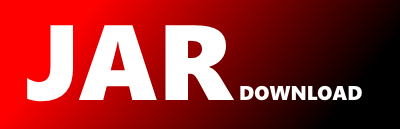
com.viaoa.hub.HubEventDelegate Maven / Gradle / Ivy
Show all versions of oa-core Show documentation
/* Copyright 1999 Vince Via [email protected]
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.viaoa.hub;
import java.lang.ref.WeakReference;
import java.util.ArrayList;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.locks.ReentrantReadWriteLock;
import com.viaoa.object.*;
import com.viaoa.remote.multiplexer.OARemoteThreadDelegate;
import com.viaoa.util.OAString;
/**
* Delegate used to register Hub listeners, get Listeners and to send Events to Hub listeners.
*/
public class HubEventDelegate {
// 20120827 might be used later, if we need to have hub changes notify masterobject
protected static void fireMasterObjectChangeEvent(Hub thisHub, boolean bRefreshFlag) {
// OAObjectHubDelegate.fireMasterObjectHubChangeEvent(thisHub, bRefreshFlag);
}
public static void fireBeforeRemoveEvent(Hub thisHub, Object obj, int pos) {
// verify with editQuery
if (!OARemoteThreadDelegate.isRemoteThread()) {
if (obj instanceof OAObject) {
OAObjectEditQuery em = OAObjectEditQueryDelegate.getVerifyRemoveEditQuery(thisHub, (OAObject) obj, OAObjectEditQuery.CHECK_CallbackMethod);
if (!em.getAllowed()) {
String s = em.getResponse();
if (OAString.isEmpty(s)) s = "edit query returned false for remove, Hub="+thisHub;
throw new RuntimeException(s, em.getThrowable());
}
}
}
// call listeners
HubListener[] hls = getAllListeners(thisHub);
int x = hls.length;
if (x > 0) {
HubEvent hubEvent = new HubEvent(thisHub,obj, pos);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
final HubEvent hubEvent = new HubEvent(thisHub, obj, pos);
if (OARemoteThreadDelegate.shouldEventsBeQueued()) {
Runnable r = new Runnable() {
@Override
public void run() {
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
final HubEvent hubEvent = new HubEvent(thisHub);
if (OARemoteThreadDelegate.shouldEventsBeQueued()) {
Runnable r = new Runnable() {
@Override
public void run() {
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub, obj, pos);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
final HubEvent hubEvent = new HubEvent(thisHub,obj,pos);
if (OARemoteThreadDelegate.shouldEventsBeQueued()) {
Runnable r = new Runnable() {
@Override
public void run() {
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub, obj, pos);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
final HubEvent hubEvent = new HubEvent(thisHub, obj, pos);
if (OARemoteThreadDelegate.shouldEventsBeQueued()) {
Runnable r = new Runnable() {
@Override
public void run() {
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
Exception exception = null;
final HubEvent hubEvent = new HubEvent(thisHub, obj, pos);
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub);
OAThreadLocalDelegate.addHubEvent(hubEvent);
try {
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub);
OAThreadLocalDelegate.addHubEvent(hubEvent);
try {
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub, obj);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
final HubEvent hubEvent = new HubEvent(thisHub, obj);
if (OARemoteThreadDelegate.shouldEventsBeQueued()) {
Runnable r = new Runnable() {
@Override
public void run() {
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub, obj);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub, obj);
OAThreadLocalDelegate.addHubEvent(hubEvent);
try {
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub, fromPos, toPos);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub, fromPos, toPos);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i
Example:
If the Department is changed for an Employee, then the Employee will be removed
from the previous Department's Hub of Employees and moved to the new Department's
Hub of Employees.
If this Hub is linked to a property in another Hub and that property is changed, this
Hub will changed it's active object to match the same value as the new property value.
@param propertyName name of property that changed. This is case insensitive
*/
public static void fireCalcPropertyChange(Hub thisHub, final Object object, final String propertyName) {
// 20180304
if (OAThreadLocalDelegate.hasSentCalcPropertyChange(thisHub, (OAObject) object, propertyName)) return;
HubListener[] hl = HubEventDelegate.getAllListeners(thisHub);
int x = hl.length;
if (x > 0) {
HubEvent hubEvent = new HubEvent(thisHub, object, propertyName, null, null);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
HubEvent hubEvent = new HubEvent(thisHub,oaObj,propertyName,oldValue,newValue);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i 0) {
final HubEvent hubEvent = new HubEvent(thisHub,oaObj,propertyName,oldValue,newValue);
if (OARemoteThreadDelegate.shouldEventsBeQueued()) {
Runnable r = new Runnable() {
@Override
public void run() {
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i[] refs = HubShareDelegate.getSharedWeakHubs(thisHub);
for (i=0; refs != null && i ref = refs[i];
if (ref == null) continue;
Hub h2 = ref.get();
if (h2 == null) continue;
propertyChangeUpdateDetailHubs(h2, object,propertyName);
}
}
/**
Used to notify listeners that a new collection has been established.
Called by select() and when a detail Hub's source of data is changed.
*/
public static void fireOnNewListEvent(Hub thisHub, boolean bAll) {
if (thisHub == null) return;
HubListener[] hl = getAllListeners(thisHub, (bAll?0:2) );
int x = hl.length;
if (x > 0) {
HubEvent hubEvent = new HubEvent(thisHub,null);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (int i=0; i= 0) {
throw new RuntimeException("dont use a property path for listener, use addHubListener(h,hl,propertyName, String[path]) instead");
}
getHubListenerTree(thisHub).addListener(hl, property, dependentPropertyPaths, false);
clearGetAllListenerCache(thisHub);
}
public static void addHubListener(Hub thisHub, HubListener hl, String property, String[] dependentPropertyPaths, boolean bActiveObjectOnly) {
if (property != null && property.indexOf('.') >= 0) {
throw new RuntimeException("dont use a property path for listener, use addHubListener(h,hl,propertyName, String[path]) instead");
}
getHubListenerTree(thisHub).addListener(hl, property, dependentPropertyPaths, bActiveObjectOnly);
clearGetAllListenerCache(thisHub);
}
public static void addHubListener(Hub thisHub, HubListener hl, String property, String[] dependentPropertyPaths, boolean bActiveObjectOnly, boolean bUseBackgroundThread) {
if (property != null && property.indexOf('.') >= 0) {
throw new RuntimeException("dont use a property path for listener, use addHubListener(h,hl,propertyName, String[path]) instead");
}
getHubListenerTree(thisHub).addListener(hl, property, dependentPropertyPaths, bActiveObjectOnly, bUseBackgroundThread);
clearGetAllListenerCache(thisHub);
}
public static void addHubListener(Hub thisHub, HubListener hl, String property) {
getHubListenerTree(thisHub).addListener(hl, property);
clearGetAllListenerCache(thisHub);
}
public static void addHubListener(Hub thisHub, HubListener hl, String property, boolean bActiveObjectOnly) {
getHubListenerTree(thisHub).addListener(hl, property, bActiveObjectOnly);
clearGetAllListenerCache(thisHub);
}
public static void addHubListener(Hub thisHub, HubListener hl, boolean bActiveObjectOnly) {
getHubListenerTree(thisHub).addListener(hl, bActiveObjectOnly);
clearGetAllListenerCache(thisHub);
}
/**
Add a new Hub Listener, that receives all Hub and OAObject events.
*/
public static void addHubListener(Hub thisHub, HubListener hl) {
getHubListenerTree(thisHub).addListener(hl);
clearGetAllListenerCache(thisHub);
}
public static int TotalHubListeners;
/**
Remove HubListener from list.
*/
protected static void removeHubListener(Hub thisHub, HubListener l) {
if (thisHub == null || l == null) return;
if (thisHub.datau.getListenerTree() == null) return;
thisHub.datau.getListenerTree().removeListener(l);
//was: thisHub.datau.getListenerTree().removeListener(thisHub, l);
clearGetAllListenerCache(thisHub);
}
private final static HubListener[] hlEmpty = new HubListener[0];
/**
Returns list of registered listeners for this Hub only.
*/
protected static HubListener[] getHubListeners(Hub thisHub) {
if (thisHub.datau.getListenerTree() == null) return hlEmpty;
HubListener[] hl = thisHub.datau.getListenerTree().getHubListeners();
if (hl == null) hl = hlEmpty;
return hl;
}
/**
Returns a count of all of the listeners for this Hub and all of Hubs that are shared with it.
*/
public static int getListenerCount(Hub thisHub) {
return getAllListeners(thisHub).length;
}
/**
Returns an array of HubListeners for all of the listeners for this Hub and all of Hubs that are shared with it.
*/
public static HubListener[] getAllListeners(Hub thisHub) {
return getAllListeners(thisHub,0);
}
// 20160606 cache for getAllListeners
static final int maxCacheGetAllListeners = 12;
private static class CacheGetAllListeners {
Hub hub;
HubListener[] hl;
}
private static final ReentrantReadWriteLock rwCacheGetAllListeners = new ReentrantReadWriteLock();
private static final CacheGetAllListeners[] cacheGetAllListeners = new CacheGetAllListeners[maxCacheGetAllListeners];
static {
for (int i=0; i al = _getAllListenersRecursive(thisHub, null, hub, type, false, false);
HubListener[] hl = new HubListener[al==null?0:al.size()];
if (al != null) al.toArray(hl);
return hl;
}
private static ArrayList _getAllListenersRecursive(Hub thisHub, ArrayList al, Hub hub, int type, boolean bHasLastChecked, boolean bHasLast) {
if (type == 0 || type == 2 || thisHub.dataa == hub.dataa) {
HubListener[] hls = getHubListeners(thisHub);
if (hls != null && hls.length > 0) {
int x;
if (al == null) {
al = new ArrayList( Math.max(hls.length*2, 10));
x = 0;
}
else x = al.size();
for (int i=0; i=0; j--) {
HubListener hl2 = (HubListener) al.get(j);
if (hl2.getLocation() == HubListener.InsertLocation.LAST) {
bHasLast = true;
}
else {
if (!bHasLast) al.add(hls[i]);
else al.add(j, hls[i]);
bDone=true;
break;
}
}
if (!bDone) al.add(0, hls[i]); // all were last, need to add to front
bHasLastChecked = true;
}
x++;
}
}
}
WeakReference[] refs = HubShareDelegate.getSharedWeakHubs(thisHub);
for (int i=0; refs != null && i ref = refs[i];
if (ref == null) continue;
Hub h2 = ref.get();
if (h2 == null) continue;
al = _getAllListenersRecursive(h2, al, hub, type, bHasLastChecked, bHasLast);
}
return al;
}
public static void fireAfterLoadEvent(Hub thisHub, OAObject oaObj) {
HubListener[] hl = getAllListeners(thisHub);
int x = hl.length;
int i;
if (x > 0) {
HubEvent hubEvent = new HubEvent(thisHub, oaObj);
try {
OAThreadLocalDelegate.addHubEvent(hubEvent);
for (i=0; i