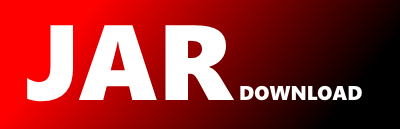
com.viaoa.util.OAEncryption Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oa-core Show documentation
Show all versions of oa-core Show documentation
Object Automation library
package com.viaoa.util;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.security.*;
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
/*
Sun:
http://java.sun.com/javase/6/docs/technotes/guides/security/crypto/CryptoSpec.html
Sample programs:
http://www.owasp.org/index.php/Digital_Signature_Implementation_in_Java
http://www.rgagnon.com/javadetails/java-0400.html
*/
public class OAEncryption {
/**
* Generates a SHA-256 hash code base64 string for a given input.
* This is a one way function (irreversible).
*
* Example: used when the real password is not stored. Instead
* the hash is stored and is used to compare the hash of user input.
*/
public static String getSHAHash(String input){
return getHash(input);
}
public static String getHash(String input){
if (input == null) return null;
MessageDigest md = null;
try {
md = MessageDigest.getInstance("SHA-256");
}
catch(NoSuchAlgorithmException e){
System.out.println("No SHA-256");
return null;
}
try {
md.update(input.getBytes("UTF-8"));
}
catch(UnsupportedEncodingException e)
{
System.out.println("Encoding error.");
}
byte raw[] = md.digest();
String hash = new String(Base64.encode(raw));
return hash;
}
/**
* Encrypt bytes into a new byte array.
* @see #decrypt(byte[])
*/
public static byte[] encrypt(byte[] bs) throws Exception {
Cipher cipher = Cipher.getInstance("DES");
cipher.init(Cipher.ENCRYPT_MODE, getSecretKey());
bs = cipher.doFinal(bs);
return bs;
}
public static byte[] encrypt(byte[] bs, String password) throws Exception {
Cipher cipher = Cipher.getInstance("DES");
SecretKey key;
if (OAString.isEmpty(password)) key = getSecretKey();
else key = getSecretKey(password);
cipher.init(Cipher.ENCRYPT_MODE, key);
bs = cipher.doFinal(bs);
return bs;
}
public static Cipher getCipher() throws Exception {
Cipher cipher = Cipher.getInstance("DES");
cipher.init(Cipher.ENCRYPT_MODE, getSecretKey());
return cipher;
}
/**
* Decrypt bytes into a new byte array.
* @see #encrypt(byte[])
*/
public static byte[] decrypt(byte[] bs) throws Exception {
Cipher cipher = Cipher.getInstance("DES");
cipher.init(Cipher.DECRYPT_MODE, getSecretKey());
bs = cipher.doFinal(bs);
return bs;
}
public static byte[] decrypt(byte[] bs, String password) throws Exception {
Cipher cipher = Cipher.getInstance("DES");
SecretKey key;
if (OAString.isEmpty(password)) key = getSecretKey();
else key = getSecretKey(password);
cipher.init(Cipher.DECRYPT_MODE, key);
bs = cipher.doFinal(bs);
return bs;
}
private static SecretKey _secretKey;
/**
* DES secret key used for encrypting data.
*/
public static SecretKey getSecretKey() throws Exception {
if (_secretKey == null) {
byte[] bs = new byte[DESKeySpec.DES_KEY_LEN];
for (int i=0; i \""+s2+"\"");
String s3 = decrypt(s2, "password");
System.out.println("Decrypted ==> \""+s3+"\"");
String s4 = getHash(s);
System.out.println("Hashed ==> \""+s4+"\"");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy