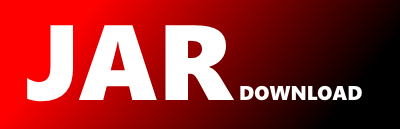
com.viaoa.object.OAObjectKey Maven / Gradle / Ivy
Show all versions of oa-core Show documentation
/* Copyright 1999 Vince Via [email protected]
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.viaoa.object;
import java.io.*;
import com.viaoa.hub.*;
import com.viaoa.ds.*;
/**
Used to represent the objectId properties of an OAObject.
It is used as the key when storing objects in a hashtable.
If the object key property is assigned it will be used, otherwise the guid is used for the hash code.
OAObjectKey overwrites equals() to work the following way:
if guid is equal then objects are equal. If property key is equal,
then objects are equal, unless either of the objects being compared is new.
For more information about this package, see documentation.
*/
public class OAObjectKey implements Serializable, Comparable {
static final long serialVersionUID = 1L;
private Object[] objectIds; // cant be changed, it will affect hashCode
protected int hc = -1;
protected boolean bNew; // if new and objectId is unassigned
protected int guid = 0; // changed to object's guid (if object not used, then it stays as 0)
protected boolean bEmpty;
/*
public OAObjectKey(Object[] ids) {
setIds(ids);
bEmpty = isEmpty();
}
*/
public OAObjectKey(Object... ids) {
setIds(ids);
bEmpty = isEmpty();
}
public OAObjectKey(Object[] ids, int guid, boolean bNew) {
setIds(ids);
this.guid = guid;
this.bNew = bNew;
bEmpty = isEmpty();
}
public OAObjectKey(OAObject obj) {
setIds(OAObjectInfoDelegate.getPropertyIdValues(obj));
guid = obj.guid;
bNew = obj.getNew();
bEmpty = isEmpty();
}
private void setIds(Object[] ids) {
if (ids == null) return;
for (int i=0; i ok.guid) return 1;
return -1;
}
else {
if (ok.bEmpty) {
if (ok.bNew && !this.bNew && this.guid == ok.guid) return 0;
return 1;
}
}
int x = this.objectIds.length;
int x2 = ok.objectIds.length;
for (int j=0; j x) return -1;
return 0;
}
if (bEmpty) return -1;
if (this.objectIds.length == 0) return -1;
if (this.objectIds[0] == null) return -1;
int cmp = compare(objectIds[0], obj);
if (cmp != 0) return cmp;
if (objectIds.length > 1) return 1;
return 0;
}
private int compare(Object obj1, Object obj2) {
if (obj1 == null) {
if (obj2 == null) return 0;
else return -1;
}
if (obj2 == null) return 1;
if (obj1 instanceof Number && obj2 instanceof Number) {
double d1 = ((Number)obj1).doubleValue();
double d2 = ((Number)obj2).doubleValue();
if (d1 == d2) return 0;
if (d1 > d2) return 1;
return -1;
}
String s1, s2;
if (obj1 instanceof String) s1 = (String) obj1;
else s1 = obj1.toString();
if (obj2 instanceof String) s2 = (String) obj2;
else s2 = obj2.toString();
return s1.compareTo(s2);
}
private boolean isEmpty() {
if (objectIds == null) return true;
for (int i=0; i