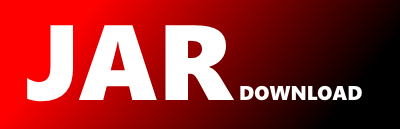
com.viiyue.plugins.mybatis.mapper.base.BaseExampleMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-mapper Show documentation
Show all versions of mybatis-mapper Show documentation
Mybatis generic mapper plugin for solving most basic operations,
simplifying sql syntax and improving dynamic execution efficiency
/**
* Copyright (C) 2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.viiyue.plugins.mybatis.mapper.base;
import java.io.Serializable;
import java.util.List;
import org.apache.ibatis.annotations.DeleteProvider;
import org.apache.ibatis.annotations.SelectProvider;
import org.apache.ibatis.annotations.UpdateProvider;
import com.viiyue.plugins.mybatis.annotation.mark.EnableResultMap;
import com.viiyue.plugins.mybatis.condition.Example;
import com.viiyue.plugins.mybatis.condition.SelectExample;
import com.viiyue.plugins.mybatis.condition.UpdateExample;
import com.viiyue.plugins.mybatis.condition.WhereExample;
import com.viiyue.plugins.mybatis.mapper.Marker;
import com.viiyue.plugins.mybatis.mapper.special.LogicallyDeleteMapper;
import com.viiyue.plugins.mybatis.provider.DynamicProvider;
import com.viiyue.plugins.mybatis.provider.base.BaseExampleProvider;
/**
* Basic example api method interface definition
*
* @author tangxbai
* @since 1.0.0
*
* @param database entity type
* @param query data return entity type
* @param primary key type, must be a {@link Serializable} implementation class.
*/
public interface BaseExampleMapper extends Marker {
/**
* Delete qualified data through the Example object
*
*
* Example example = Example.query( Bean.class )
* .gt( "total", 10 )
* .lt( "time", new Date() )
* .like( "name", "mybatis-mapper" )
* .startsWith( "name", "mybatis-" )
* .xxx();
* int rows = deleteByExample( example );
*
*
* Note: This operation is irreversible and belongs to physical
* deletion. If you want to operate the data safely, please use the
* {@link LogicallyDeleteMapper#logicallyDeleteByExample(Example)
* logicallyDeleteByExample(Example)} method instead.
*
*
* @param example the example object
* @return the number of rows affected
* @see WhereExample
*/
@DeleteProvider( type = BaseExampleProvider.class, method = DynamicProvider.dynamicSQL )
int deleteByExample( WhereExample example );
/**
* Modify data with the Example object
*
*
* Example example = Example.update( Bean.class );
*
* // 1. Direct setting
* example.set( "property1", "property2", "property3", ... ).values( "value1", "value2", "value3", ... );
* // 2. Binding object
* example.bind( Bean );
*
* // Use conditional query chain
* example.when()
* .gt( "total", 10 )
* .lt( "time", new Date() )
* .like( "name", "mybatis-mapper" )
* .startsWith( "name", "mybatis-" )
* .xxx();
*
* int rows = updateByExample( example );
*
*
* @param example the example object
* @return the number of rows affected
* @see UpdateExample
*/
@UpdateProvider( type = BaseExampleProvider.class, method = DynamicProvider.dynamicSQL )
int updateByExample( Example example );
/**
* Query the list of qualified data through the Example object
*
*
* Example example = null;
*
* // 1. Specify statistical fields
* example = Example.select( Bean.class )
* .includes( "id", "name", ... )
* .excludes( "name", ... )
* .when()
* .gt( "total", 10 )
* .lt( "time", new Date() )
* .like( "name", "mybatis-mapper" )
* .startsWith( "name", "mybatis-" )
* .xxx();
*
* // 2. Or directly query all columns
* example = Example.query( Bean.class );
* .gt( "total", 10 )
* .lt( "time", new Date() )
* .like( "name", "mybatis-mapper" )
* .startsWith( "name", "mybatis-" )
* .xxx();
*
* // Get statistical results
* List<DTO> results = selectByExample( example );
*
*
* @param example the example object
* @return the result list
* @see SelectExample
* @see WhereExample
*/
@EnableResultMap
@SelectProvider( type = BaseExampleProvider.class, method = DynamicProvider.dynamicSQL )
List selectByExample( Example example );
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy