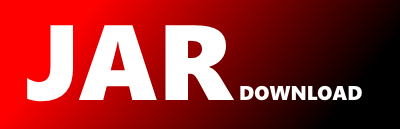
com.viiyue.plugins.mybatis.annotation.member.Conditional Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-mapper Show documentation
Show all versions of mybatis-mapper Show documentation
Mybatis generic mapper plugin for solving most basic operations,
simplifying sql syntax and improving dynamic execution efficiency
/**
* Copyright (C) 2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.viiyue.plugins.mybatis.annotation.member;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import com.viiyue.plugins.mybatis.Constants;
import com.viiyue.plugins.mybatis.enums.Template;
import com.viiyue.plugins.mybatis.enums.ValueStyle;
import com.viiyue.plugins.mybatis.template.builder.ColumnBuilder;
import com.viiyue.plugins.mybatis.template.function.BlankFunction;
import com.viiyue.plugins.mybatis.template.function.EqualFunction;
import com.viiyue.plugins.mybatis.template.function.NotBlankFunction;
import com.viiyue.plugins.mybatis.template.function.NotNullFunction;
import com.viiyue.plugins.mybatis.template.function.NullFunction;
/**
* Dynamic conditional judgment annotation
*
* @author tangxbai
* @since 1.1.0
*/
@Documented
@Target({ ElementType.FIELD, ElementType.METHOD })
@Retention( RetentionPolicy.RUNTIME )
public @interface Conditional {
/**
* Conditional judgment expression for dynamic value
*
* 1. {@link BlankFunction} - isBlank( Object )
*
* - isBlank( null ) -> true
* - isBlank( "" ) -> true
* - isBlank( " " ) -> true
* - isBlank( "\n\t\s" ) -> true
* - isBlank( "a" ) -> false
* - isBlank( "a b" ) -> false
*
*
* 2. {@link NotBlankFunction} - isNotBlank( Object )
*
* - isNotBlank( null ) -> false
* - isNotBlank( "" ) -> false
* - isNotBlank( " " ) -> false
* - isNotBlank( "\n\t\s" ) -> false
* - isNotBlank( "a" ) -> true
* - isNotBlank( "a b" ) -> true
*
*
* 3. {@link EqualFunction} - equals( Object, Object )
*
* - equals( null, null ) -> true
* - equals( "foo", null ) -> false
* - equals( null, "foo" ) -> false
* - equals( "foo", "foo" ) -> true
*
*
* 4. {@link NullFunction} - isNull( Object )
*
* - isNull( null ) -> true
* - isNull( [others] ) -> false
*
*
* 5. {@link NotNullFunction} - isNotNull( Object )
*
* - isNotNull( null ) -> false
* - isNotNull( [others] ) -> true
*
*
*/
String value() default Constants.DEFAULT_CONDITIONAL;
/**
* Conditional expression holder, default is "{@code =}".
* For example : column = #{property}
*/
Holder holder();
/**
* Conditional judgment expression operator holder
*
* @author tangxbai
* @since 1.1.0
* @see Template
*/
enum Holder {
/** @see Template#IsNull */
IsNull( Template.IsNull ),
/** @see Template#NotNull */
NotNull( Template.NotNull ),
/** @see Template#Equal */
Equal( Template.Equal ),
/** @see Template#NotEqual */
NotEqual( Template.NotEqual ),
/** @see Template#Like */
Like( Template.Like ),
/** @see Template#NotLike */
NotLike( Template.NotLike ),
/** @see Template#StartsWith */
StartsWith( Template.StartsWith ),
/** @see Template#EndsWith */
EndsWith( Template.EndsWith ),
/** @see Template#Regexp */
Regexp( Template.Regexp ),
/** @see Template#NotRegexp */
NotRegexp( Template.NotRegexp ),
/** @see Template#GreaterThan */
GreaterThan( Template.GreaterThan ),
/** @see Template#GreaterThanAndEqualTo */
GreaterThanAndEqualTo( Template.GreaterThanAndEqualTo ),
/** @see Template#LessThan */
LessThan( Template.LessThan ),
/** @see Template#LessThanAndEqualTo */
LessThanAndEqualTo( Template.LessThanAndEqualTo );
private Template template;
private Holder( Template template ) {
this.template = template;
}
public String format( ColumnBuilder column, ValueStyle valueStyle ) {
return template.format( column, valueStyle );
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy