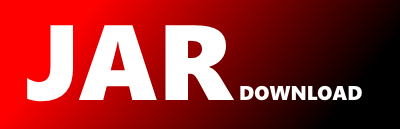
com.viaoa.util.OAJsonWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oa-core Show documentation
Show all versions of oa-core Show documentation
Object Automation library
/* Copyright 1999 Vince Via [email protected]
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.viaoa.util;
import java.util.*;
import java.io.*;
import com.viaoa.hub.*;
import com.viaoa.object.*;
/**
see: 20200127 OAJaxb.java
OAJsonWriter creates an JSON file that can then be read using an OAJsonReader.
If an object has already been stored in the file, then its key will be stored.
@see OAJsonReader
*/
public class OAJsonWriter {
protected PrintWriter pw;
protected StringWriter sw;
public int indent;
private String pad="";
private int indentLast;
public int indentAmount=2;
private boolean bInit;
private String encodeMessage;
private boolean bCompress=true;
private ArrayList alInclude;
private ArrayList alExclude;
protected Hashtable hash; // used for storing objects without an Object Key - updated by OAObject
private Stack
© 2015 - 2025 Weber Informatics LLC | Privacy Policy