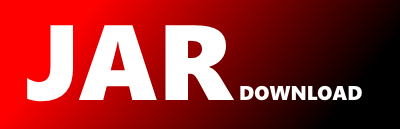
com.viaoa.util.OAMail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oa-core Show documentation
Show all versions of oa-core Show documentation
Object Automation library
The newest version!
/* Copyright 1999 Vince Via [email protected]
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.viaoa.util;
import java.util.Properties;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.mail.*;
import javax.mail.internet.*;
import javax.mail.util.ByteArrayDataSource;
public class OAMail implements java.io.Serializable {
private static final long serialVersionUID = 1L;
private String host;
private int port;
private String userId;
private String password;
private boolean bDebug;
private boolean bUseSSL;
public static class OAMailAttachment {
public byte[] bsData;
public String mimeType;
public String fileName;
}
/**
* Used to send emails.
* @param host
* @param port if < 1, then it will use default 25
* @param user user id for mail server
* @param pw user password for mail server
*/
public OAMail(String host, String user, String pw) {
this.host = host;
this.port = 25;
this.userId = user;
this.password = pw;
}
public OAMail(String host, int port, String user, String pw) {
this.host = host;
this.port = port;
this.userId = user;
this.password = pw;
}
public void setUseSSL(boolean b) {
bUseSSL = b;
}
public boolean getUsesSSL() {
return bUseSSL;
}
public void setDebug(boolean b) {
this.bDebug = b;
}
public void sendSmtp(
String to, String cc, String from,
String subject, String text,
String contentType,
String[] fileNames) throws Exception
{
sendSmtp(new String[] {to}, new String[] {cc}, from, subject, text, contentType, fileNames);
}
public void sendSmtp(
String to, String cc, String from,
String subject, String text,
String contentType,
byte[] bsAttachment, String mimeTypeBs, String bsFileName) throws Exception
{
sendSmtp(new String[] {to}, new String[] {cc}, from, subject, text, contentType, null, bsAttachment, mimeTypeBs, bsFileName);
}
/**
*
* @param to
* @param cc
* @param from
* @param subject
* @param text
* @param contentType if null or blank, then will default to "text/html; charset=UTF-8"
* , others: "text/html", "text/plain", "text/richtext", "text/css", "image/gif"
* @param fileNames
* @throws Exception
*/
public void sendSmtp(
String[] to, String[] cc, String from,
String subject, String text,
String contentType,
String[] fileNames) throws Exception
{
sendSmtp(to, cc, from, subject, text, contentType, fileNames, null, null, null);
}
public void sendSmtp(
String[] to, String[] cc, String from,
String subject, String text,
String contentType
) throws Exception
{
sendSmtp(to, cc, from, subject, text, contentType, null, null, null, null);
}
public void sendSmtp(
String[] to, String[] cc, String from,
String subject, String text,
String contentType,
byte[] bsAttachment, String mimeTypeBs, String bsFileName) throws Exception
{
sendSmtp(to, cc, from, subject, text, contentType, null, bsAttachment, mimeTypeBs, bsFileName);
}
public void sendSmtp(
String[] to, String[] cc, String from,
String subject, String text,
String contentType,
String[] fileNames, byte[] bsAttachment, String mimeTypeBs, String bsFileName) throws Exception
{
OAMailAttachment[] mas = null;
if (bsAttachment != null) {
mas = new OAMailAttachment[1];
OAMailAttachment ma = new OAMailAttachment();
ma.bsData = bsAttachment;
ma.fileName= bsFileName;
ma.mimeType = mimeTypeBs;
mas[0] = ma;
}
sendSmtp(to, cc, from, subject, text, contentType, fileNames, mas);
}
public void sendSmtp(
String[] to, String[] cc, String from,
String subject, String text,
String contentType,
String[] fileNames, OAMailAttachment[] attachments) throws Exception
{
String msg = "";
if (from == null) from = "";
Transport transport = null;
if (port < 1) port = 25;
if (contentType == null || contentType.length() == 0) {
// contentType = "text/html; charset=iso-8859-1";
contentType = "text/html; charset=UTF-8";
}
// create some properties and get the default Session
Properties props = System.getProperties();
props.put("mail.smtp.auth", "true"); // required
//props.put("mail.smtp.host", host);
// if (port > 0) props.put("mail.smtp.port", port+""); // default 25
// props.put("mail.smtp.user", "test.com@test-smtp");
// props.put("mail.smtp.password", "testPw");
// props.setProperty ("mail.transport.protocol", "smtp");
// props.setProperty("mail.smtp.starttls.enable", "true");
// see: http://javamail.kenai.com/nonav/javadocs/com/sun/mail/smtp/package-summary.html
/*
Authenticator auth = new Authenticator() {
public PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("test.com@test-smtp", "testPw");
}
};
*/
Session session = Session.getInstance(System.getProperties());
// Session session = Session.getDefaultInstance(System.getProperties(), null);
// Session session = Session.getInstance(System.getProperties(), auth);
session.setDebug(bDebug);
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(from));
for (int i=0; to != null && iThis is another email from the test
, with an attachment