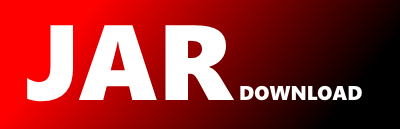
com.viaoa.pojo.PojoLinkOneDelegate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oa-core Show documentation
Show all versions of oa-core Show documentation
Object Automation library
The newest version!
package com.viaoa.pojo;
import java.util.ArrayList;
import java.util.List;
import com.viaoa.util.OAString;
public class PojoLinkOneDelegate {
public static PojoLinkOne getPojoLinkOne(Pojo pojo, String linkName) {
if (pojo == null) {
return null;
}
if (OAString.isEmpty(linkName)) {
return null;
}
List alPjp = new ArrayList<>();
for (PojoLink pl : pojo.getPojoLinks()) {
if (linkName.equalsIgnoreCase(pl.getName())) {
return pl.getPojoLinkOne();
}
}
return null;
}
public static List getLinkFkeyPojoProperties(Pojo pojo, String linkName) {
PojoLinkOne plo = getPojoLinkOne(pojo, linkName);
if (plo == null) {
return null;
}
return getLinkFkeyPojoProperties(plo);
}
public static List getLinkFkeyPojoProperties(final PojoLinkOne plo) {
if (plo == null) {
return null;
}
List alPjp = new ArrayList<>();
for (PojoLinkFkey plfk : plo.getPojoLinkFkeys()) {
alPjp.add(plfk.getPojoProperty());
}
return alPjp;
}
public static List getImportMatchPojoProperties(Pojo pojo, String linkName) {
PojoLinkOne plo = getPojoLinkOne(pojo, linkName);
if (plo == null) {
return null;
}
return getImportMatchPojoProperties(plo);
}
public static List getImportMatchPojoProperties(final PojoLinkOne plo) {
List alPjp = new ArrayList<>();
if (plo == null) {
return alPjp;
}
for (PojoImportMatch pim : plo.getPojoImportMatches()) {
PojoProperty pjp = pim.getPojoProperty();
if (pjp != null) {
alPjp.add(pjp);
} else {
PojoLinkOneReference plor = pim.getPojoLinkOneReference();
if (plor != null) {
PojoLinkOne plox = plor.getPojoLinkOne();
_getLinkOnePojoProperties(plox, alPjp);
}
}
}
return alPjp;
}
public static List getLinkUniquePojoProperties(Pojo pojo, String linkName) {
PojoLinkOne plo = getPojoLinkOne(pojo, linkName);
if (plo == null) {
return null;
}
return getLinkUniquePojoProperties(plo);
}
public static List getLinkUniquePojoProperties(final PojoLinkOne plo) {
List alPjp = new ArrayList<>();
if (plo == null) {
return alPjp;
}
PojoLinkUnique plu = plo.getPojoLinkUnique();
if (plu == null) {
return alPjp;
}
PojoProperty pjp = plu.getPojoProperty();
if (pjp != null) {
alPjp.add(pjp);
} else {
PojoLinkOneReference plor = plu.getPojoLinkOneReference();
if (plor != null) {
PojoLinkOne plox = plor.getPojoLinkOne();
_getLinkOnePojoProperties(plox, alPjp);
}
}
return alPjp;
}
public static List getLinkOnePojoProperties(final PojoLinkOne plo) {
final List alPjp = new ArrayList<>();
if (plo == null) {
return alPjp;
}
_getLinkOnePojoProperties(plo, alPjp);
return alPjp;
}
protected static void _getLinkOnePojoProperties(final PojoLinkOne plo, final List alPjp) {
boolean b = false;
for (PojoLinkFkey plfk : plo.getPojoLinkFkeys()) {
alPjp.add(plfk.getPojoProperty());
b = true;
}
if (b) {
return;
}
for (PojoImportMatch pim : plo.getPojoImportMatches()) {
b = true;
PojoProperty pjp = pim.getPojoProperty();
if (pjp != null) {
alPjp.add(pjp);
} else {
PojoLinkOneReference plor = pim.getPojoLinkOneReference();
if (plor != null) {
PojoLinkOne plox = plor.getPojoLinkOne();
_getLinkOnePojoProperties(plox, alPjp);
}
}
}
if (b) {
return;
}
PojoLinkUnique plu = plo.getPojoLinkUnique();
if (plu != null) {
PojoProperty pjp = plu.getPojoProperty();
if (pjp != null) {
alPjp.add(pjp);
} else {
PojoLinkOneReference plor = plu.getPojoLinkOneReference();
if (plor != null) {
PojoLinkOne plox = plor.getPojoLinkOne();
_getLinkOnePojoProperties(plox, alPjp);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy