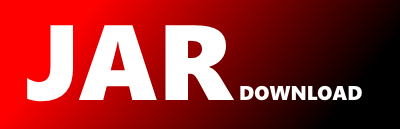
com.viaoa.hub.HubSelectDelegate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oa-core Show documentation
Show all versions of oa-core Show documentation
Object Automation library
/* Copyright 1999 Vince Via [email protected]
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.viaoa.hub;
import java.util.HashSet;
import java.util.concurrent.ConcurrentHashMap;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.viaoa.ds.*;
import com.viaoa.object.*;
import com.viaoa.util.OAFilter;
import com.viaoa.util.OAString;
/**
* Delegate used for Hub selecting and lazy loading (fetching) from datasource.
* @author vvia
*
*/
public class HubSelectDelegate {
private static Logger LOG = Logger.getLogger(HubSelectDelegate.class.getName());
/** Internal method to retrieve objects from last select() */
protected static int fetchMore(Hub thisHub) {
int x = fetchMore(thisHub, HubSelectDelegate.getSelect(thisHub));
return x;
}
protected static int fetchMore(Hub thisHub, OASelect sel) {
if (sel == null) return 0;
int x = sel.getFetchAmount();
x = fetchMore(thisHub, sel, x);
return x;
}
/** Internal method to retrieve objects from last select() */
protected static int fetchMore(Hub thisHub, int famt) {
int x = fetchMore(thisHub, HubSelectDelegate.getSelect(thisHub), famt);
return x;
}
private static int cntWarning;
private static ConcurrentHashMap hmHubFetch = new ConcurrentHashMap(11, .85f);
protected static int fetchMore(Hub thisHub, OASelect sel, int famt) {
try {
// get fetch lock
for (;;) {
synchronized (hmHubFetch) {
if (hmHubFetch.get(thisHub) == null) {
hmHubFetch.put(thisHub, 0);
break;
}
try {
hmHubFetch.put(thisHub, 1);
hmHubFetch.wait(5);
}
catch (Exception e) {
}
}
}
return _fetchMore(thisHub, sel, famt);
}
finally {
synchronized (hmHubFetch) {
int x = hmHubFetch.remove(thisHub);
if (x > 0) hmHubFetch.notifyAll();
}
}
}
protected static int _fetchMore(Hub thisHub, OASelect sel, int famt) {
if (sel == null) return 0;
int fa = sel.getFetchAmount(); // default amount to load
HubData hubData = thisHub.data;
boolean holdDataChanged = hubData.changed;
if (famt > 0) fa = famt;
int cnt = 0;
try {
int capacity = hubData.vector.capacity(); // number of available 'slots'
int size = hubData.vector.size(); // number of elements
for ( ; cnt < fa || fa == 0; ) {
Object obj;
if ( !HubSelectDelegate.isMoreData(sel) ) {
thisHub.cancelSelect();
sel.cancel();
break;
}
obj = sel.next();
if (obj != null) {
if (size == (capacity-1)) { // resize Vector according to select
/*
if (thisHub.data.loadingAllData) {
capacity = HubSelectDelegate.getCount(thisHub);
if (capacity <= 0) capacity = size+1;
}
*/
capacity += (capacity > 250) ? 75 : capacity; // this will override the default behavior of how the Vector grows itself (which is to double in size)
//LOG.config("resizing, from:"+size+", to:"+capacity+", hub:"+thisHub);
HubDataDelegate.ensureCapacity(thisHub, capacity);
}
try {
OAThreadLocalDelegate.setLoading(true);
HubAddRemoveDelegate.add(thisHub, obj);
/***qqqqqqqqqqqqqqqq
if (sel.getNextCount() != thisHub.getCurrentSize()) {
//qqqqqqqqqqqqqq
int xx = 4;
xx++;
long ts = System.currentTimeMillis();
if (ts > msLAST+2500) {
msLAST = ts;
LOG.log(Level.WARNING, "VINCE qqqqqqqqqqqqqqqqqqqqqq hub="+thisHub, new Exception("fetchMore hub.add counts not the same qqqqqqqqqqqqqqqqqq"));
}
}
*/
}
finally {
OAThreadLocalDelegate.setLoading(false);
}
size++;
cnt++;
}
}
}
catch (Exception ex) {
LOG.log(Level.WARNING, "Hub="+thisHub+", will cancel select", ex);
cancelSelect(thisHub, false);
sel.cancel();
throw new RuntimeException(ex);
}
finally {
hubData.changed = holdDataChanged;
}
return cnt;
}
/**
Find out if more objects are available from last select from OADataSource.
*/
public static boolean isMoreData(Hub thisHub) {
OASelect sel = getSelect(thisHub);
if (sel == null) return false;
if (!sel.hasBeenStarted()) {
sel.select();
}
return sel.hasMore();
}
public static boolean isMoreData(OASelect sel) {
if (sel == null) return false;
if (!sel.hasBeenStarted()) {
sel.select();
}
return sel.hasMore();
}
/**
This will automatically read all records from current select().
By default, only 45 objects are read at a time from datasource.
*/
public static void loadAllData(Hub thisHub) {
loadAllData(thisHub, thisHub.getSelect());
}
public static void loadAllData(Hub thisHub, OASelect select) {
if (thisHub == null || select == null) return;
// 20121015 adjusted for locking
for (int i=0; ;i++) {
boolean bCanRun = false;
synchronized (thisHub.data) {
if (!thisHub.data.isLoadingAllData()) {
thisHub.data.setLoadingAllData(true);
bCanRun = true;
}
}
if (bCanRun) {
try {
while ( isMoreData(select) ) {
fetchMore(thisHub, select);
}
}
finally {
synchronized (thisHub.data) {
thisHub.data.setLoadingAllData(false);
}
}
break;
}
// else wait and try again
try {
Thread.sleep(25);
}
catch (Exception e) {
}
}
/* was;
synchronized (thisHub.data) {
if (!thisHub.data.loadingAllData) {
thisHub.data.loadingAllData = true;
try {
while ( isMoreData(thisHub) ) {
fetchMore(thisHub);
}
}
finally {
thisHub.data.loadingAllData = false;
}
}
}
*/
}
/**
Returns OASelect used for querying datasource.
*/
protected static OASelect getSelect(Hub thisHub) {
return getSelect(thisHub, false);
}
protected static OASelect getSelect(Hub thisHub, boolean bCreateIfNull) {
if (thisHub == null) return null;
OASelect sel = thisHub.data.getSelect();
if (sel != null || !bCreateIfNull) return sel;
sel = new OASelect(thisHub.getObjectClass());
thisHub.data.setSelect(sel);
return sel;
}
/**
Used to populate Hub with objects returned from a OADataSource select.
By default, all objects will first be removed from the Hub, OASelect.select() will
be called, and the first 45 objects will be added to Hub and active object will be
set to null. As the Hub is accessed for more objects, more will be returned until
the query is exhausted of objects.
*/
public static void select(final Hub thisHub, OASelect select) { // This is the main select method for Hub that all of the other select methods call.
cancelSelect(thisHub, true);
if (select == null) {
return;
}
if (thisHub.datau.getSharedHub() != null) {
select(thisHub.datau.getSharedHub(), select);
return;
}
if (thisHub.data.objClass == null) {
thisHub.data.objClass = select.getSelectClass();
if (thisHub.data.objClass == null) return;
}
if (thisHub.datam.getMasterObject() != null && thisHub.datam.liDetailToMaster != null) {
if (select != thisHub.data.getSelect() && thisHub.data.getSelect() != null) {
throw new RuntimeException("select cant be changed for detail hub");
}
if (thisHub.datam.getMasterObject() != null) {
if (thisHub.datam.getMasterObject() != select.getWhereObject()) {
if (select.getWhere() == null || select.getWhere().length() == 0) {
OAObjectInfo oi = OAObjectInfoDelegate.getOAObjectInfo(thisHub.datam.getMasterObject().getClass());
if (oi.getUseDataSource()) {
select.setWhereObject(thisHub.datam.getMasterObject());
}
}
else {
// cant call select on a hub that is a detail hub
// 20140308 removed, ex: ServerRoot.getOrders() has a select
// throw new RuntimeException("cant call select on a detail hub");
}
}
}
}
if (select.getWhereObject() != null) {
if (thisHub.datam.liDetailToMaster != null && select.getWhereObject() == thisHub.datam.getMasterObject()) {
select.setPropertyFromWhereObject(thisHub.datam.liDetailToMaster.getReverseName());
}
}
select.setSelectClass(thisHub.getObjectClass());
OAObjectInfo oi = OAObjectInfoDelegate.getOAObjectInfo(thisHub.getObjectClass());
HubEventDelegate.fireBeforeSelectEvent(thisHub);
boolean bRunSelect;
bRunSelect = oi.getUseDataSource();
// 20160110 selects now have hubFinders, etc to do selects
bRunSelect = (bRunSelect && (select.getDataSource() != null || select.getFinder() != null));
//was: bRunSelect = (bRunSelect && select.getDataSource() != null);
HubDataDelegate.incChangeCount(thisHub);
if (select.getAppend()) {
thisHub.data.setSelect(select);
}
else {
thisHub.setAO(null); // 20100507
if (thisHub.isOAObject()) {
int z = HubDataDelegate.getCurrentSize(thisHub);
for (int i=0; i filter = new OAFilter() {
@Override
public boolean isUsed(Hub h) {
if (h != thisHub && h.dataa != thisHub.dataa) {
if (h.datau.getLinkToHub() == null) return true;
}
return false;
}
};
Hub[] hubs = HubShareDelegate.getAllSharedHubs(thisHub, filter);
for (int i=0; i hs = new HashSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy