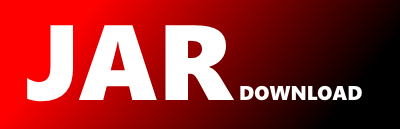
com.viiyue.plugins.mybatis.condition.WhereExample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-mapper Show documentation
Show all versions of mybatis-mapper Show documentation
Mybatis generic mapper plugin for solving most basic operations,
simplifying sql syntax and improving dynamic execution efficiency
/**
* Copyright (C) 2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.viiyue.plugins.mybatis.condition;
import static com.viiyue.plugins.mybatis.Constants.MYBATIS_PARAMETER_PATTERN;
import static com.viiyue.plugins.mybatis.Constants.SEPARATOR;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import com.viiyue.plugins.mybatis.Constants;
import com.viiyue.plugins.mybatis.enums.Template;
import com.viiyue.plugins.mybatis.enums.ValueStyle;
import com.viiyue.plugins.mybatis.metadata.Entity;
import com.viiyue.plugins.mybatis.metadata.Property;
import com.viiyue.plugins.mybatis.metadata.info.LogicallyDeleteInfo;
import com.viiyue.plugins.mybatis.metadata.info.OrderByInfo;
import com.viiyue.plugins.mybatis.template.TemplateHandler;
import com.viiyue.plugins.mybatis.template.builder.ColumnBuilder;
import com.viiyue.plugins.mybatis.template.handler.KeywordsHandler;
import com.viiyue.plugins.mybatis.utils.Assert;
import com.viiyue.plugins.mybatis.utils.ObjectUtil;
import com.viiyue.plugins.mybatis.utils.StringAppender;
import com.viiyue.plugins.mybatis.utils.StringUtil;
/**
* Example query condition construction
*
* @author tangxbai
* @since 1.0.0
*/
public final class WhereExample> extends AbstractExample {
private final T example;
private final ColumnBuilder column;
private final StringAppender condition = new StringAppender();
private final Set queryProperties = new HashSet( 16 );
private final Set orderByProperties = new HashSet( 8 );
private final Set groupByProperties = new HashSet( 8 );
private String delimiter;
protected WhereExample( Entity entity ) {
this( null, entity );
}
protected WhereExample( T example ) {
this( example, example == null ? null : example.getEntity() );
}
private WhereExample( T example, Entity entity ) {
super( entity );
Assert.isTrue( example != null || entity != null, "Example and Entity cannot be null at the same time" );
this.example = example;
this.column = new ColumnBuilder( entity );
}
protected Set getQueryProperties( Set merge ) {
return new HashSet( this.queryProperties );
}
protected Set getOrderByProperties() {
return new HashSet( this.orderByProperties );
}
protected Set getGroupByProperties() {
return new HashSet( this.groupByProperties );
}
protected Map getOriginalParameters() {
return super.getParameters();
}
private WhereExample join( String delimiter ) {
this.delimiter = delimiter;
return this;
}
public WhereExample and() {
return join( " [and] " );
}
public WhereExample or() {
return join( " [or] " );
}
public WhereExample isNull( String propertyName ) {
return byTemplate( Template.IsNull, propertyName );
}
public WhereExample notNull( String propertyName ) {
return byTemplate( Template.NotNull, propertyName );
}
public WhereExample lt( String propertyName, Object value ) {
return lessThan( propertyName, value );
}
public WhereExample lessThan( String propertyName, Object value ) {
return byTemplate( Template.LessThan, propertyName, value );
}
public WhereExample lte( String propertyName, Object value ) {
return lessThanAndEqualTo( propertyName, value );
}
public WhereExample lessThanAndEqualTo( String propertyName, Object value ) {
return byTemplate( Template.LessThanAndEqualTo, propertyName, value );
}
public WhereExample gt( String propertyName, Object value ) {
return greaterThan( propertyName, value );
}
public WhereExample greaterThan( String propertyName, Object value ) {
return byTemplate( Template.GreaterThan, propertyName, value );
}
public WhereExample gte( String propertyName, Object value ) {
return greaterThanAndEqualTo( propertyName, value );
}
public WhereExample greaterThanAndEqualTo( String propertyName, Object value ) {
return byTemplate( Template.GreaterThanAndEqualTo, propertyName, value );
}
public WhereExample eq( String propertyName, Object value ) {
return equal( propertyName, value );
}
public WhereExample equal( String propertyName, Object value ) {
return byTemplate( Template.Equal, propertyName, value );
}
public WhereExample neq( String propertyName, Object value ) {
return notEqual( propertyName, value );
}
public WhereExample notEqual( String propertyName, Object value ) {
return byTemplate( Template.NotEqual, propertyName, value );
}
public WhereExample like( String propertyName, String value ) {
return byTemplate( Template.Like, propertyName, value );
}
public WhereExample contains( String propertyName, String value ) {
return byTemplate( Template.Like, propertyName, value );
}
public WhereExample startsWith( String propertyName, String value ) {
return byTemplate( Template.StartsWith, propertyName, value );
}
public WhereExample notLike( String propertyName, String value ) {
return byTemplate( Template.NotLike, propertyName, value );
}
public WhereExample notContains( String propertyName, String value ) {
return byTemplate( Template.NotLike, propertyName, value );
}
public WhereExample endsWith( String propertyName, String value ) {
return byTemplate( Template.EndsWith, propertyName, value );
}
public WhereExample regexp( String propertyName, String value ) {
return byTemplate( Template.Regexp, propertyName, value );
}
public WhereExample notRegexp( String propertyName, String value ) {
return byTemplate( Template.NotRegexp, propertyName, value );
}
public WhereExample between( String propertyName, Object value1, Object value2 ) {
return byTemplate( Template.Between, propertyName, value1, value2 );
}
public WhereExample notBetween( String propertyName, Object value1, Object value2 ) {
return byTemplate( Template.NotBetween, propertyName, value1, value2 );
}
public WhereExample inRange( String propertyName, Object value1, Object value2 ) {
return byTemplate( Template.InRange, propertyName, value1, value2 );
}
public WhereExample overRange( String propertyName, Object value1, Object value2 ) {
return byTemplate( Template.OverRange, propertyName, value1, value2 );
}
public WhereExample in( String propertyName, Object ... values ) {
return byTemplate( Template.In, propertyName, values );
}
public WhereExample in( String propertyName, List> values ) {
return byTemplate( Template.In, propertyName, values.toArray() );
}
public WhereExample notIn( String propertyName, Object ... values ) {
return byTemplate( Template.NotIn, propertyName, values );
}
public WhereExample notIn( String propertyName, List> values ) {
return byTemplate( Template.NotIn, propertyName, values.toArray() );
}
public WhereExample byTemplate( String templateName, String propertyName, Object ... values ) {
return byTemplate( Template.findOf( templateName ), propertyName, values );
}
public WhereExample byTemplate( Template template, String propertyName, Object ... values ) {
Assert.notNull( template, "Example template cannot be null" );
Property property = getProperty( propertyName );
ValueStyle valueStyle = getEntity().getValueStyle();
this.queryProperties.add( propertyName );
this.column.apply( property ).alias( parmeterAlias );
this.condition.addDelimiter( delimiter );
this.condition.append( template.format( column, valueStyle ) );
if ( template.isNeedParameter() ) {
for ( int i = 0, size = values.length; i < size; i ++ ) {
putParameter( property.getName() + ( size > 1 ? i : Constants.EMPTY ), values[ i ] );
}
}
return and();
}
public WhereExample condition( String condition, Object ... values ) {
Assert.notBlank( condition, "Example custom condition cannot be null or blank text" );
this.condition.addDelimiter( delimiter );
if ( ObjectUtil.isNotEmpty( values ) ) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy