog());
/**
* Set the sonar.branch property to the git branch name (if the property is not defined).
* @throws MojoExecutionException will be thrown when something goes wrong while retrieving the git branch name.
* @throws MojoFailureException will not be thrown
*/
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
getLog().info( String.format( "%s start execution of '%s'", SonarStrings.LOG_PREFIX, SonarStrings.MOJO_NAME_SET_GIT_BRANCH ) );
try {
final String existingBranchValue = project.getProperties().getProperty( SonarStrings.BRANCH );
getLog().info( String.format( "%s existing %s: '%s'", SonarStrings.LOG_PREFIX, SonarStrings.BRANCH, existingBranchValue ) );
if ( StringUtils.isBlank( existingBranchValue ) ) {
final String sonarBranchName = gitService.getBranchName( Runtime.getRuntime() );
getLog().info( String.format( "%s set property '%s' to '%s'", SonarStrings.LOG_PREFIX, SonarStrings.BRANCH, sonarBranchName ) );
project.getProperties().setProperty( SonarStrings.BRANCH, sonarBranchName );
}
}
catch ( final GitException e ) {
getLog().error( String.format( "%s %s", SonarStrings.LOG_PREFIX, e.getLocalizedMessage() ) );
throw new MojoExecutionException( String.format( "%s %s", SonarStrings.LOG_PREFIX, e.getLocalizedMessage() ), e );
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy