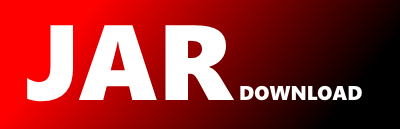
com.viaoa.remote.rest.annotation.OARestParam Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oa-core Show documentation
Show all versions of oa-core Show documentation
Object Automation library
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy