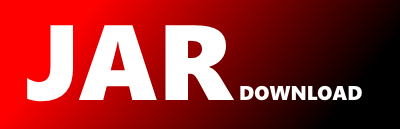
com.viaoa.remote.rest.annotation.OARestMethod Maven / Gradle / Ivy
Show all versions of oa-core Show documentation
/* Copyright 1999 Vince Via [email protected]
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.viaoa.remote.rest.annotation;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* Remoting information about remote methods using HTTP(S).
*
* Important: this annotation needs to be added to the Java Interface, not the Impl class.
*
* @author vvia
*/
@Documented
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface OARestMethod {
MethodType methodType() default MethodType.Unassigned;
String name() default "";
/**
* URL path for endpoint. If it does not include "://", then the OARestClient.baseUrl will be used as prefix.
*
* Supports path template names, example: "/emp/{id}/{name}"
* tags (case insensitive) are filled in from method params with paramType==UrlPathValue, and must have the @RestParam.name defined to
* match name used in template.
*
* Used by non-OA* method types
*
* Supports path template {name}, example: "/emp/{id}"
* Supports path template ?tags, example: "/emp/?/?"
* ? tags will use method params with paramType==UrlPathValue, to fill in from left to right.
*
* verify: if tags names, that there are matching param(s) with matching name for all {x} tags
* verify: if ?tags, that there are matching paramType==UrlPathValue
* verify: cant mix {} and ? tags
*/
String urlPath() default "";
/**
* http query string.
*
* verify: ignores leading '?'
*/
String urlQuery() default "";
/**
* search Query to use, with ?tags from param(s) with type=SearchWhereTagValue.
*
* only used when methodType=OASearch
*
* verify: query ?tags having mathcing params with type=SearchWhereTagValue
*/
String searchWhere() default "";
/**
* Search Query OrderBy to use.
*
* only used when methodType=OASearch
*
* verify: RestMethod.queryWhere is not empty, and param type=MethodQueryOrderBy is not empty
*/
String searchOrderBy() default "";
/**
* PropertyPath to include in OAGraph result.
*
* only used when methodType=OA*
*/
String includePropertyPath() default "";
/**
* PropertyPath to include in OAGraph result.
*
* only used when methodType=OA*
*/
String[] includePropertyPaths() default {}; // PP to include in result, supported by OARestServlet
/**
* Number of reference levels to include in OAGraph result.
*
* only used when methodType=OA*
*/
int includeReferenceLevelAmount() default 0;
/**
* The method name for remote method calls.
*
* only used by methodType=OAObjectMethodCall, or methodType=OARemote
*/
String methodName() default "";
/**
* value used as the page size for returns that are collection of values (zero or more).
* A value <= 0 will use the servers default pagesize.
*
* This will be added as an http query value "pageSize"
*
* verify: only needed when return value is array,List,Hub
* verify: pageNumber is required if pageSize>0
*/
int pageSize() default 0;
/**
* Type of return class. This is used/needed when it can't be determined what the actual return class is.
* For example: if using a List or Hub and the actual objects can not be discovered using generics.
*
* Note: generics are able to be discovered for return values (not affected by generics erasure).
* Note: calling OARestMethodInfo.verify() will check to see if returnClass is needed or not.
*
*/
Class returnClass() default Void.class;
/**
* The type of method, that define how it will use HTTP(S) to call the remote server.
*
* @author vvia
*/
public static enum MethodType {
/**
* Unassigned/not set, which will produce an error.
*
* verify: it should throw an exception
*/
Unassigned,
/**
* Uses http GET.
*
* required:
* urlPath or param type=MethodUrlPath,
*
* valid method annotations:
* urlPath, urlQuery, pageSize
*
* valid param annotation types:
* MethodUrlPath, UrlPathTagValue, UrlQueryNameValue, MethodReturnClass, OARestInvokeInfo, BodyObject, BodyJson, Header, Cookie,
* PageNumber
*/
GET(),
/**
* Uses http GET to get an object from OAGraph using an object Id.
*
* automatically adds the default url for OARestServlet.
*
* required:
* method signature must return a subclass of OAObject
* must have a param type=OAObjectId
* OARestServlet on server.
*
* derives: urlPath as "/customer/{id}[/{id2}..]"
* using the return class name, and the value(s) from param with type=OAObjectId
*
* valid method annotations:
* includePropertyPath, includePropertyPaths, includeReferenceLevelAmount
*
* valid param annotation types:
* OAObjectId (required),
* ResponseIncludePropertyPaths
* OARestInvokeInfo, Header, Cookie,
*/
OAGet(false),
/**
* Uses http POST to query OAGraph objects.
*
* required:
* method signature must return a collection (array,list,hub) of objects that are a subclass of OAObject
* OARestServlet on server.
*
* Supports using "?tags" as variable holders in query, to be matched with value(s) from params with type=SearchWhereTagValue
* Supports param(s) of type = SearchWhereAddNameValue, that will be added to query. Note: value can be an array, which will add to
* the search "(.. OR ..)" using the values.
*
* valid method annotations:
* searchWhere, searchOrderBy,
* pageSize,
* includePropertyPath(s), includeReferenceLevelAmount,
* returnClass
*
* valid param annotation types:
* SearchWhereTagValue,
* SearchWhereAddNameValue,
* PageNumber,
* ResponseIncludePropertyPaths,
* MethodSearchWhere, MethodSearchOrderBy,
* UrlQueryNameValue, MethodReturnClass, Header, Cookie,
*/
OASearch(false),
/**
* Uses http POST
*
* http Body will use param values of type=FormNameValue or BodyObject or BodyJson.
*
* required:
* urlPath or param type=MethodUrlPath
*
* valid method annotations:
* urlPath, urlQuery, pageSize
*
* valid param annotation types:
* UrlPathTagValue, UrlQueryNameValue,
* FormNameValue, BodyObject, BodyJson,
* MethodUrlPath, Header, Cookie, PageNumber, OARestInvokeInfo, MethodReturnClass
*/
POST,
/**
* Use http PUT
*
* see: POST
*/
PUT,
/**
* Use http PATCH
*
* see: POST
*/
PATCH,
/**
* Use http POST to call OARestServlet to call a method on an OAObject.
*
* required:
* methodName
* must have a param type=OAObject
* OARestServlet on server.
*
* derives: urlPath and query params required by OARestServlet to make the remote method call
*
* valid method annotations:
* methodName,
* includePropertyPath(s), includeReferenceLevelAmount, returnClass
*
* valid param annotation types:
* OAObject, MethodCallArg,
* OARestInvokeInfo, ResponseIncludePropertyPaths,
* Header, Cookie, PageNumber
*/
OAObjectMethodCall(false),
/**
* Used internally when calling methods on a remote object, that get invoked on server running OARestServlet.
*
* required:
* OARestServlet on server.
* registered object on server, using OARestServlet.register(). This is the implementation of the Java interface that was called on
* the client computer.
*
*/
OARemote(false),
/**
* Uses http PUT to call OARestServlet to insert a new OAObject.
*
* required:
* must have a param type=OAObject
* OARestServlet on server.
*
* derives: urlPath
*
* valid method annotations:
* includePropertyPath(s), includeReferenceLevelAmount
*
* valid param annotation types:
* OAObject (required),
* OARestInvokeInfo, Header, Cookie, PageNumber, ResponseIncludePropertyPaths
*/
OAInsert(false),
/**
* Uses http POST to call OARestServlet to update an existing OAObject.
*
* required:
* must have a param type=OAObject
* OARestServlet on server.
*
* derives: urlPath
*
* valid method annotations:
* includePropertyPath(s), includeReferenceLevelAmount
*
* valid param annotation types:
* OAObject (required),
* OARestInvokeInfo, Header, Cookie, PageNumber, ResponseIncludePropertyPaths
*/
OAUpdate(false),
/**
* Uses http DELETE to delete an object from OAGraph.
*
* automatically adds the default url for OARestServlet.
*
* required:
* must have a param type=OAObject
* OARestServlet on server.
*
* derives: urlPath
*
* valid method annotations:
* includePropertyPath(s), includePropertyPaths, includeReferenceLevelAmount
*
* valid param annotation types:
* OAObject (required),
* OARestInvokeInfo, Header, Cookie
*/
OADelete(false);
public boolean requiresUrlPath = true;
MethodType() {
}
MethodType(boolean requiresUrlPath) {
this.requiresUrlPath = requiresUrlPath;
}
boolean isOA() {
String s = this.toString();
return "OA".equals(s);
}
}
}