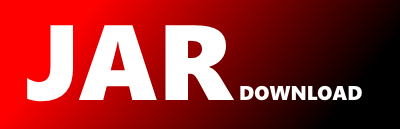
com.viaoa.util.OATimeZone Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oa-core Show documentation
Show all versions of oa-core Show documentation
Object Automation library
The newest version!
package com.viaoa.util;
import java.util.*;
import java.util.concurrent.*;
/**
* Helper for building timezone list, display and lookups.
*
* @author vvia
*/
public class OATimeZone {
private static volatile ArrayList alTZ;
private static String[] shortNames;
private static TimeZone tzUTC;
public static final String TZ_Eastern = "America/New_York";
public static final String TZ_NewYork = "America/New_York"; // Eastern
public static final String TZ_Central = "America/Chicago"; // Central
public static final String TZ_Chicago = "America/Chicago"; // Central
public static final String TZ_Mountain = "America/Phoenix";
public static final String TZ_Phoenix = "America/Phoenix"; // Mountain
public static final String TZ_Pacific = "America/Los_Angeles";
public static final String TZ_LosAngeles = "America/Los_Angeles"; // Pacific
public static final String TZ_Anchorage = "America/Anchorage";
public static final String TZ_London = "Europe/London";
public static final String TZ_Tokyo = "Asia/Tokyo";
public static final String TZ_HongKong = "Asia/Hong_Kong";
public static final String TZ_GMT = "GMT";
public static final String TZ_Zulu = "Zulu"; // UTC-00
// public static final String TZ_ = "";
public static final String TZ_UTC = "UTC";
public static class TZ {
public String id;
public String utcValue;
public String shortName;
public String longName;
public TimeZone timeZone;
public String getDisplay() {
return "(" + utcValue + ") " + id + " (" + longName + "/" + shortName + ")";
}
}
public static TimeZone getTimeZoneUTC() {
if (tzUTC == null) {
tzUTC = TimeZone.getTimeZone("UTC");
}
return tzUTC;
}
public static TZ getLocalOATimeZone() {
TimeZone timeZone = TimeZone.getDefault();
TZ tz = getOATimeZone(timeZone);
return tz;
}
public static TimeZone getLocalTimeZone() {
TimeZone timeZone = TimeZone.getDefault();
return timeZone;
}
public static String[] getShortNames() {
if (shortNames != null) return shortNames;
List al = new ArrayList();
Set set = new HashSet();
for (TZ tz : getOATimeZones()) {
if (!set.contains(tz.shortName)) {
set.add(tz.shortName);
al.add(tz.shortName);
}
}
al.sort(new Comparator() {
@Override
public int compare(String o1, String o2) {
return OAStr.compare(o1, o2);
}
});
String[] ss = new String[al.size()];
al.toArray(ss);
shortNames = ss;
return shortNames;
}
private static final Object lockTimeZones = new Object();
private static long msNextUpdate = 0;
public static ArrayList getOATimeZones() {
if (alTZ == null || msNextUpdate < System.currentTimeMillis()) {
synchronized (lockTimeZones) {
if (alTZ == null) {
alTZ = _getOATimeZones();
OADate d = new OADate();
d = (OADate) d.addDay();
msNextUpdate = d.getTime();
}
}
}
return alTZ;
}
protected static ArrayList _getOATimeZones() {
ArrayList alTZ = new ArrayList<>();
String[] tzs = TimeZone.getAvailableIDs();
final ArrayList al = new ArrayList<>();
for (String s : tzs) {
TimeZone tz = TimeZone.getTimeZone(s);
al.add(tz);
}
Collections.sort(al, (o1, o2) -> {
int x1 = o1.getRawOffset();
int x2 = o2.getRawOffset();
if (x1 == x2) {
return 0;
}
if (x1 > x2) {
return 1;
}
return -1;
});
for (TimeZone timeZone : al) {
long hours = TimeUnit.MILLISECONDS.toHours(timeZone.getRawOffset());
long minutes = TimeUnit.MILLISECONDS.toMinutes(timeZone.getRawOffset()) - TimeUnit.HOURS.toMinutes(hours);
// avoid -4:-30 issue
minutes = Math.abs(minutes);
String utcValue = "";
if (minutes == 0) {
if (hours > 0) {
utcValue = String.format("UTC+%02d", hours);
} else {
utcValue = String.format("UTC-%02d", Math.abs(hours));
}
} else {
if (hours > 0) {
utcValue = String.format("UTC+%02d:%02d", hours, minutes);
} else {
utcValue = String.format("UTC-%02d:%02d", Math.abs(hours), minutes);
}
}
String shortName = timeZone.getDisplayName(timeZone.useDaylightTime(), timeZone.SHORT, Locale.getDefault());
String longName = timeZone.getDisplayName();
TZ tz = new TZ();
tz.id = timeZone.getID();
tz.shortName = shortName;
tz.longName = longName;
tz.utcValue = utcValue;
tz.timeZone = timeZone;
alTZ.add(tz);
}
return alTZ;
}
/**
* Find the java TimeZone.
*
* @param value can be the tz.id, display name, short name, or long name.
*/
public static TimeZone getTimeZone(final String value) {
if (OAString.isEmpty(value)) {
return TimeZone.getDefault();
}
TimeZone timeZone = TimeZone.getTimeZone(value);
if (timeZone != null) {
return timeZone;
}
for (TZ tz : getOATimeZones()) {
if (value.equalsIgnoreCase(tz.id) || value.equalsIgnoreCase(tz.utcValue) || value.equalsIgnoreCase(tz.shortName)
|| value.equalsIgnoreCase(tz.longName) || value.equalsIgnoreCase(tz.getDisplay())) {
timeZone = TimeZone.getTimeZone(tz.id);
if (timeZone != null) {
return timeZone;
}
}
}
return null;
}
public static TZ getOATimeZone(TimeZone timeZone) {
if (timeZone == null) {
return null;
}
final String id = timeZone.getID();
for (TZ tz : getOATimeZones()) {
if (id.equalsIgnoreCase(tz.id)) {
return tz;
}
}
return null;
}
/**
*
* @param value number from UTC, 0 to +14, and -12 to 0
* @return
*/
public static TZ getUtcTimeZone(int value) {
String s = "UTC" + (value > 0 ? "+" : "-") + String.format("%02d", Math.abs(value));
for (TZ tz : getOATimeZones()) {
if (s.equalsIgnoreCase(tz.utcValue)) {
return tz;
}
}
return null;
}
private static ArrayList alTZhold;
private static Map hmTZbyId = new ConcurrentHashMap();
public static TimeZone getTimeZoneById(String id) {
if (alTZhold != alTZ) {
hmTZbyId.clear();
alTZhold = alTZ;
}
if (OAString.isEmpty(id)) {
id = TimeZone.getDefault().getID();
}
TZ tzx = hmTZbyId.get(id);
if (tzx != null) return tzx.timeZone;
for (TZ tz : getOATimeZones()) {
if (id.equalsIgnoreCase(tz.id)) {
hmTZbyId.put(id, tz);
return tz.timeZone;
}
}
return null;
}
public static TZ getOATimeZone(String value) {
if (OAString.isEmpty(value)) {
value = TimeZone.getDefault().getID();
}
for (TZ tz : getOATimeZones()) {
if (value.equalsIgnoreCase(tz.id) || value.equalsIgnoreCase(tz.utcValue) || value.equalsIgnoreCase(tz.shortName)
|| value.equalsIgnoreCase(tz.longName) || value.equalsIgnoreCase(tz.getDisplay())) {
return tz;
}
}
return null;
}
public static void main(String[] args) {
{
OATimeZone.TZ tz = OATimeZone.getOATimeZone("America/Chicago");
OADateTime dtNow = (new OADateTime()).convertTo(tz);
OADate dToday = new OADate( dtNow.convertTo(tz) );
int i = 0;
i++;
}
int i = 0;
for (TZ tz : getOATimeZones()) {
System.out.println((++i) + ") " + tz.getDisplay());
}
final OATimeZone.TZ tzz = OATimeZone.getOATimeZone("America/Chicago");
final OADateTime dtNowz = (new OADateTime()).convertTo(tzz);
TZ tz1 = getOATimeZone("UTC-06");
TZ tz2 = getOATimeZone("CST");
TZ tz3 = getOATimeZone("CDT");
OATimeZone.TZ tz = OATimeZone.getOATimeZone("America/Chicago");
OADateTime dtNow = new OADateTime();
OADateTime dtNowCST = dtNow.convertTo(tz);
OADateTime dtx = dtNow.convertTo(tz1);
dtx = dtNow.convertTo(tz2);
dtx = dtNow.convertTo(tz3);
int xx = 4;
xx++;
//System.out.println(tz.getDisplay());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy